Export MySQL Data to CSV in CakePHP
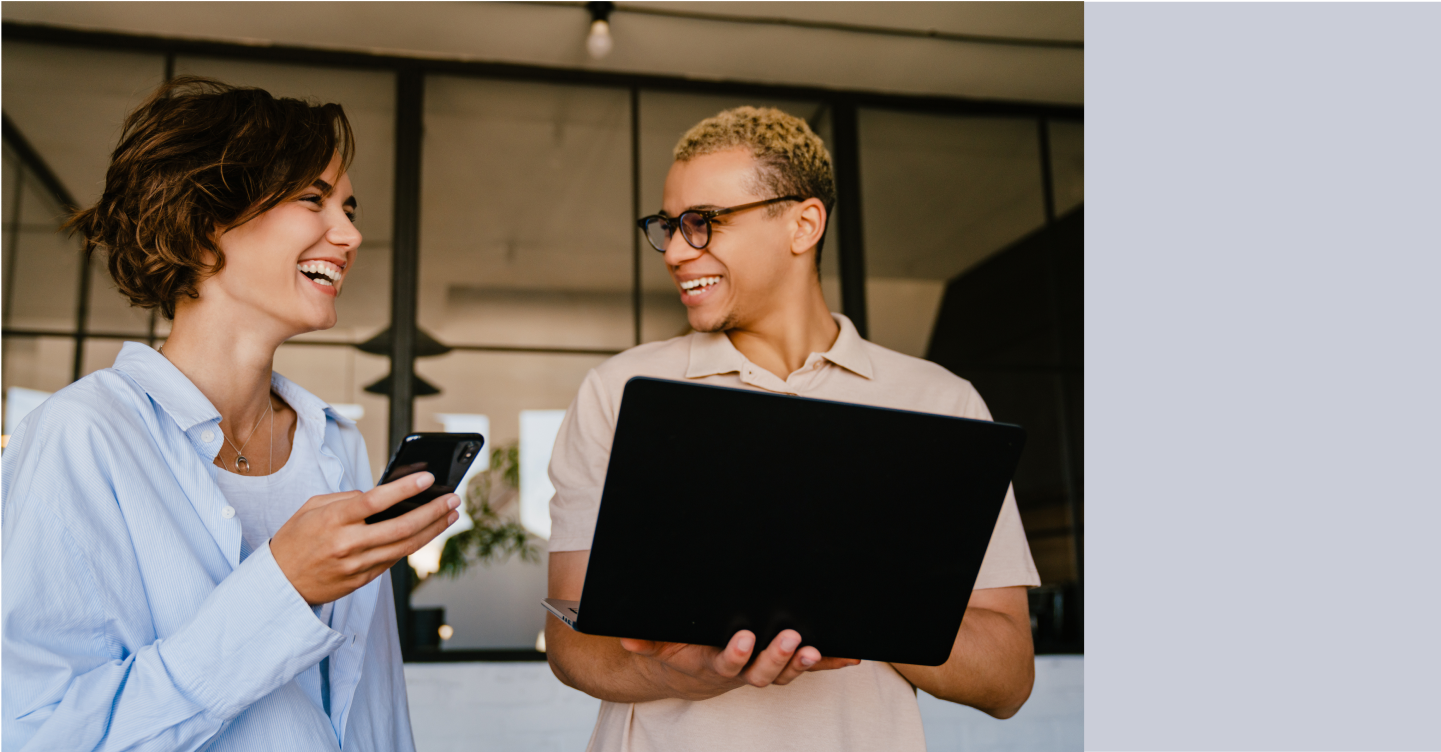
Time to read: 3 minutes
Data is king, so managing it efficiently is essential! One common requirement of working with data is exporting data from a database to a CSV (Comma-Separated Values) file, a universal format for sharing structured information.
In this tutorial, you will learn how to export data from a MySQL database to a CSV file with the CakePHP framework.
Prerequisites
Before we dive into the tutorial, make sure you have the following:
- Basic knowledge of PHP and web development concepts
- PHP 8.2 installed with the PDO MySQL extension
- Access to a MySQL server
- Composer installed globally
Create a CakePHP Project
To do this, navigate to the folder where you want to install the project and run this command:
When asked, "Set Folder Permissions ? (Default to Y) [Y,n]?", answer with Y
.
This will install the latest version of CakePHP in a new directory named cakephp_csv.
Create the database
To begin, we need a database with a table to store the information which will be exported to a CSV file. To keep things simple, the database will store details about a list of workers, including their name, email address, and mobile phone number.
Create a database. I'll be naming mine fiie_test. The next thing is to, create a new table in your database called workers using the migrations feature in CakePHP. The table needs to contain the following fields:
- id: This field will serve as the unique identifier for each record. It should have a type of integer and be the table's primary index, with the auto-increment attribute attached to it.
- name: This field will store the worker's name. It should have a data type of varchar
- email: This field will store the worker's email address and have a datatype of varchar
- mobile: this field will store the worker's mobile phone number, and also have a type of varchar
To do this, open up the terminal and run this command:
This will create a migrations file in config/Migrations/. Navigate to the just created migrations file and replace the change()
function with this:
Next, run this command to create the table schema:
This will not only create a workers table but also insert the data into the database. if you look at the contents of the workers
table, it should contain data matching those in the screenshot below.
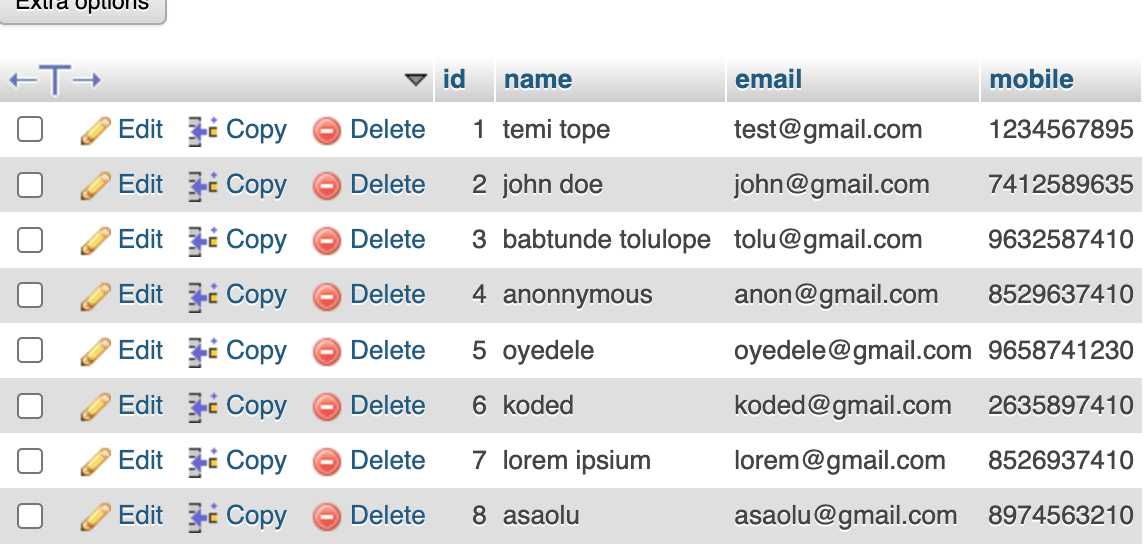
Connect to the database
To connect the database to the application, open the project folder in your preferred code editor or IDE and open config\app_local.php. In the default
section, inside the Datasource
section, update the default configuration by changing the host, username, password, and database properties to match the credentials of your database. For example:
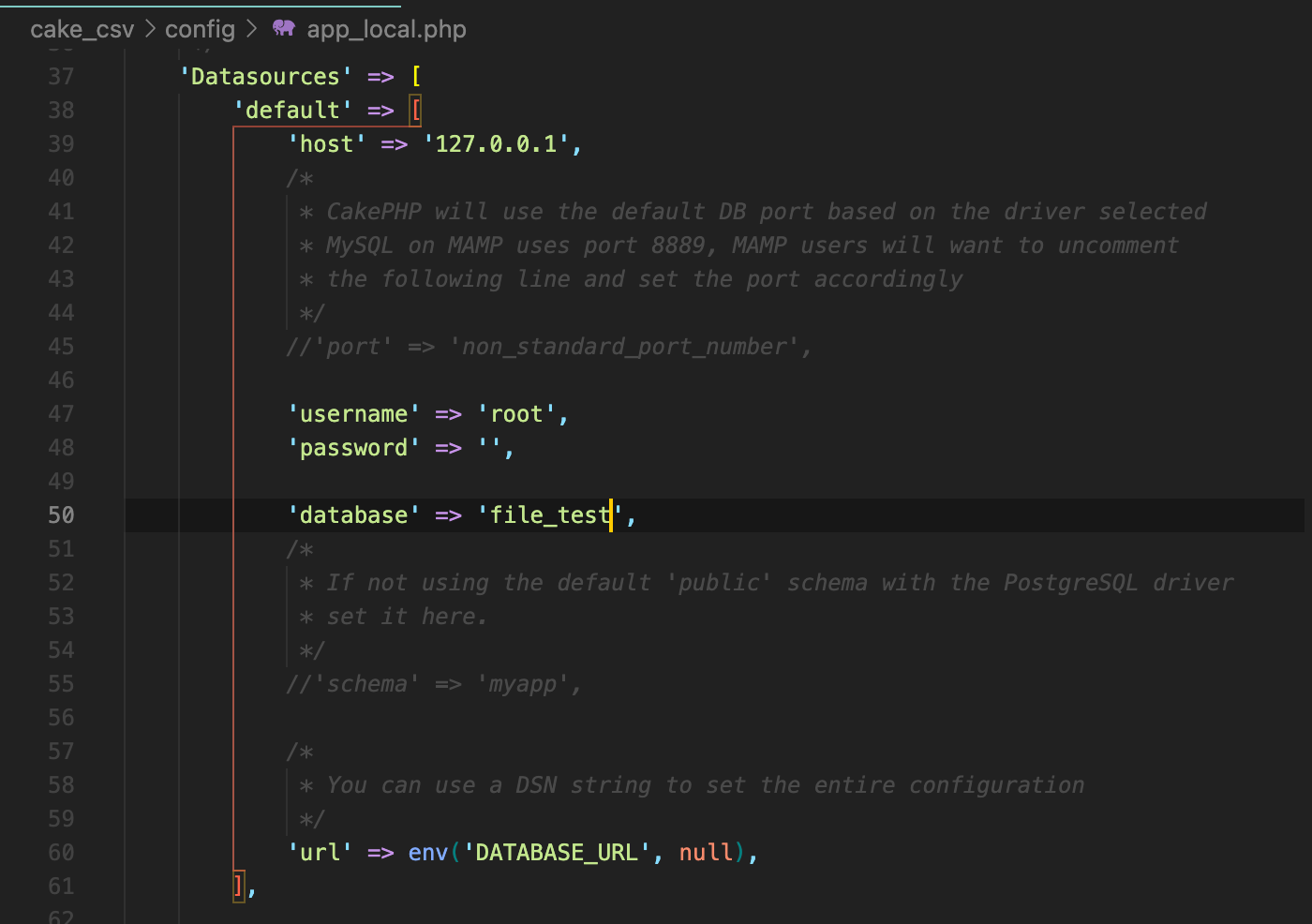
From the image above, the host was changed to 127.0.0.1
, the username to root
, the password was left blank, and the database was set to the one created earlier.
Now, start the development server in your terminal, by running this command:
If you open http://localhost:8765, it should look similar to the screenshot below.
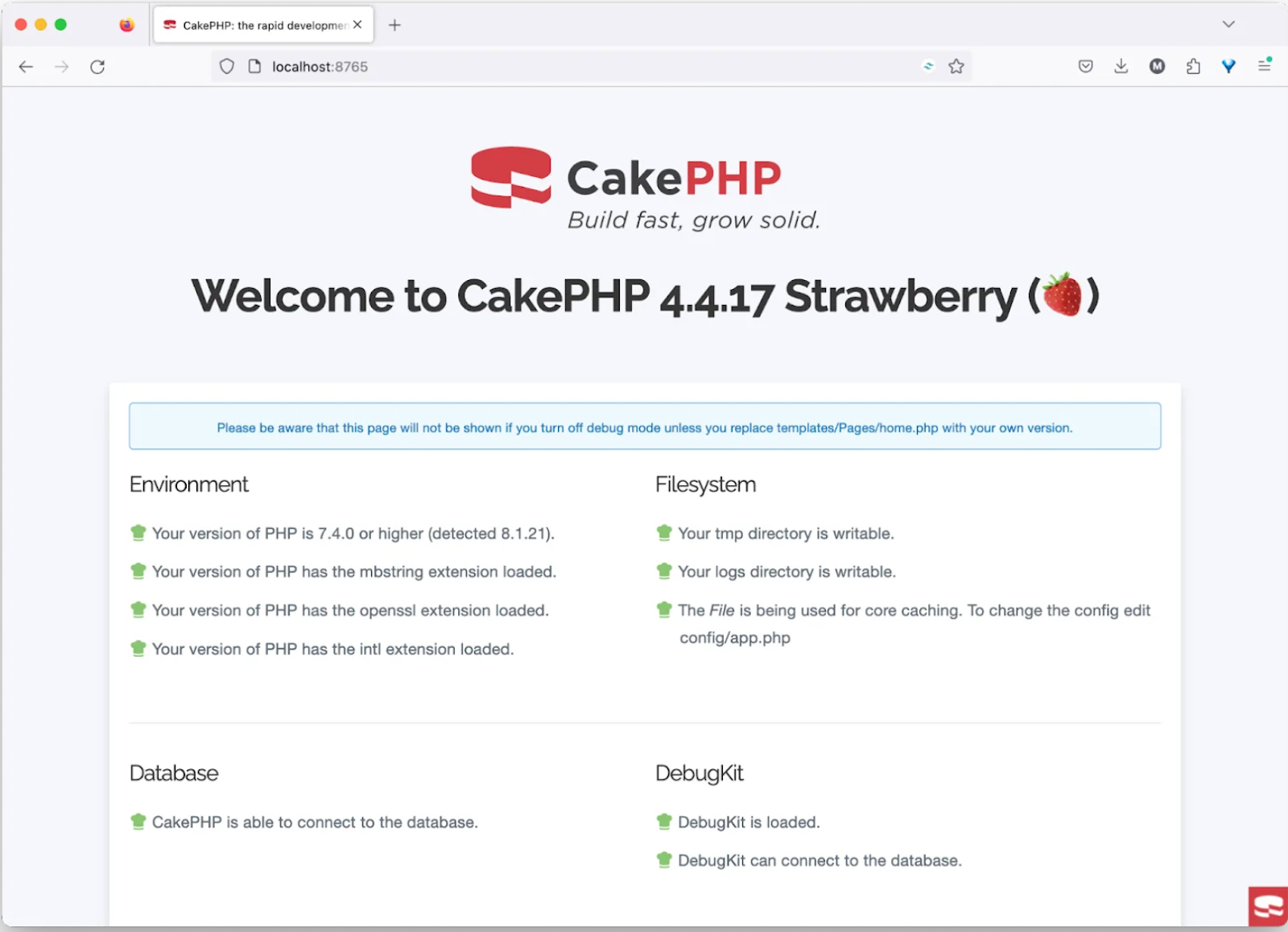
Create a model and an entity
To create a model and entity, open up a new terminal and run this command:
Running this command will create the model file WorkersTable.php inside the src/Model/Table folder. Also, we should see the entity file Workers.php inside the src/Model/Entity folder.
Create a controller
To create a controller, open up the terminal once again and run this command:
Running this command will create a file called DetailsController.php file inside the src/Controller folder. Open this file and paste the following into it.
The code above generates a CSV report of worker data from a database and prompts the browser to download the file. To do this, it initializes the controller, loads a model, and sets HTTP headers for CSV content. It then retrieves worker data from the workers table and writes it to the output stream of the PHP process (php://output); which becomes the body of the response.
Add a Route
Adding a route will be the final step. Navigate to config/routes.php and inside the call to $routes->scope()
, paste the following:
Test the application
Now, let’s check that the application works as expected by opening to http://localhost:8765/download-csv in your preferred browser.
You'll notice that a CSV file with the name workers-{datetime}.csv will be downloaded. Its contents should match those in the screenshot below.
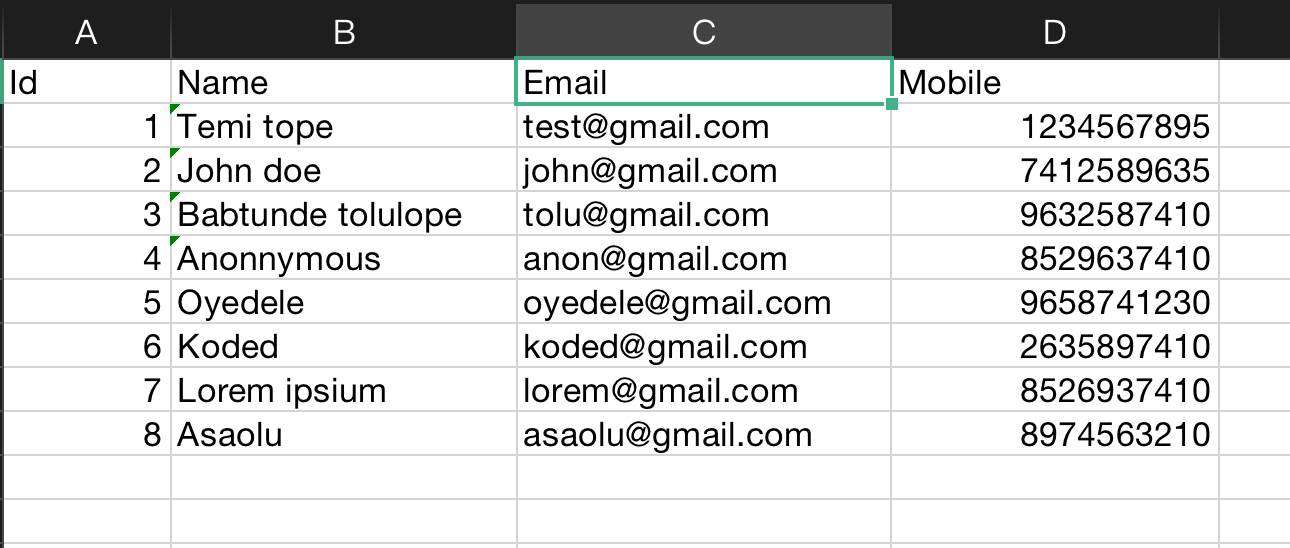
Conclusion
In this article, we learned how to export data from a database to CSV in CakePHP. By following the steps outlined in this tutorial, you're now equipped with the essential knowledge to perform this task using CakePHP. Happy coding!
Temitope Taiwo Oyedele is a software engineer and technical writer. He likes to write about things he’s learned and experienced.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.