How to Send SMS Notifications to Readers When New WordPress Posts are Published
Time to read: 4 minutes
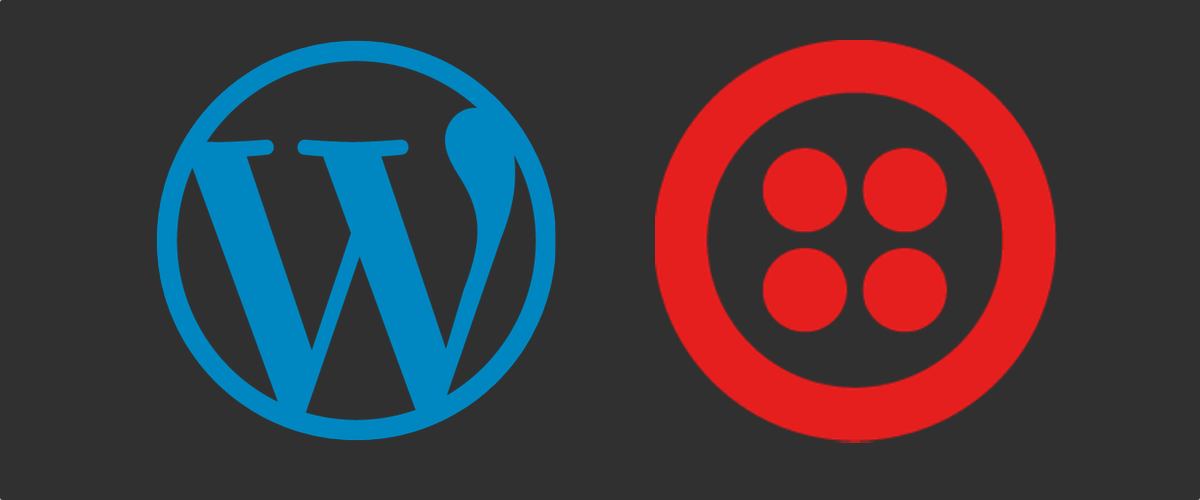
Make sure your subscribers see every post by offering instant SMS notifications when you publish new blog posts in WordPress.
At the end of this project, you will have a custom WordPress Plugin that allows:
1. WordPress users to have a “Mobile Phone” field in their profile to sign up for notifications
2. posts to automatically send a notification message to every subscriber who signs up
If you’re in a hurry, you can find the almost-ready code on GitHub, just drop in the latest Twilio PHP Library and update your Twilio variables in sms-notify.php
.
Setup
You will need to already have a WordPress Blog running to build this plugin.
In your WordPress directory, go to wp-content/plugins
and make a directory called sms-notify
. Inside that directory, make a file named sms-notify.php
. In this file we’ll start with some plugin metadata. This is what WordPress reads to show in our Plugin Dashboard.
Next, activate our new plugin. Go to your WordPress Dashboard at the “/wp-admin” path and click the Plugins link on the left column. Click the “Activate” link on our plugin called SMS Notify.
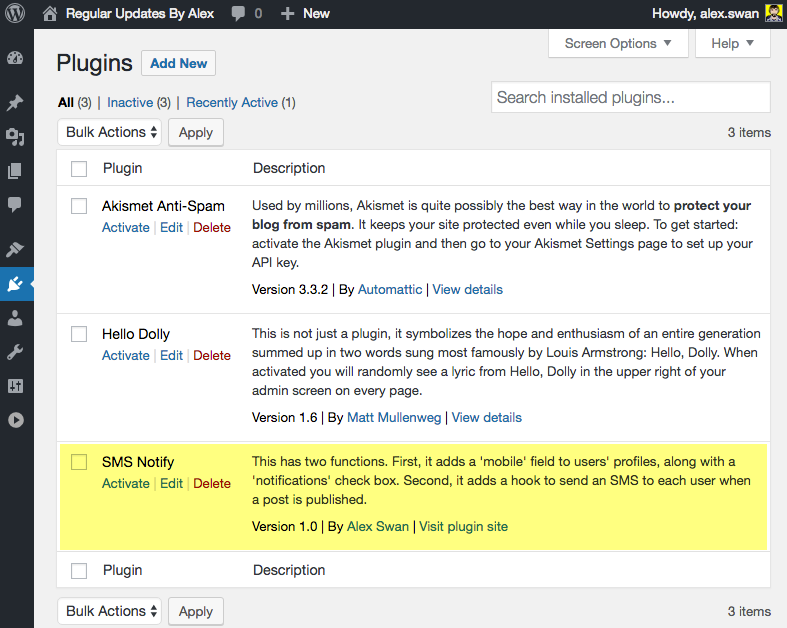
Once it’s active, the link will say “Deactivate”. If something went wrong, you’ll see an error. Time to start modifying WordPress!
Let Users Store Their Phone Number
We want users to be able to sign up for SMS notifications, and we’ll do that by adding a phone field in their user profile. WordPress makes this easy with the user_contactmethods function. Add this code to sms-notify.php
:
When WordPress shows the user_contactmethods
data, it will now pass it to our function before going on to display it to the user. We add the mobile
field to it so our user can put their phone number in it. Let’s try it out!
Back at the “/wp-admin” path, go to the Users tab and click the Add User button. Create a user with the role Subscriber. In an incognito window, log in to your blog with that user’s credentials, then go to “/wp-admin/profile.php”. Add a phone number for your new user, then hit Save.
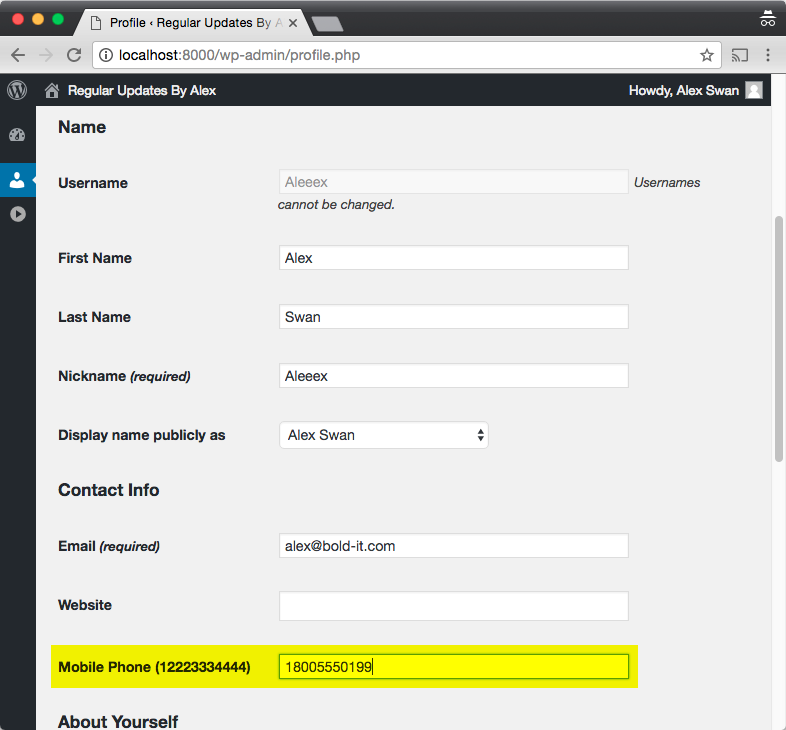
If This Action Happens, Then Send an SMS
Create an Action Hook
Next, set up a function that is invoked during a publish_post action and use get_users to iterate over all the subscribers of the blog. This code is in preparation for sending a text message to each subscriber with a number attached to their profile.
For each of the blog’s Subscribers, WordPress gets the phone number stored using get_user_meta. Finally, add_action
gets configured to execute post_published_notification
when the publish_post
action happens. Now, to send our SMS.
Set Up Your Twilio Account
Go to the Twilio Console (sign up for a free account if you do not already have one) and note your Account SID, Auth Token and a phone number you purchase. You’ll need to add this information in next.
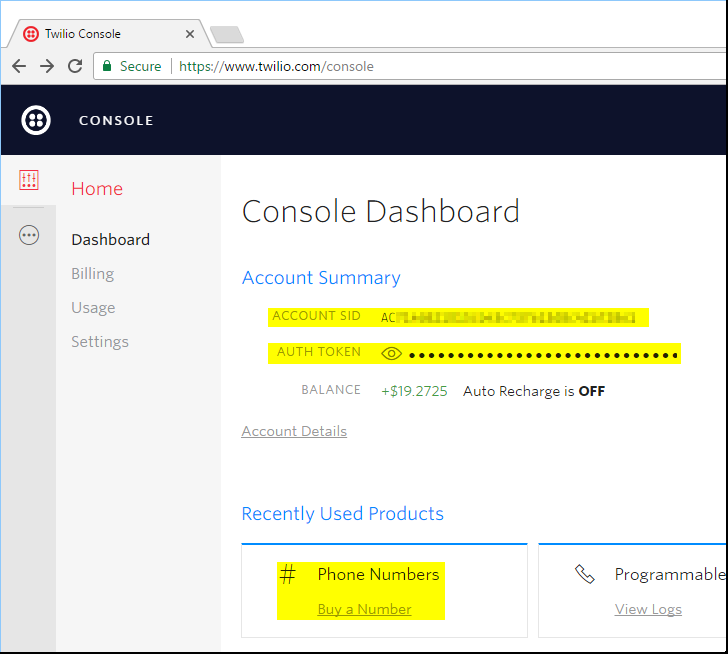
Use Twilio to Send an SMS
Go to Twilio’s PHP Library and install the library. It’s recommended to use Composer, but copying the twilio-php-master
folder into our sms-notify
folder works as well. Now add references to the library in the top of sms-notify.php (after the metadata).
Modify our post_published_notification
function to make a Twilio client, and send a message in the foreach
loop.
Be sure to update the $sid
, $token
, and $from
variables to your own Account SID, Auth Token and a Twilio phone number that you own.
Test
Head back to your WordPress admin page at “/wp-admin” and add a new post. Write your deepest desires, then hit the publish button.
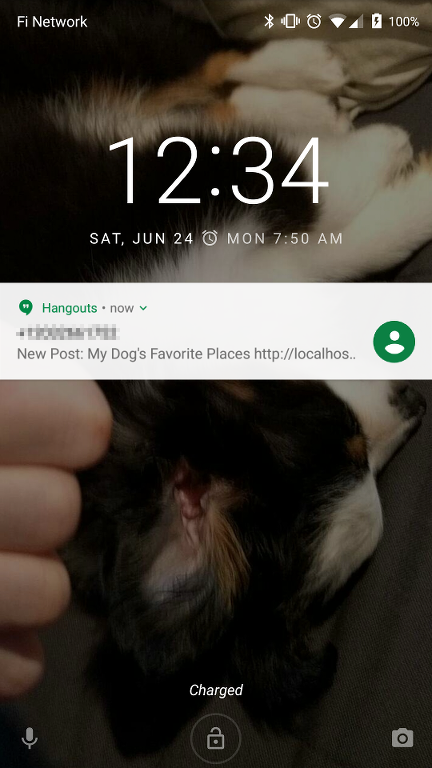
That’s it! We got our SMS notification that a new post has been published.
What’s Next
Now that basic functionality is done, you can use this as a springboard for your own ideas. Here are a few ways to build on this project:
- Validate the user’s input, and user the Twilio Lookup API to check phone numbers
- Create a Twilio Function to handle users’ responses to their notifications
- Call a user and read the post body to them
- Create an Options page to manage Twilio credentials or message templates
- Verify a user’s phone number by having them respond to an SMS
Thanks for using this code to enable SMS notifications on newly-published WordPress posts. Let me know what you build by contacting me via boldbigflank on GitHub or @boldbigflank on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.