Twilio for .NET Developers Part 3: Using the Twilio REST API Helper Library
Time to read: 5 minutes
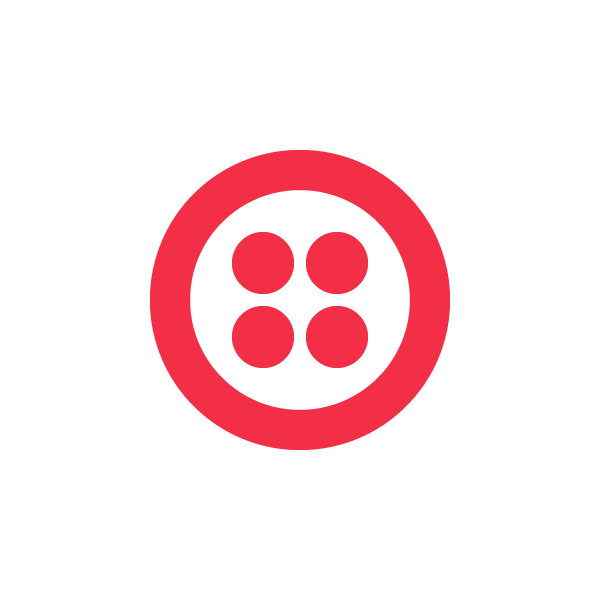
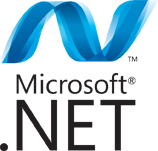
This is a series of blog posts that introduce and walk you through using the different .NET Helper Libraries that Twilio provides.
These libraries simplify using the Twilio REST API for .NET developers, and provide as set of utilities that make it easy to work with TwiML and Twilio Client. Make sure to read part one and part two of the series.
Using the Twilio REST API Helper Library
In this post we’ll look at how you can use the helper library to make calls against the Twilio REST API. The REST API allows you to initiate outbound phone calls, send SMS messages and send or receive other data to or from Twilio. The .NET helper library wraps the entire REST API in a set of managed API’s allowing you to access the same capabilities exposed by the REST API, but via a familiar .NET API. This frees you from having to understand the ins and outs of making REST calls and receiving JSON results in .NET.
To get started make sure you’ve added the Twilio helper library to your project using NuGet:
The main class you will use from the helper library is the TwilioRestClient class. When you instantiate this class you must provide your Account SID and Auth Token, which you can get from your twilio.com account dashboard (if you haven’t done so already, head over to twilio.com, create a new account):
Now that you’ve create an instance of the TwilioRestClient class, you can start interacting with the API. To show the basics of how helper libraries API, let’s use it to send an SMS message by calling the SendSmsMessage method:
By default, the SendSmsMessage method takes three parameters, the To and From phone numbers and the Message. The values provided for the To and From parameter must be a Twilio phone number, or a number you have verified with Twilio and both the To and From values must be formatted according to the Twilio phone number formatting rules. You can consult the Twilio documentation for more information:
Every method exposed by the TwilioRestClient class returns an object that has status information about the call. In the case of the SendSmsMessage method, it returns an instance of the SMSMessage object, which you can use to check the status of the call:
In this sample we are using the SMSMessage object to check the RestException property to see if an error occurred during the call to the REST API. We’ll look at using the RestException property to help debug application errors in a future blog post.
By default the SendSmsMessage method runs synchronously, meaning that it will block your application from continuing until it completes. Since you are making a call to a remote web service, its possible that the call may take some time, meaning your application will be unresponsive. To help this problem, many of the methods allow you to provide an Action; parameter which will be raised by the method when it completes execution:
In this example, the test for a RestException value is encapsulated into an anonymous method that will be called when the SendSmsMessage method completes.
Finally, some of the REST API calls allow you to specify a large number of options as part of the REST request, most of them optional. Rather than exposing methods with large numbers of optional parameters, the .NET helper library translates these into objects that you can set the properties on, and then provide to the methods.
To see an example of this, let’s look at how you can use the InitiateOutboundCall method to make outbound calls from Twilio. The default method signature for the InitiateOutboundCall accepts an instance of a CallOptions object. This object allows you specify all of the different properties allowed by Twilio.
In this example we are not only specifying the To, From and Url parameters required by the REST API, but also setting the Timeout and IfMachine parameters. In this case the Url we specify will be the Url that Twilio calls when the call is answered. Once we’ve got all of the options set, we simply pass the entire CallOptions object to the InitiateOutboundCall method.
One other important item to note from this example is the format of the value provided to the Url property. When specifying Url values using the helper library, you should always make sure to provide absolute Urls.
That’s all you need to use the Twilio.API library to access the REST API. In the next post we will look at how you can use the Twilio.TwiML library to generate TwiML responses.
Read the rest of the .NET series:
Twilio for .NET Developers Part 1: Introducing the Helper Libraries
Twilio for .NET Developer Part 2: Adding Twilio Helper Libraries to your Project
This series is written by Twilio Developer Evangelist Devin Rader. As a co-founder of the St. Louis .NET User Group, a current board member of the Central New Jersey .NET User Group and a former INETA board member, he’s an active supporter of the .NET developer community. He’s also the co-author or technical editor of numerous books on .NET including Wrox’s Professional Silverlight 4 and Wrox’s Professional ASP.NET 4. Follow Devin on Twitter @devinrader
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.