Send Driving Directions Using Bing Maps and Twilio MMS
Time to read:
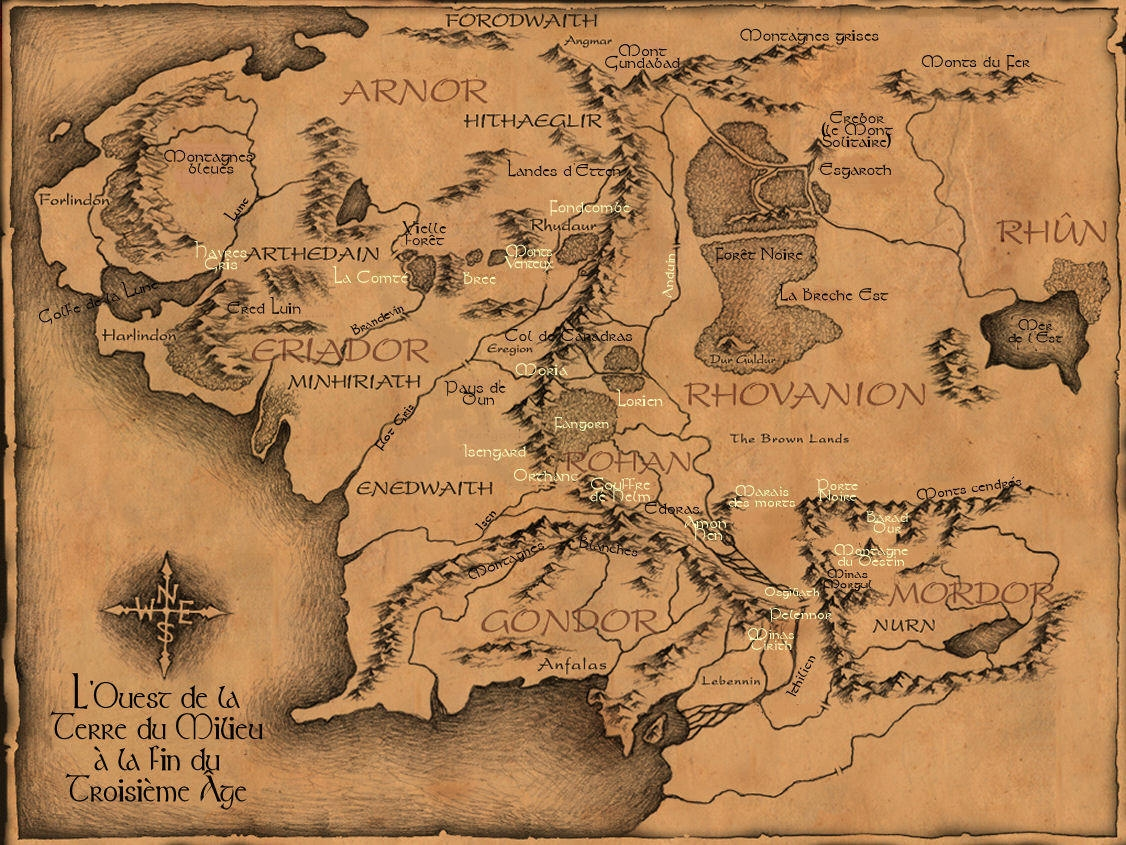
Recently my parents bought themselves a shiny new laptop. They got it home, sat it on their desk and realized that the only display port on the laptop was HDMI. This unfortunately would not work with their external monitor which only had VGA and DVI ports. As the resident family IT guy they called me.
I could have ordered the adapter, but I figured going local would be a faster way to solve their problem. I searched for the part on Best Buy’s website and found a generic HDMI to DVI cable which would work perfectly for them.
Now I’ve been burned in the past by seeing something online and rushing out to the local store only to find out its not actually in stock, so this time I picked up the phone and called my local Best Buy and had a conversation that went a bit like this:
BB: Hello, Best Buy, how can I help you
Me: Ya, I’m looking to see if you have a whatsihoozits in stock. The part number is 123456789.
BB: One moment sir, let me check. It doesn’t look like we have that in stock, but I am showing the Flooverville store has 4.
Me: Flooverville, eh? I’m not quite sure where that is.
BB: Oh, that store is located just outside of the Flooverville Mall.
Me: I’ve never been there. Can you give me an idea of how to get there?
BB: Sure. Hop on Route 345 for a few miles. Left at a gas station, I think it might be a Gas-O-Rama, but maybe not. Go for a few miles and look for the next big road. Not sure what its called, but it eventually becomes Flooverville Road. I think you turn right. You’ll see a McRonalds. When you do start……..[lots more vague directions go here]….and then you’ll see the sign for Best Buy.
Needless to say, the instructions were less than helpful. Here is how I wish that conversation had gone:
BB: Oh, well that store is located just outside of the Flooverville Mall.
Me: I’ve never been there. Can you give me an idea of how to get there?
BB: Sure. If you give me your address and phone number I’ll be happy to send you a map with driving directions directly to your phone.
Me: Awesome! I live at 123 Main Street Cityton and my phone number is 555-555-5555.
BB: The map should arrive at your phone momentarily. Is there anything else I can do for you today?
This post will show you how to build that experience. By combining the Best Buy API, Bing Maps and a bit of Twilio with some basic web development using JavaScript and ASP.NET MVC, we’ll create a website that can locate a product at a Best Buy near you and then send a customized driving route map directly to your phone as an MMS message. Let’s get building!
Gathering Your Materials
Like any building project, we’ll need some materials to get started. In this case we’ll leverage a number of different APIs including:
- Best Buy API – Gives us product availability and store location information. Sign up for free access to the Best Buy API
- Bing Maps REST API – Lets us generate a static map image with a driving route overlayed. Access to the Bing Maps REST API is also free
- Twilio API – Using a US or Canadian MMS enabled phone number, lets us send the generated map image to a phone via an MMS message. Sign up for your free Twilio account
Of course if you’re not interested in writing the code yourself and just want to deploy a completed app, you can do that as well. All of the code shown in this post is available as a completed website in GitHub.
Where Can I Find A Whatsihoozits?
The first problem we need to tackle is locating products at a local Best Buy. To do that we’ll build a simple web page that a store employee could use to check for product availability in a location. The page uses Bootstrap to display a nicely designed HTML form that lets the employee enter a product SKU, a zipcode and a search distance:
When the Search button is clicked, the web page uses jQuery to send the form values as an AJAX request to the Best Buy API. The API endpoint we are using will take those values and locate any Best Buy stores within the specified distance that has the product SKU in stock, returning to us a list of those store locations formatted as JSON.
Because this request comes directly from the web page, jQuery needs to be configured to make the request using JSONP, which the Best Buy API supports. To do this set the dataType parameter to jsonp
and the jsonpCallback parameter to a function named listProducts which you can add to your page.
Once the Best Buy API is successfully returning results to the web app, we’ll need to display them. To do this I chose to use Mustache templates, and created a simple template to show the address of each store returned from the Best Buy API:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.