Automated Survey with PHP and Laravel
Time to read: 4 minutes
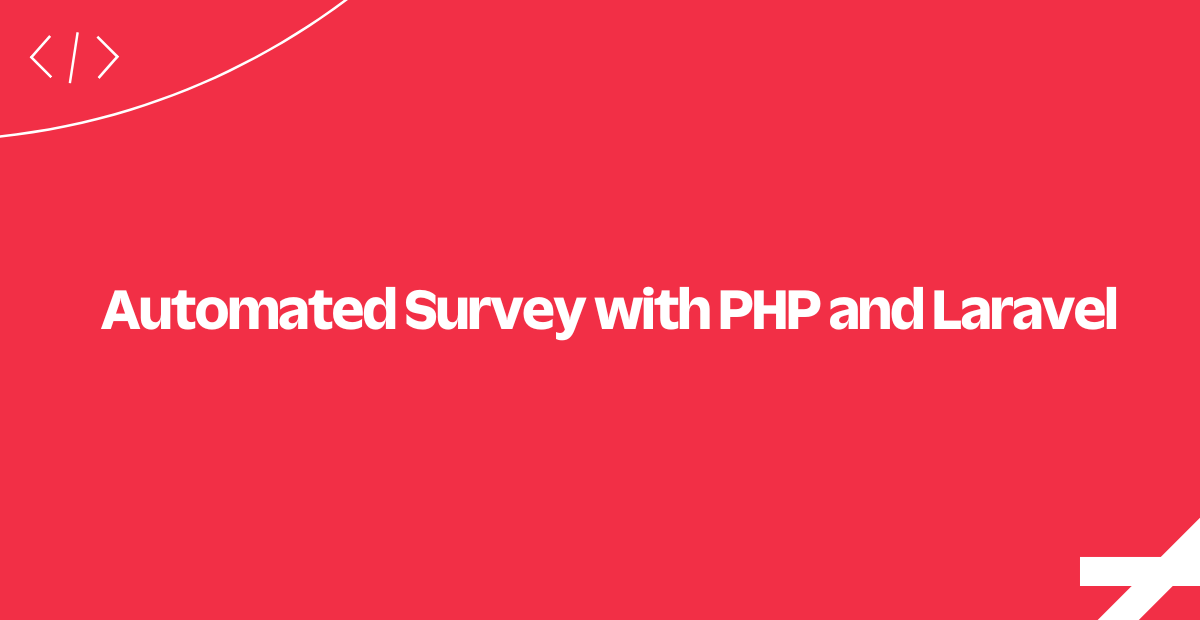
Have you ever wondered how to create an automated survey that can be answered over phone or SMS?
This tutorial will show how to do it using the Twilio PHP SDK.
Here's how it works at a high level
- The end user calls or sends an SMS to the survey's phone number.
- Twilio gets the call or text and makes an HTTP request to your application asking for instructions on how to respond.
- Your web application instructs Twilio (using TwiML) to
Gather
orRecord
the user's voice input, and prompts text input withMessage
if you are using SMS. - After each question, Twilio makes another request to your server with the user's input, which your application stores in its database.
- After storing the answer, our server will instruct Twilio to
Redirect
the user to the next question or finish the survey.
Creating a Survey
In order to perform an automated survey we first need to have some questions we want to ask a user. For your convenience, this sample application's repository already includes one survey that can be loaded into your database. You can take a look at the Readme File for detailed instructions.
You can modify the questions from the survey by editing the bear_survey.json file located in the root of the repository and re-running the app.
Before we dive in, let's take a moment to understand the flow of a Twilio-powered survey as an interview loop.
The Interview Loop
The user can answer a question for your survey over the phone using either their phone's keypad or by voice. After each interaction Twilio will make an HTTP request to your web application with either the string of keys the user pressed or a URL to a recording of their voice input.
For SMS surveys the user will answer questions by replying with another SMS to the Twilio number that sent the question.
It's up to the application to process, store and respond to the user's input.
Let's dive into this flow to see how it actually works.
Configuring a Twilio Number
To initiate the interview process, we need to configure one of our Twilio numbers to send our web application an HTTP request when we get an incoming call or text.
Click on one of your numbers and configure Voice and Message URLs that point to your server. In our code, the routes are /voice/connect
and /sms/connect
, respectively.
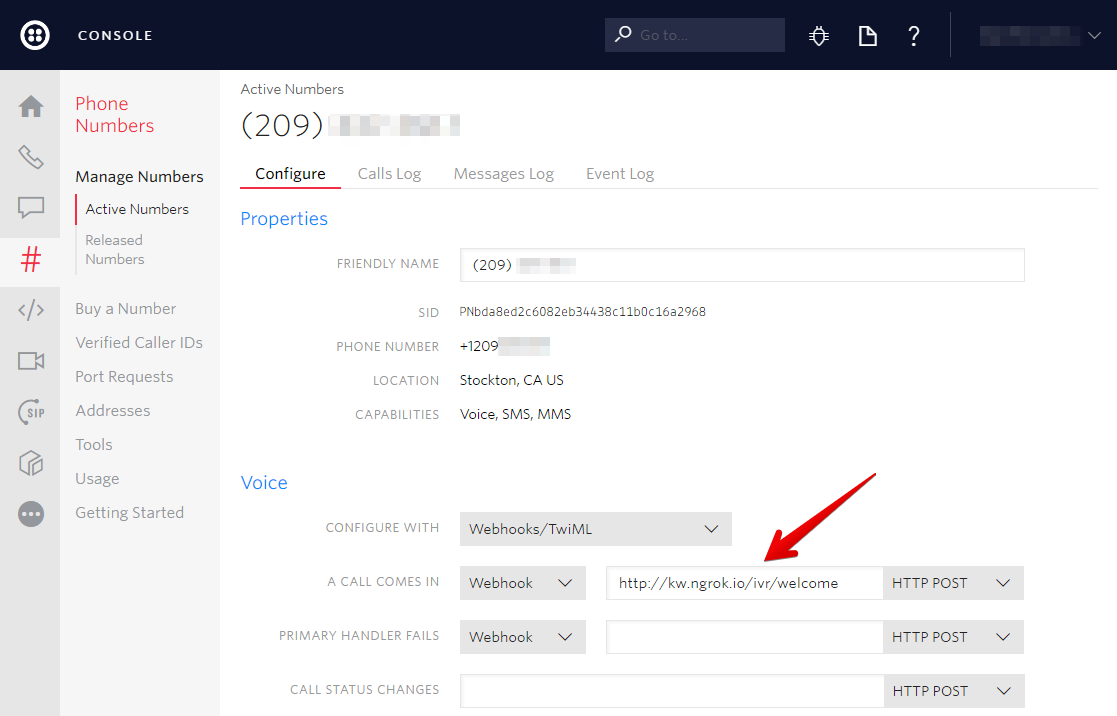
If you don't already have a server configured to use as your webhook, ngrok is a great tool for testing webhooks locally.
Next, we'll see how to handle requests to our webhooks.
Responding to a Twilio Request
Right after receiving a call or SMS, Twilio will send a request to the URL specified in that phone number's configuration (/voice/connect
for calls and /sms/connect
for sms).
Each of these endpoints will receive the request and return a welcome message to the user. When a user calls the Twilio number, our application will use the Say
verb. For users that respond via SMS, the application will use a Message
. It also includes a Redirect
to the question's endpoint in order to continue the survey flow.
Next, we will see how to build a TwiML response that will play back questions and gather input from the user.
Asking Questions (Voice)
After hearing the welcome message, the user will be redirected to the first question.
Each type of question will produce different instructions on how to proceed. If the question is "numeric" or "yes-no" in nature then we use the <Gather>
verb. However, if we expect the user to record an answer we use the <Record>
verb. Both verbs take an action
attribute and a method
attribute.
Twilio uses both attributes to define the response endpoint that will be used as callback. This endpoint will be responsible for receiving and storing the caller's answer.
During the Record verb creation, we also ask for a Transcription. Twilio will process the voice recording and extract all useful text. When the transcription is done it will make a request to our response's endpoint.
Next, we will learn how to ask questions via SMS.
Asking Questions (SMS)
To ask questions over SMS we just need to use the <Message>
TwiML verb. When users start a new survey, they will receive a welcome message as well as a message with the first survey question.
When the user is interacting over SMS we don't have something like an ongoing call session with a well defined state. It becomes harder to know if an SMS is answering question 2 or 20, since all requests will be sent to our main SMS endpoint at /sms/connect
. To solve that, we can use Twilio Cookies to keep track of what question is being answered at the moment.
Up next, we will see how to store the state of the survey.
Storing Answers
After the user has finished inputting voice commands or pressing keys, Twilio sends us a request that tells us what happened and asks for further instructions.
At this point, we need to recover data from Twilio's request parameters and store them.
Recovered parameters vary according to the questions:
Body
contains the text message from an answer sent with SMS.Digits
contains the keys pressed for a numeric question.RecodingUrl
contains the URL for listening to a recorded message.TranscriptionText
contains the result of a recording transcription.
Finally we redirect to our question controller, which will ask the next question in the loop. This is done in the _redirectToQuestion()
method.
Now, let's see how to visualize the results.
Displaying the Survey Results
You can access the survey results from the application's root route.
The results will be shown grouped by survey. To hear recorded answers, you can click on a playback icon to listen to the user's response. Any transcribed voice answers will be shown when available.
That's it!
If you have configured one of your Twilio numbers for the application built in this tutorial you should be able to take the survey and see the results under the root route of the application. We hope you found this sample application useful.
Where to next?
If you're a PHP developer working with Twilio, you might enjoy these other tutorials:
Put a button on your web page that connects visitors to live support or salespeople via telephone.
Improve the security of Laravel's built-in login functionality by adding two-factor authentication via text message.
Did this help?
Thanks for checking this tutorial out! If you have any feedback to share with us, we'd love to hear it. Reach out to us on Twitter and let us know what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.