Verify Phone Numbers with Java and the Twilio Lookup API
Time to read: 6 minutes
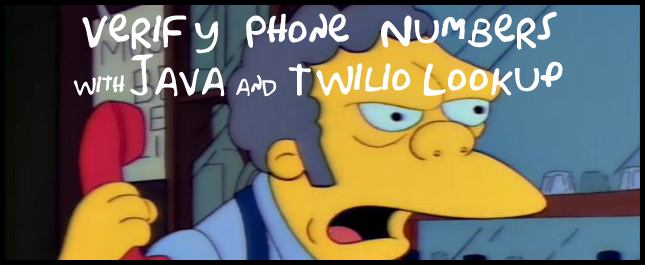
Twilio Lookup is a simple REST API for obtaining information about a phone number. Lookup can determine if a number exists, its type (landline, mobile, VoIP), and its carrier (Verizon, Sprint, etc) association. Lookup can also check if a number is able to receive text messages as well as format numbers into a standard format.
Let’s validate phone numbers using the Twilio Java library and the Lookup API and make the code easy to port into your existing Java applications. This post will be directed towards macOS and Linux users, as some of the commands will not work for Windows users.
If you just want to see the finished code, see this Github repository.
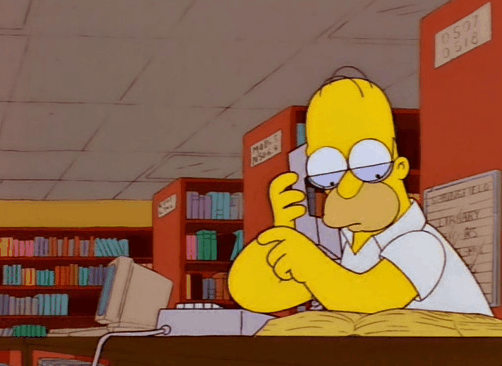
Configuring Our Environment
Our code will use the latest version of Java 8 (1.8.0_121). You can download the latest version of the Java JDK from here. You can test your Java install and see what version is configured by running java -version
from your terminal.

We will also be using Gradle as our build tool and for dependency management. Follow the Gradle Installation guide and run gradle -v
in your terminal to check that Gradle is installed and using the same version of the JVM as above. If not, you may need to re-configure your JAVA_HOME
environment variable. Note: Your version of Java does not need to be the same as mine, but it is important that gradle is using the same JVM version as your system.

Additionally, make sure you have a Twilio account. A trial account will be sufficient for this post.
Getting Started
Create a new directory for our app and navigate to it using the following command:
Create a new file in this directory called build.gradle
. This file will contain all of the project’s build settings. Gradle is an extremely powerful tool, but like most build tools it can be overwhelming to a beginner. We’ll try to keep our interactions with it as simple as possible.
Next we need to create our Java file which will contain our source code. Gradle expects to find your source code under the src/main/java
directory. In the same terminal run the following:
Navigate down to the lookup directory and create a new file called Lookup.java
.
build.gradle
and Lookup.java
are the only two files we need, the rest will be handled by Gradle.
Before moving on, you should store your Twilio credentials as environment variables by running the following commands:
This will allow us to use Twilio later without having to save our credentials to source code. Your ACCOUNT SID
and AUTH TOKEN
can be found on your Console Dashboard.
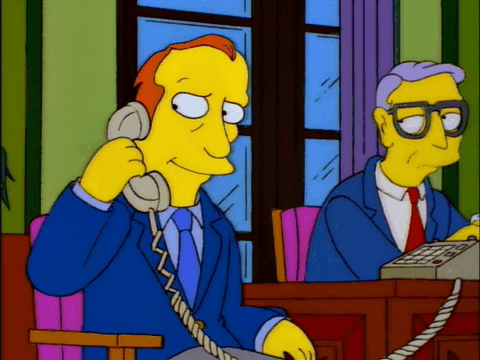
Configuring Gradle
Open the build.gradle
file at the top of your directory in your preferred editor and add the following, then we will go over what it does:
The first line of the file is applying the application plugin to our Gradle project. Plugins, when applied, extend the project’s capabilities. This plugin adds Java compilation, testing and bundling capabilities, and adds tasks for running and bundling a Java project as a command-line application.
The next line tells the application plugin which class in src/main/java/
contains the app’s main()
method.
The repositories
and dependencies
blocks declare and configure the project’s dependencies. Rather than copying a bunch of Twilio code into our own files, we are using the Twilio Java SDK. Gradle will handle fetching and storing this code for us later.
Looking Up Valid Phone Numbers
Our environment is set up and we can finally write some Java. You can play around with the Lookup page to see what type of data a request to the Lookup API will return. Enter a phone number and take a look at the JSON response object.
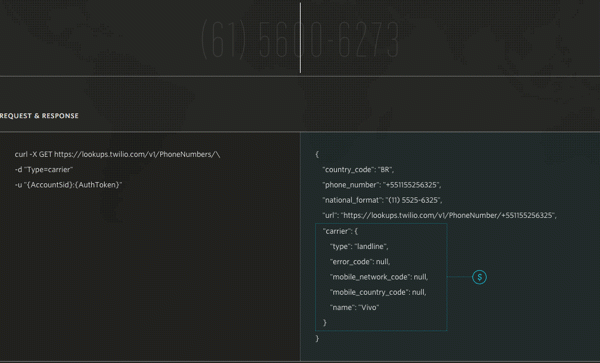
Open up src/main/java/lookup/Lookup.java
and start by adding the following at the top of the file:
These lines will add our file to the lookup
package and import the Twilio classes we’re going to use. Below those lines add the following:
At the top of the class we define our Twilio ACCOUNT_SID
and AUTH_TOKEN
using the environment variables set earlier. Inside of the class’s main
method we initialize Twilio, create a PhoneNumber
object, call it’s fetch
method, and print the results in the national format.
Note: It’s okay to hard-code a phone number in this case because we are only looking it up and it is not associated with our Twilio account.
Save this file and return to your terminal. We need to use Gradle to compile and run the app. Gradle allows you to execute multiple tasks in a single command by simply listing each task in the order you want them to run. Run the following:
This command might take a while the first time you run it because it will need to start the Gradle daemon and download the dependencies we need.
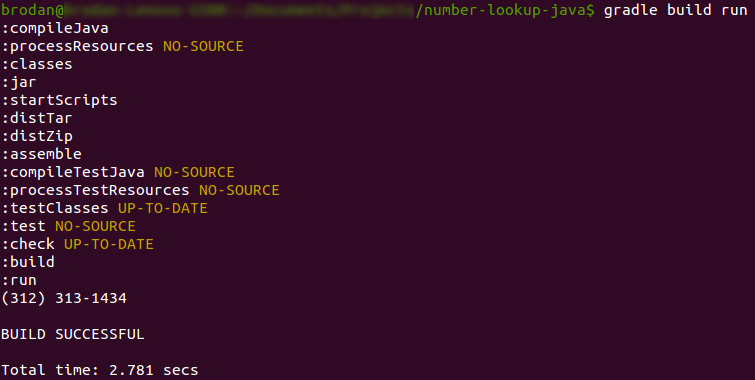
You’ll notice a mess of things printed to the terminal because a lot just happened. Gradle first built your project by compiling all of the project’s classes, and then started the application using the application
plugin’s run task. Notice that the desired output is also printed above the BUILD SUCCESSFUL
message. If you only want to print the application’s output and nothing from Gradle, add the —quiet
flag the above command (e.g. $ gradle build run —quiet
).
More Informative Lookups
Basic functionality like number formating is free, but for a small cost you can use Lookup to get useful information about any phone number. Let’s change our app so that it can retrieve a number’s type and carrier. Modify Lookup.java
as follows:
Run your app again with $ gradle build run —quiet
and you’ll see some more useful output than before.
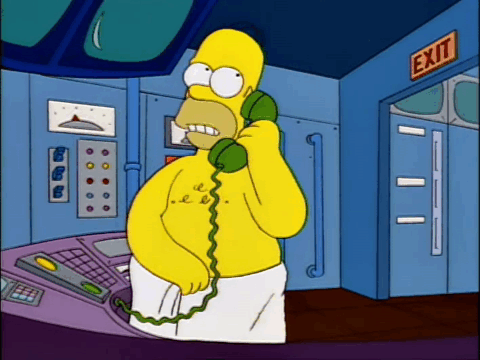
Looking Up Invalid Phone Numbers
By now you’ve probably played around with the code to Lookup numbers other than 13123131434
. It’s possible that you looked up a phone number that isn’t valid (such as (555)555-5555
). If so, you would have seen that an exception was raised because the number could not be found:
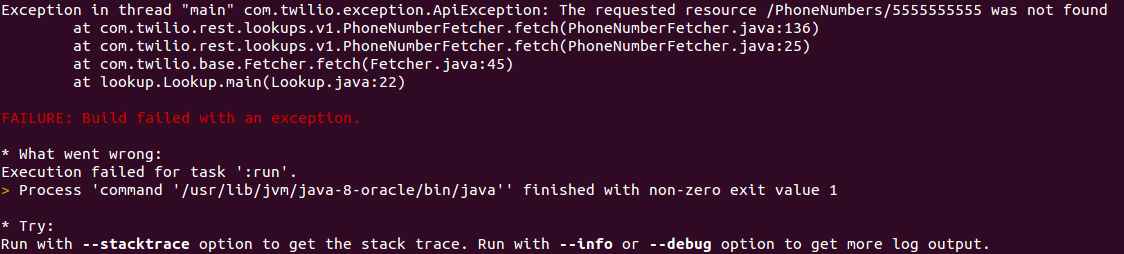
We need to add some error handling to our app so that it doesn’t crash when an invalid number is looked up. Modify Lookup.java
again to include the following:
Now if you look up an invalid phone number you’ll see Phone number not found.
printed to the terminal instead of the exception being raised.
Using Command Line Arguments
We have a finished, working application. You can stop here, but it’s a pain to look up a new number because you need to open Lookup.java
, change the hard-coded phone number, and then clean, build, and run the Gradle project. Let’s make the application more dynamic by modifying it to accept a command line argument.
Open up build.gradle
again and add the following to the bottom of the file:
Then in Lookup.java
modify the main
method as such:
We can now look up new numbers without modifying our code. The -P flag is used to set properties for the build script (see $ gradle -h | grep "-P"
). Try the following:

Wrapping Up
This completes the introduction to Twilio’s Lookup API. For more information on Lookup, see the API Docs and the auto-generated JavaDoc for Twilio’s Java SDK.
If you want to see guides on the Lookup API in other languages, they are available in Python, Ruby, PHP, and NodeJS.
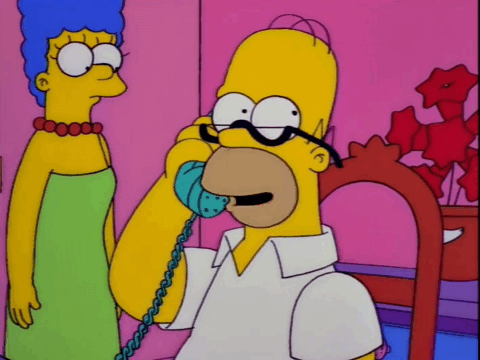
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.