SMS and MMS Notifications with Java and Spark
Time to read:
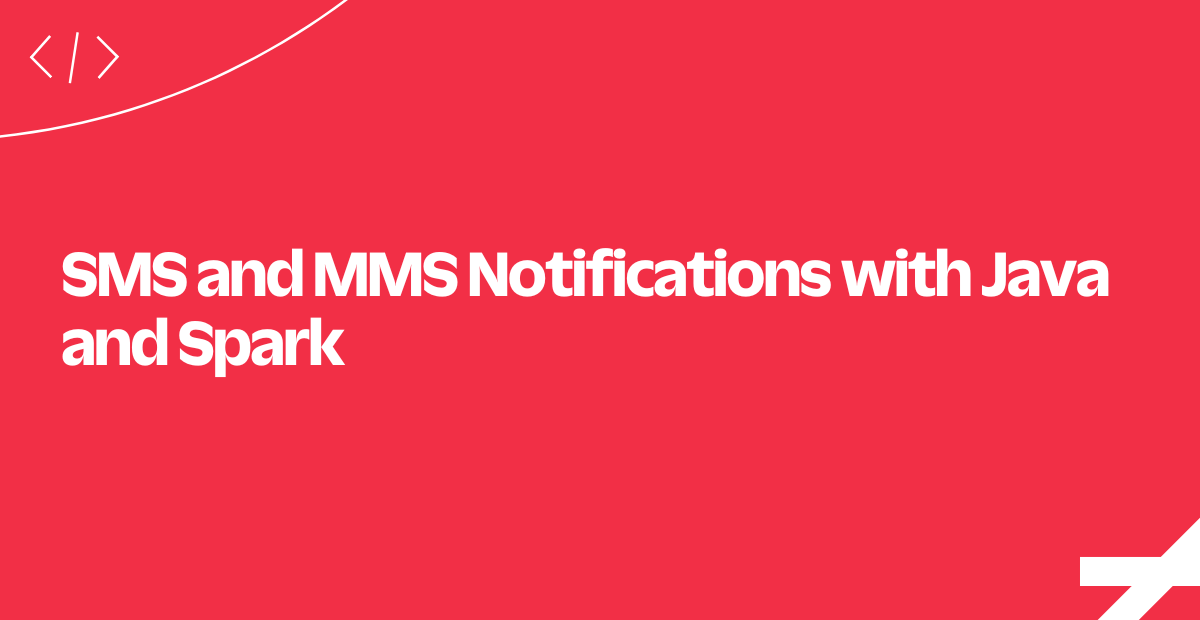
Today we'll look at a Spark sample application that demonstrates how to send SMS alerts to your system administrators when something goes wrong on your server. We'll do a deep dive on all of the plumbing so you can see how easy it'll be to add notifications to your own application.
Let's get started!
List Your Server Administrators, and Whomever Else
Here we create a JSON list of administrators who should be notified if a server error occurs.
The only essential piece of data we need is a phone_number
for each administrator.
Next, let's take a look at configuring the Twilio REST Client.
Configuring the Twilio REST Client
To send a message we'll need to initialize the TwilioRestClient
defined in the Twilio Java Helper Library. It requires reading a TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
from environment variables.
The values for your account SID and Auth Token will be in the Twilio console:
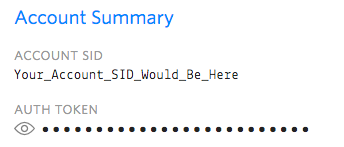
Click the eyeball to show your Auth Token to copy and paste.
Next up, we will look at how to handle application exceptions.
Handling the Application Exceptions
In a Spark application, we can handle exceptions of a configured type for all routes and filters by using exception mapping. This is also where we'll eventually loop through the list and send our alerts.
Now let's look at how to create a custom message for our notifications.
Create a Custom Alert Message
Here we create an alert message to send out via text message.
You might also decide to include a picture with your alert message and make it an MMS. Perhaps a screenshot of the application when the crash happened? An 'Everything is Okay' meme image?
Let's move forward and look at loading the list of administrators.
Reading the Administrators from a JSON File
Next, we read the admins - and any other lucky people - from our JSON file. We use the Gson Java library to convert our JSON text file into Administrator
objects.
And next, we will see how to send a text message.
Sending a Text Message
There are three parameters needed to send an SMS using the Twilio REST API: From
, To
, and Body
.
US and Canadian phone numbers can also send an image with the message. Other countries will have the image URL automatically shortened and appended to the body.
And that's a wrap!
We've just implemented an automated server notification system for Java and Spark that can push server alerts if anything goes wrong.
Let's see what else you can do with the Twilio Java SDK, next.
Where to Next?
We love Java here at Twilio. Here're just a couple more examples of using our Java SDK to easily add some features in your application:
How well is your team doing when interacting with customers? Instantly collect structured data from your users with a survey conducted over a voice call or SMS.
A simple tutorial to prevent no-shows at your business - send your customers automatic appointment notifications.
Did this help?
Thanks for building with us today. Tweet us a comment @twilio to let us know what you think and what you're building!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.