Instant Lead Alerts with Java and Servlets
Time to read: 2 minutes
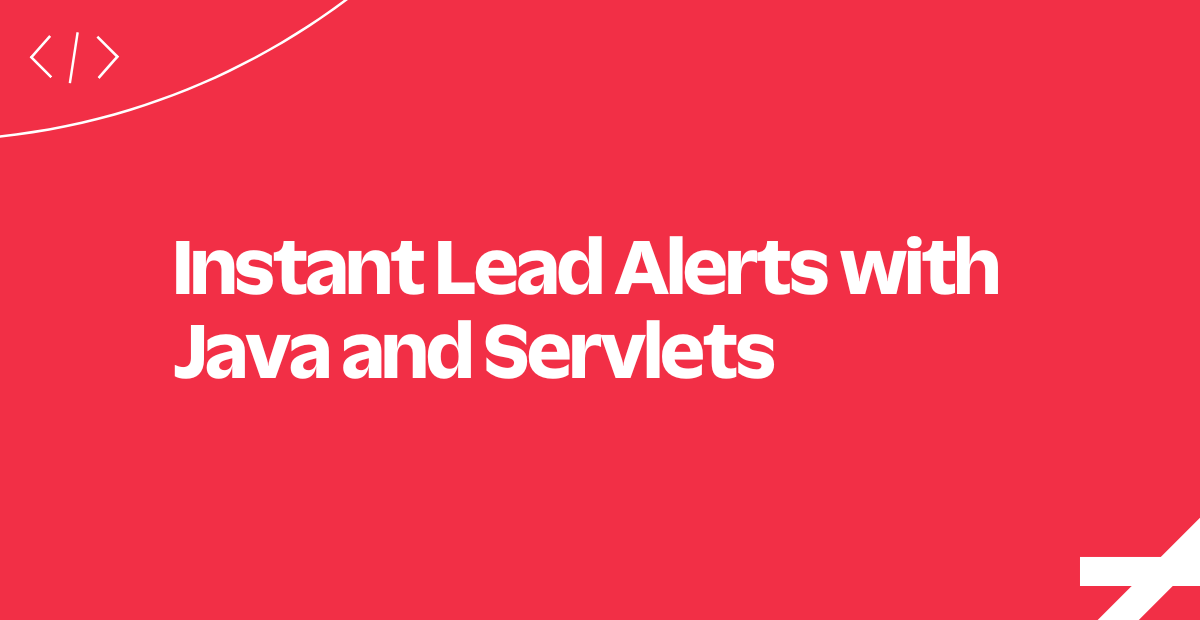
You probably already have landing pages or product detail views which you're using to generate some excellent leads for your business. Would you like to let the sales team know when you've got a new qualified lead?
In this tutorial, we'll use Twilio Programmable SMS with Java and Servlets to send a message when a new lead is found. In this example, we'll be implementing instant lead alerts for a fictional real estate agency.
We'll create a landing page for a new house on the market and a form for web surfers to request additional information. The form will trigger notifications to our agents in the field, who can follow up and hopefully close the deal.
Populate Landing Page Data
To display a landing page for the house, we need to have some information to show the user. For demonstration purposes, we've hard-coded the information we'd like to show.
Now we have a method to serve the house data.
Next up, let's see how to use this data to render the Landing Page.
Render the Landing Page
In our landing page we'll insert the data about the house. We'll also add a sidebar form for the user to enter in their contact information and request additional information.
Our landing page template is ready (and looks great).
Now let's see how to initialize the Twilio REST Client to send messages.
Create a Twilio REST API Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
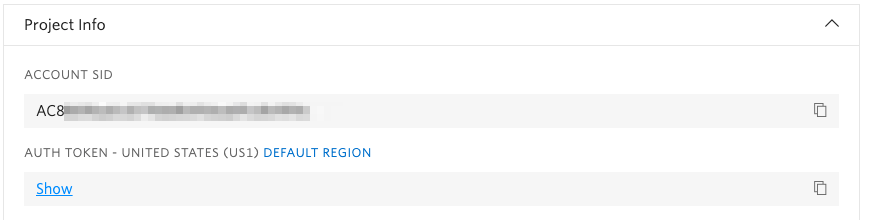
Our Twilio Client is now ready to send messages! Next up, let's see how to handle incoming leads.
Handle Incoming POST Requests
This code handles the HTTP POST
request from our landing page when a browser expresses interest through our form.
It uses our MessageSender
class to send an SMS message to the real estate agent's phone number, which is stored as an environment variable. We include the lead's name, phone number, and inquiry directly in the body of the text message we send to the agent.
Now the agent has all the information he or she needs to quickly follow up with the new lead.
That's a wrap! We've just implemented an application to instantly route leads to salespeople using text messages.
Next, we'll point out a few other useful features that are perfect for your application.
Where to Next?
Java and Twilio go oh so well together. We'll prove it; here are a couple of our favorite Java tutorials:
Click-to-call enables your company to convert web traffic into phone calls with the click of a button.
Easily route callers to the right people and information with an IVR (interactive voice response) system.
Did this help?
Thanks for checking out this tutorial... tweet @twilio to let us know what you're building!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.