Send Rich Content with WhatsApp and the Twilio Content API and Content Template Builder
Time to read: 8 minutes
Send Rich Content with WhatsApp and the Twilio Content API and Content Template Builder
Engaging customers through messaging has become essential for businesses. Whether it’s SMS, MMS, RCS, or WhatsApp, messaging channels offer a direct and effective way to communicate. And adding rich content to your messages takes the experience to the next level, making interactions more engaging and impactful.
In this tutorial, I’ll walk you through how to send SMS, MMS, and WhatsApp messages using Twilio. We’ll start with the Twilio Content Template Builder and then make our way toward using Twilio’s Content API. You’ll learn how to send rich, personalized messages to your customers and get a glimpse of features that can take your messaging strategy even further.
Getting started with Messaging
To begin, make sure you have a Twilio account. If you don’t have one, you can create an account to begin using Twilio for free.
Once you’ve logged in to the Twilio console, click Explore Products in the sidebar on the left. Search in the list of products for Messaging. Since you’ll be using Messaging quite a bit, pin it to your sidebar.
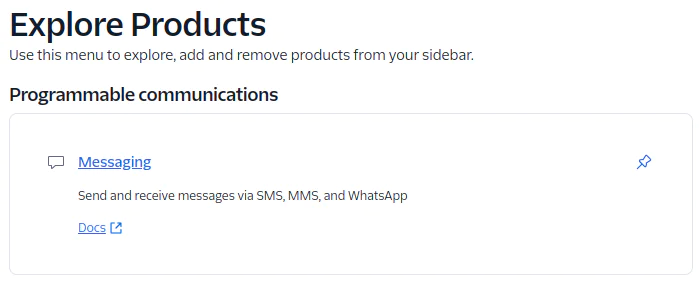
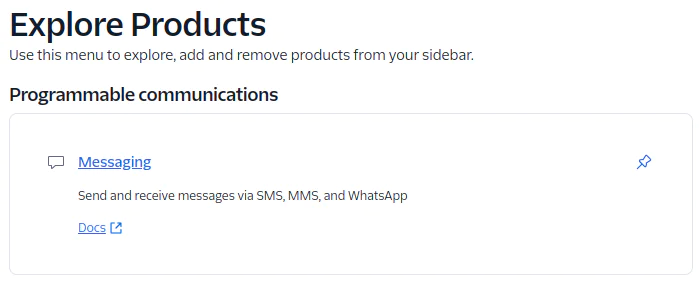
Set up your WhatsApp sandbox
On the Messaging Overview page—if this is your first time using Twilio Messaging—you’ll see a button to Try WhatsApp. Click this button.
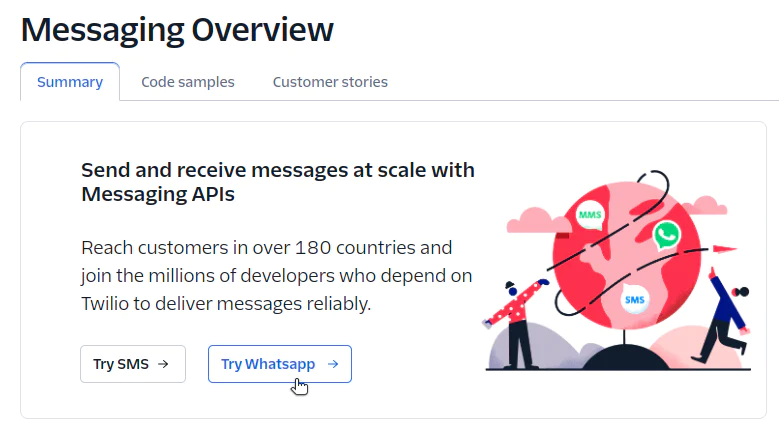
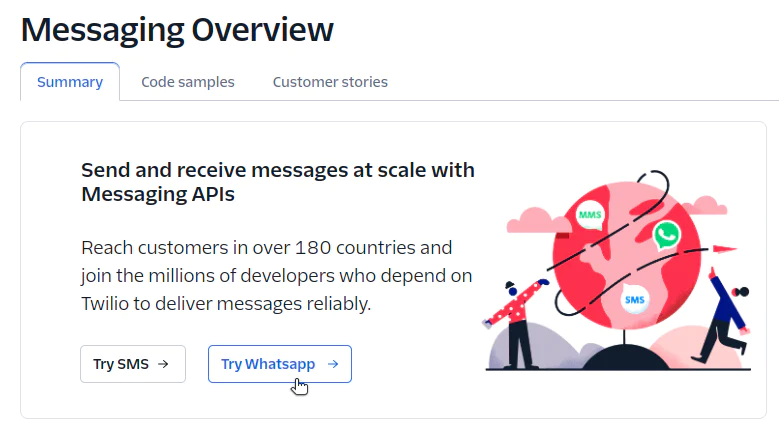
You can also find this in the left sidebar, under Messaging > Try it out > Send a WhatsApp Message.
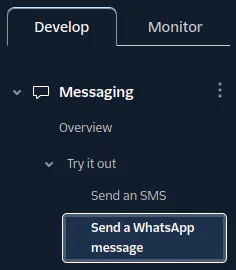
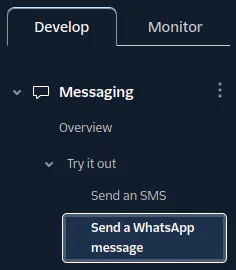
This takes you to the Twilio Sandbox for WhatsApp. As a developer, you can use this sandbox to practice sending WhatsApp messages from a pre-approved WhatsApp phone number. Begin by accepting the terms and conditions to activate your sandbox.
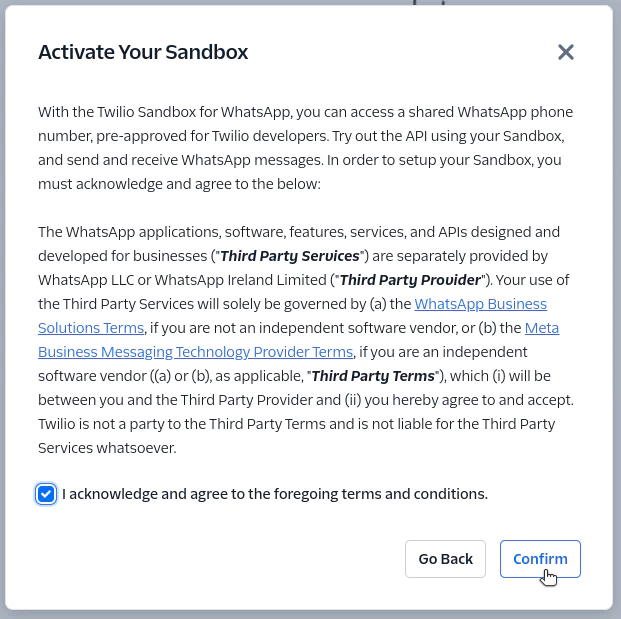
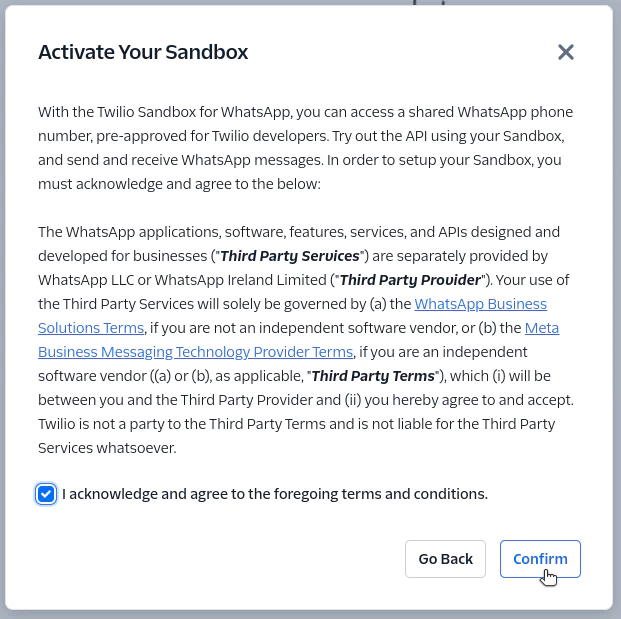
Before you can send a message from your sandbox to a WhatsApp user, that user must opt in to connecting with your sandbox. They do this within the WhatsApp application by sending a code to the sandbox number. The code to use is unique to your WhatsApp sandbox.
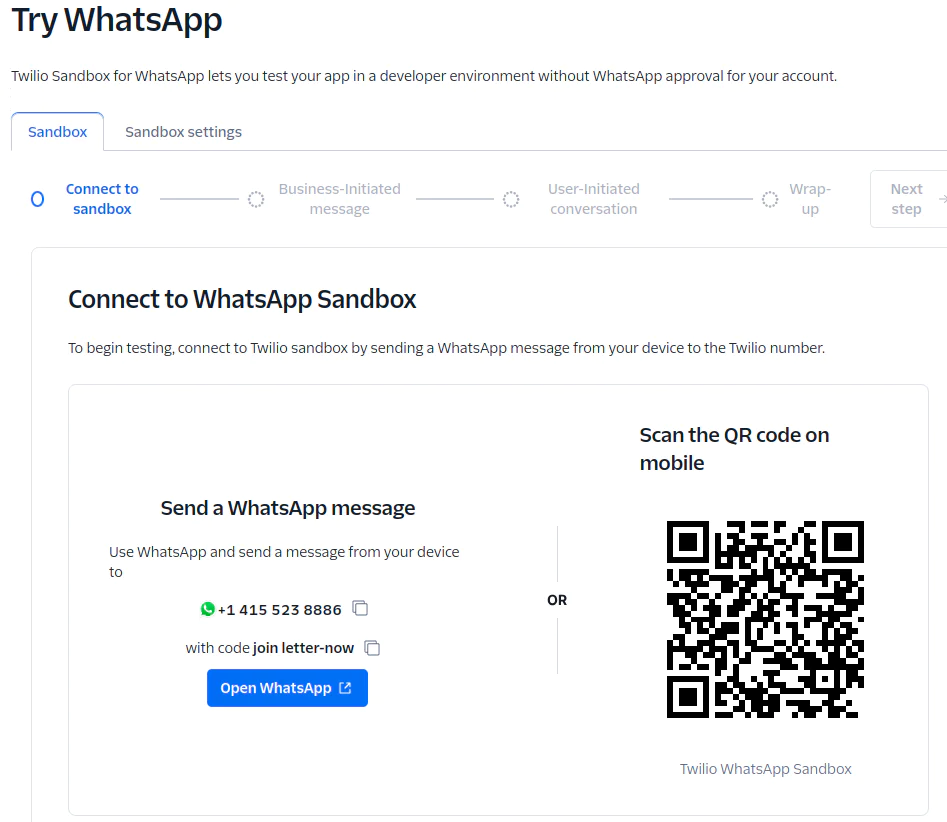
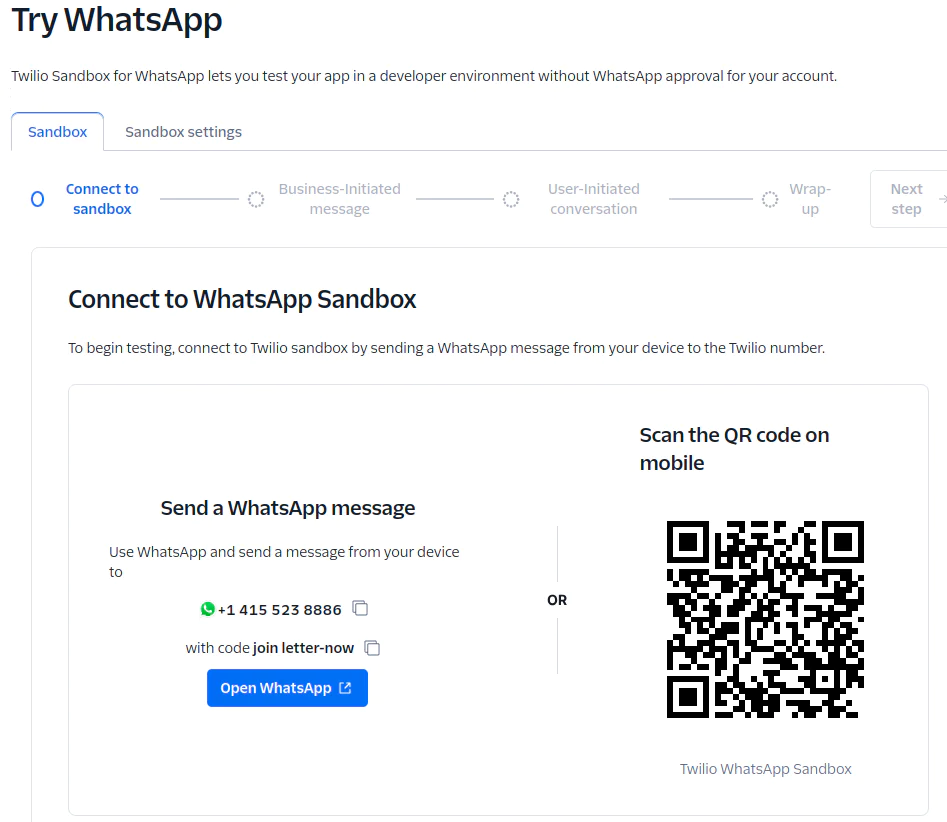
Connect test user to sandbox
From a device with WhatsApp installed, send a message with your unique sandbox code to the listed WhatsApp phone number. For example, in this demo, the code is letter-now
, so I would send join letter-now
. Send a message to join the sandbox, using the code in your console.
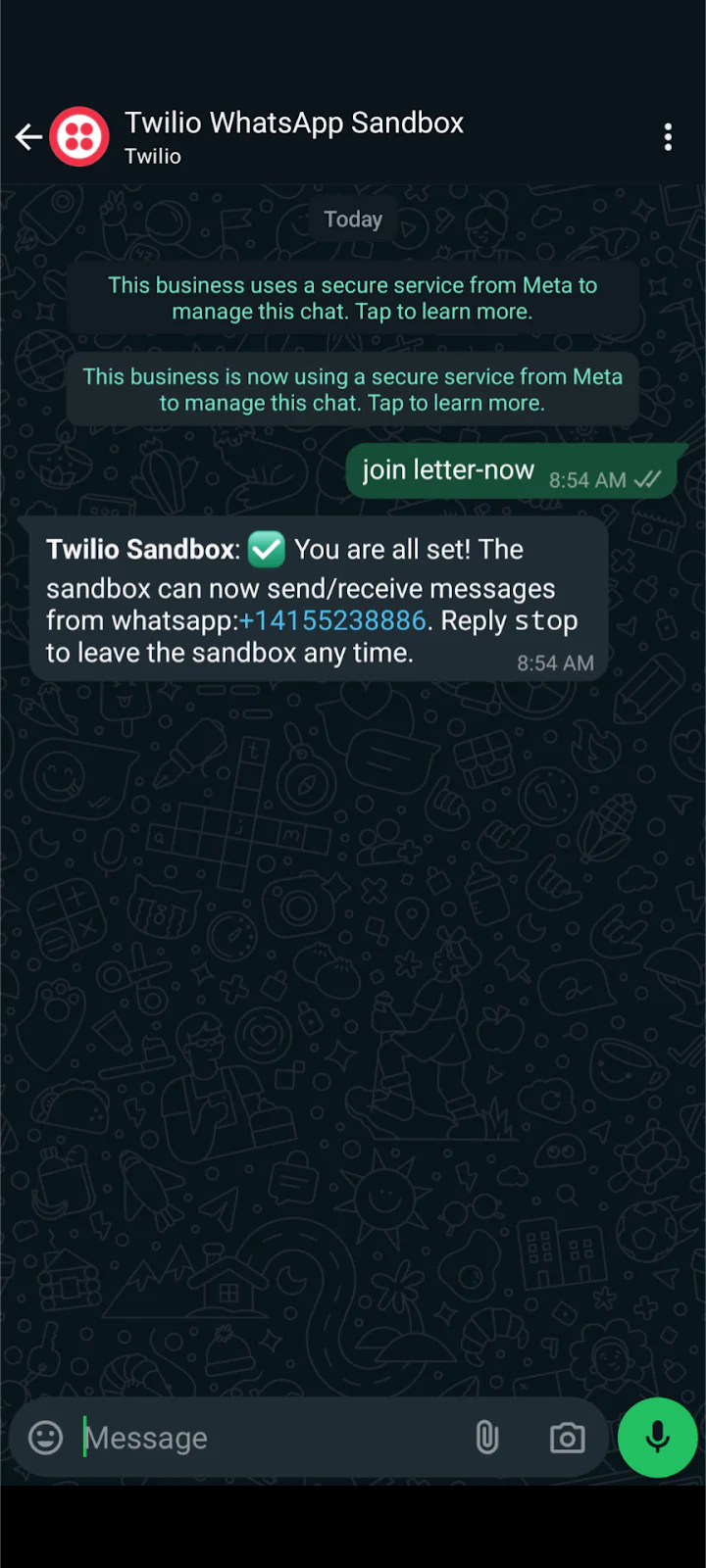
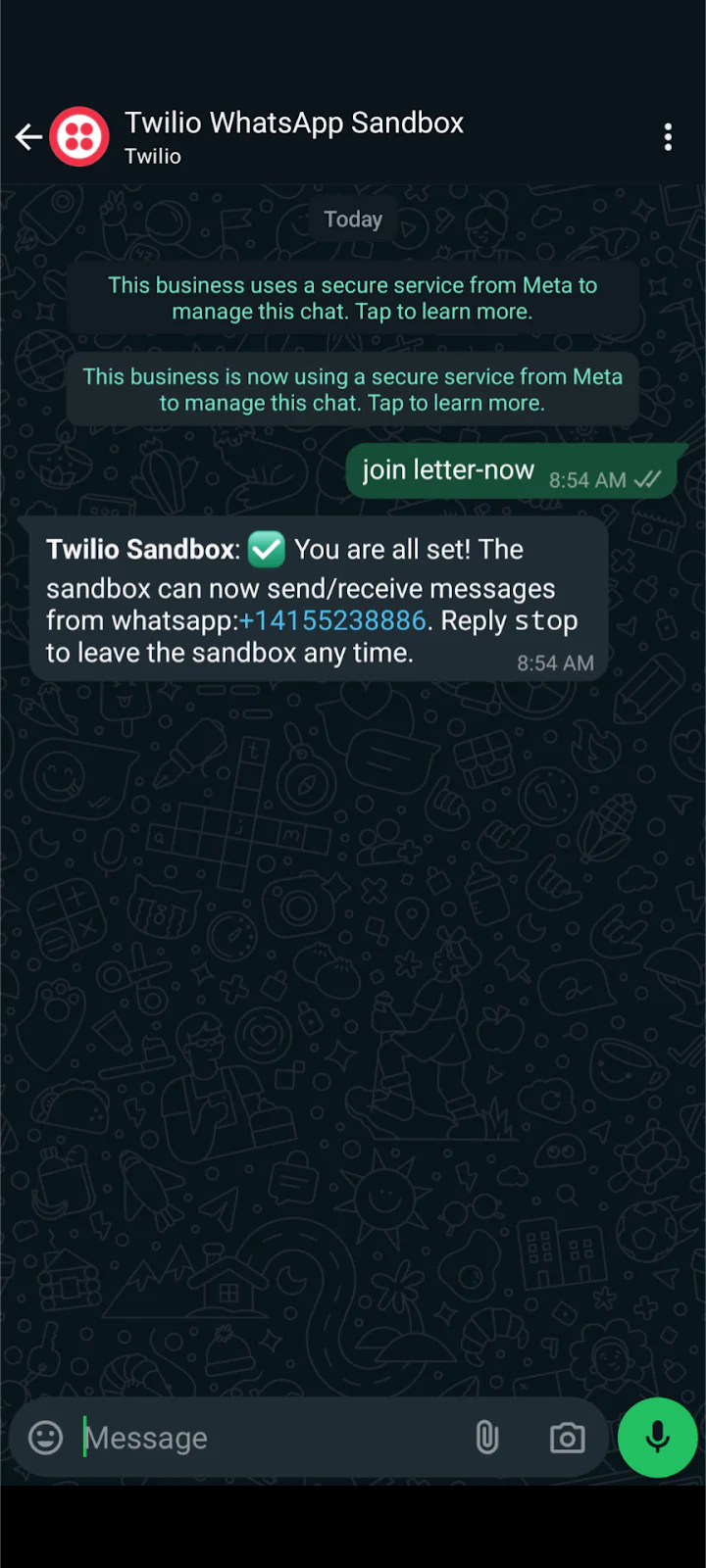
The sandbox account immediately responds to let you know that you are connected to the sandbox. Now, you can send test messages from your sandbox to this WhatsApp end user.
Send a test message
Next, send a business-initiated message from your WhatsApp sandbox to the connected end user. You can choose from the available content templates. For example, choose the Appointment Reminders type.
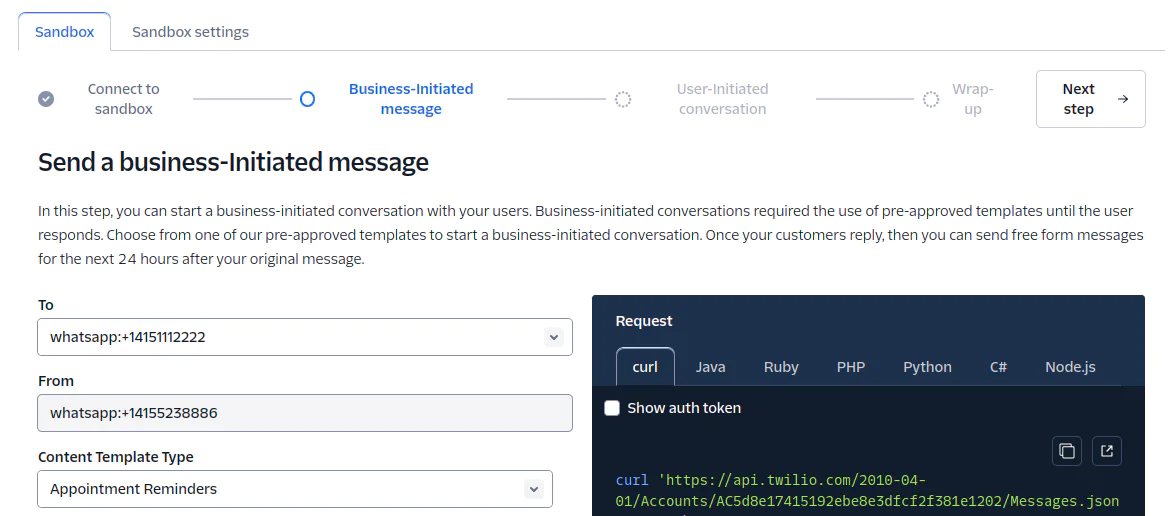
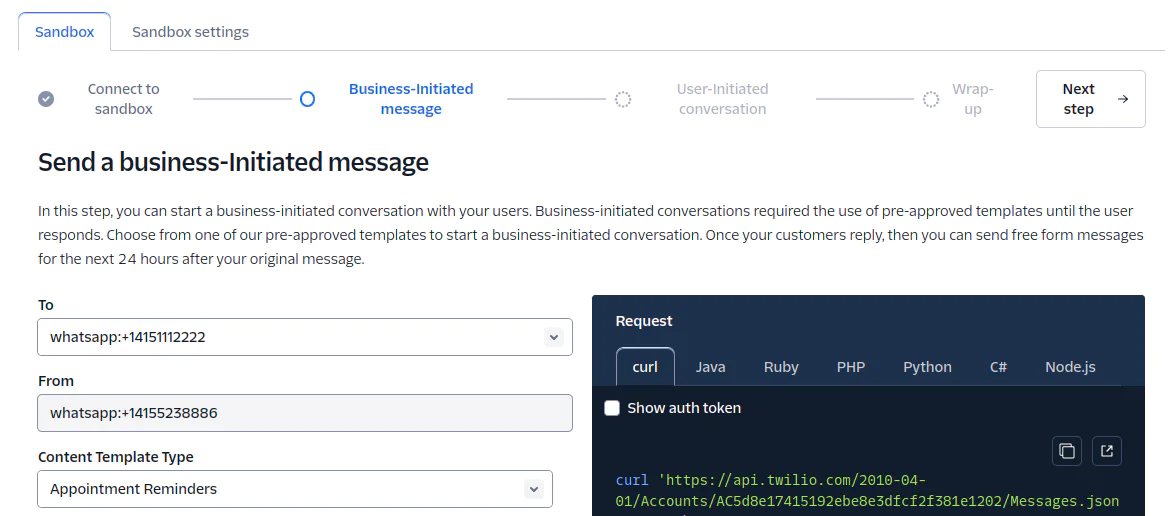
Content templates might have placeholders for you to insert on-the-fly values specific to the message recipient. Provide some test values for the date and time for this appointment reminder, and then click Send template message.
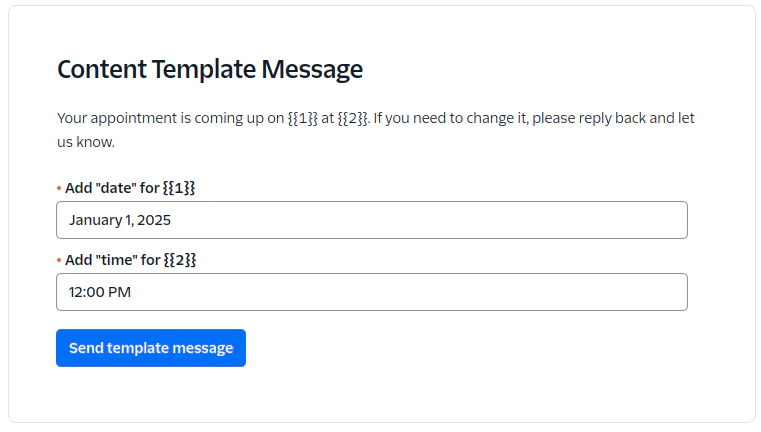
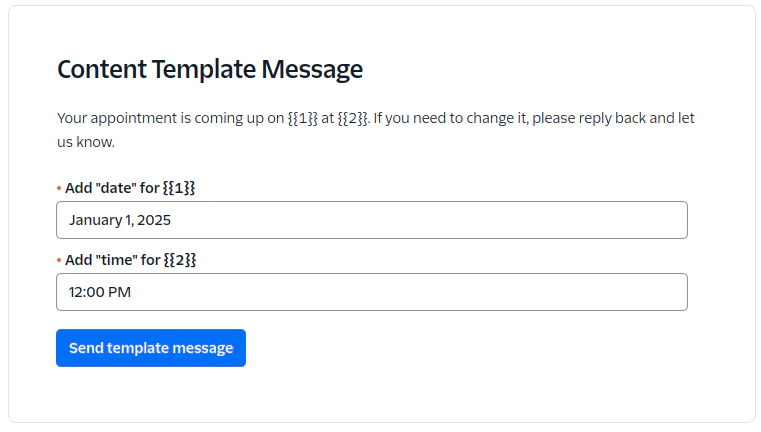
The sandbox will show the API request response, indicating a successful send. But there’s more – notice in the body
that the on-the-fly provided values have been inserted into the message template.
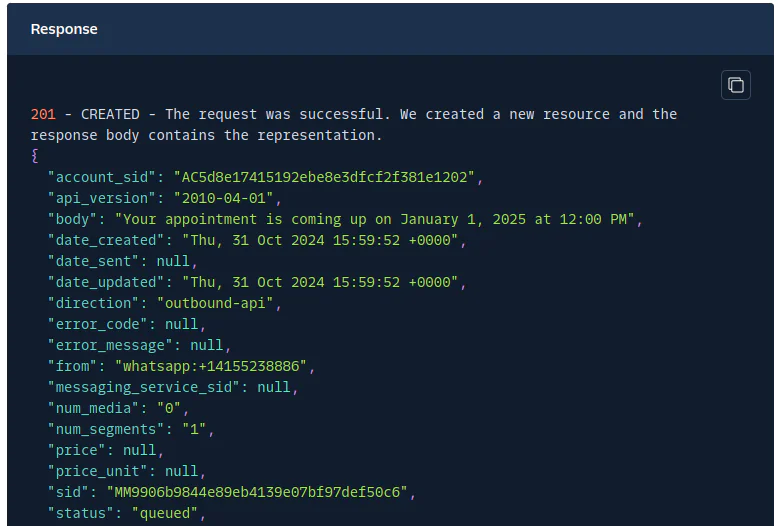
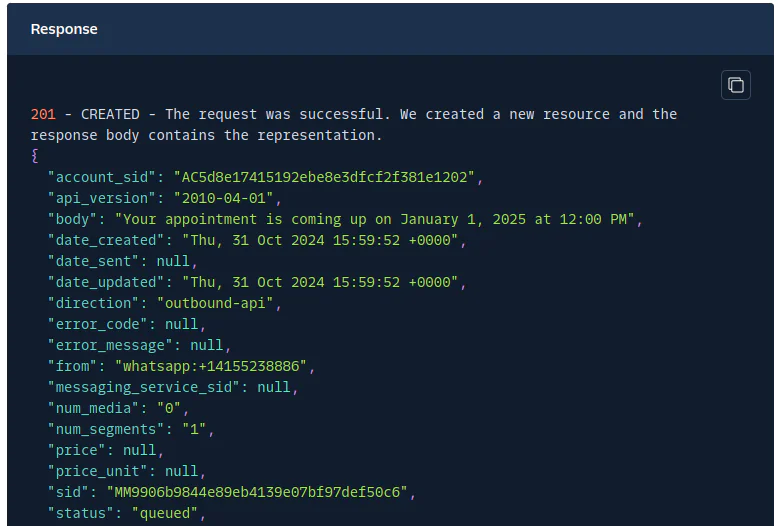
The intended recipient of the test message receives the message in their WhatsApp application. In this case, it’s probably your cell phone – check out how the appointment reminder template looks:
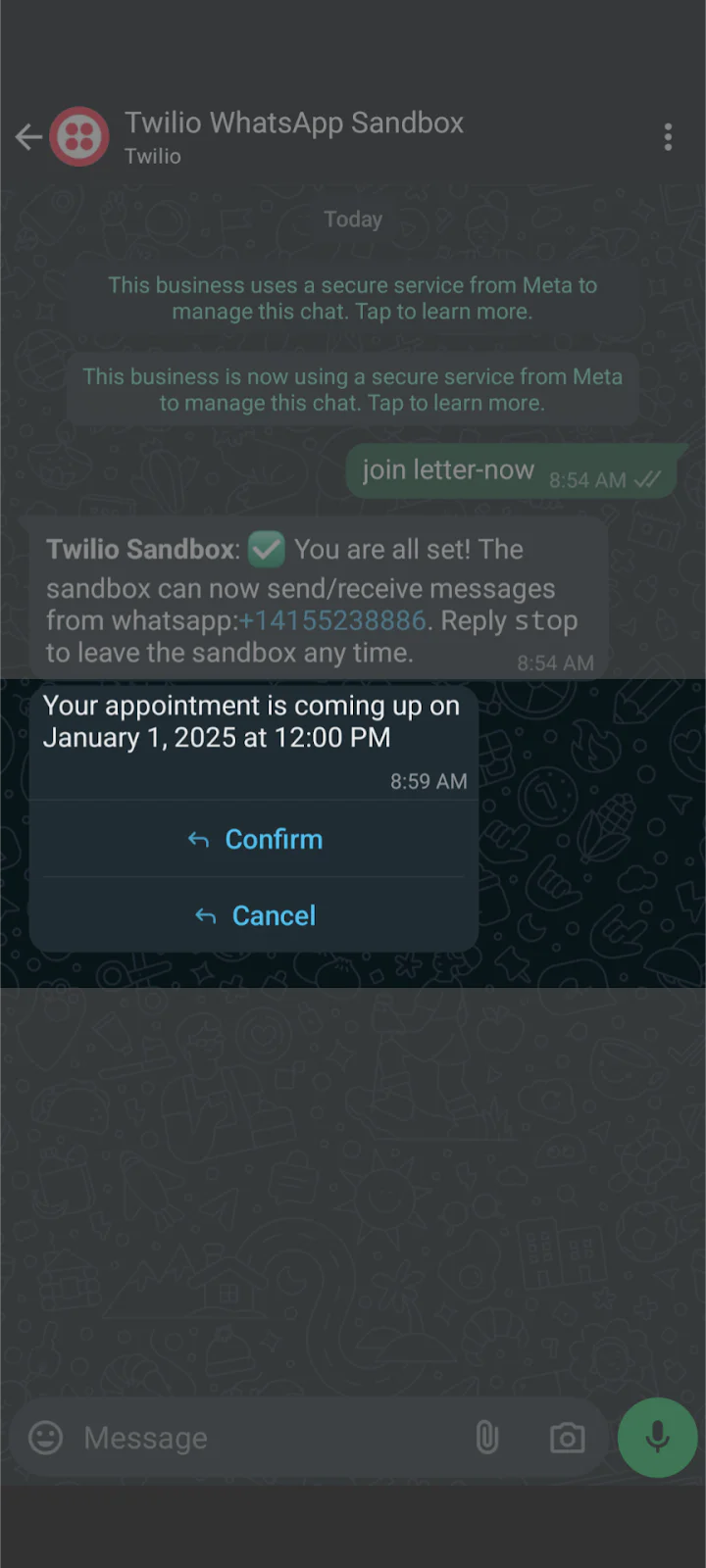
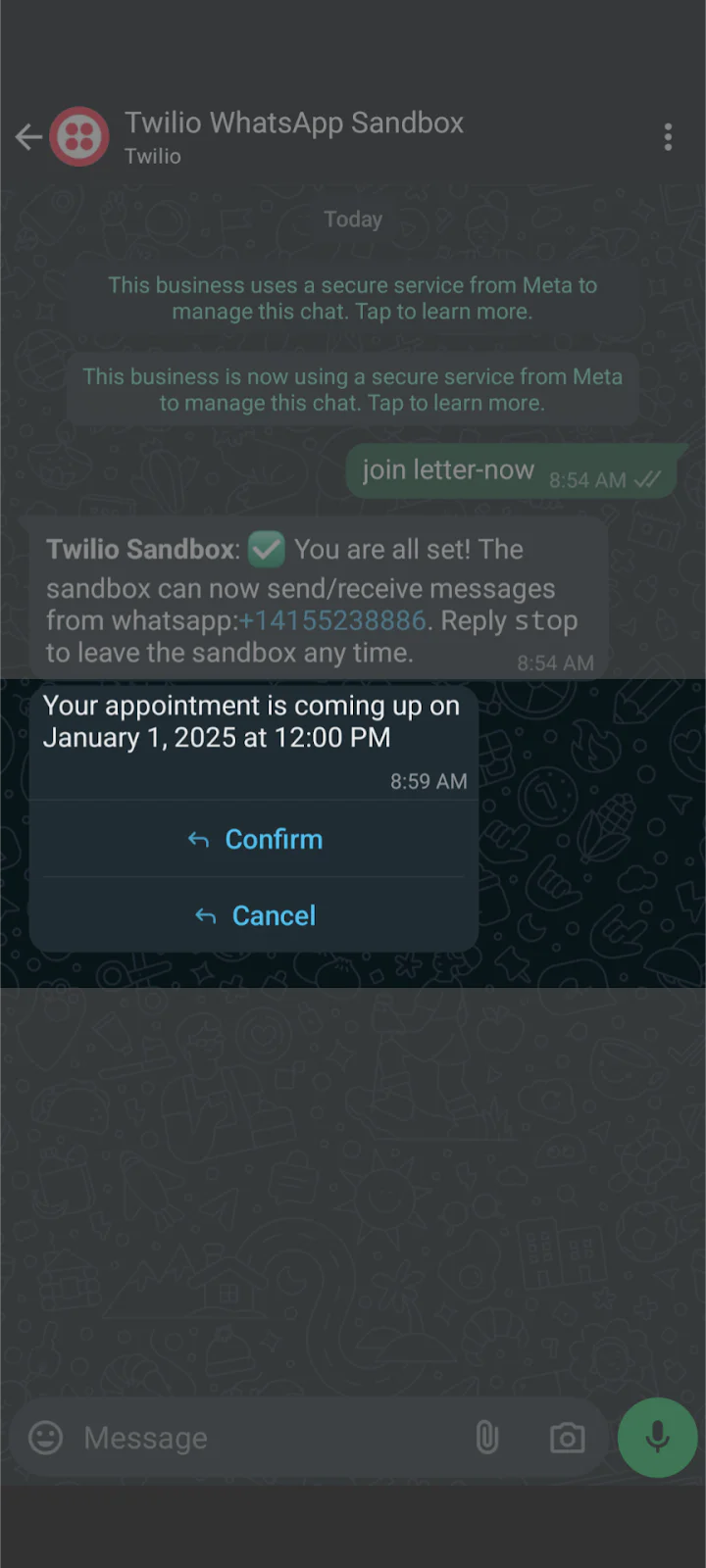
This is a great start for sending WhatsApp messages from your business to your customers. As you can see, you’re presented with the options to confirm or cancel the test appointment with a single click.
Let’s continue on to look at how we might use the Twilio Content Template Builder to build dynamic, rich content messages.
Working with the Content Template Builder
The Content Template Builder is a tool that lets you design reusable templates for rich content that can be sent across various messaging channels, such as SMS, MMS, WhatsApp, and Facebook Messenger.
What are content templates?
Content templates are predefined message structures that help you streamline communication by making your messaging consistent, repeatable, and easily programmable. They support rich media elements such as images, buttons (as you saw above), and text while adhering to the specific requirements of different messaging channels.
Create a content template
In the Messaging section of the left sidebar, click the Content Template Builder link.
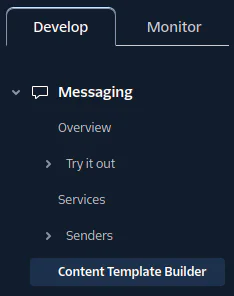
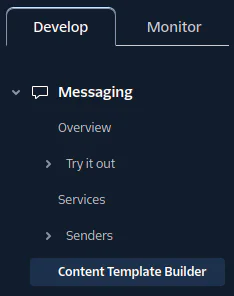
There, you’ll see a button to Create your first content template.
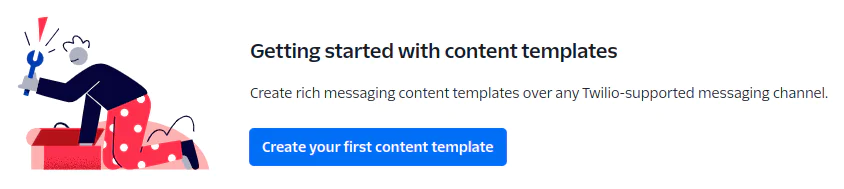
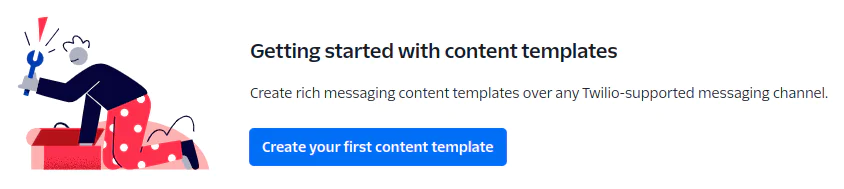
As you can see, Twilio provides the initial structure for several types of content. For each type, you can see the supported channels and a sample of what each message type might look like to the recipient.
We’ll create a template with the Media content type. Provide a name for the template and select a template language.
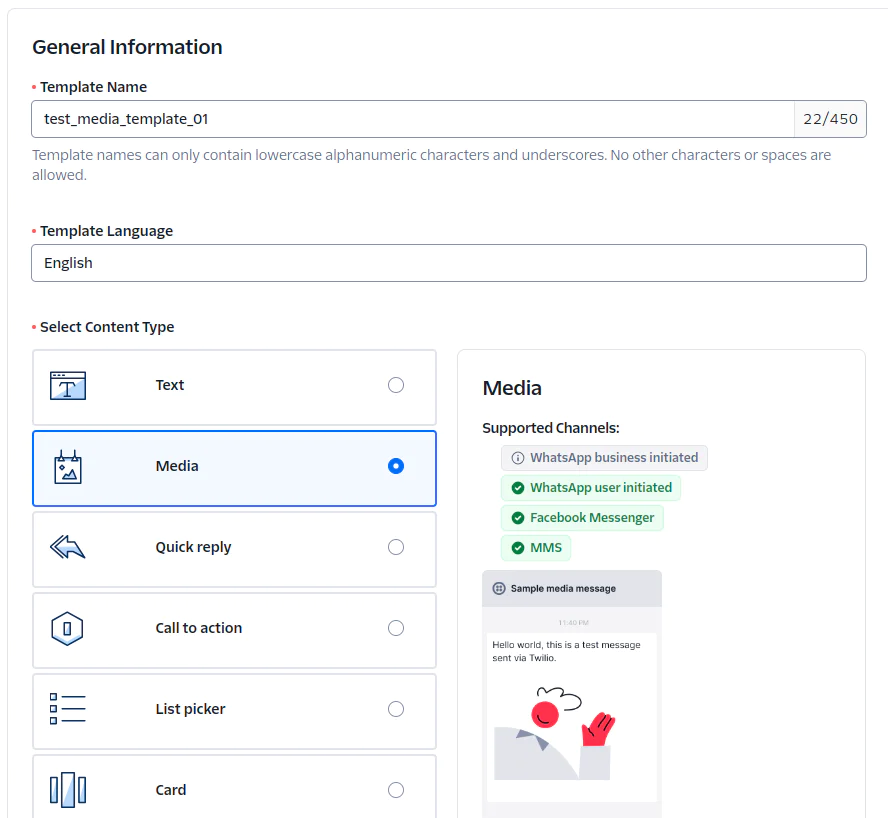
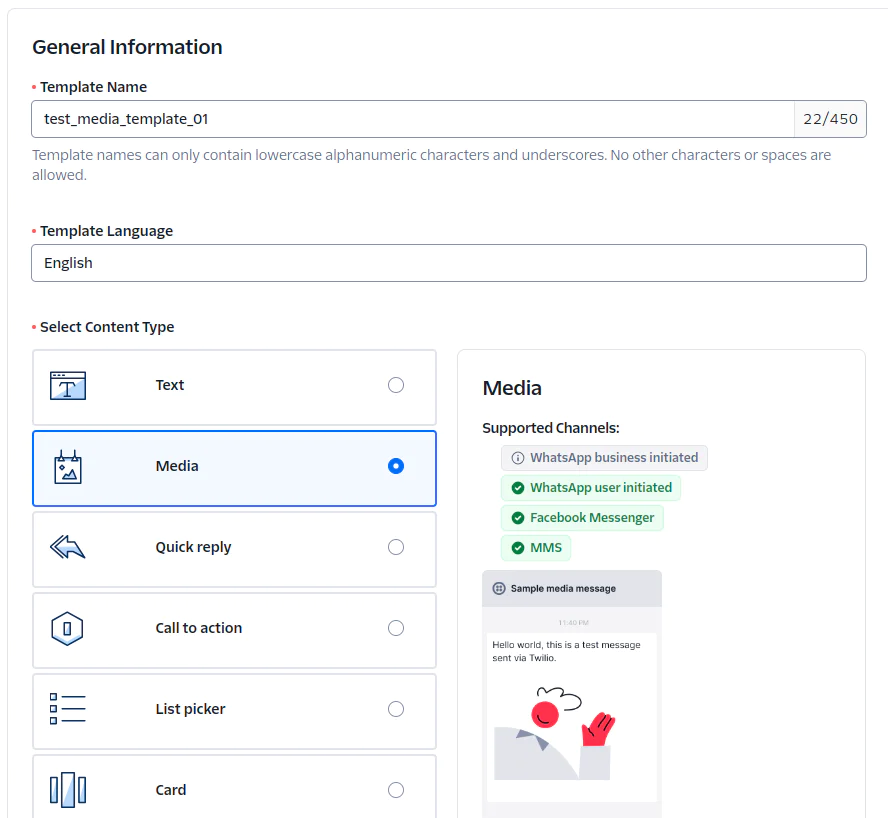
You’ll notice that the supported channels for this content type include Facebook Messenger and MMS, along with two different channels for WhatsApp: WhatsApp business initiated and WhatsApp user initiated.
WhatsApp business-initiated messages
A business-initiated message is the start of a conversation that your business initiates with a customer. In order to protect WhatsApp users from unsolicited messages, WhatsApp Business has strict requirements around how businesses can use WhatsApp to send messages, especially when the business initiates the conversation. When a business initiates a conversation with a customer, it must use a template that has undergone WhatsApp’s review and approval process.
WhatsApp user-initiated messages
On the other hand, a user may initiate a conversation with a business, perhaps sending an inquiry about an order status or a product offering. These user-initiated conversations open a session for 24 hours (from when the user sent the first message). In this scenario, WhatsApp Business is more permissive about the templates that a business can use to send follow-up messages.
You can see from the Media template type that a business-initiated message using this template must have the template pre-approved, whereas using the template in response to a user-initiated message does not require template approval.
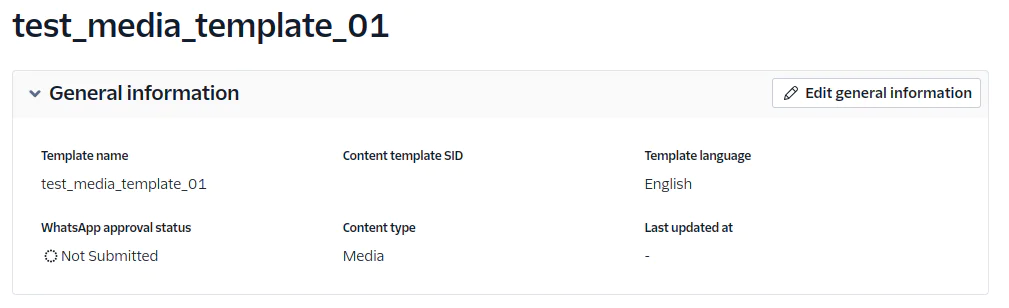
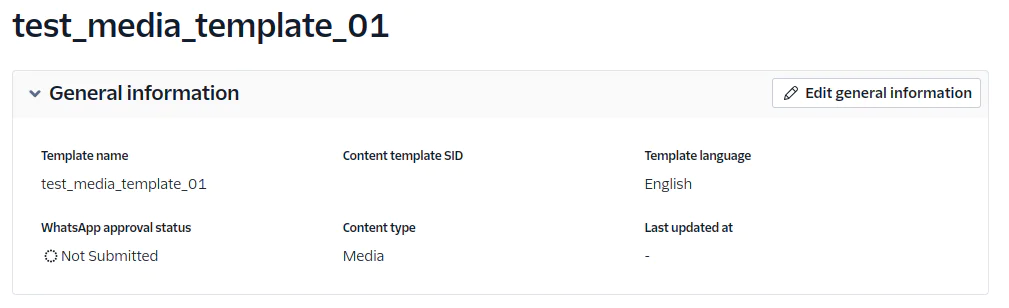
After completing this initial step of creating a template, you can continue on to configuring the template’s content.
Configure the template content
Using the Media type template as an example, we start by specifying a message body. When setting the message body, if you click Add Variable, it will insert a placeholder—such as {{1}}
—which you can use to inject a value on-the-fly when you send the message. The example below shows two variables in the message body: {{1}}
for the customer name, and {{2}}
for a coupon code:
For this content template type, we also provide a URL for a piece of media. In my case, I pointed to a publicly accessible image file. Alternatively, you can use Twilio’s Assets to host an image.
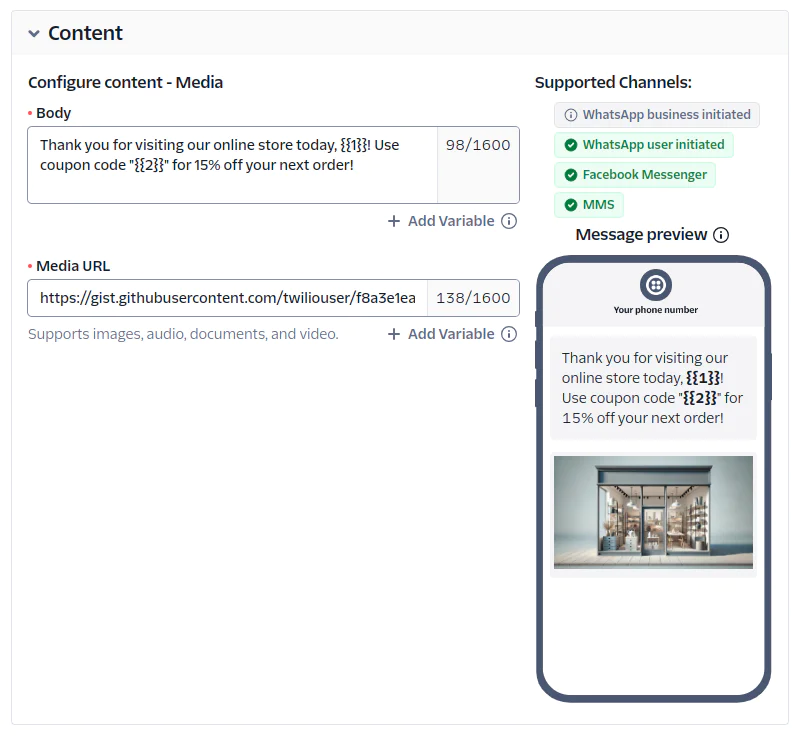
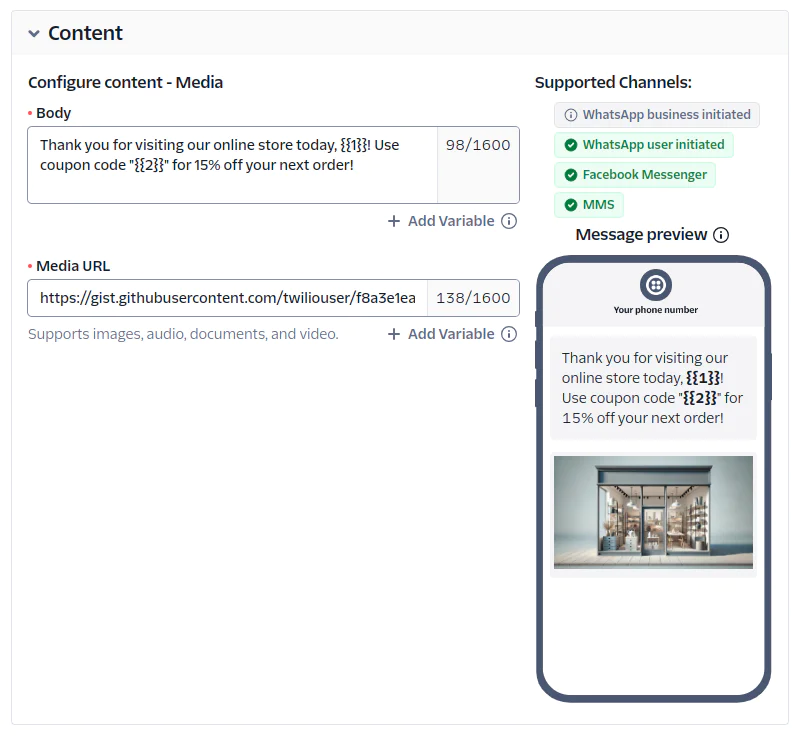
After entering the information for your content template, click Save. You’ll be given the option to provide sample values for the variables. Provide some sample values, then click Save with samples.
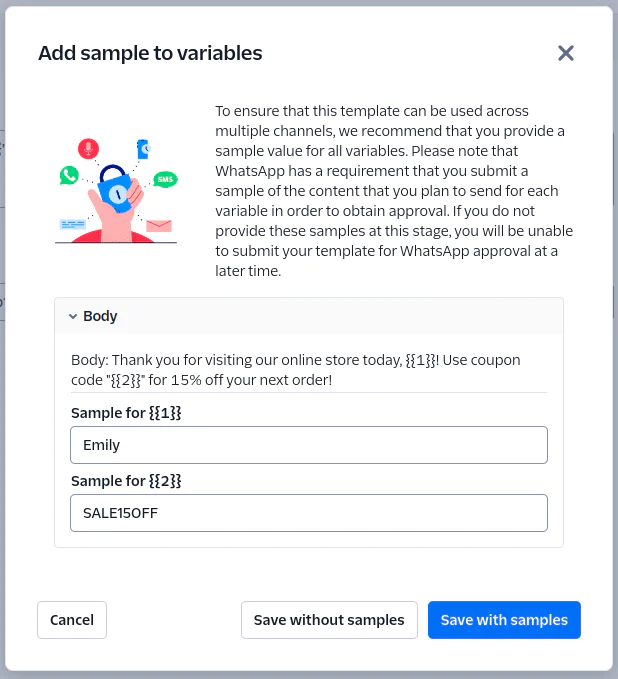
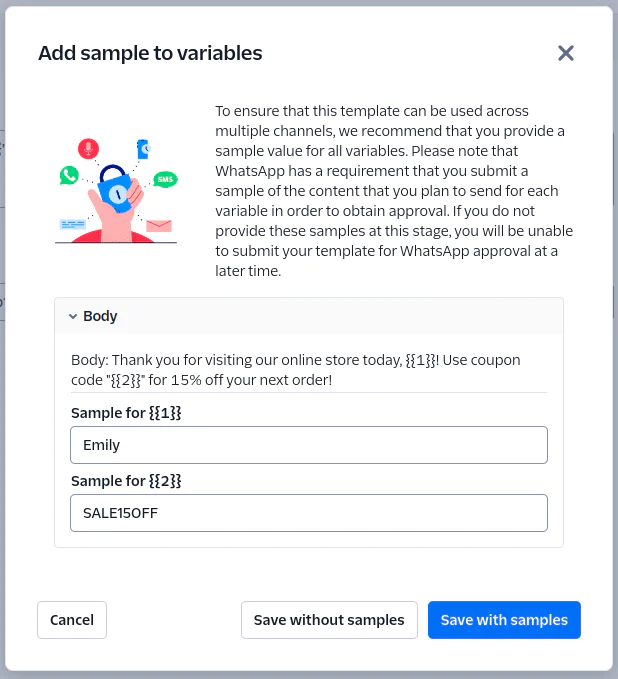
After you have saved your content template, you will see it listed on the Content Template Builder main page. Take note of the SID for your template, which appears in the leftmost column.
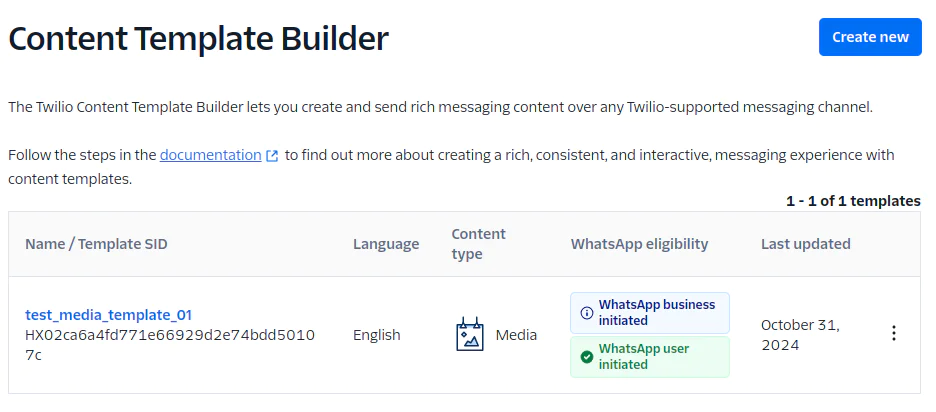
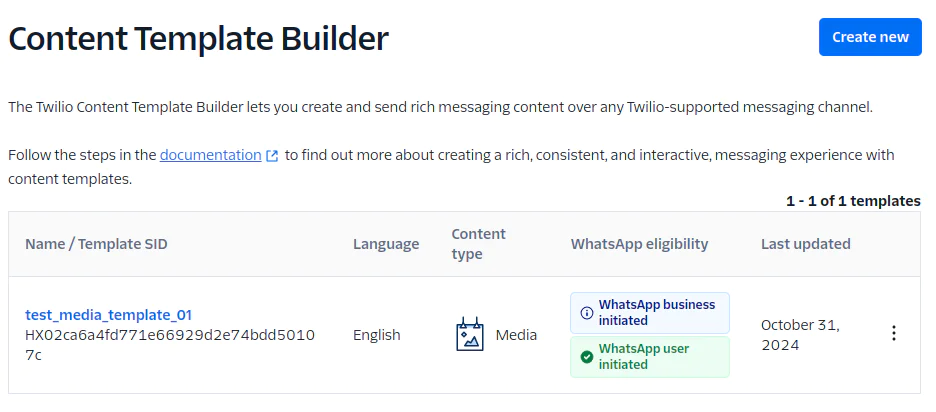
Working with the Content API
While the Content Template Builder is a web interface for building templates, you can also use Twilio’s Content API to programmatically build rich templates and send rich content from the command line.
Create a content template
Consider the Media type content template created above in the Content Template Builder. We can build the exact same template by sending a curl request to the API endpoint, https://content.twilio.com/v1/Content.
For authentication, you will provide your Twilio account SID and Auth Token. You can find these values on the Account management page of your Twilio Console.
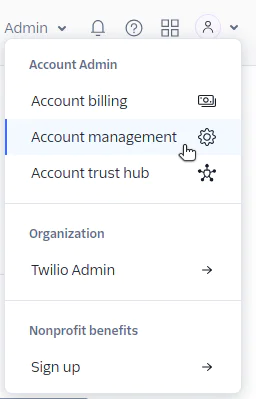
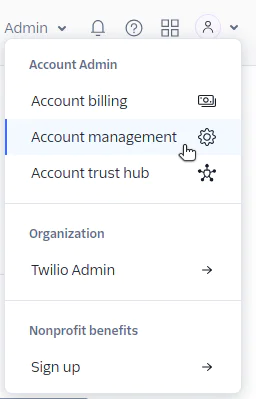
Once you’ve obtained these authentication credentials, send a curl request from your command line that looks like this (again, replacing TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
with the respective values, and friendly_name
with what you want to call the template):
After this request, when we look again at our list of templates, we see the newly created template included:
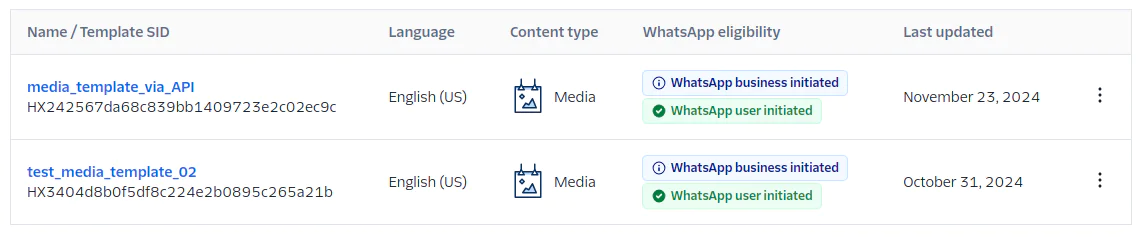
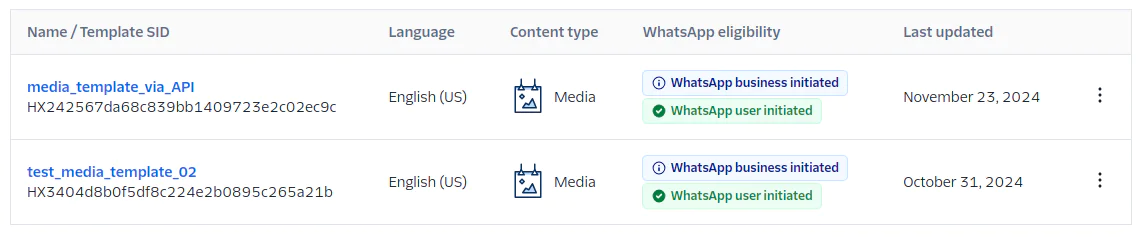
Send a message using a template
You can also use the Content API to send rich content via a templated message. To do so, you will need the following pieces of information:
TWILIO_ACCOUNT_SID
andTWILIO_AUTH_TOKEN
: For API authentication.ContentSID
: The SID of the content template you would like to use.From
: Either your Twilio phone number (or approved Twilio WhatsApp Business number) or Twilio Messaging Service SID. Change the+18885551212
to the number you’d like to test sending from.- If you don’t have one yet, info on how to find and purchase a Twilio number can be found here.
Let’s use the recently createdmedia_template_via_API
(if you used a different name, be sure to change it) template to send a message from our configured messaging service as an MMS message to a phone number. Our curl request would look like this:
The recipient at the To
phone number receives the following MMS:
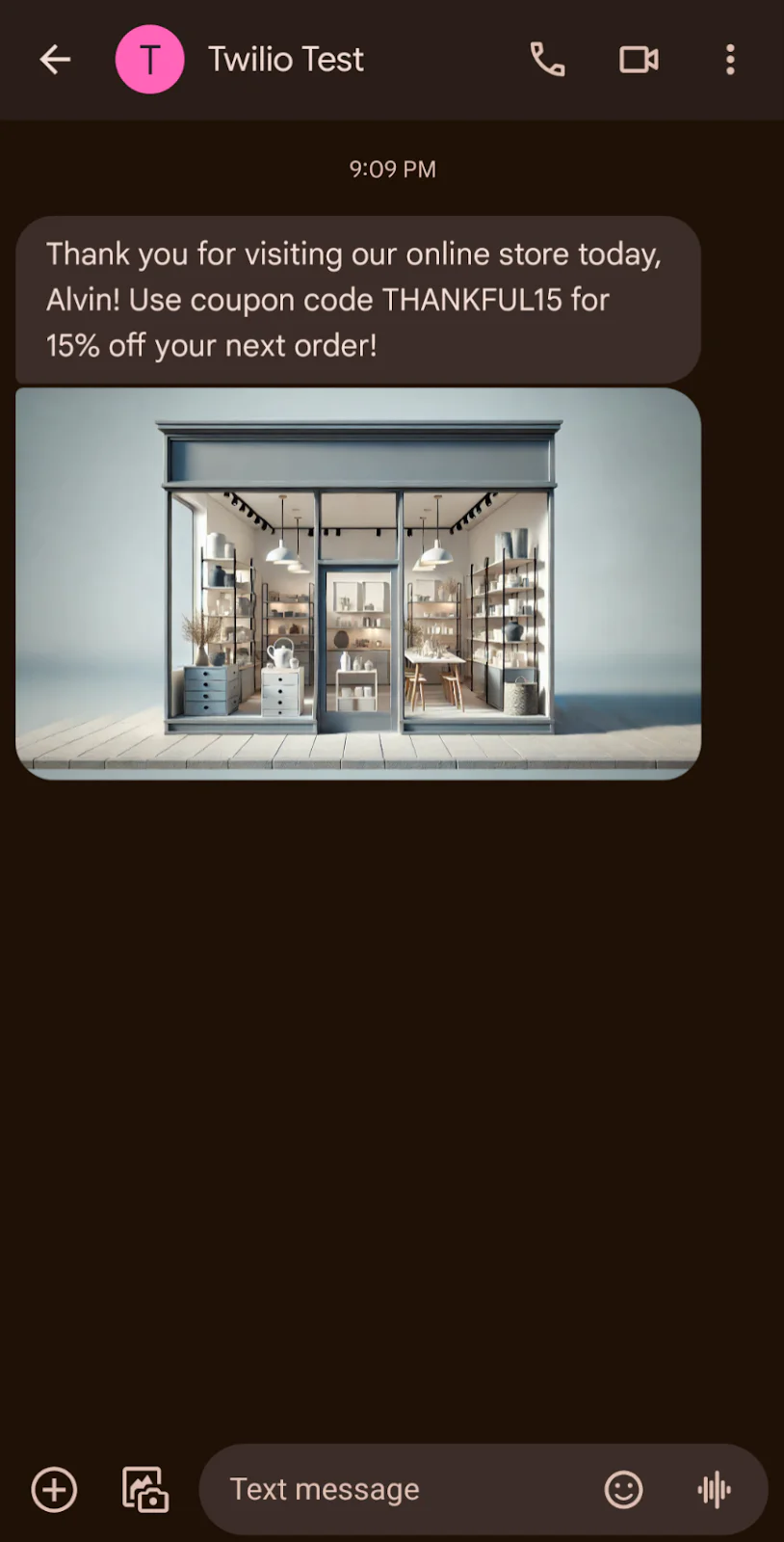
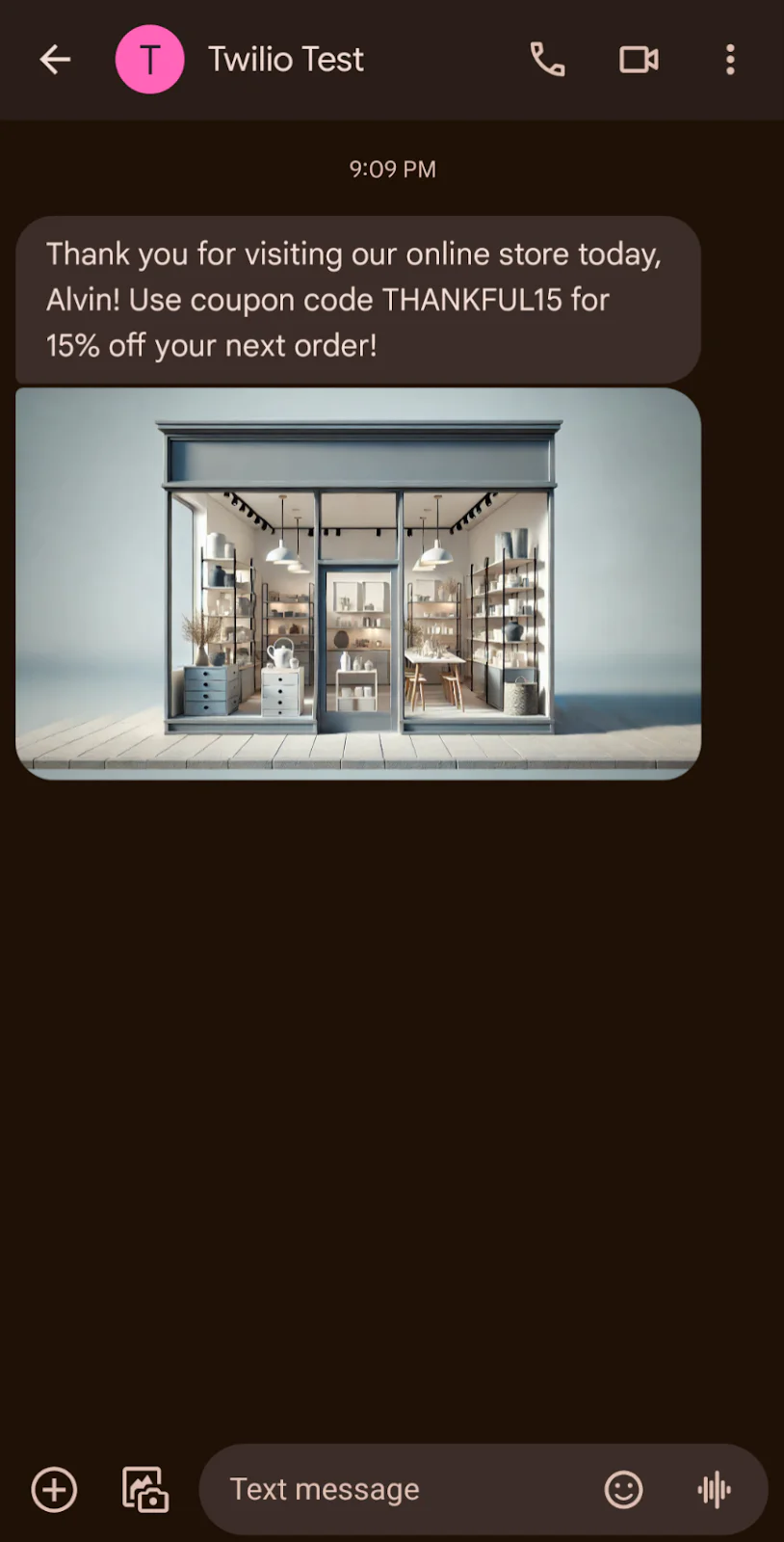
It worked! We were able to use the Content API to create a content template and then send a rich content message as an MMS to a phone number. As you
Considerations for Using WhatsApp
In the previous curl request, the To
value in the request data payload was a standard phone number formatted as +14151112222
. If we were to send to a WhatsApp recipient, the To
value would take on this format: whatsapp:+14151112222
However, before you can send content over WhatsApp, you need to complete a few steps.
1. Create a WhatsApp Business account
Before you can use your Twilio phone number to send WhatsApp messages, you must create a WhatsApp Business account. Complete this process first at the WhatsApp Business site.
2. Link your WhatsApp Business account to Twilio
After you have created a WhatsApp Business account, return to the Twilio Console. In the left sidebar, navigate to Messaging > Senders > WhatsApp Sender.
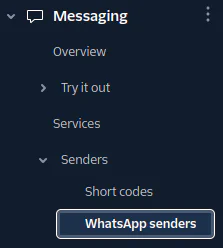
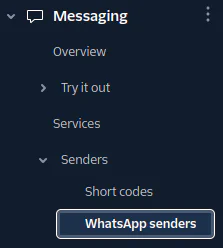
To create a new WhatsApp sender in Twilio, you will link your WhatsApp Business account with your Twilio phone number.
First, select your Twilio phone number.
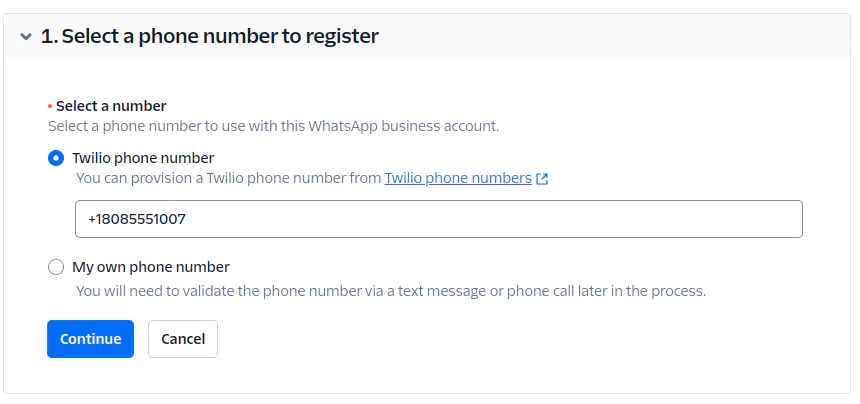
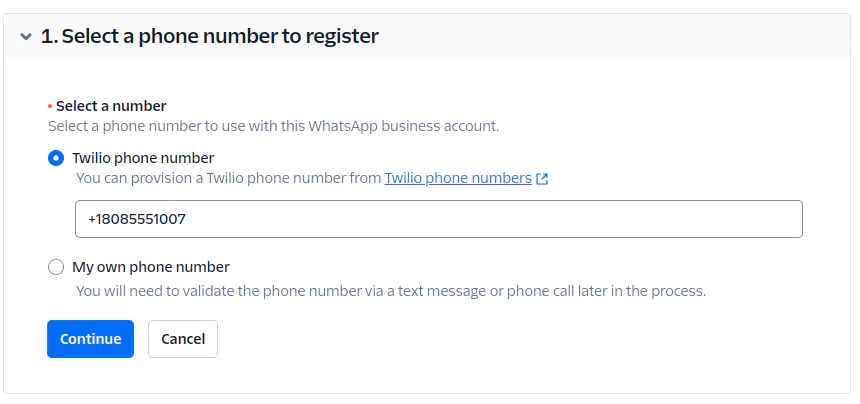
Next, you’ll authenticate with your WhatsApp Business account and then connect the account to your Twilio phone number.
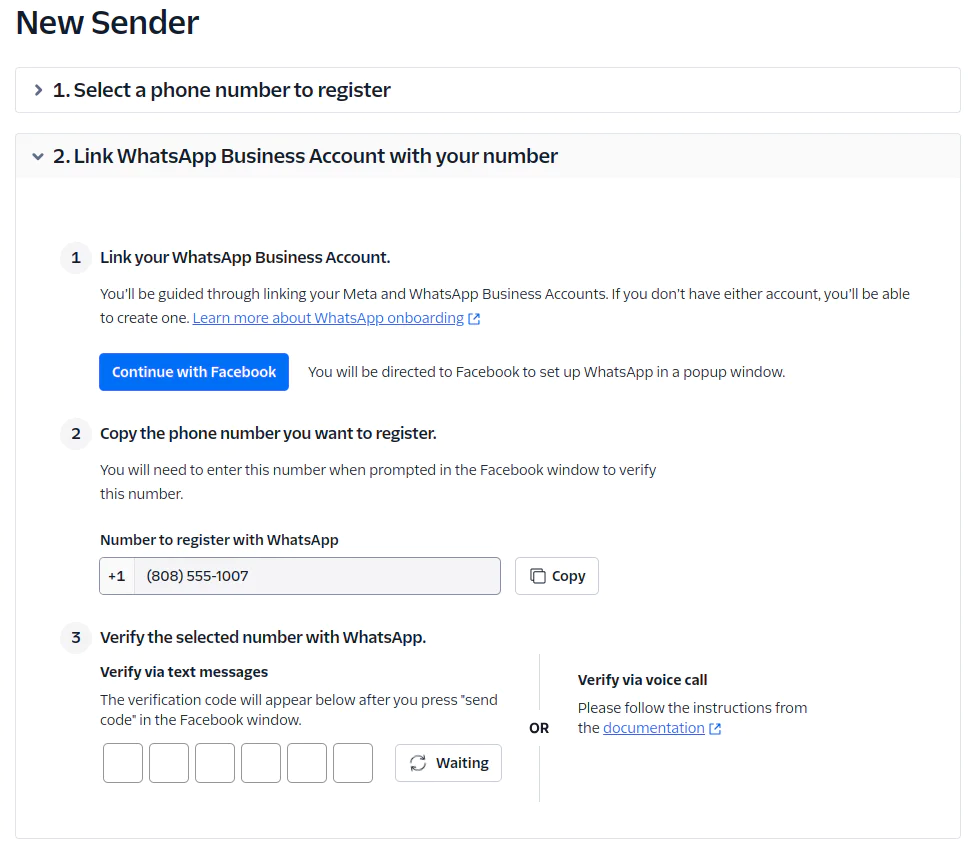
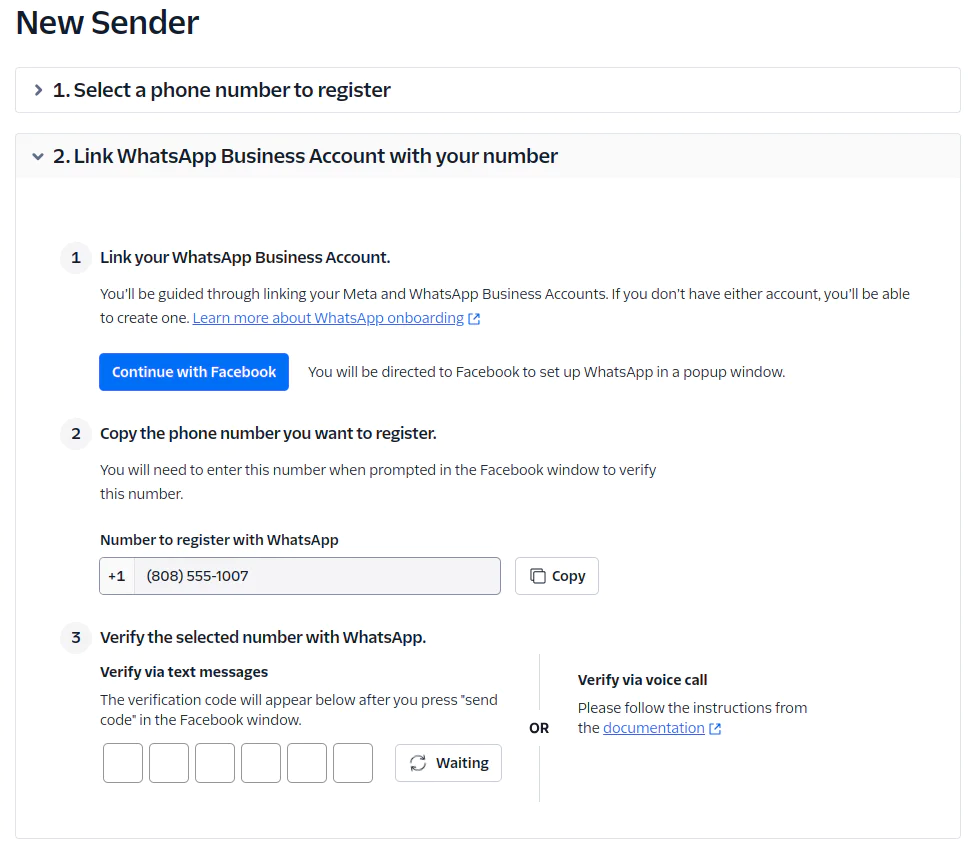
Once you have completed this process, you will be ready to use your Twilio phone number as an authorized WhatsApp Business messaging sender.
3. Submit content templates for WhatsApp approval
Finally, submit content templates for WhatsApp approval. Whether you are saving a content template for the first time or returning to an existing template, click the Submit for WhatsApp approval button to submit the template for approval.
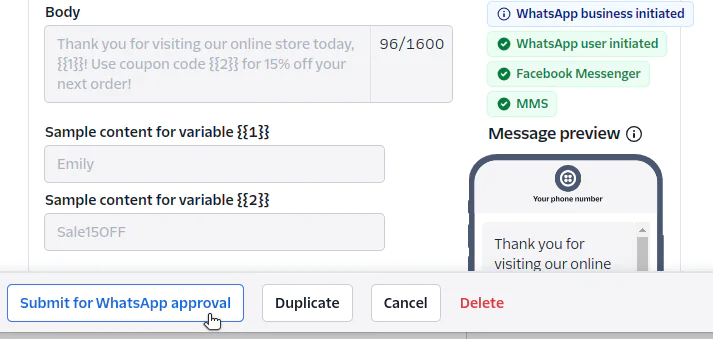
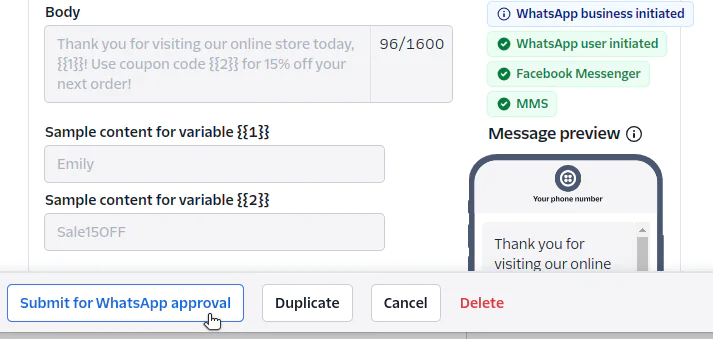
You’ll be asked to specify the template category. Click Submit.
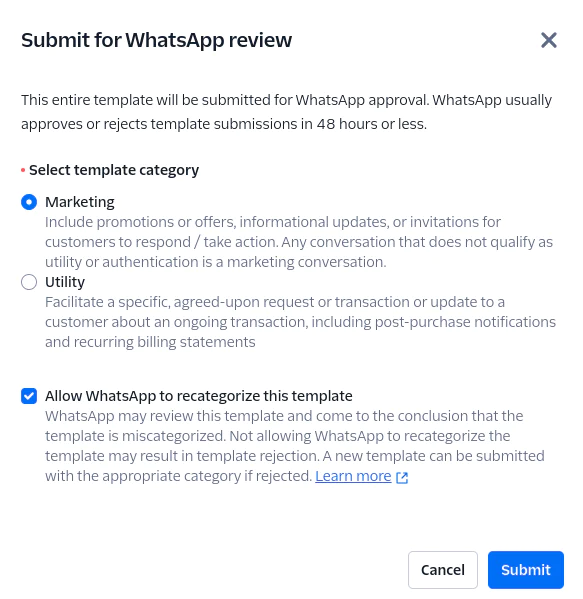
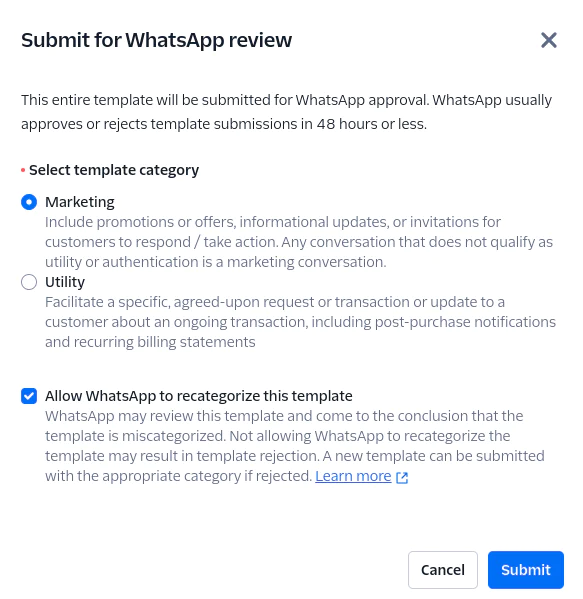
You should be notified with the review results within 48 hours. After your content template has been approved, you can begin to use it for WhatsApp business-initiated messages. Remember that some content templates can be used for WhatsApp user-initiated messages. Check the channel for any template type to know the requirements and restrictions for each type.
Wrap-up and Next Steps
In this guide, we’ve explored how to use Twilio’s tools to create and send rich, dynamic messages to your customers.
- Starting with the Content Template Builder, we walked through creating reusable templates for messaging channels like SMS, MMS, and WhatsApp. We touched on the need to submit content templates for WhatsApp approval if you’re planning to use them on that channel.
- We used the Content API to send these templates as messages, adding personalized content and media to deliver a more engaging customer experience.
- Finally, we covered the steps your business would need to undertake to create a WhatsApp Sender with Twilio, including the need to create a WhatsApp Business account.
By sending messages with WhatsApp Business and Twilio, you quickly unlock the ability to reach your customers through a powerful and immersive channel. But you send over WhatsApp or SMS and MMS, using the Content Template Builder and the Content API will level up your ability to engage your customers more efficiently and effectively.
As you grow your messaging strategy, consider exploring additional channels like RCS to further enhance your customer interactions.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.