How to sanitize phone numbers before sending mass alerts
Time to read:
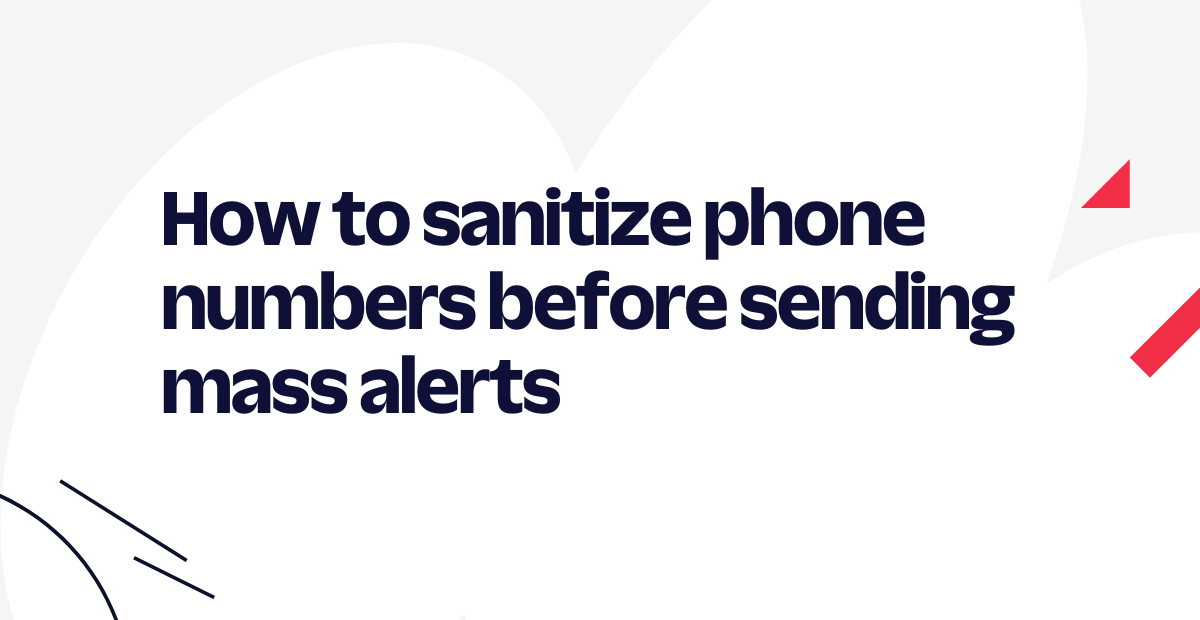
If you're planning on sending mass text notifications you'll want to make sure that the numbers you're sending to are valid. This post will quickly show how to use the Twilio Lookup API to sanitize your data, checking that:
- Phone numbers are real
- Phone numbers are formatted correctly
- Phone numbers are mobile
Validating and sanitizing phone numbers will mean fewer API errors for sending to non-existent, incorrectly formatted, or landline numbers, giving you greater confidence in your system.
Prerequisites for sanitizing phone numbers
- A Twilio account.
- The Twilio Python helper library. Follow instructions to install it here.
Option #1: Normalize phone numbers with national formatting to E.164 and check their validity
This option requires that you know the ISO country code for every phone number, but assuming you have that information you can standardize national formats with parentheses or hyphens to an E.164 formatted number. E.164 is required for many Twilio API phone number inputs.
Copy the following code to a new file, I called mine sanitize.py
:
Valid phone numbers will return the E.164 formatted number. If the function is called with an invalid phone number, it will return None
and print an error message:
Option #2: You have phone numbers in E.164 and want to check their validity
This code sample shows you how to run a phone number through the validation function of the API. Add this to the bottom of your Python file:
For a valid phone number like the one in the example above, this will return True
. Try it with an invalid phone number like +12345
, the function will return False
and provide the reasons.
Check for mobile phone number type
If you want to check the phone number line type, use the following code. Contact Twilio Sales for pricing if you anticipate a large number of requests.
This code will check the line type of the phone number to see if it is mobile. The possible line types include mobile
, landline
, or nonFixedVoip
among others.
Next steps after sanitizing phone numbers
The Twilio Lookup API is a great way to validate new inputs or sanitize data you already have. Once you have trust in the data check out more ways to engage with your customers:
I can't wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.