Send Email Attachments Using Twilio SendGrid and PHP
Time to read: 2 minutes
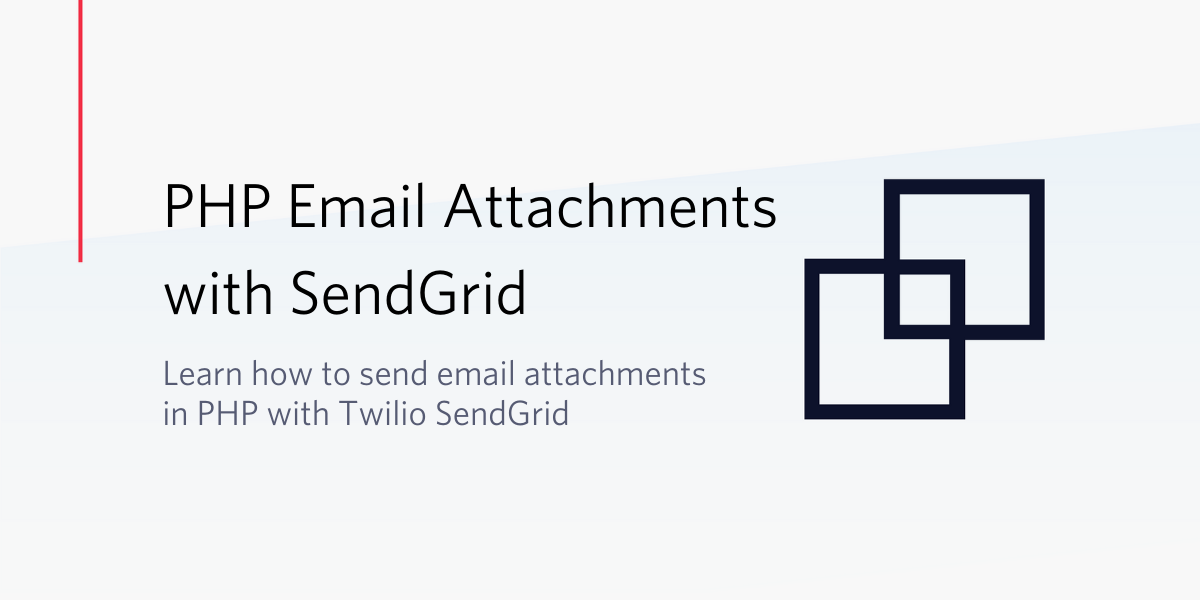
Companies with a clear commitment to efficient communication rely heavily on email as a communication tool. At some point, this dependency requires sending email attachments to customers for transactions such as receiving their invoice after an online purchase, sending of travel itinerary and many more. These attachments can be images, videos, or even documents. Twilio SendGrind API provides a simple and efficient way of doing this. In this tutorial, I will take you through how to send email attachments with SendGrid and PHP.
Prerequisites
Ensure you have the following installed in your local development environment:
Also, head over to SendGrid and create a free account.
Getting Started
In your preferred terminal, run the following commands to set up the project:
The above commands created a folder called demo
which you will use to store all of your project files. We have also installed two packages that will be useful when building this project. The vlucas/phpdotenv
package is responsible for loading environment variables into your application and the sendgrid/sendgrid
package gives access to the SendGrid API inside the PHP application.
Obtaining Your SendGrid API Key
You’ll need a SendGrid API key in order to send your emails. After creating an account with SendGrid, navigate to the API Keys section under Settings to create one. Be sure to select the Full Access
option like shown below:
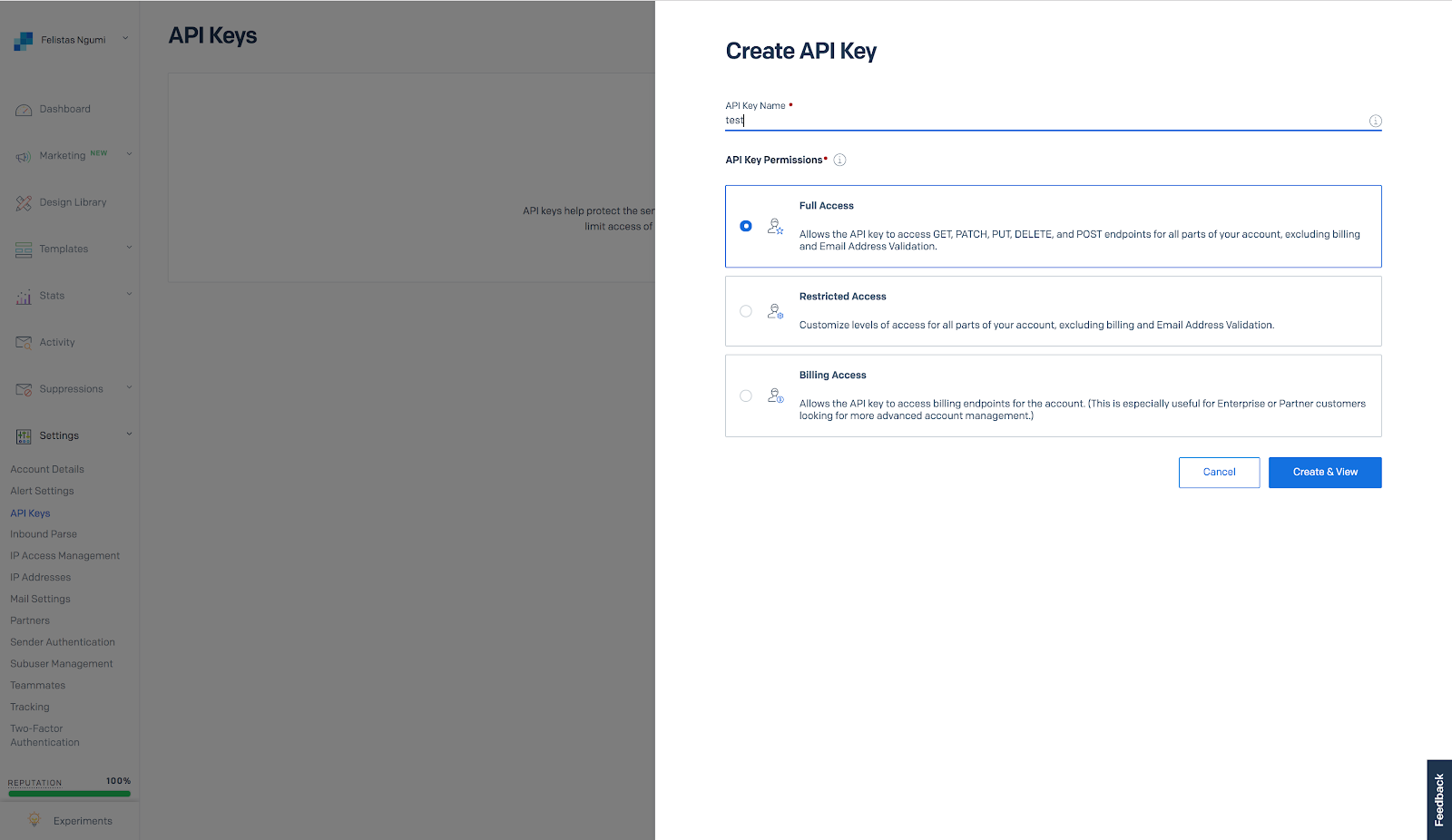
NOTE: You should never use sensitive credentials directly in your code e.g API Keys. Always store them in a .env
file.
Run the following command in your terminal to create the .env
file.
Next, add the following key to your file and copy the API Key you just generated.
Send Email Attachments
In your preferred code editor, add the following lines of code in the email.php
file.
Note: Be sure to replace the recipient address to yours.
The above code loads the set environment variables, then instantiates a new SendGrid Mail class that we use to call methods such as setFrom
to construct the final email object sent to the recipient.
Testing
Add a pdf file in the root directory of this project. Replace test.pdf
in the code with the name of the file you have added.
In your terminal, run php email.php
. You should see a new email in your inbox!
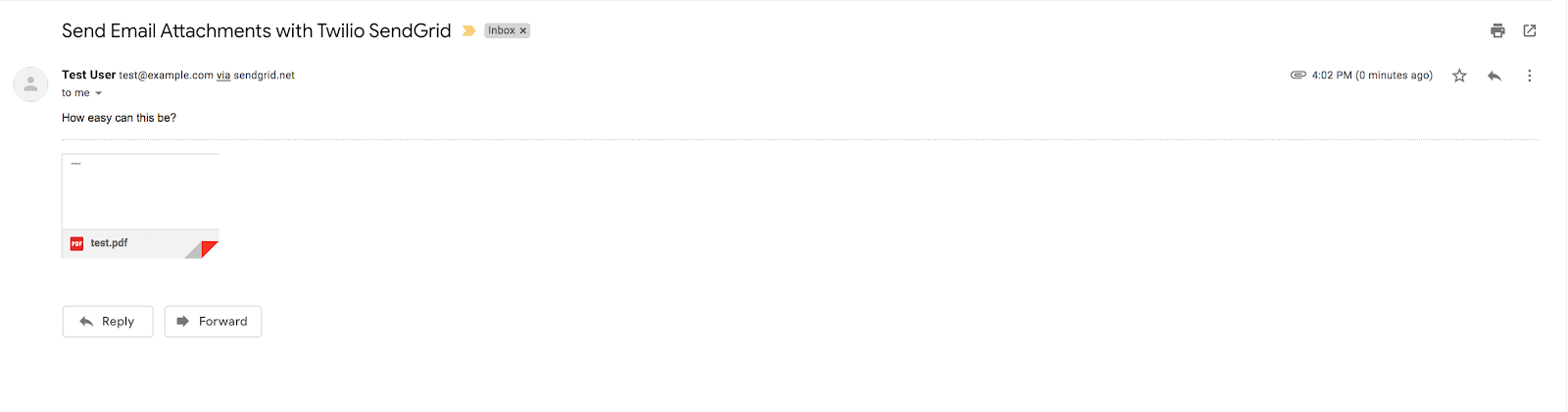
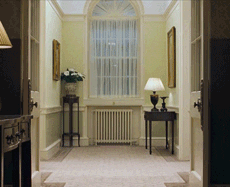
Conclusion
In this tutorial, we have learned how to send email attachments using Twilio SendGrid and PHP. I would love to hear from you! Happy hacking!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.