ETA Notifications with C# and ASP.NET MVC
Time to read: 2 minutes
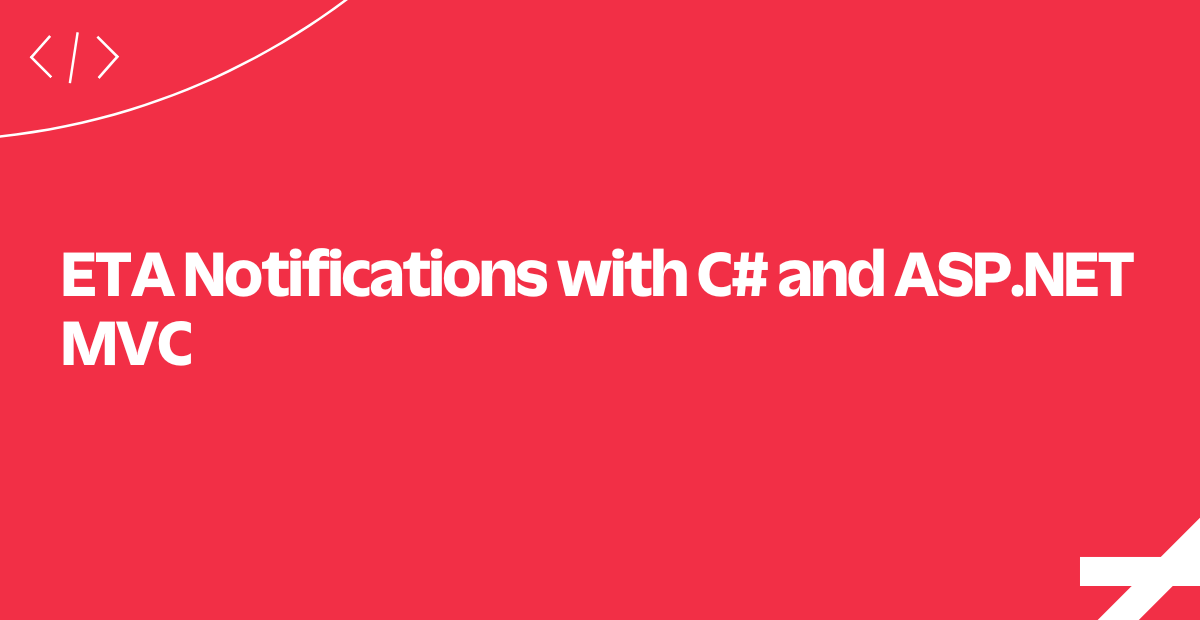
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly, wherever we are. The key to those services working? Notifying customers when things change.
In this tutorial, we'll build a notification system for a fake on-demand laundry service Laundr.io in C# and the ASP.NET MVC.
Let's get started!
Trigger a Notifications
There are two cases we'd like to handle:
- Delivery person picks up laundry to be delivered (
/Pickup
) - Delivery person is arriving at the customer's house (
/Deliver
)
In a production app we would probably trigger the second notification when the delivery person was physically near the customer, using GPS.
(In this case we'll just use a button!)
Next, let's look at how we set up the Twilio REST API Client to push out notifications.
Setting up the Twilio REST Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio account credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
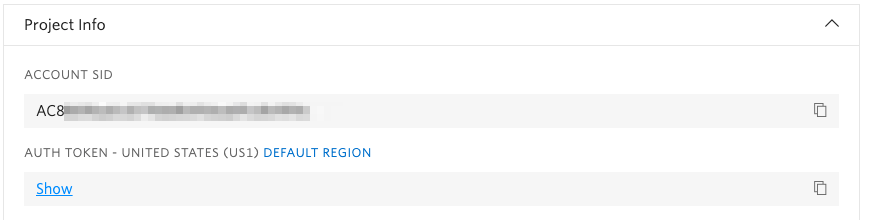
Twilio REST Client ready, routes in place? Let's now look at how we handle notification triggers.
Handle a Notification Trigger
This code handles a HTTP POST
request triggered by a delivery person in the field.
It uses our INotificationServices
instance to send an SMS message to the customer's phone number, which we have registered in our database. Simple!
Now let's look closer at how we send out the SMS.
Send an SMS (or MMS)
This code demonstrates how we actually send the SMS.
Something missing? An image of the clothes, perhaps?
You can add an optional mediaUrl
as well. Glad you pointed that out!
var mediaUrls = new List<Uri> () {
new Uri("http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg")
};
var to = new PhoneNumber(phoneNumber);
await MessageResource.CreateAsync(
to,
from: new PhoneNumber(_twilioNumber),
body: message,
statusCallback: new Uri(statusCallback),
client: _client,
mediaUrl: mediaUrls);
In addition to the required parameters, we can pass a `status_callback
` url. Twilio will then POST
there to let us know when the status of our outgoing message changes.
Let's look at the status callback in greater detail.
Handle a Twilio Status Callback
Twilio will make a POST
request to this resource each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notificationStatus
on the Order
and business logic can take over. This is a great place to add logic that would resend the message upon failure, or send out an automated survey a few minutes after a customer receives an order.
That's a wrap (fold?)! We've just implemented an on-demand notification service that alerts our customers when their order is picked up and arriving.
Now let's look at other easy to add features for your application.
Where to Next?
We love ASP.NET and C# here at Twilio. Here are a couple other tutorials we've written:
Increase your rate of response by automating the workflows that are key to your business. In this tutorial, learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand so two users can communicate without exchanging personal information.
Did this Help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.