Send Bulk SMS With Go and Twilio
Time to read:
Sometimes, nothing beats a good old SMS. The delivery of an SMS is not reliant on the recipient having any form of connection to the internet. This makes it highly reliable when delivering critical notifications. In certain instances, such as Amber alerts, for example, these notifications need to be sent in bulk to a wide audience.
You might be wondering how difficult it would be to implement such a system. Well, with Twilio it’s actually a breeze. In this post, I will show you how to build a bulk SMS-sending application using Golang, powered by Twilio's Messaging Services.
Prerequisites
To follow this tutorial, you will need the following:
- A free Twilio account
- A Twilio phone number with SMS capabilities
- A phone that can send and receive SMS
- A basic understanding of Go
- Go 1.19
- Git
Set up the project
The first thing you need to do is to create a project directory and change in to it. To do that, run the following commands.
Next, create a new Go module by running the following command
Then, add the project's two dependencies:
- GoDotEnv: This will help with managing environment variables
- Twilio Go Helper Library: This simplifies interacting with the Twilio API
To install them, run the command below.
Next, create a new file named .env to store the environment variables that the application requires. In the new file, paste the following code.
After that, create a local version of the .env file using the following command.
Note: .env.**.local files are ignored by Git as an accepted best practice for storing credentials outside of code to keep them safe.
Make your Twilio credentials available to the application
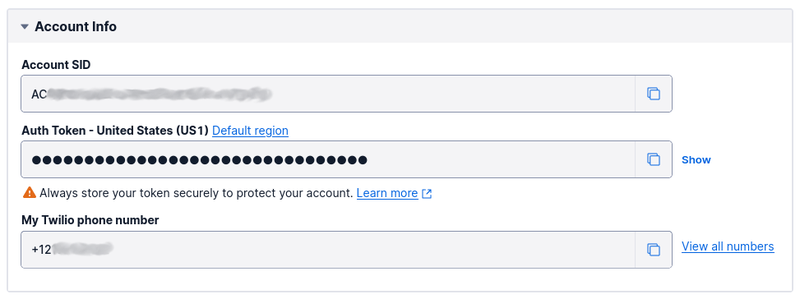
The next thing you need to do is to retrieve your Twilio Auth Token and Account SID. To do that:
- Login to the Twilio Console
- Copy the details from the "Account Info" panel
- In .env.local, replace
<<TWILIO_ACCOUNT_SID>>
and<<TWILIO_AUTH_TOKEN>>
respectively, with the copied details
Create a Messaging Service
The next thing to do is to create a new Messaging Service and retrieve its SID. To do that, in the Twilio Console, under Explore Products > Messaging > Services click Create Messaging Service.
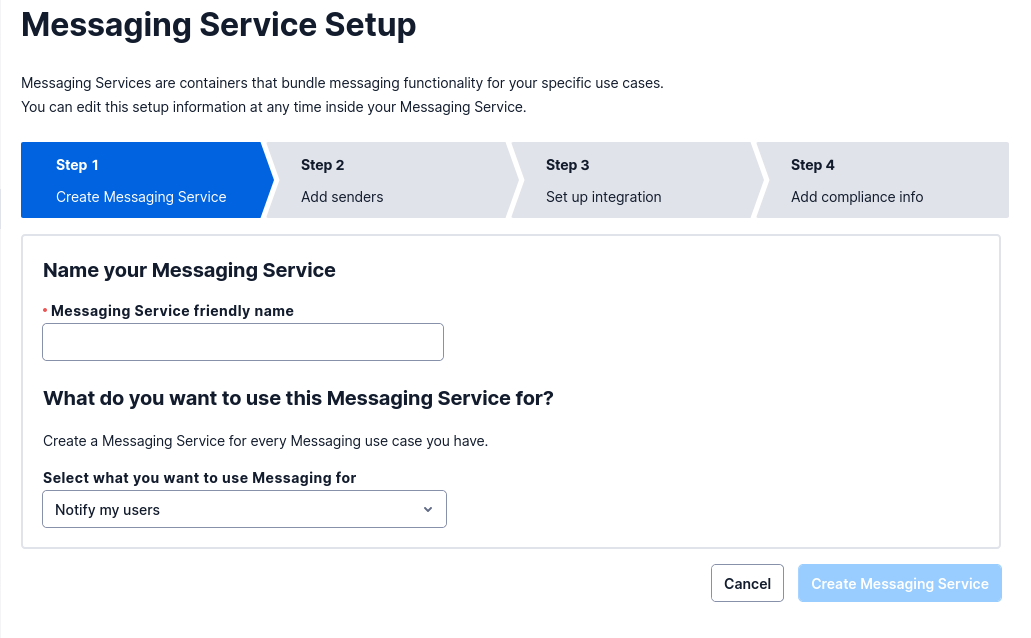
In Step 1, enter a name for the service in the "Messaging Service friendly name" field, then click "Create Messaging Service".
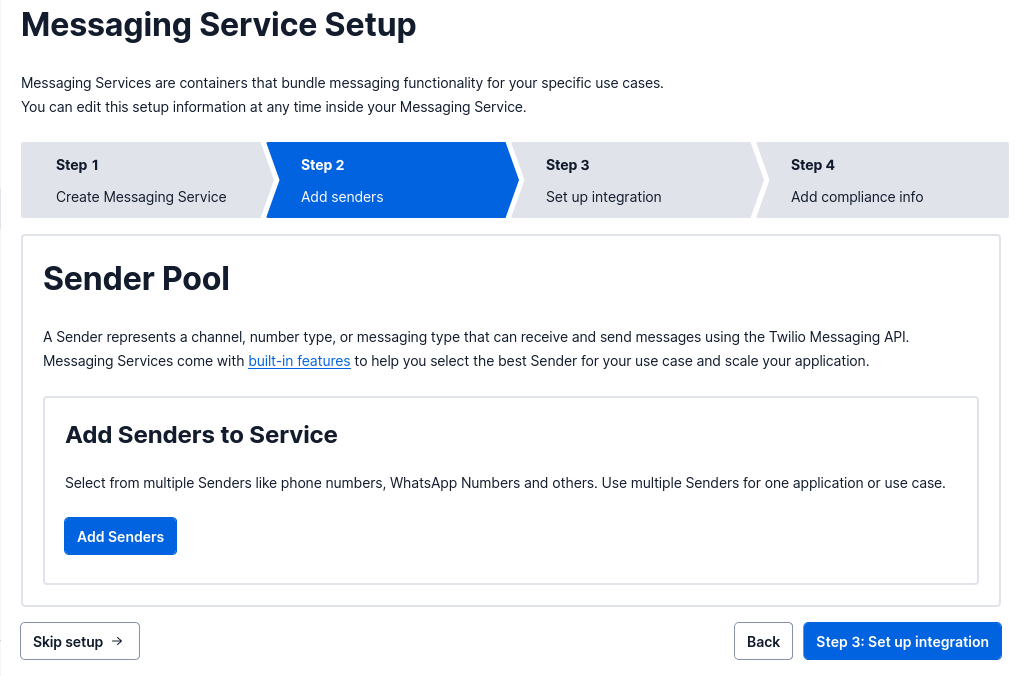
Then, in Step 2, click Add Senders to start adding senders to the service.
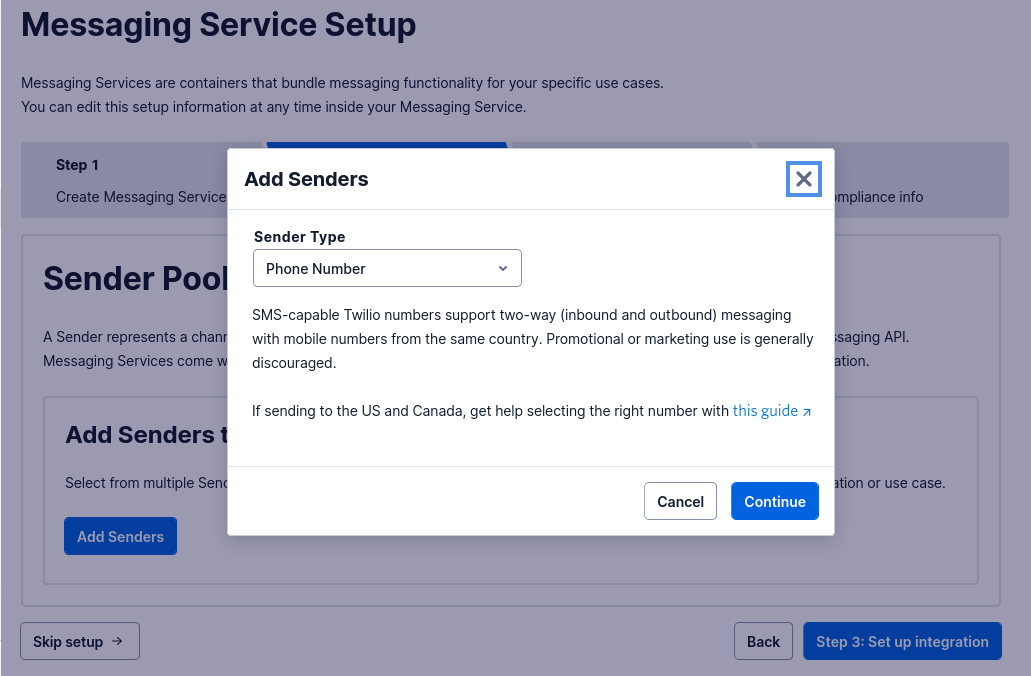
There are multiple sender types that you can choose from, but for the purposes of this tutorial, leave the Sender Type dropdown set to "Phone Number" and click Continue.
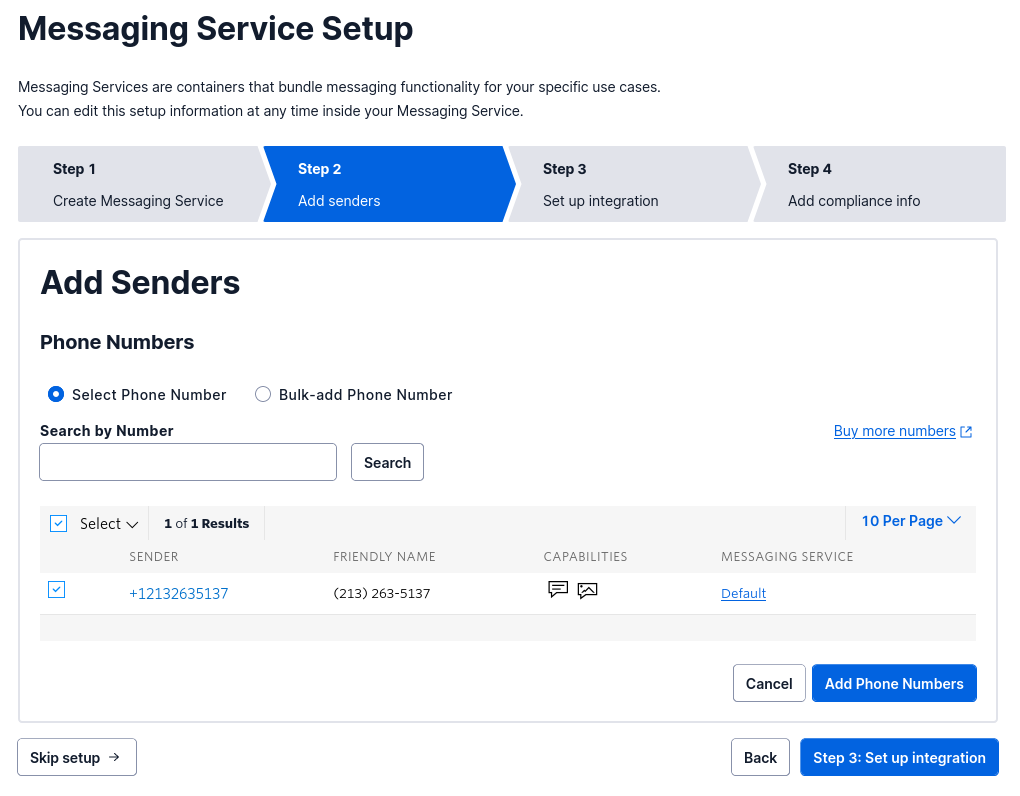
Next, check a Sender from the list that has SMS capabilities and click Step 3: Set up integration. SMS-capable phone numbers are indicated by the left of the two icons in the Capabilities column in the screenshot above.
In Step 4, leave the options as they are and click Step 4: Add compliance info. Finally, in Step 5, again, leave the options as they are, click Complete Messaging Service Setup, and click View my new Messaging Service in the modal dialogue that appears.
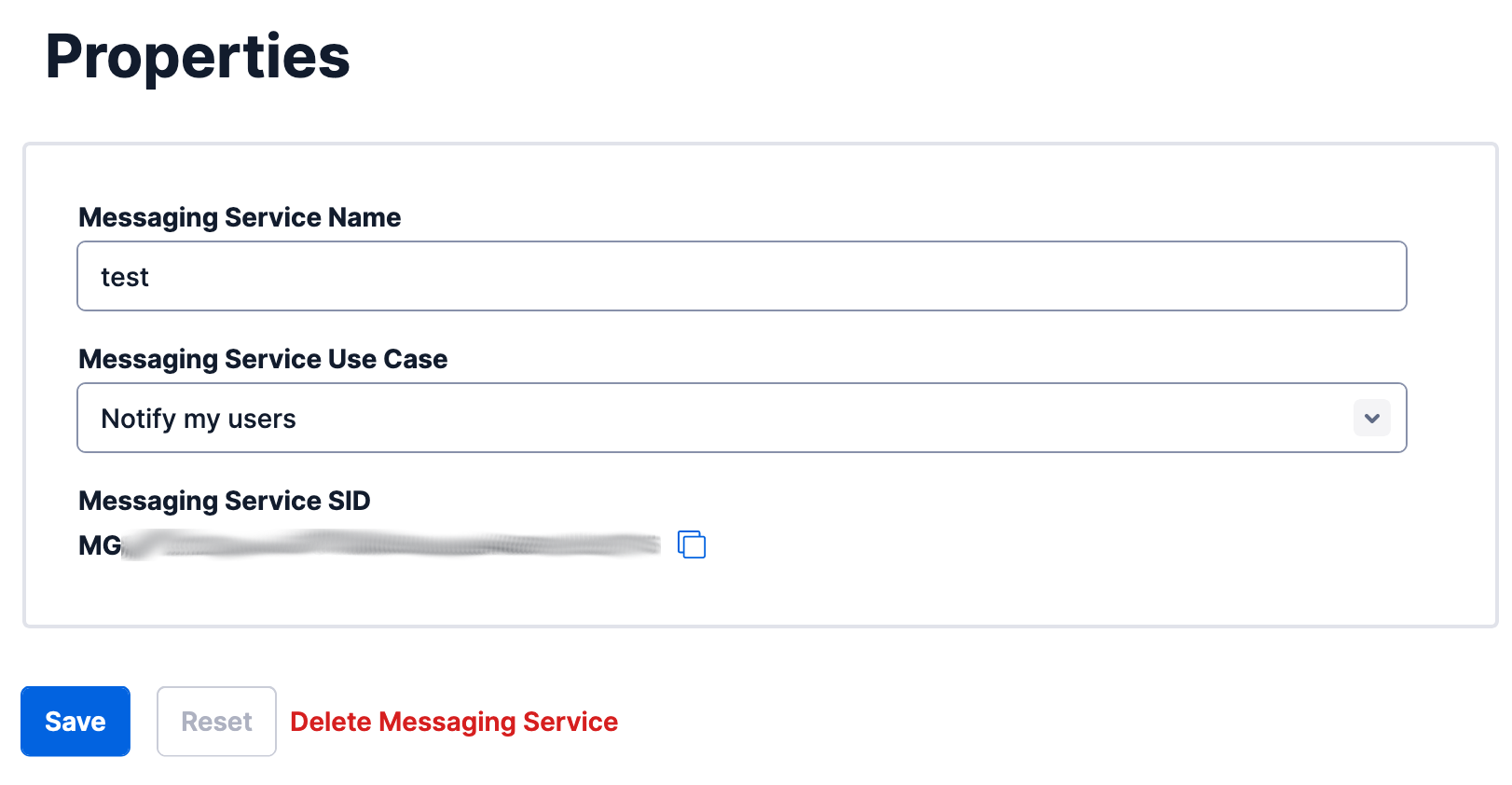
With the Messaging Service created, copy the Messaging Service SID and paste it into .env.local in place of <<TWILIO_MESSAGING_SERVICE_SID>>
.
Build the backend
Prepare the request model
First, create a struct named SMSRequest
. This struct will be used to marshal and validate POST requests to the endpoint responsible for sending bulk SMS. It will also be passed to the helper module responsible for making the relevant Twilio requests.
To do this, in the application’s top-level folder, create a new folder named model. In this folder, create a new file named SMSRequest.go and add the following to it.
The struct is made up of two fields: Recipients
and Messages
. The Recipients
field is a slice of strings - the phone numbers to be contacted via SMS. The Message
field is a string that corresponds to the content of the message to be sent. The appropriate JSON bindings are also specified for both fields.
A Validate()
function is provided which ensures that the Message
field is not empty, and that all the phone numbers specified are in the expected format (E.164).
Prepare the helper module for sending messages
In the application’s top-level directory, create a new folder named helper. In this directory, create a new file named SMS.go and paste the following code into the file.
The BulkSMS()
function instantiates a Twilio client and iterates through the recipients in SMSRequest
, sending a CreateMessage()
request for each phone number. If, for some reason, the request fails, numberOfFailedRequests
is updated accordingly.
The process is considered successful if there are no failed requests. Otherwise, an error is returned letting the user know the number of requests which failed. More information will be available in the errors in the Twilio logs.
Prepare the entry point for the application
Finally, you need an entry point for the application. This is usually a file named main.go. In the application’s top-level directory, create a new file named main.go. Then, paste the following code in the newly created file.
The main()
function loads the environment variables, serves the static files, and declares the handler for the API route which will handle sending the messages.
Build the user interface
In the application’s top-level folder, create a new folder named static. This folder will hold the HTML, CSS, and JS files for the application's interface.
Then, create a file named index.css in the new folder, and paste the following code into it.
When phone numbers are entered, they are displayed as badges prior to submission, and the badge styles the padding for such elements. The .hidden
property is used to hide elements when required.
Next, create a file named index.js in the newly created folder and add the following code to it.
To add multiple phone numbers, the user will press the Enter key after typing each phone number. This is handled by the keyup event listener. For each new phone number, a badge is created with a random colour and rendered on the page. The phone number is also added to an array named recipients
.
A handleSubmit()
function is also declared which displays a loading indicator while the array of recipients is sent to the backend via a POST request. When a response is received, an alert is displayed to let the user know the outcome of the request.
Finally, create a new file named index.html in the static folder and add the following to it.
Test that the application works
First, start the application using the following command.
Then, view the application in your browser by navigating to http://localhost:8000/.
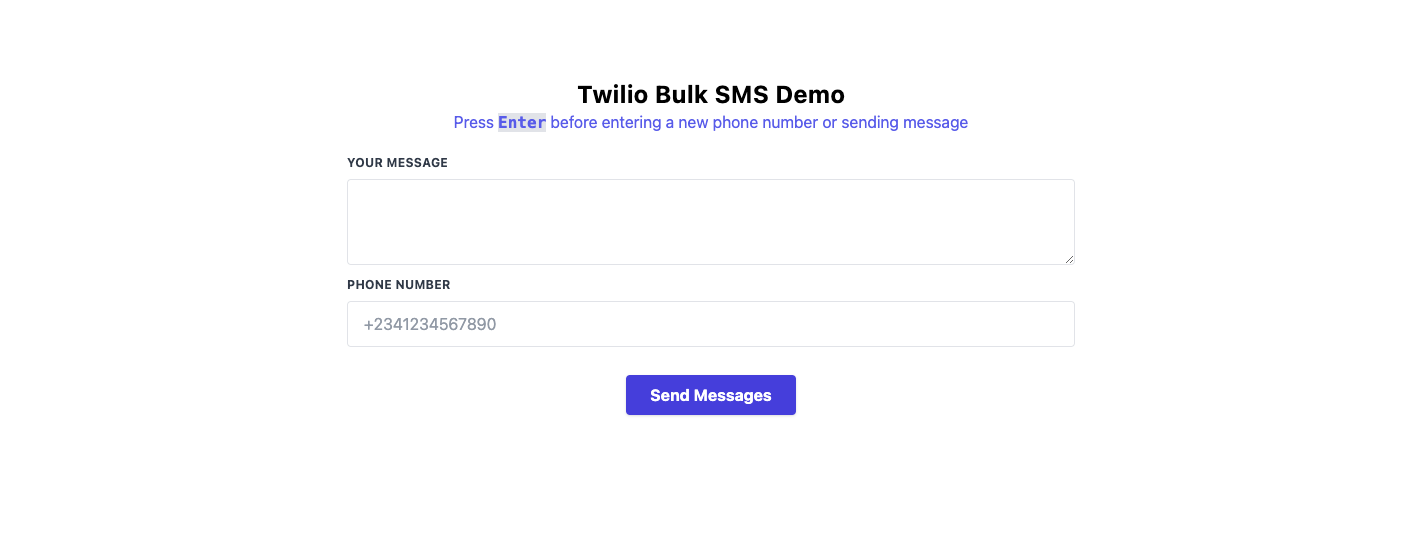
Add as many valid phone numbers as you want (remember to press Enter after each phone number). When you're finished adding phone numbers, click Send Messages.
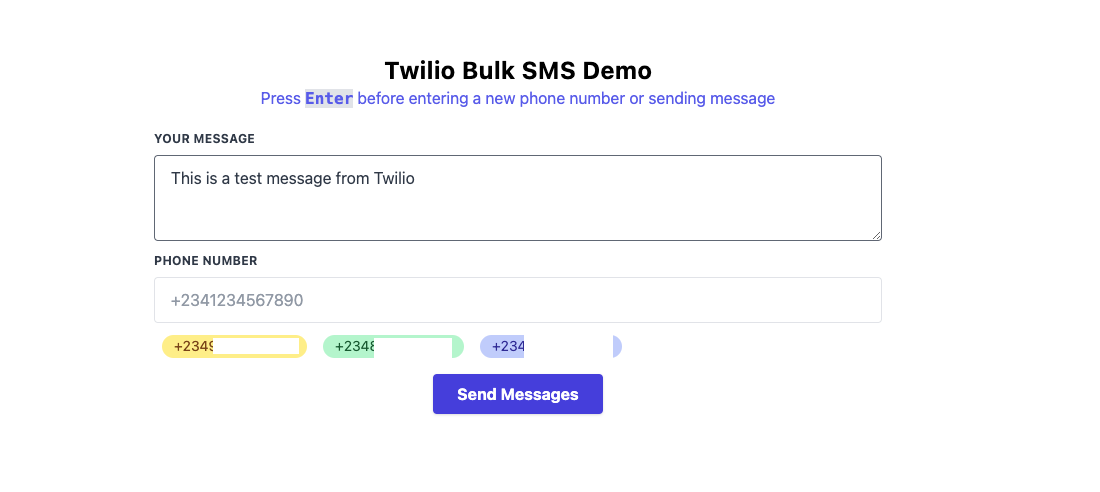
Once the messages have been sent, an alert will be displayed.
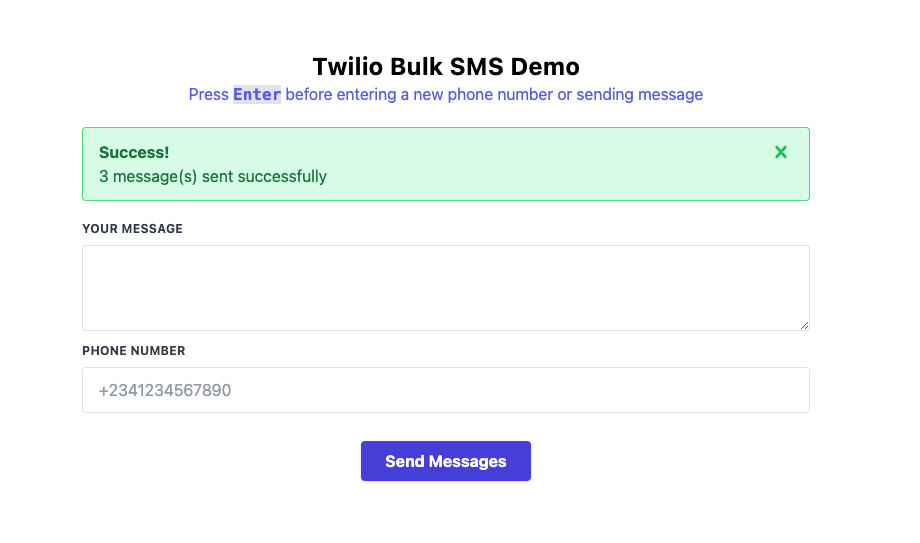
There you have it!
You’ve successfully built a Go-powered bulk SMS-sending application
Notice how you don't have to worry about either the queuing or the delivery process. Twilio's infrastructure is capable of handling notifications for your users in real-time.
The entire codebase for this tutorial is available on GitHub. Feel free to explore further. Happy coding!
Oluyemi is a tech enthusiast with a background in Telecommunication Engineering. With a keen interest to solve day-to-day problems encountered by users, he ventured into programming and has since directed his problem-solving skills at building software for both web and mobile.
A full-stack software engineer with a passion for sharing knowledge, Oluyemi has published a good number of technical articles and content on several blogs on the internet. Being tech-savvy, his hobbies include trying out new programming languages and frameworks.
- Twitter: https://twitter.com/yemiwebby
- GitHub: https://github.com/yemiwebby
- Website: https://yemiwebby.com.ng/
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.