Automated Survey with Java and Spark
Time to read: 3 minutes
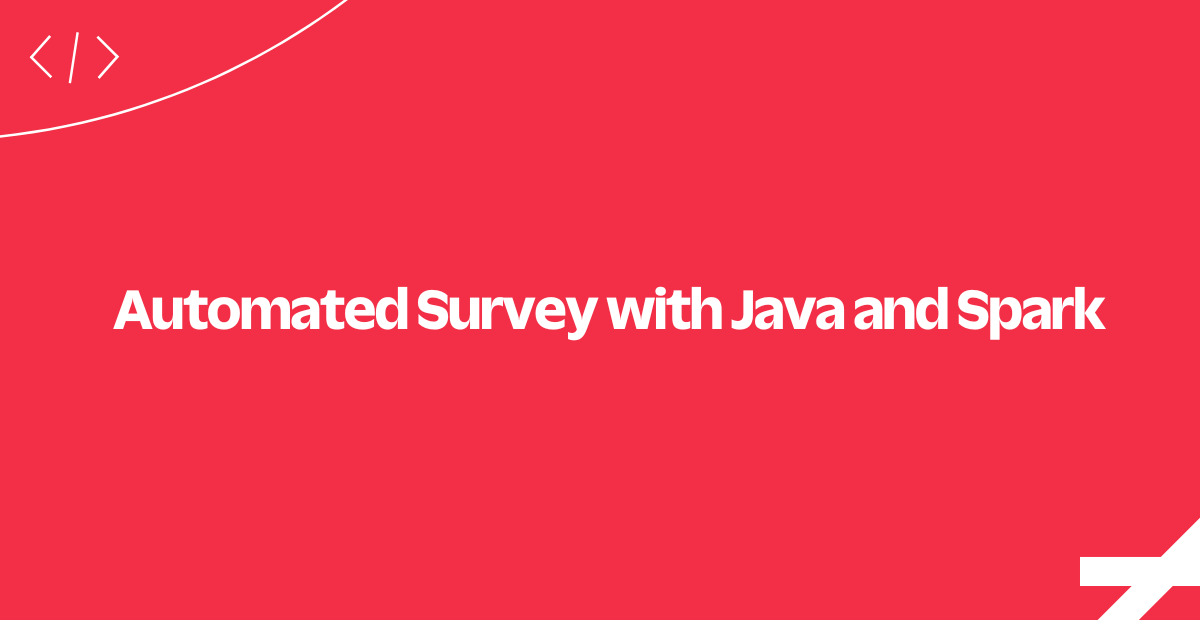
This Spark application uses the Twilio API to create an automated survey conducted over SMS or phone call. Callers will interact with this application over the phone, and you will be able to view the results on a dynamic dashboard.
Check out the source code for this application on GitHub.
Creating a Survey
Before we gather responses, let's specify the questions we would like to ask.
Each question is created as a JSON object with two attributes: text
, which holds the question we'd like to ask, and type
, which describes the kind of input we expect a caller to provide when asked that question.
Our app reads this JSON into a Java object using Google's Gson library.
In this tutorial, we'll highlight the code that interacts with Twilio and in turn makes the application tick. Check out the project README on GitHub to see how to run the code yourself.
Our users will need to contact us in order to take our survey. Let's see how to accept a call or SMS.
Accepting a Call
Every time your Twilio number receives a call or an SMS, Twilio will send an HTTP request to the application asking what to do next. The application responds with TwiML that describes the action (<Say>
a phrase, <Gather>
input, <Message>
an SMS, among others).
Your Twilio number must be configured to make requests to the /interview
route of this application. Go to your Manage Numbers page, and set up a new TwiML application using one of your Twilio numbers.
If you don't already have a server configured to use as your webhook, ngrok is a great tool for testing webhooks locally.
Now that we can receive a call, let's look at how to ask our user a question.
Asking a Question
Once the application receives an HTTP request from Twilio, it uses a different factory based on whether an SMS or phone call generated the request. This factory helps us build the appropriate TwiML response.
For SMS requests, the application will use the <Message>
verb to answer all requests.
For requests generated by phone calls, the TwiML will use the <Say>
verb for the question's text, and <Gather>
or <Record>
to collect input based on expected user input.
TwiML verbs have properties that allow you to change the way the verb works. For example, the <Record>
verb always collects audio input, but using the transcribeCallback
property, you can specify a route to which Twilio will send a transcript of the audio input. For more on TwiML verbs and their properties, check out the TwiML API documentation.
Let's see how to work with the transcription of a recorded response.
Using a Transcript of a Recorded Response
When Twilio finishes transcribing a free-form voice response, the application finds the corresponding survey and updates the response for that question to include the transcription. This makes it easier to skim the responses without listening to the full call.
Let's take a look at how to store the caller's response.
Storing the Caller's Response
After each TwiML verb is executed and input is collected, Twilio sends that input to the application along with a request for more TwiML. The/interview
route in our controller handles this input and passes it to the model to update the survey. Using MongoDB's Morphia document-object mapper, we can update the question index, responses, and completeness status of the survey.
Finally, let's take a look at how to display our survey results.
Displaying Survey Results
In order to view our results, the completed surveys are serialized to JSON which then populates the dashboard. The dashboard is served at the root (/
) URL of the application. We use jQuery to call this route via Ajax and display the survey results in charts on the home page.
Pat yourself on the back! If you have configured one of your Twilio numbers to the application built in this tutorial, you should be able to take the survey and see the results under the root route of the application. We hope you found this sample application useful.
Where to Next?
If you're hungry for more ways to work with Twilio APIs in Java, check out one of our other tutorials, like:
Automate the process of reaching out to your customers in advance of an upcoming appointment.
Did this help?
Thanks for checking out this tutorial! If you have any feedback to share with us, we'd love to hear it. Connect with us on Twitter and let us know what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.