How To Send a WhatsApp Message in 30 Seconds with Python
Time to read: 2 minutes
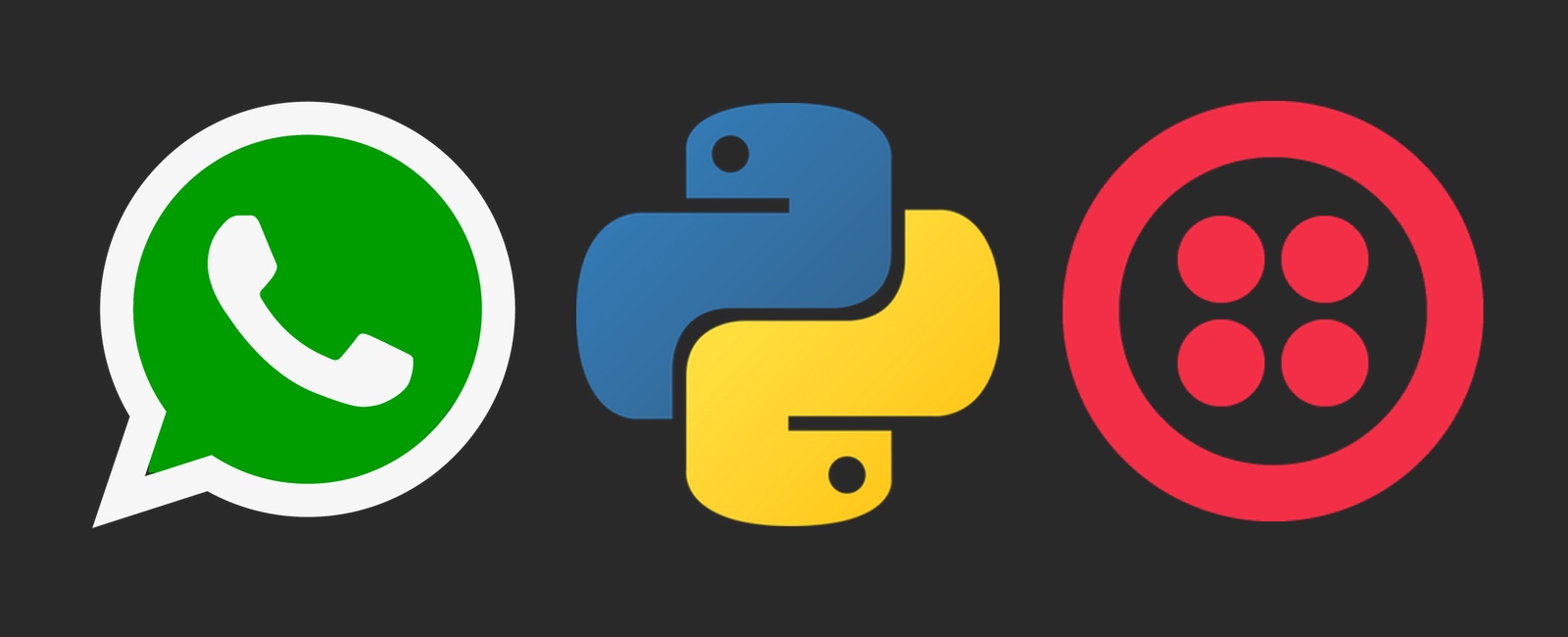
WhatsApp is an over-the-top (OTT) messaging service widely used throughout the world. In this tutorial, we will learn how to quickly send WhatsApp messages through the Twilio Messaging API with reusable code that can be added to any Python application.
Development Environment Setup
We need the following dependencies installed on our local development environment to send WhatsApp messages.
- Python version 2 or 3
- A free Twilio account with an activated WhatsApp Sandbox
- The Twilio Python Helper Library
If you do not have Python already installed on your machine, go to the Python downloads page and install the latest version now.
Next, log into your existing Twilio account or sign up for a new free Twilio account.
After you log into the Twilio Console, take note of your Account SID and Auth Token. The Account SID is a unique identifier for your account while the Auth Token is a secret key that should never be shared or else anyone will have full access to your Twilio account.
We now need to set environment variables to export our Twilio credentials that will allow the Python script to access our Twilio account and use the API.
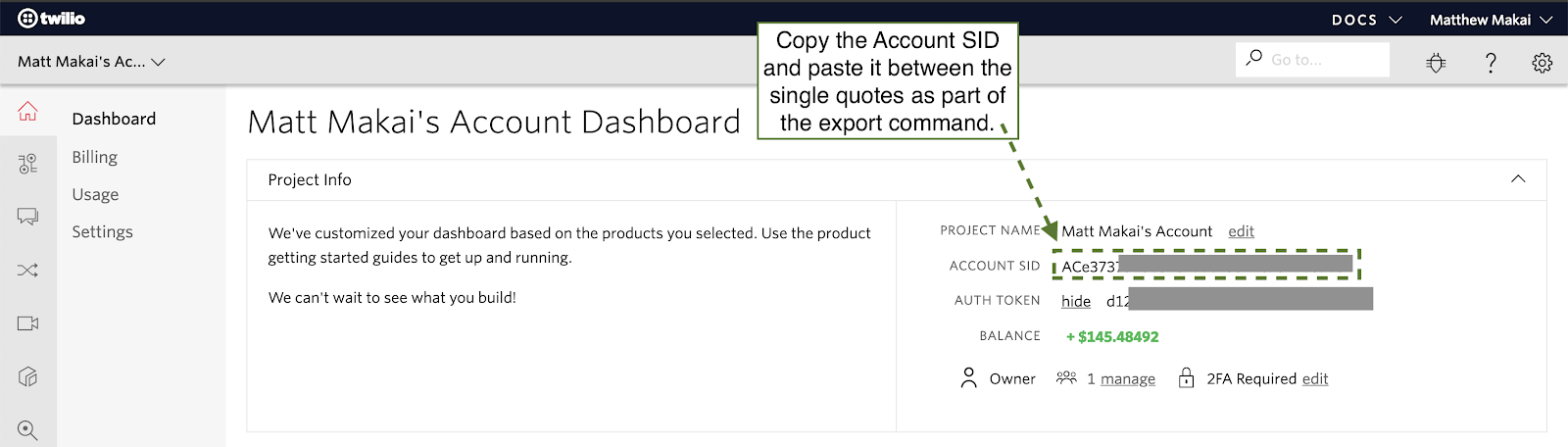
Copy the Account SID and open your terminal. Run the following command and paste your Account SID to export it as an environment variable named TWILIO_ACCOUNT_SID
.
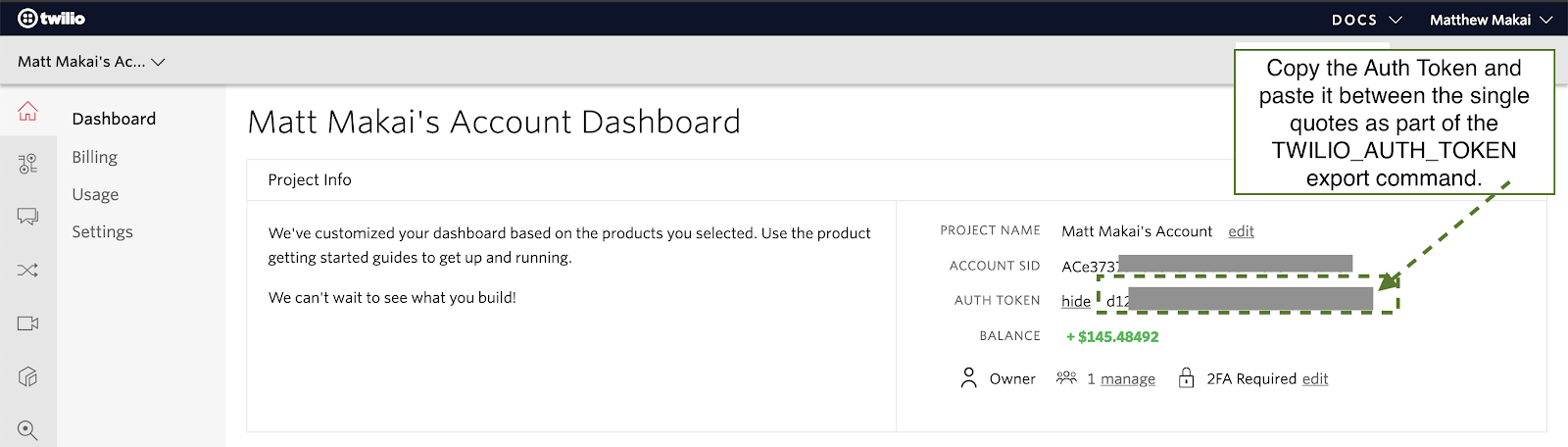
Copy the Auth Token and paste it in between the single quotes for the TWILIO_AUTH_TOKEN
environment variable when you run the following command:
Our environment variables are now set so let's write our Python script and test it out.
Python Code for Sending WhatsApp Messages
Create a new virtual environment with the following Python 3 command:
If you are running Python 2, first install the virtualenv
package then run the following command:
After either of those steps, activate the virtual environment:
Install the Twilio Python helper library into the virtualenv:
Create a file named whatsapp.py
and write or paste in the following code:
The above code imports the Twilio Python helper library, instantiates the helper library client, sets a from and to WhatsApp number, then sends a single message with the client.messages.create
function call.
Our Python script is ready to go and we just need to activate the Twilio WhatsApp sandbox to test it out.
Sending WhatsApp Messages
Go to the WhatsApp page in the Twilio Console and activate the sandbox.
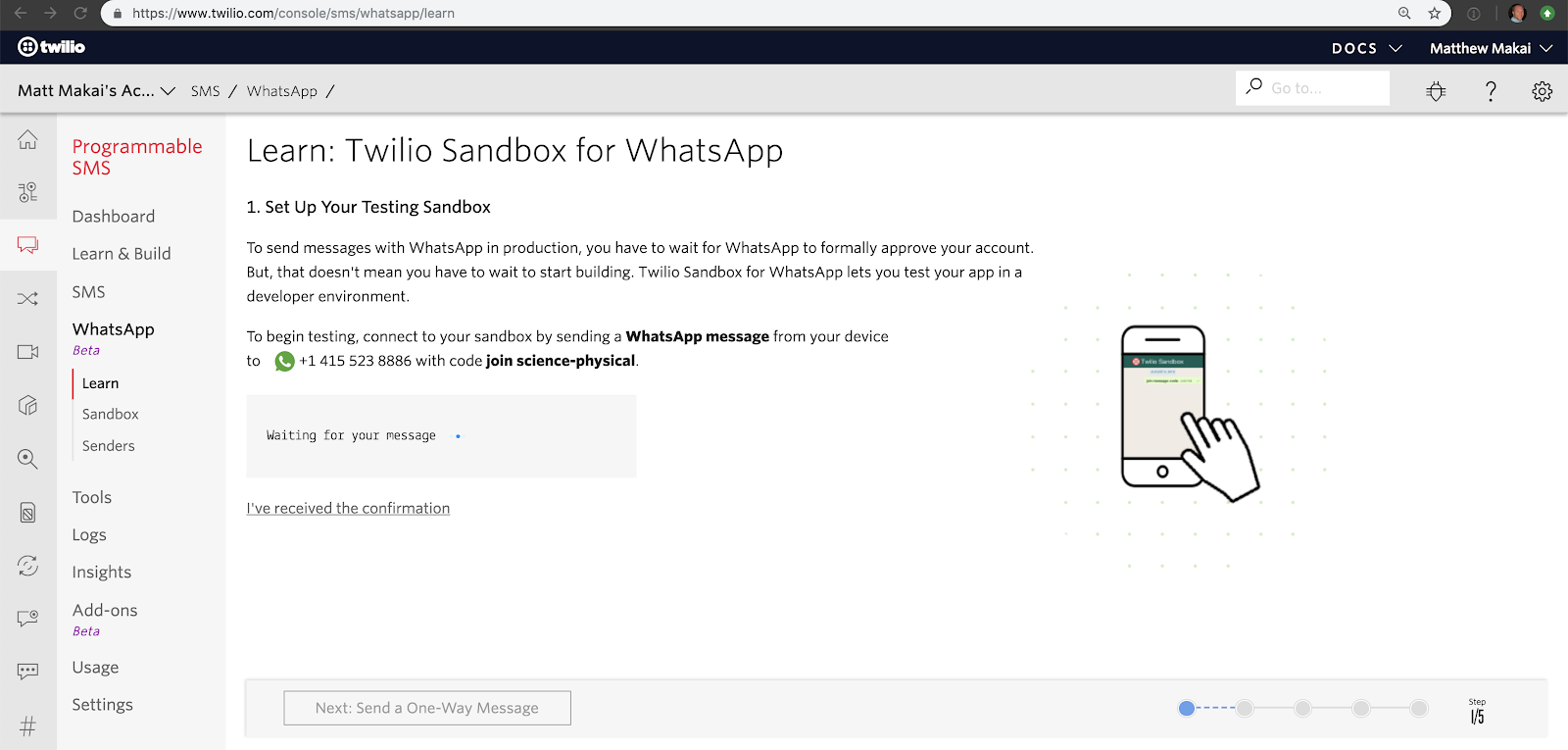
You will be redirected to the page above which instructs you how to connect to your sandbox by sending a WhatsApp message through your device. In my case, I’m required to send join science-physical
to +14155238886.
We are ready to run our Python code and send our first WhatsApp message.
Head back to the terminal. Ensure your virtual environment is still activated and your TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
environment variables remain exported before running the following command:
Check out your WhatsApp Messaging app and you should see your first message sent through the Twilio API.
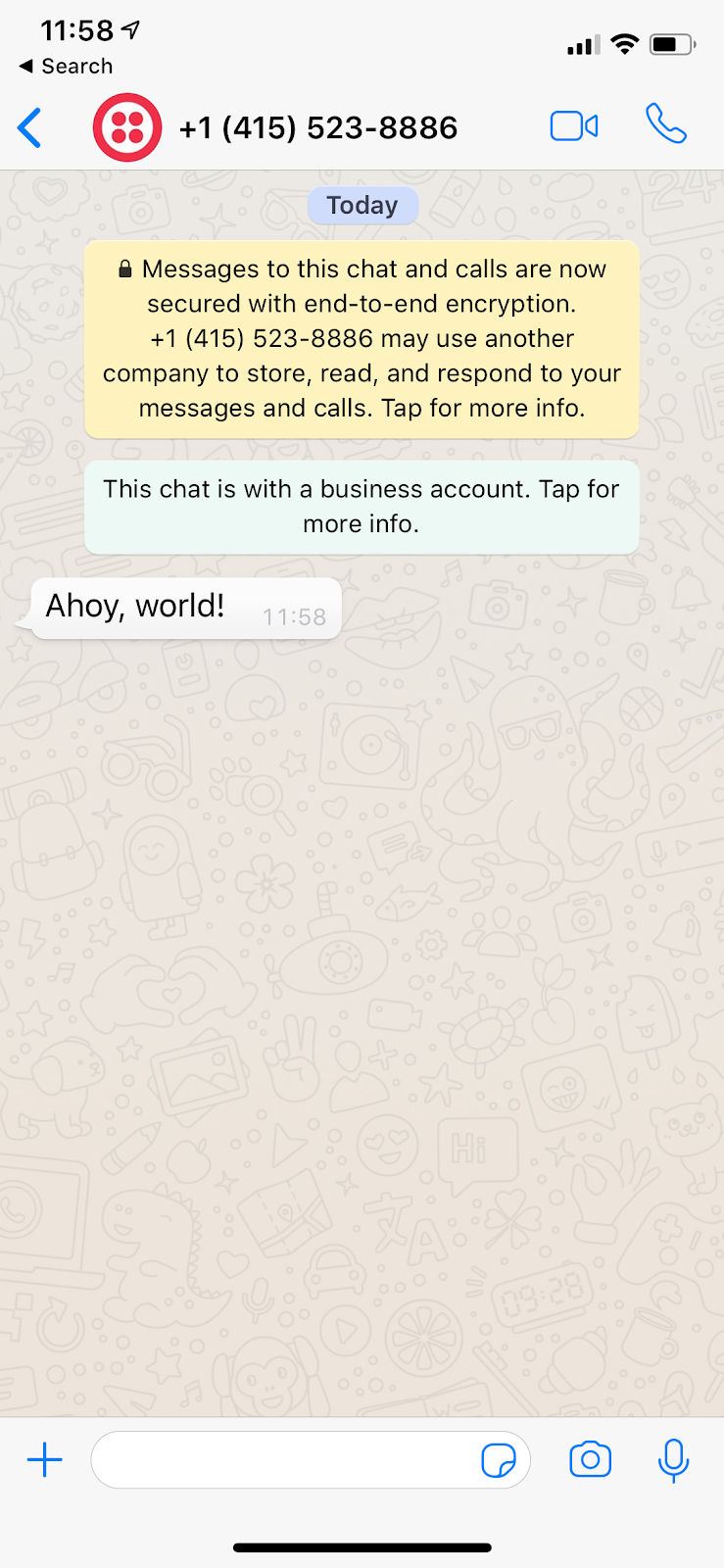
Onward!
In this tutorial, we have learned how to send WhatsApp messages using the Twilio API for WhatsApp Messaging. Next you can try the following tutorials to do even more with the Twilio API and many other forms of communication:
- Try out the WhatsApp Messaging Templates
- Learn how to programmatically receive WhatsApp messages
- Work through the Twilio WhatsApp Quickstart Docs to learn more
Questions about this tutorial? Ping me on Twitter @mattmakai.
Twilio.org helps social impact organizations expand their reach and scale their missions. Build your first application with Twilio and start engaging your audience at no cost with product credits from the Impact Access Program. Learn more about eligibility and how to start your benefits today.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.