How to Read Top Live News Headlines Using the News API and Twilio SMS
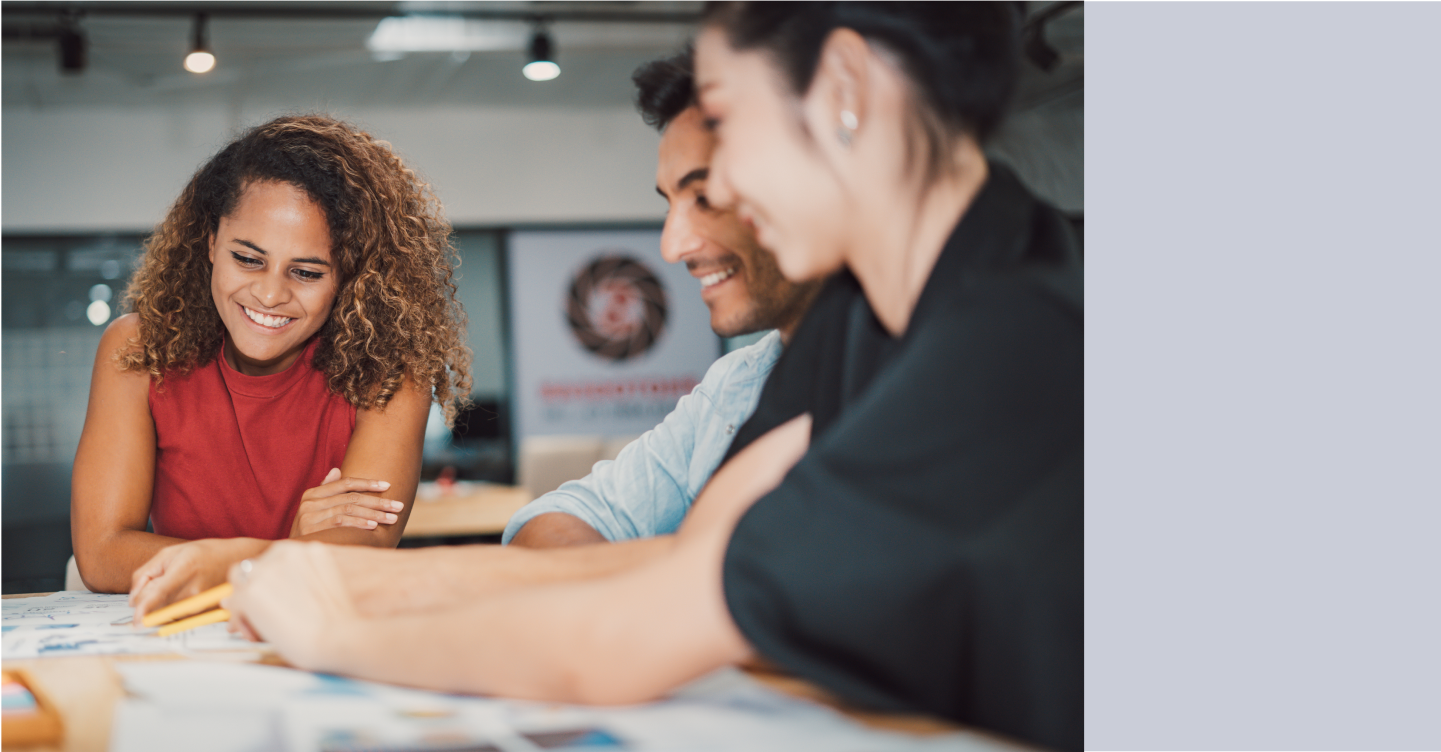
Time to read: 5 minutes
To be successful in anything you do, you should know that knowledge is power. However, you cannot have knowledge without information. Have you ever missed an opportunity that everyone was informed about?
Information has great power because it enables you to arrange your actions according to the effects that may occur. For instance, it is regarded as good practice for day traders to look up market information to make sure their deals are unaffected.
One of the primary information sources that one can use to stay current on world events is the news. The BBC News, CNN, Bloomberg, Financial Times, and others are some of the most popular news sources.
How do you make sure you stay current on news without scrolling the entire internet given all these news sources? Learn how to use News API and Twilio SMS API to obtain the most recent live news headlines from the US.
Prerequisites
- Knowledge of Java programming language.
- Java development kit (JDK) 8 and above.
- Twilio account - Twilio offers trial credits for the APIs. You can receive a Twilio phone number by creating a Twilio account, which you will use to send an SMS.
- News API account - Developers can test the APIs for free with a News API account. Create a News API account, and make a note of the API key you will need to submit news queries later.
- IntelliJ IDEA community edition - Development environment for the Java programming language.
Project setup
Open Intellij and select File > New > Project to start a new project. Enter BBCNewsSMS as the project name, choose Java as the language, and Maven as the Build system in the window that appears.
Enter org.twilio as the GroupId and bbcNewsTwilioSMS as the ArtifactId in the Advanced Settings. Your project should look like the example below:
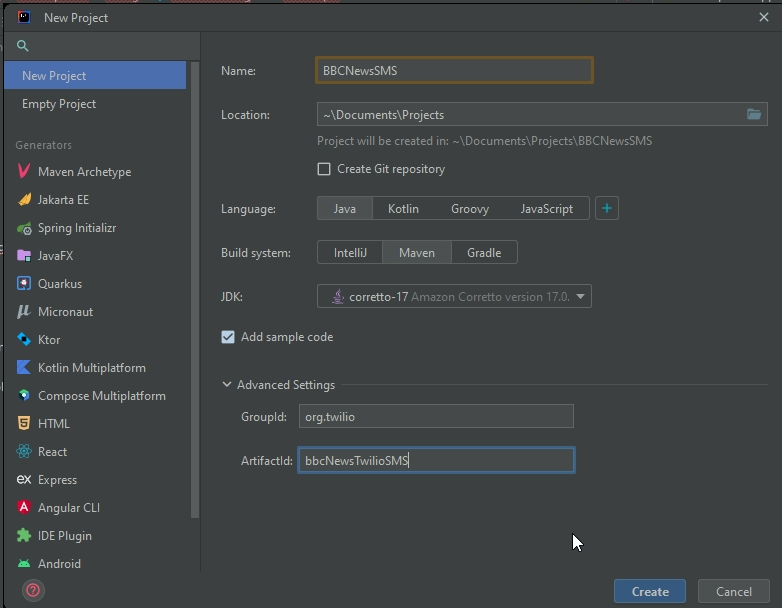
To start a fresh Maven project, click the Create button.
Add dependencies
The following dependencies should be copied and pasted into the pom.xml file after the properties
tag.
- Twilio - This dependency is necessary to send an SMS conveying the news is made possible by this dependency.
- HttpClient - To obtain a selection of the most recent top news headlines in the US, use the HttpClient to send HTTP queries to the News API.
- JSON - The JSON requirement will enable you to extract the crucial news details from the JSON response supplied by the HTTP client.
Create a class to format the date
Create a file named BBCDateFormat.java under the package src/main/java/org/twilio. Copy and paste the following code into the file.
The timestamp from the News API response that was parsed by JSON is accepted as a string by the method formatTimestamp()
.
The SimpleDateFormat
declaration is returned by the News API and formatted accordingly for the SMS.
To parse the date, call the parse()
method and pass the string representation of the timestamp as the argument of the method. Call the format()
method and pass the Date
object returned by the parse()
method as the argument of the format()
method.
Create a class to fetch and send the latest news
Create a file named BBCNewsSMS.java under the package src/main/java/org/twilio. Copy and paste the following code into the file.
You must first specify the Twilio phone number, your personal phone number, the news API key, and the news API URL in order to successfully send an SMS and retrieve news from the News API.
The variables TWILIO_PHONE_NUMBER
, RECIPIENTS_PHONE_NUMBER
, NEWS_API_KEY
, and NEWS_API_URL
, respectively, declare these attributes.
The mechanism to fetch and deliver a Twilio SMS is defined by the method fetchAndSendLatestNews()
. You must provide the News API key as part of the URL for the news API to function properly.
You should add the query parameter?country=us
to retrieve news from the US. You should add the query parameter?source=bbc-news
if you want to retrieve news from a specific source, like the BBC. For additional information on the News API, visit its user guide.
The string apiUrl
specifies the News API URL for retrieving US top news stories. Call the createDefault()
function of HttpClients
, then call the execute()
method on the same method, to send a request to this URL.
Since the request is a GET request, the execute()
method should take a new instance of HttpGet
as an argument, and then it should take apiUrl
as an argument.
If the call was successful, a JSON response with an array of articles should have been sent. To obtain the necessary quantity of news headlines, you should parse the array of articles from the JSON response. Only the first top news headline from the US is parsed in this example. Later, you can edit the code to add more.
Create a new instance of JSONObject
, supply the JSON response as the input, and the response will be converted into a JSON object. To obtain the array of articles, use the JSON object's getJSONArray()
function with the string "articles" as a parameter.
Use the JSON array object's getJSONObject()
function with parameter 0
to retrieve the first News article from the array.
After receiving the first article, construct a message using the article's characteristics. The title, summary, URL, and publication date of the news piece will all be included in the message.
Call the JSON object's getString()
function with the string argument title to retrieve the title. Follow the same procedure for the description, URL, and publication date. Call the formatTimestamp()
function you defined in the section before producing the message and supply the publication date to format the date.
The message to be sent to your phone number is defined by the string variable message, and it must have the following information: a title, a description, a formatted timestamp, and a URL. Call the create()
method of the Message class and supply the inputs RECIPIENT_PHONE_NUMBER
, TWILIO_PHONE_NUMBER
, and a message to send the message. Once the program has been launched, it will send the message to your Twilio SMS account.
Create the main class
Create a file named Main.java under the package src/main/java/org/twilio. Copy and paste the following code into the file.
You must define the account source identification and the authentication token in order to send a Twilio SMS properly. These attributes are set using the variables AUTH_TOKEN
and ACCOUNT_SID
.
Call Twilio's init()
method and supply the account to use and the account's authentication token as parameters to set up the Twilio environment.
Finally, use the fetchAndSendLatestNews()
function to retrieve and send the most recent US top headline news. You don't need to make a new object because the method is static.
Run the application
Press the run icon in the upper right corner of IntelliJ to launch the program. The location of the run icon is depicted in the following picture.

You should receive an SMS with the most recent top news headline from the US, as seen below, if your program functions smoothly and without any mistakes.
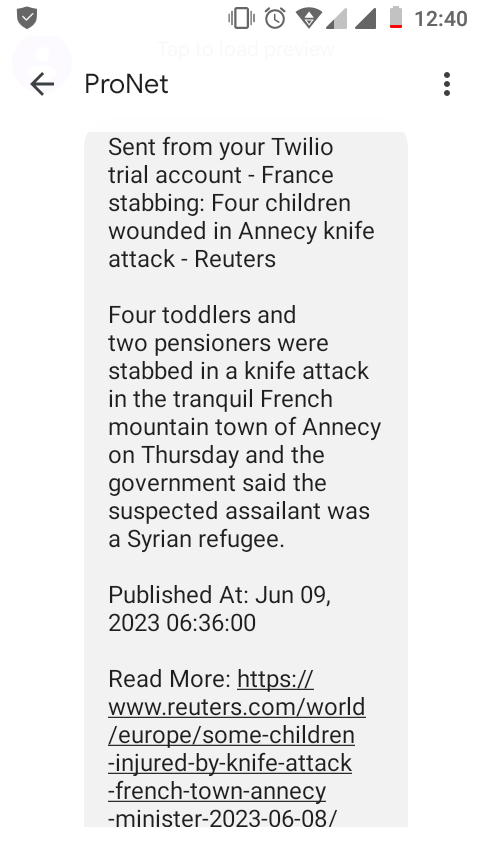
What's next for using the Twilio SMS API with the News API?
You have learned how to use News API and Twilio SMS to obtain the most recent live news headlines from the US in this tutorial. As previously said, you should modify this program to return news based on your location.
You can change this application to receive news from other outlets, like the BBC, CNN, Al Jazeera, and others. You can always make sure that you are up to date with the most recent information by using this program.
This program can be modified to receive news from additional sources, including the BBC, CNN, Al Jazeera, and others. Using this tool, you may always be certain that you are informed of the most recent developments.
You can change the application to construct a scheduler that runs the application after a set time and sends you the most recent top news headlines in your area if you want to receive the news on a regular basis. Finally, look through the Twilio documentation to discover more real-time news services you may employ, such as SendGrid and Twilio WhatsApp.
David is a computer science major who specializes in back-end development. He enjoys learning new things, meeting new friends, and using technology to solve issues. Currently working as a technical writer, David enjoys simplifying complex ideas for other developers to comprehend. His work has appeared on numerous websites. His blog can be found at javawhizz.com. You are welcome to follow him on GitHub and LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.