How to Send SMS using NodeJS and AWS Lambda
Time to read: 3 minutes
How to Send SMS using NodeJS and AWS Lambda
In the era of digital communication, SMS remains a vital tool for businesses to connect with their customers promptly and effectively. Leveraging the power of cloud computing and APIs, developers have found innovative ways to send SMS messages seamlessly. One method involves using AWS Lambda, a serverless computing platform by Amazon Web Services, in conjunction with Twilio. This integration allows for the development of lightweight, scalable applications for sending SMS messages, without the overhead of managing infrastructure.
Prerequisites
Before we can move forward, make sure you have the following.
- A Twilio Account: You can sign-up here
- An AWS Account: Register at AWS to open an account.
AWS Lambda to send SMS
Today we will create our own SMS sending service using AWS Lambda Functions and Twilio. Just imagine all the value and convenience that it puts in your hands.
Let’s start with creating the Lambda Function in AWS Console. Once you are logged into your AWS Account, you can search for AWS Lambda in the search bar.
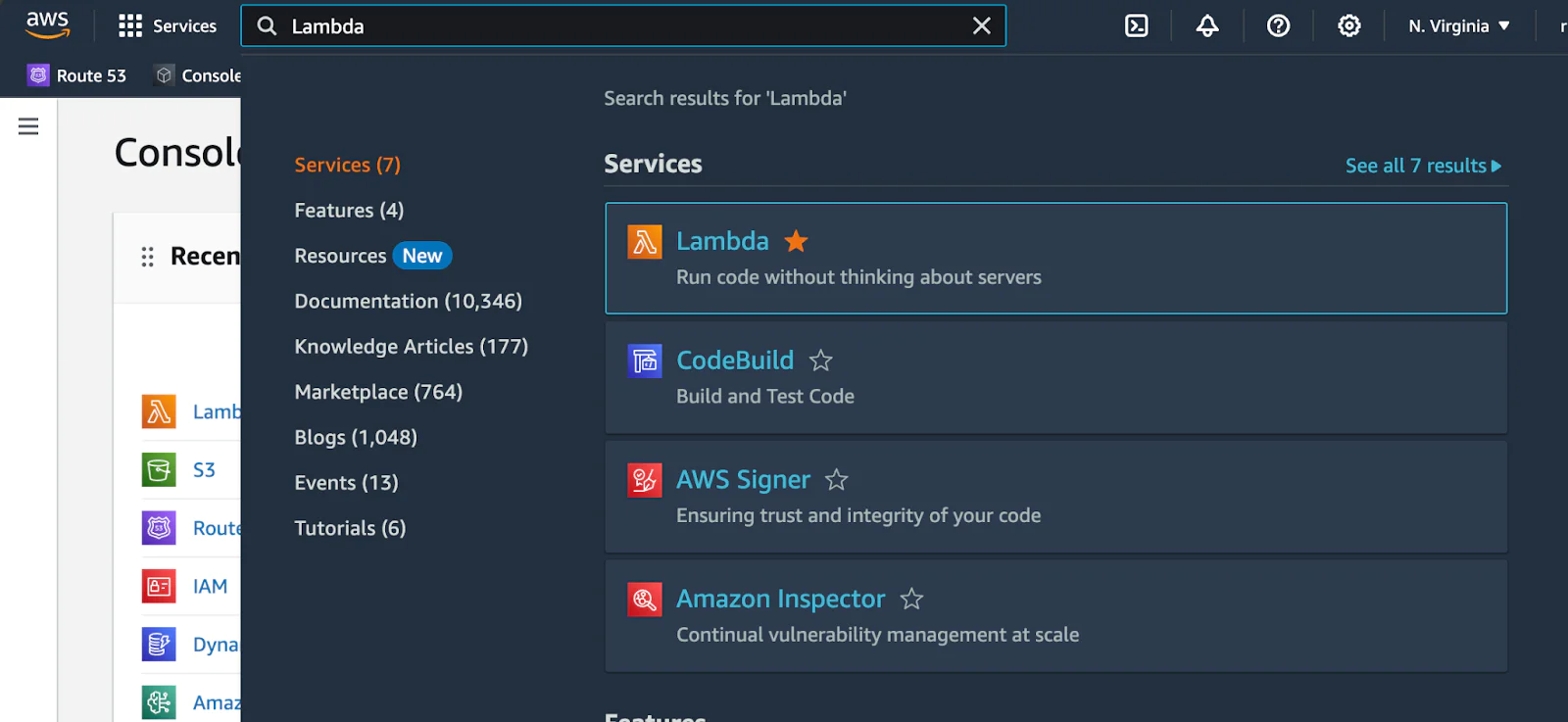
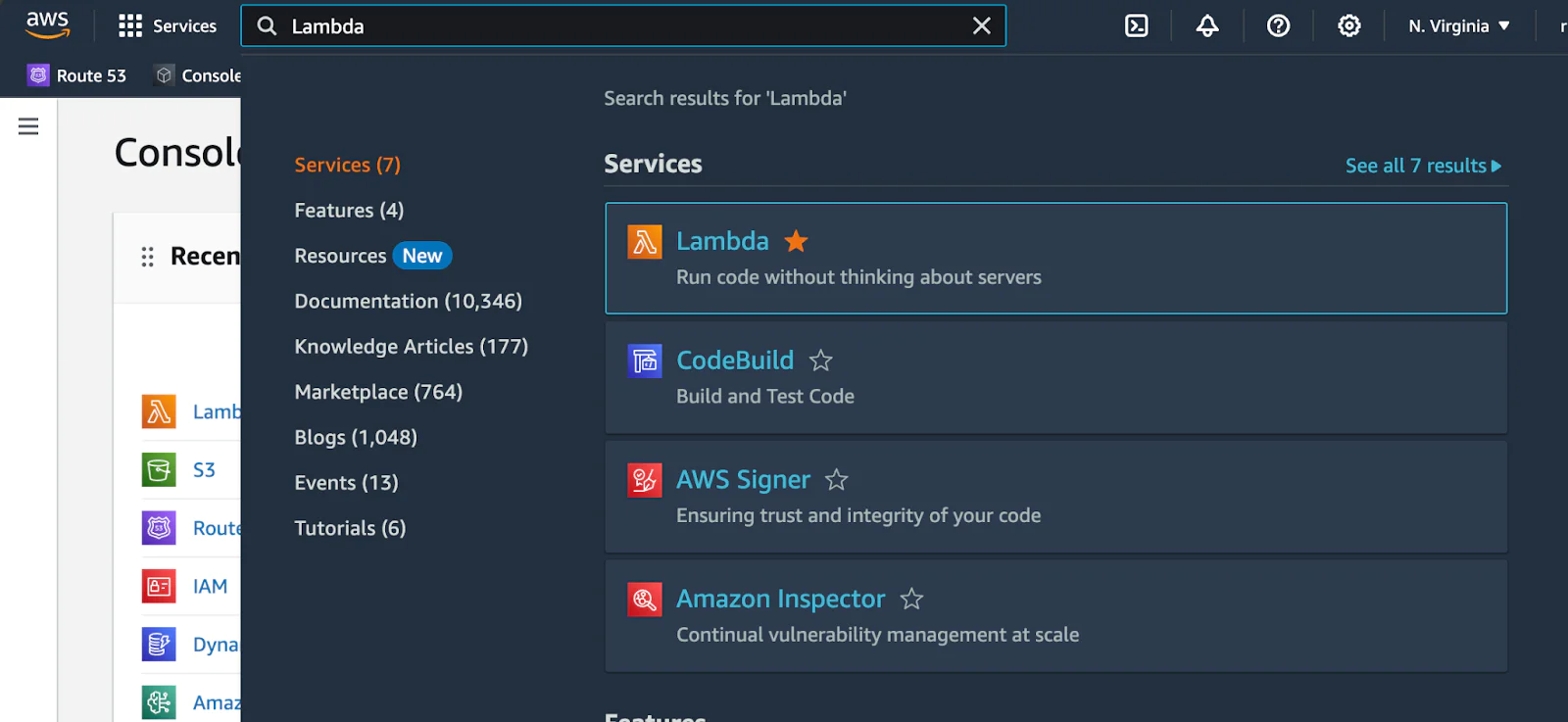
You will see all the Lambda Functions you might have, but to create a new one, click on Create function.
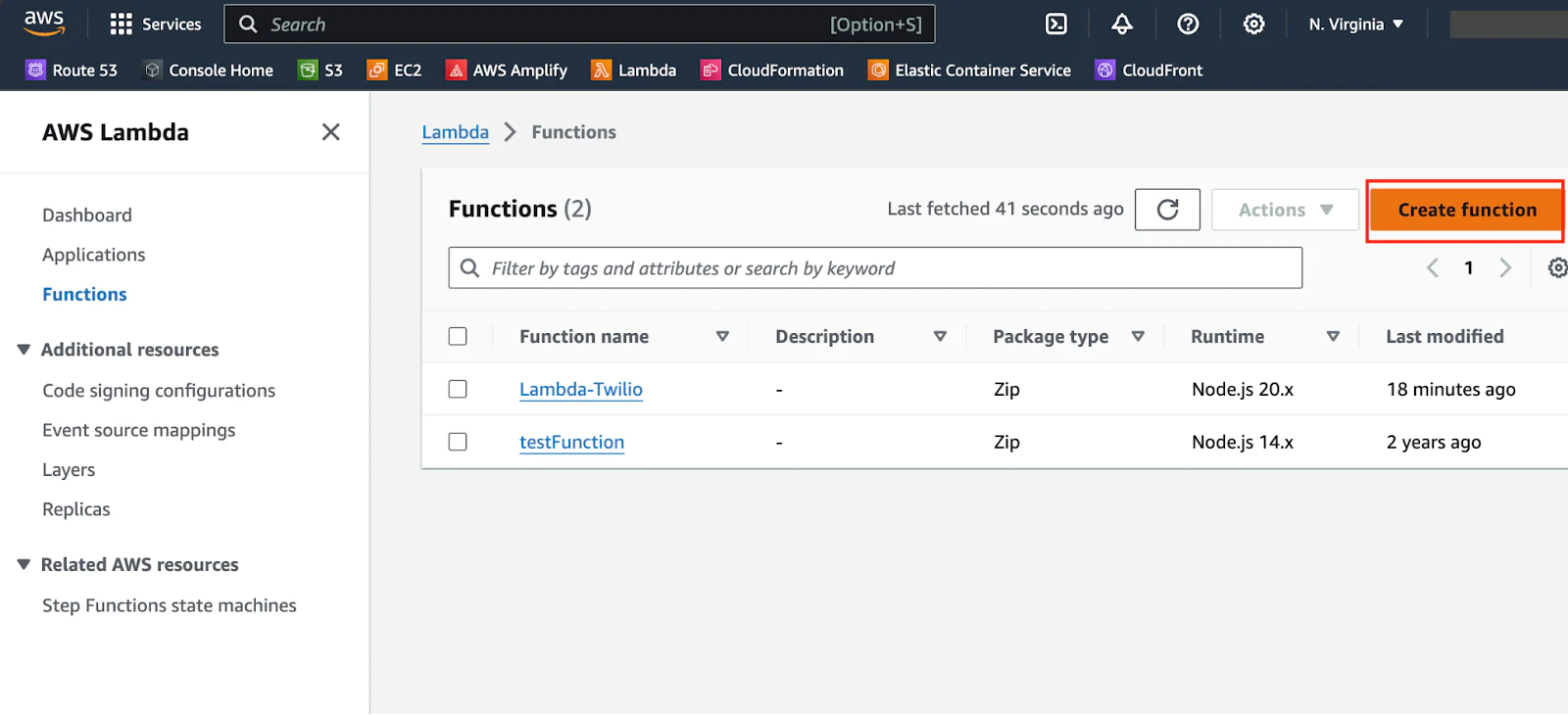
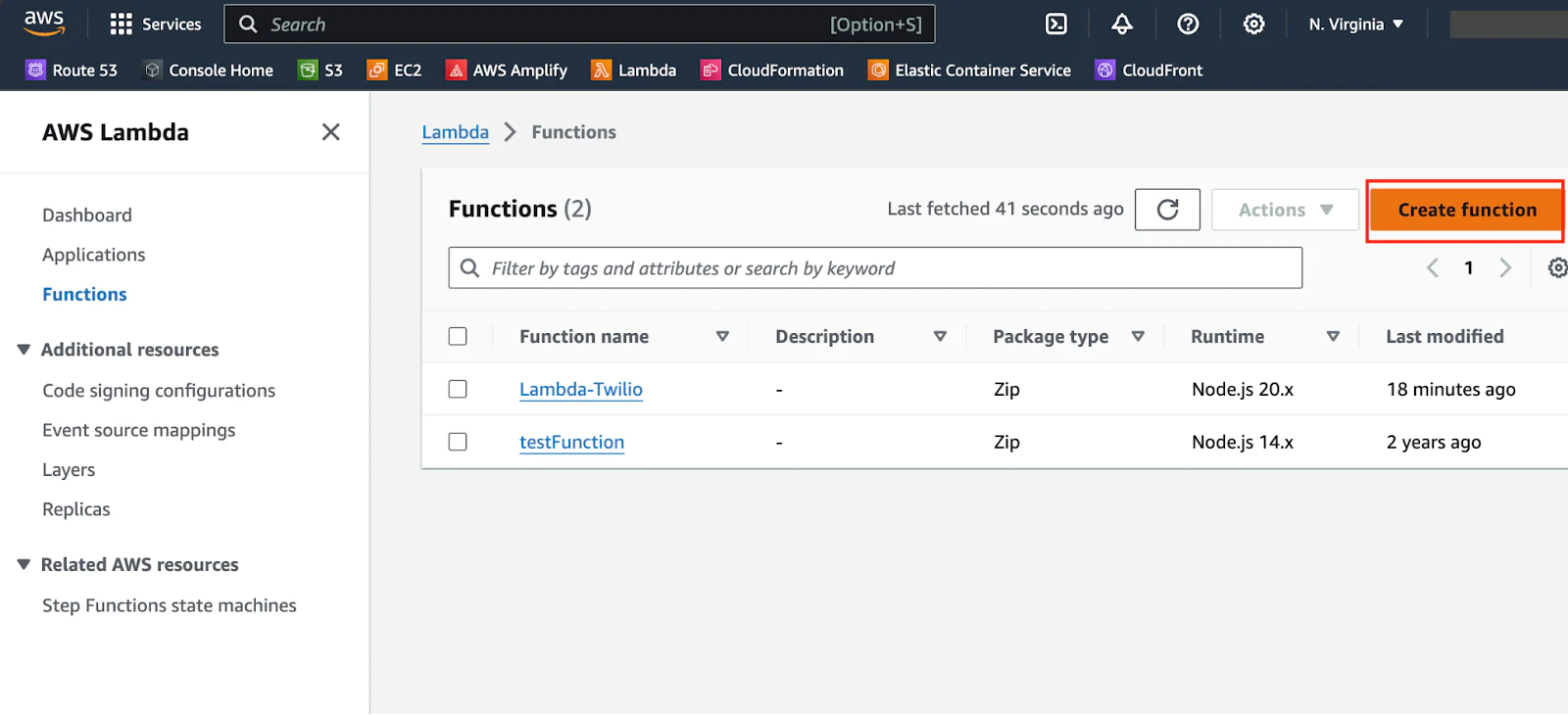
Let’s name the function lambda-twilio-sms
. Runtime is Node.js 20.x
. Leave the Architecture as x86_64
.
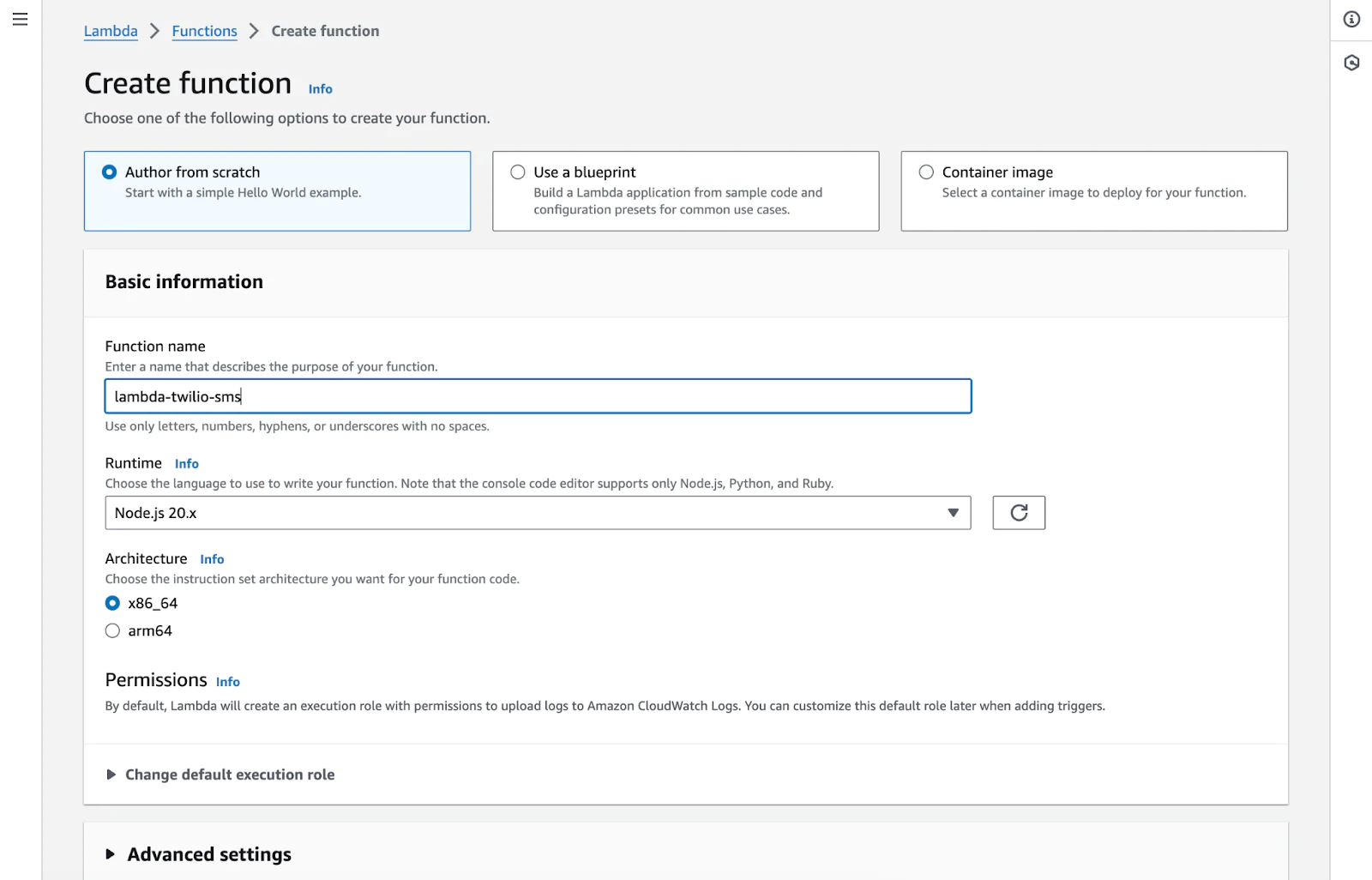
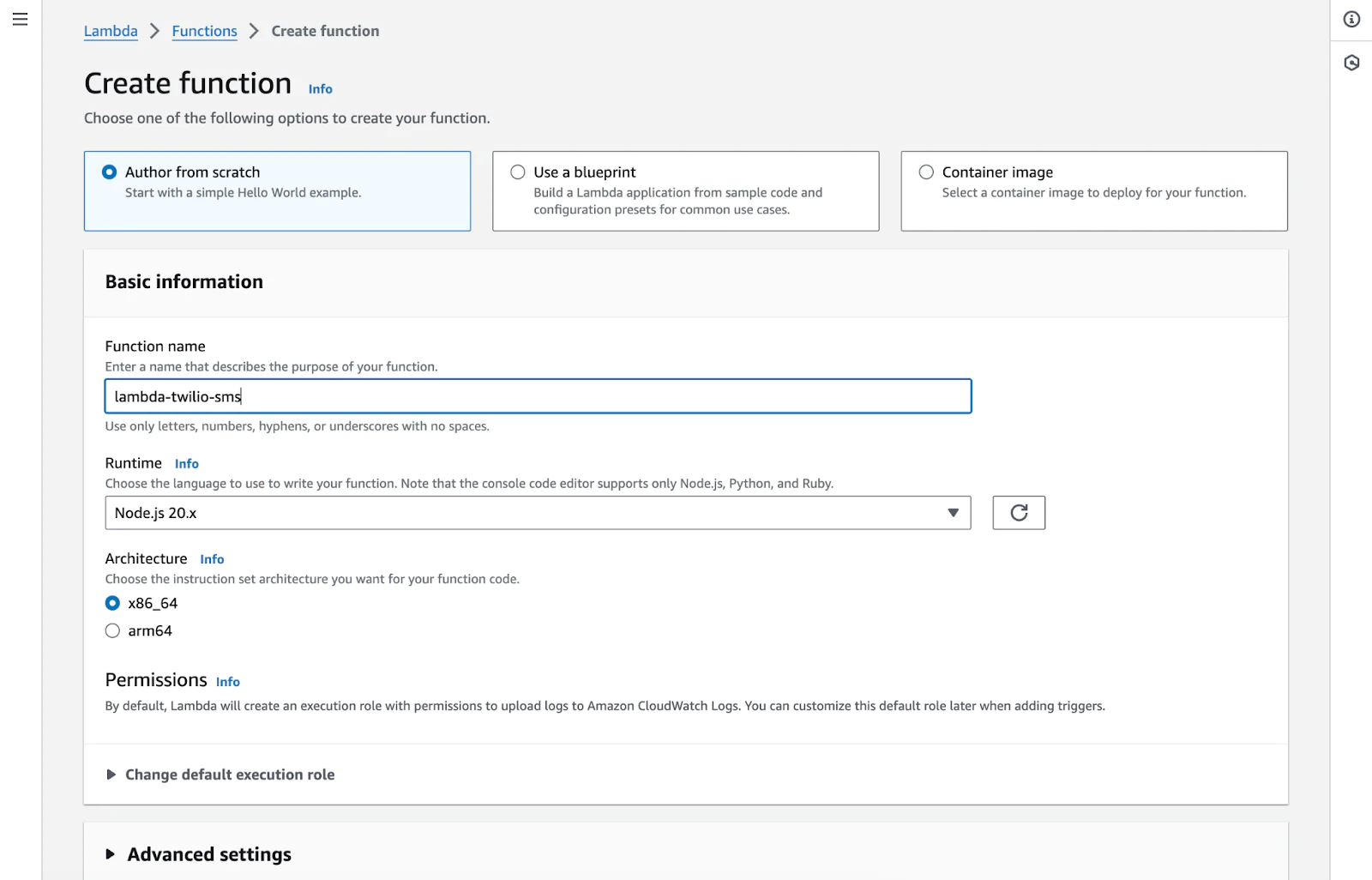
Now under Advanced settings, check the Enable function URL and select Auth type NONE. This allows us to send HTTP requests to our Lambda Function.
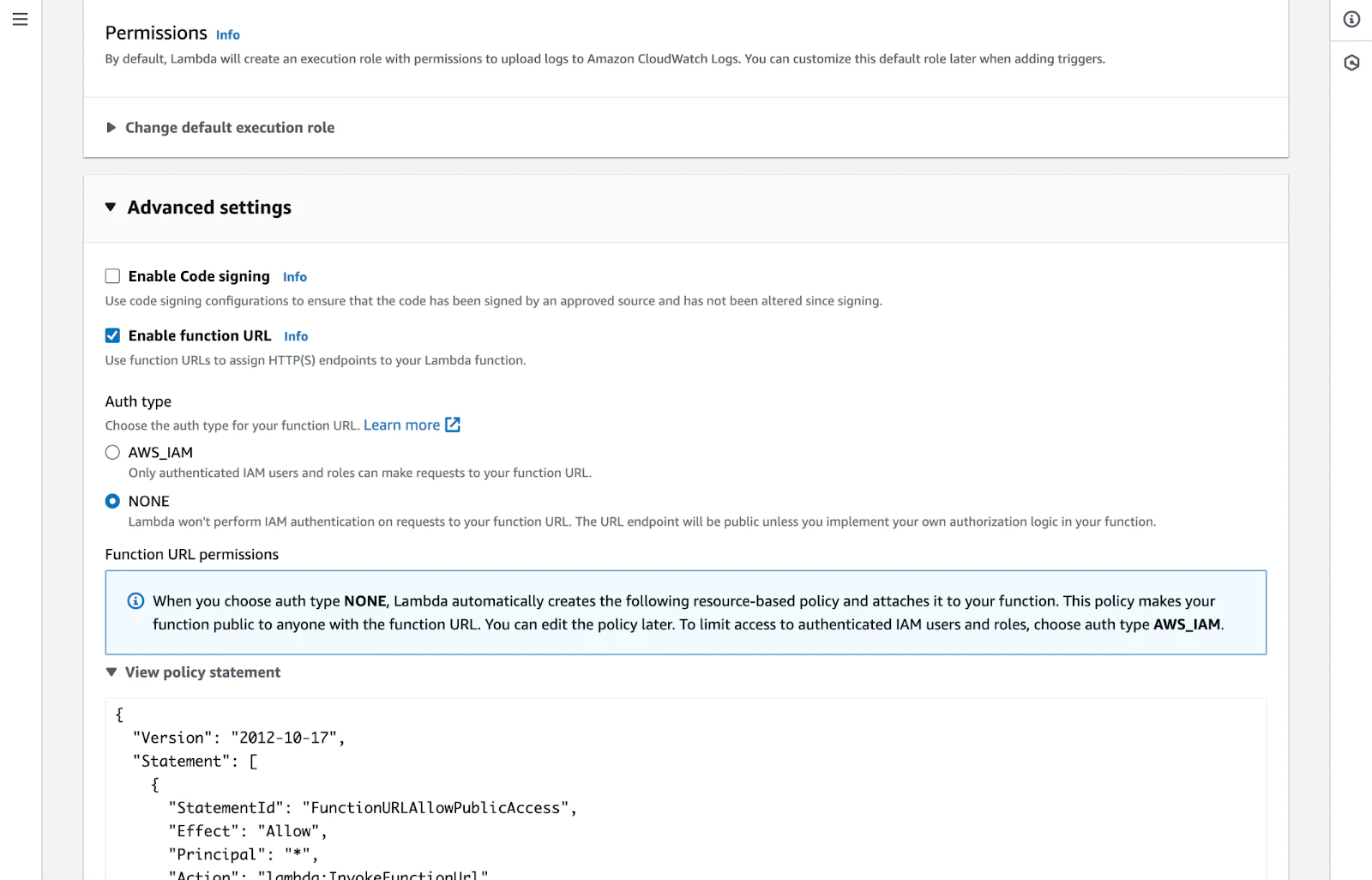
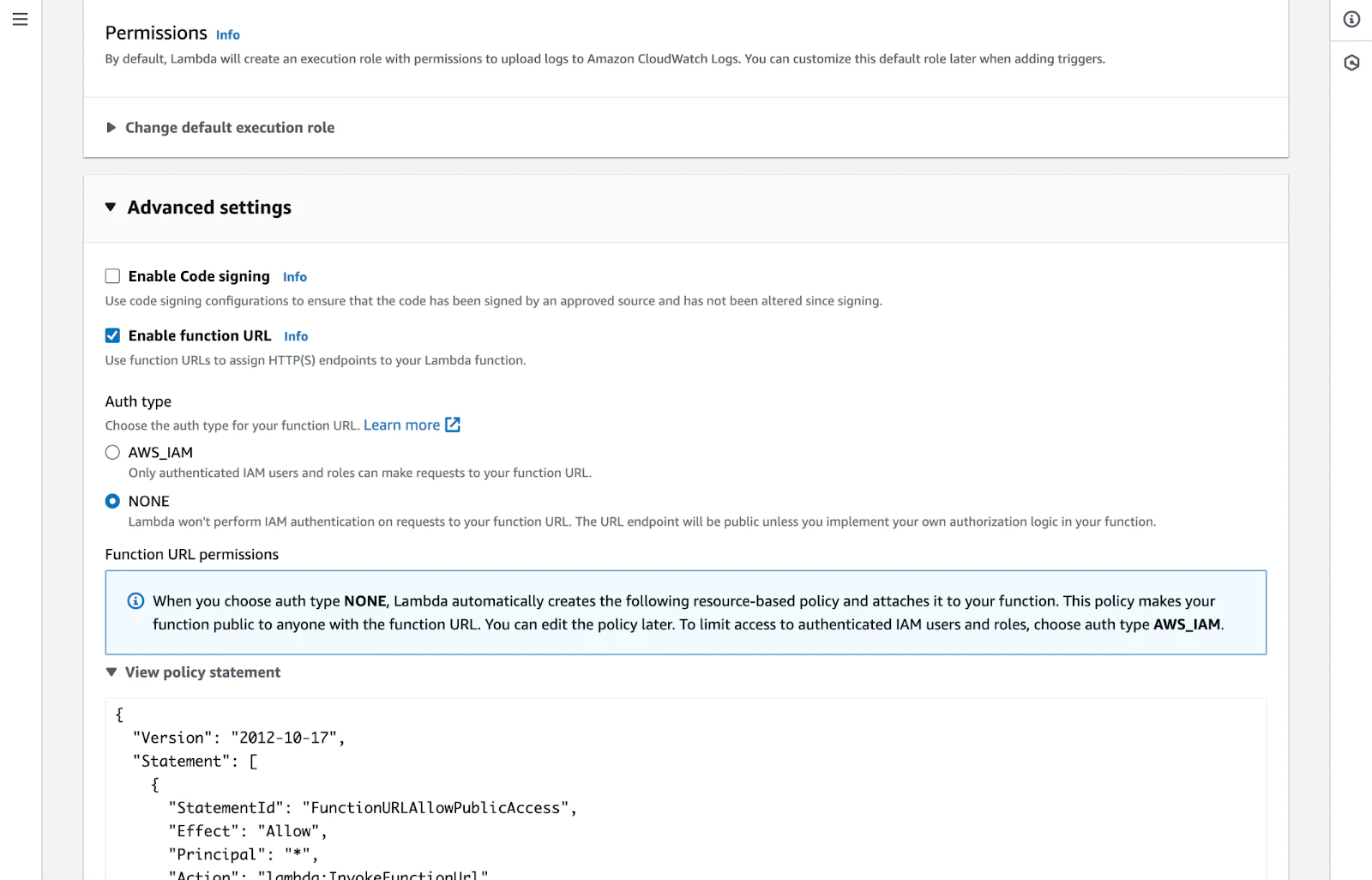
Writing Lambda functions in Node.js
Now let’s write some JavaScript code. Start by creating a directory locally named twilio-aws-lambda and initializing the node.
We will need the twilio
npm library, install it by running the following command in the console.
Let’s implement the function that uses the twilio
package to send SMS based on the provided request parameters. The account credentials will automatically be retrieved from the corresponding environment variables which we will set up later:
We have to zip the folder to upload to Lambda. You can do so by opening up the terminal, navigating into the folder and running.
Now, your project folder should have the zip file twilio-aws-lambda.zip that we will upload to AWS Lambda. In the AWS Management Console, within your Lambda Function, click on the Code tab, and you will see an Upload from
button on the right hand side, then select “.zip file” as the option and upload twilio-aws-lambda.zip.
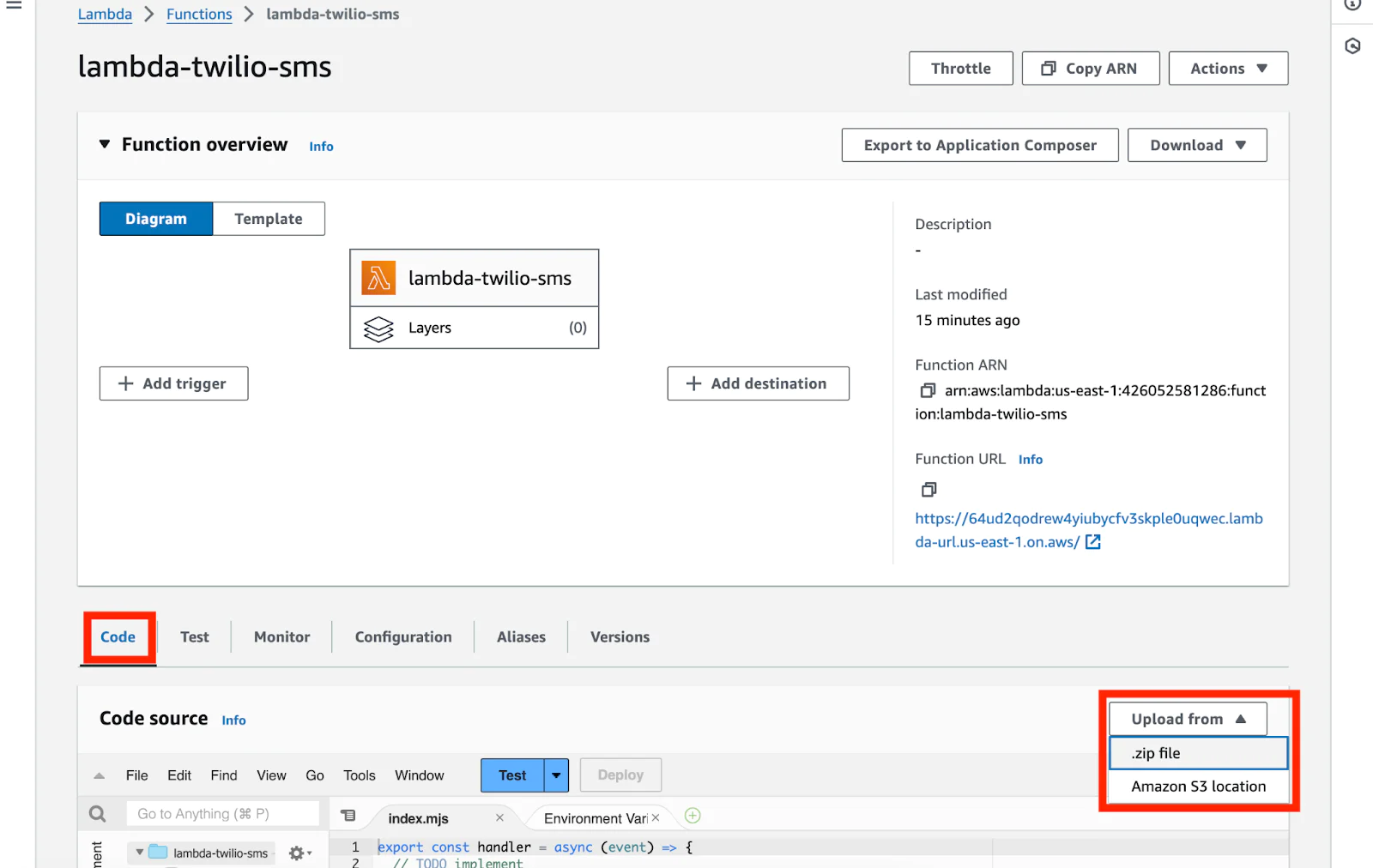
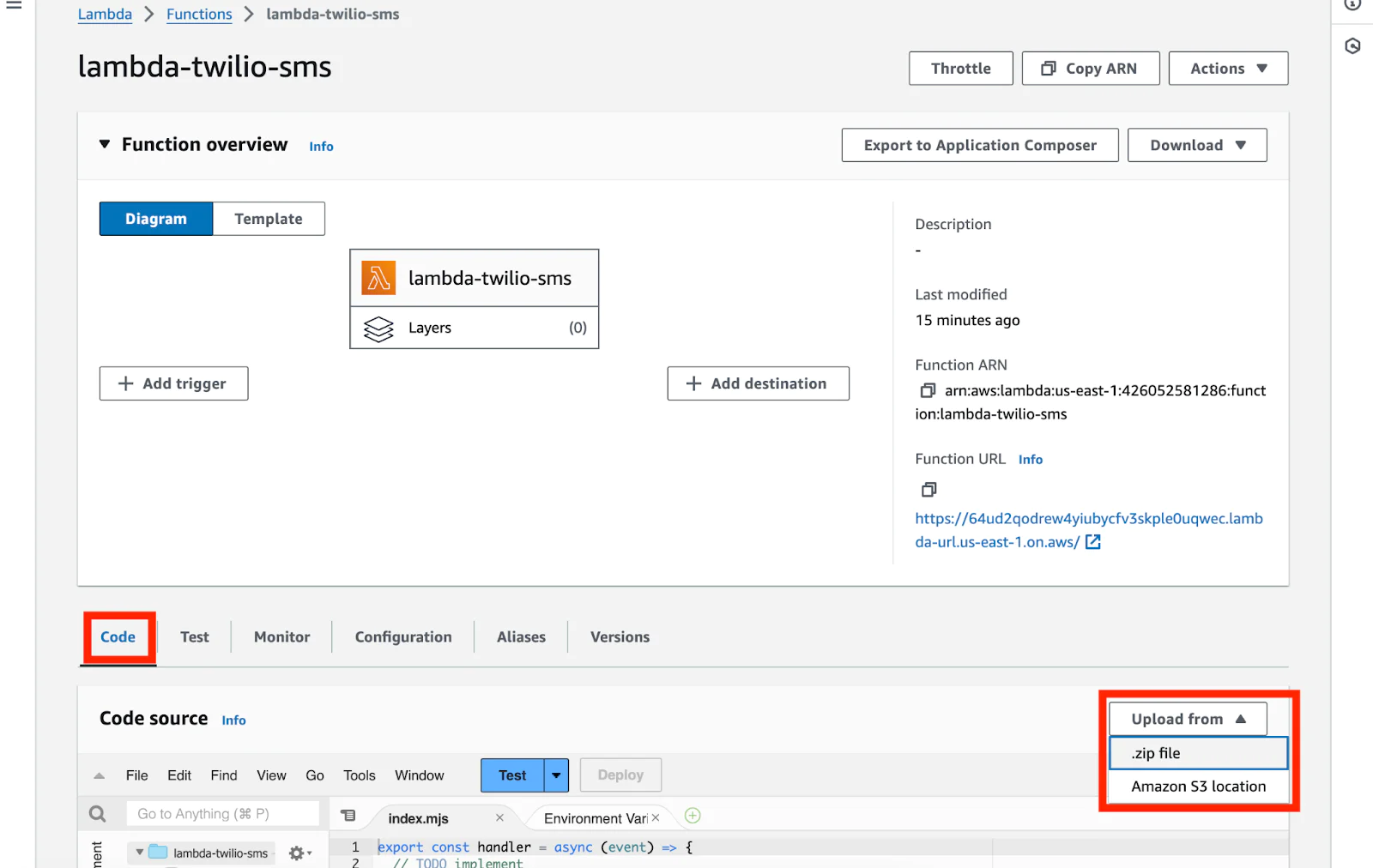
You can also use the AWS CLI. For this step, make sure you have installed and configured the AWS CLI and then utilize the update-function-code
, more details can be found here.
Before we can test our Lambda function, there is one last configuration we have to modify. The Lambda function timeout is set to 3 seconds by default, let’s change that to 60 seconds. Go to the Configuration tab and under General Configuration you will find the setting for timeout. Edit the timeout to 1 minute and then click Save.
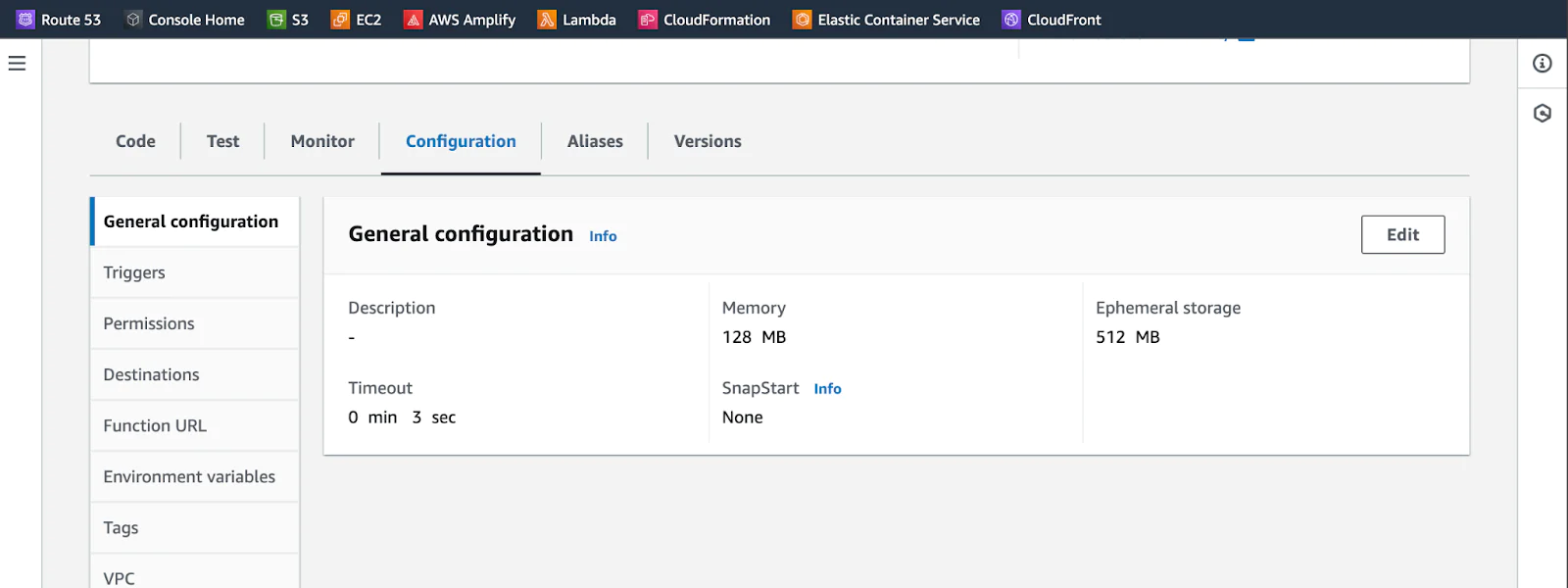
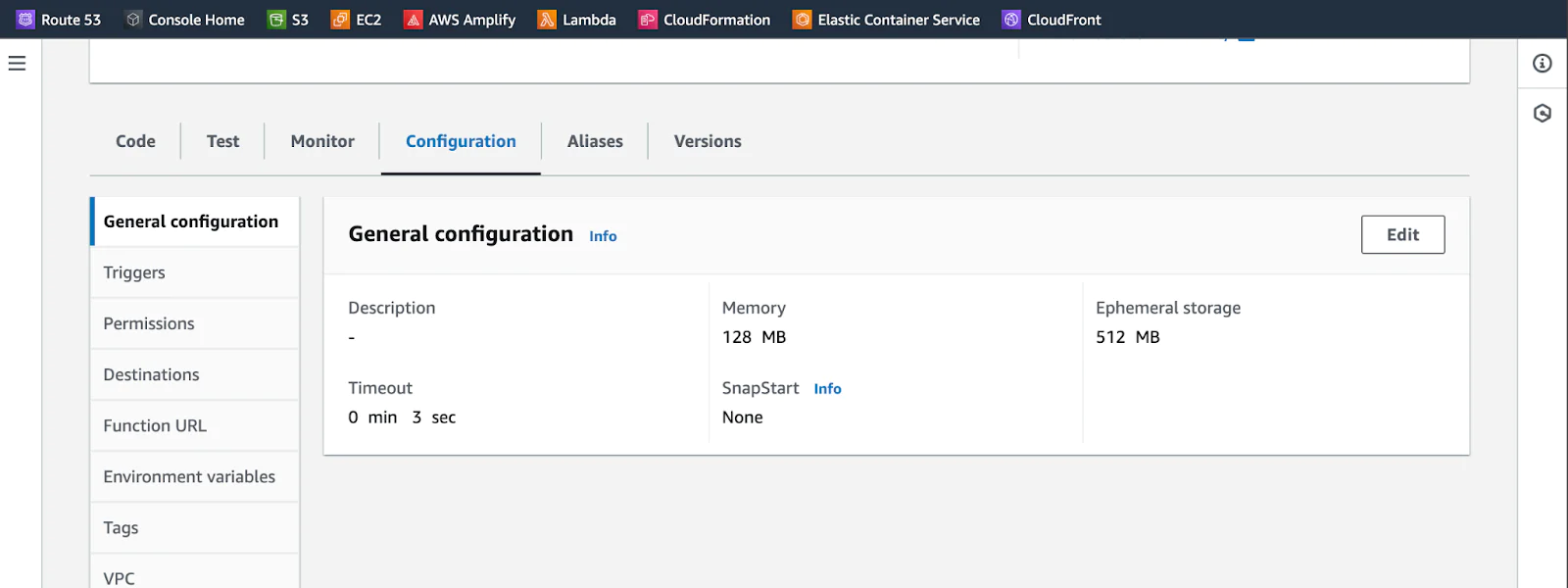
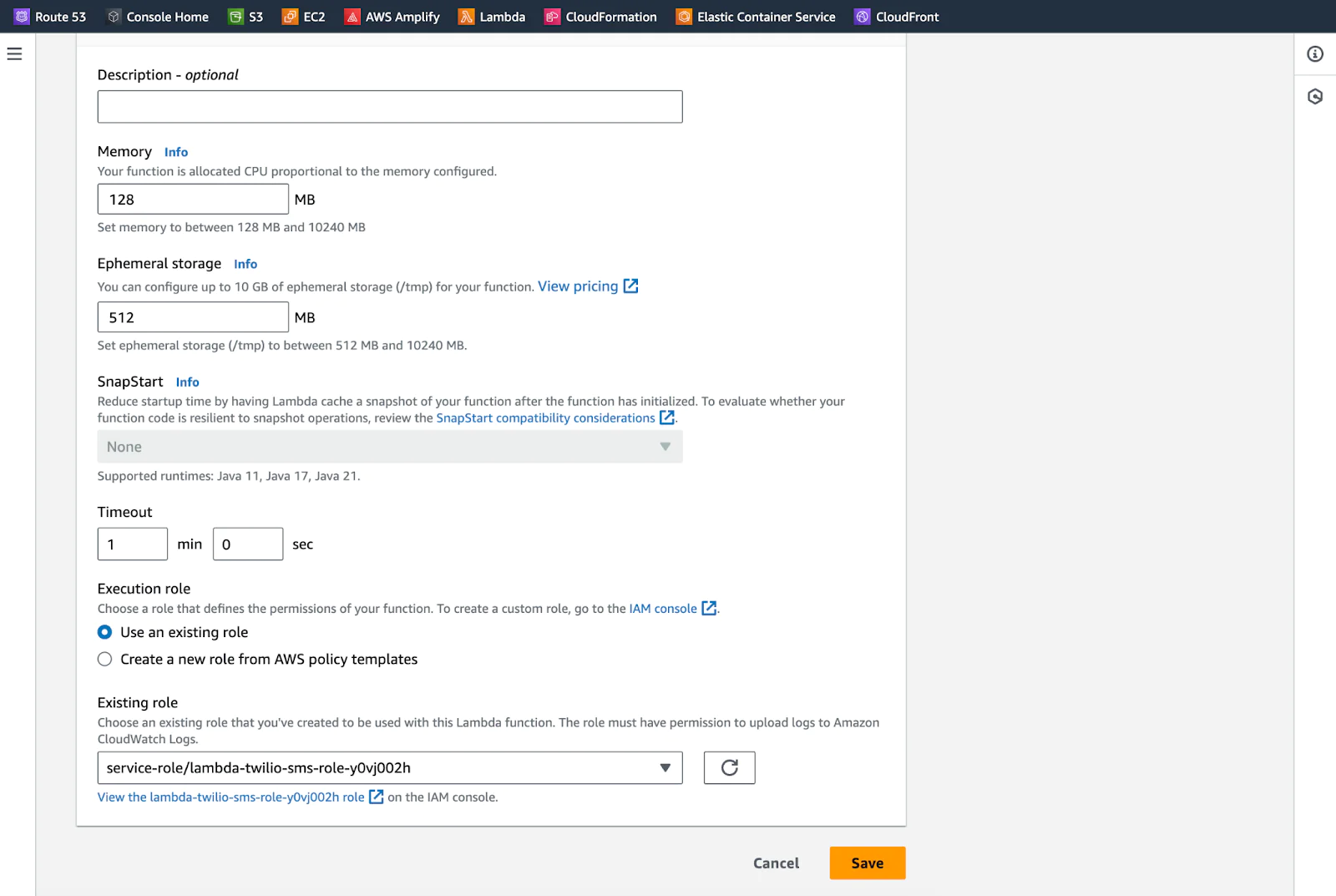
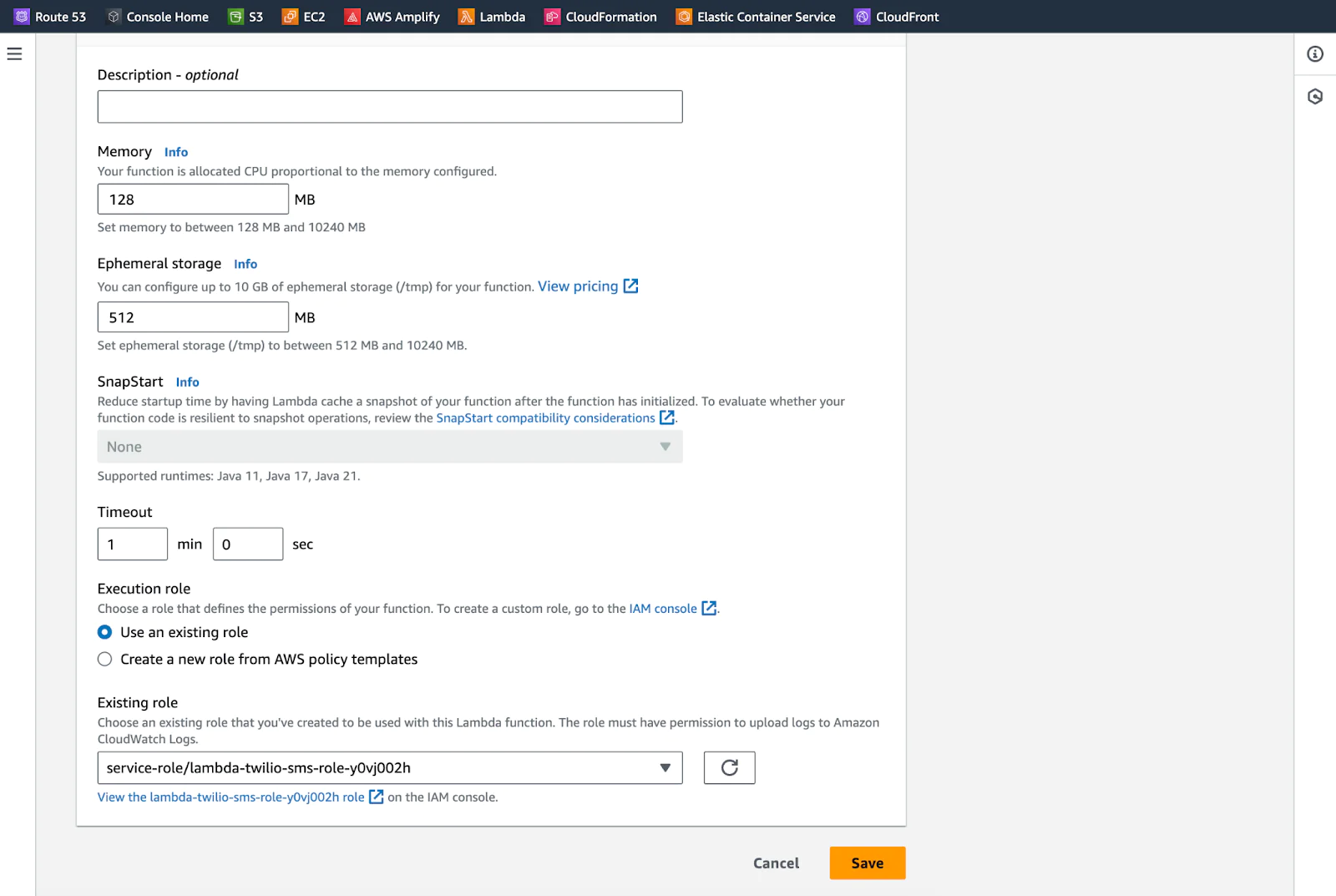
Configuring your Twilio Account
Once you are logged in to the Twilio Console, scroll down to find your Account SID and Auth Token. Copy these values, as we will save these as Environment Variables within AWS Lambda.
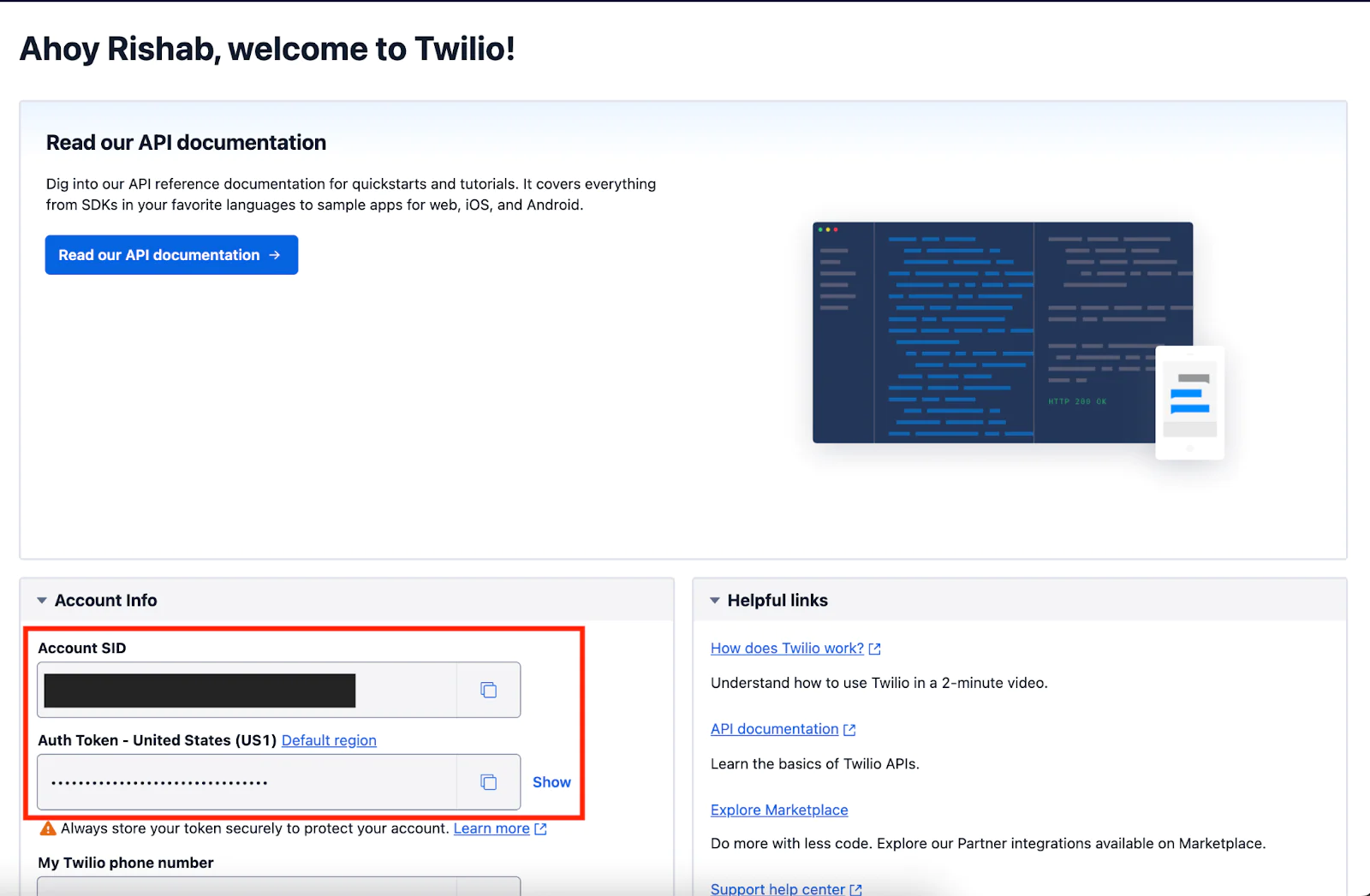
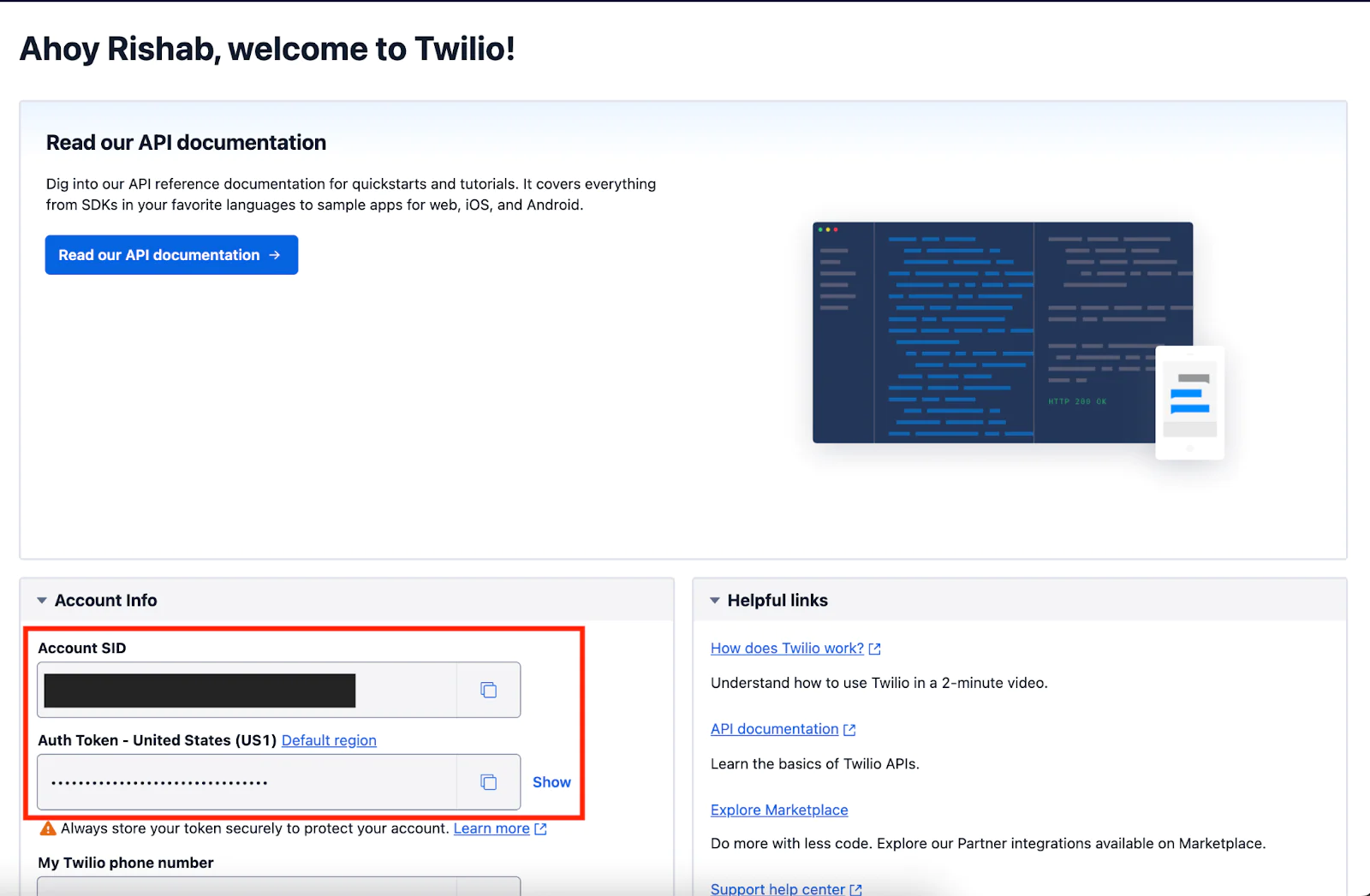
To send a message, you will need to buy a phone number—ideally from the region you are based in—to avoid roaming charges. To accomplish this, go to the Twilio Console and search for a number you’d like to buy.
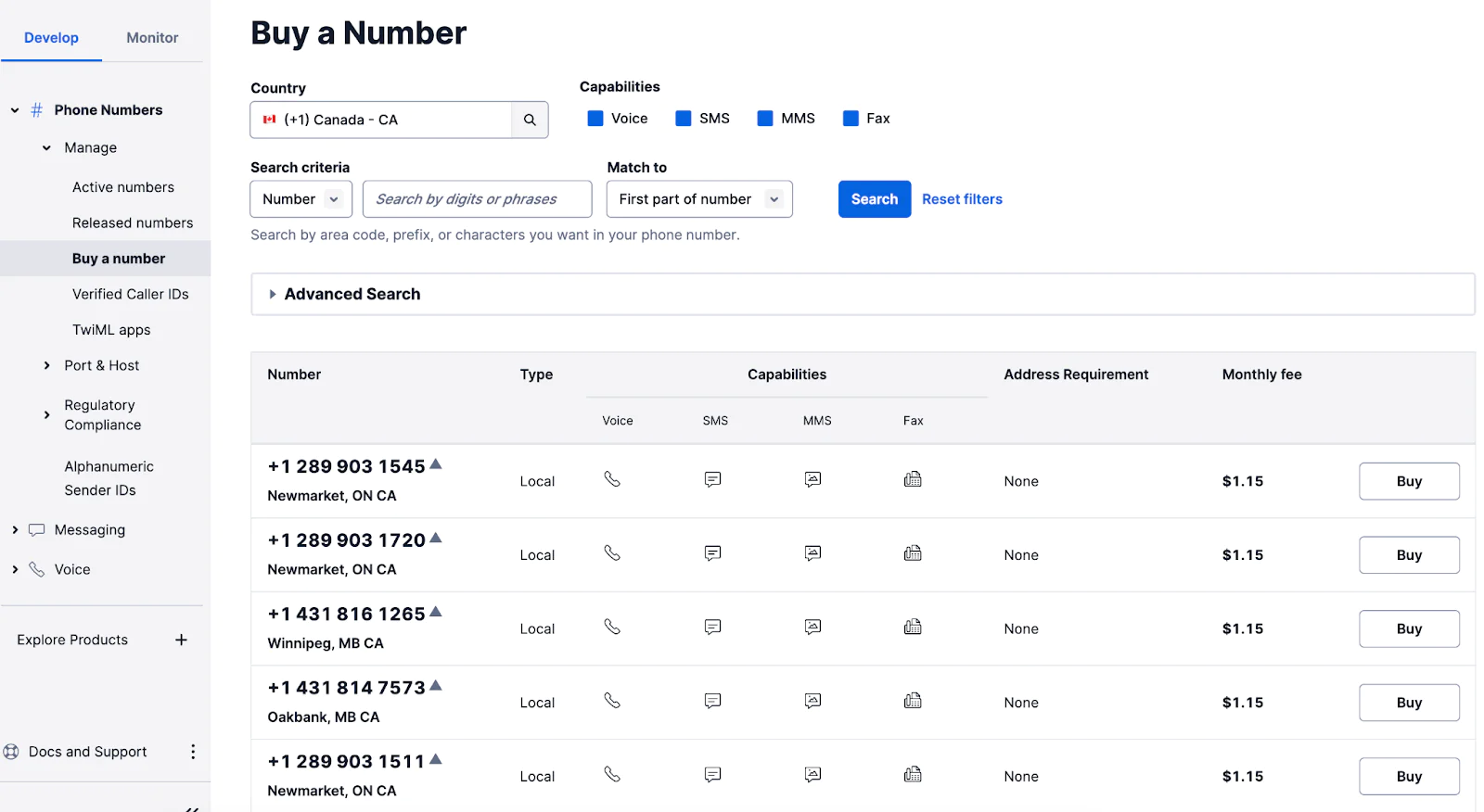
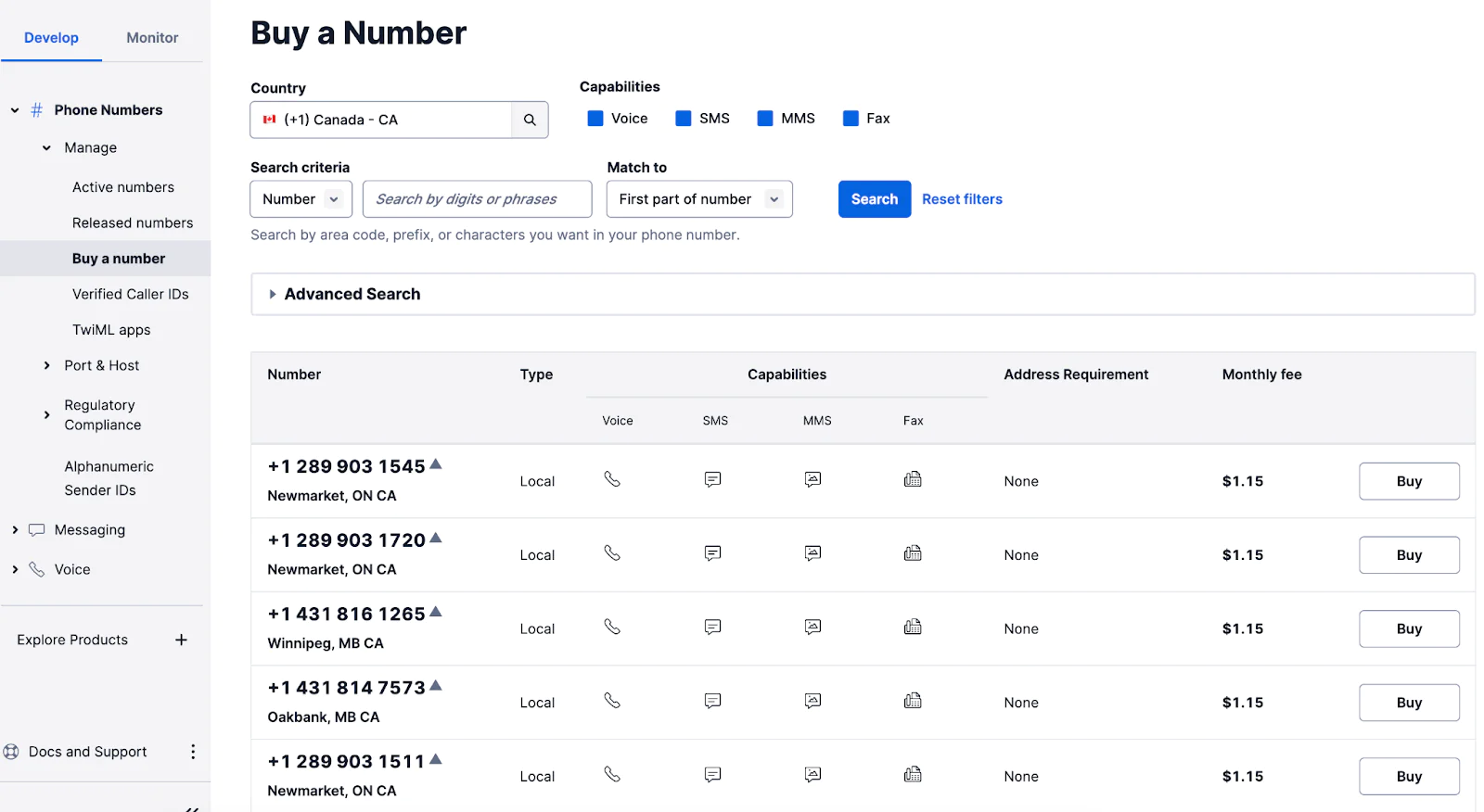
Head over to AWS Management Console and within your Lambda Function, click on Configuration tab and then select Environment Variables. Add the three values (TWILIO_ACCOUNT_SID, TWILIO_AUTH_TOKEN, and TWILIO_NUMBER) you copied earlier.
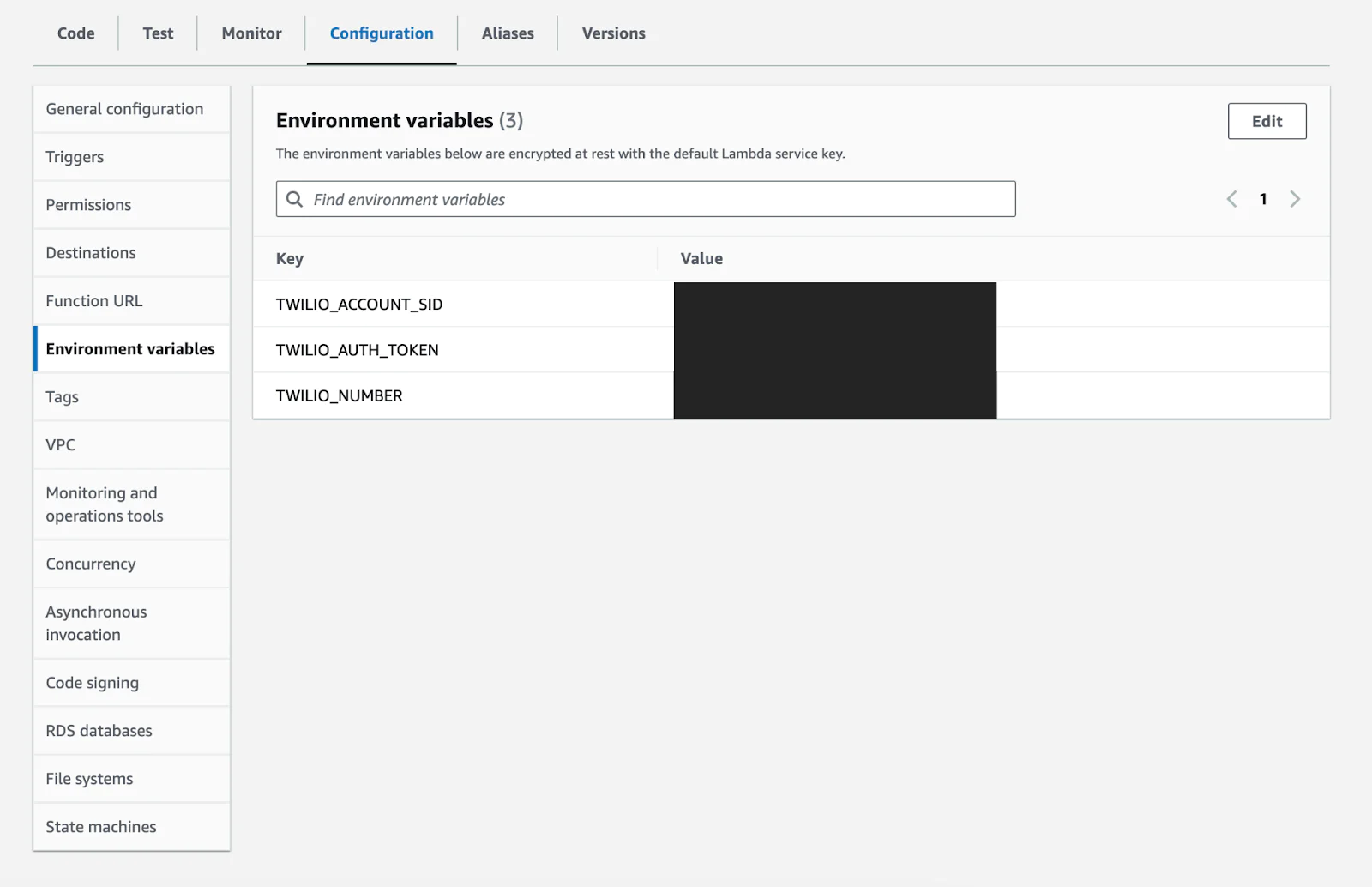
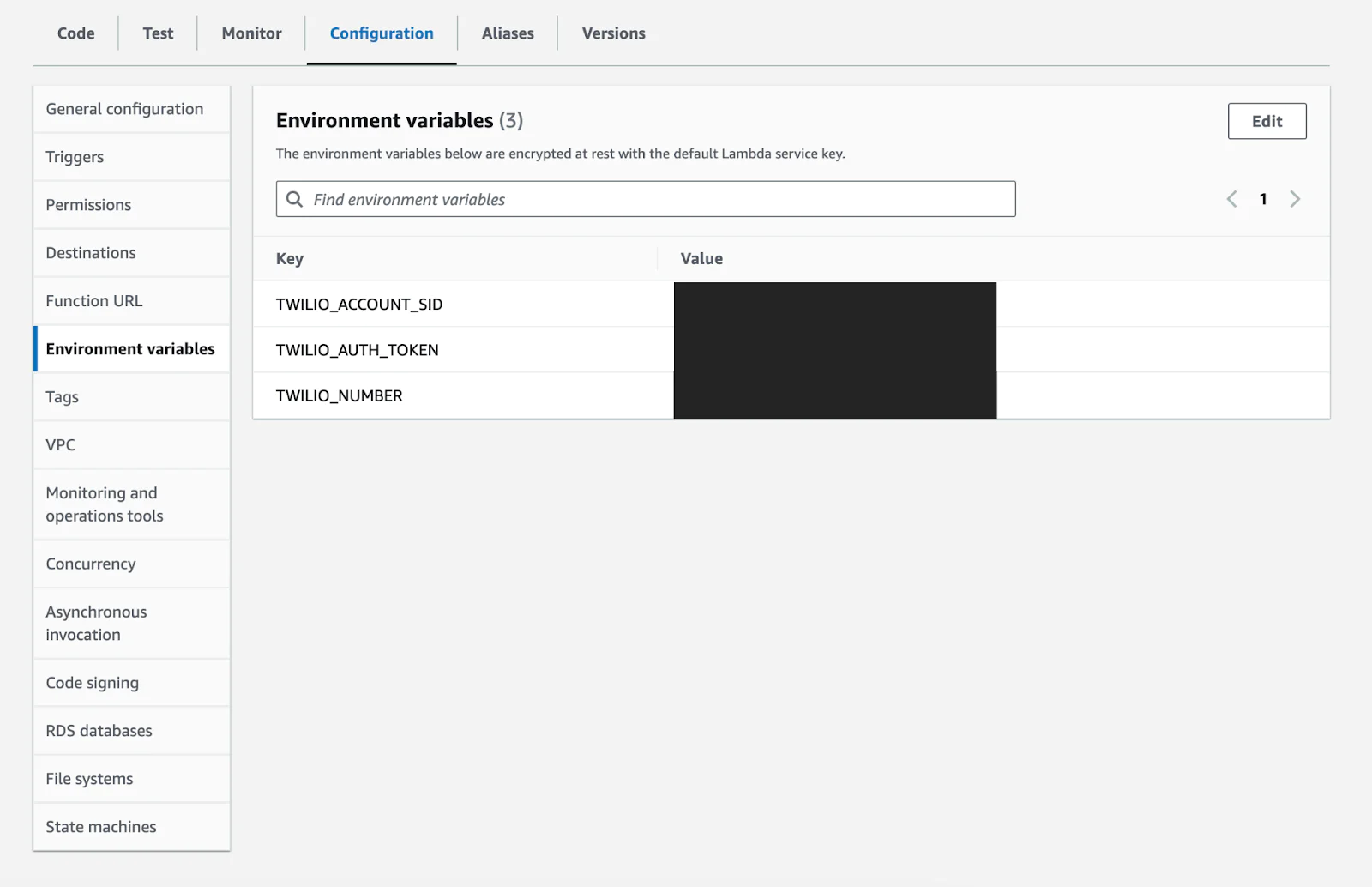
Send a test message with AWS Lambda
Go to the Test tab and Create new event. Let’s name it “TestSMS” and in the Event JSON field add a valid to
number (number you want to send text to) and body
for the SMS.
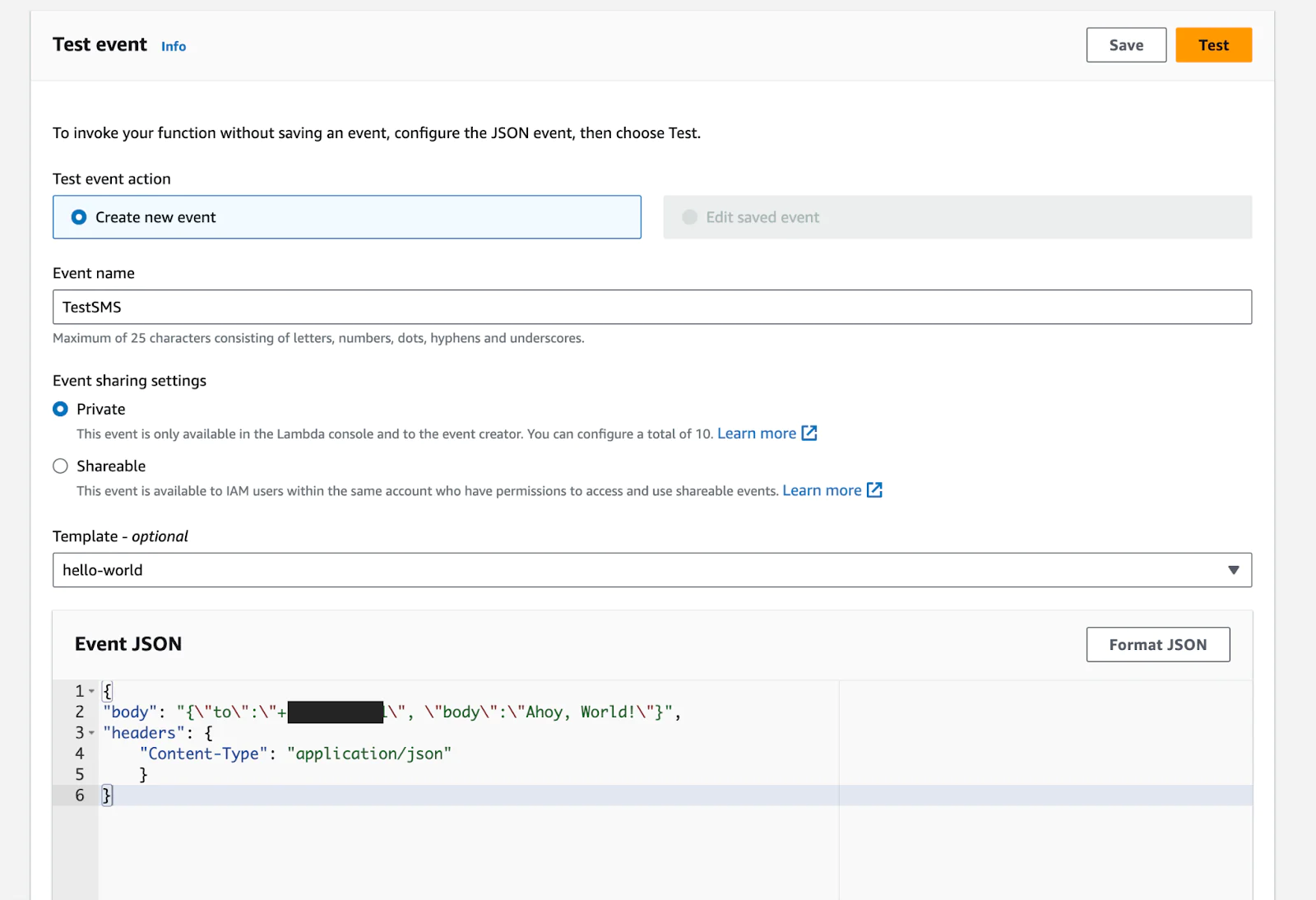
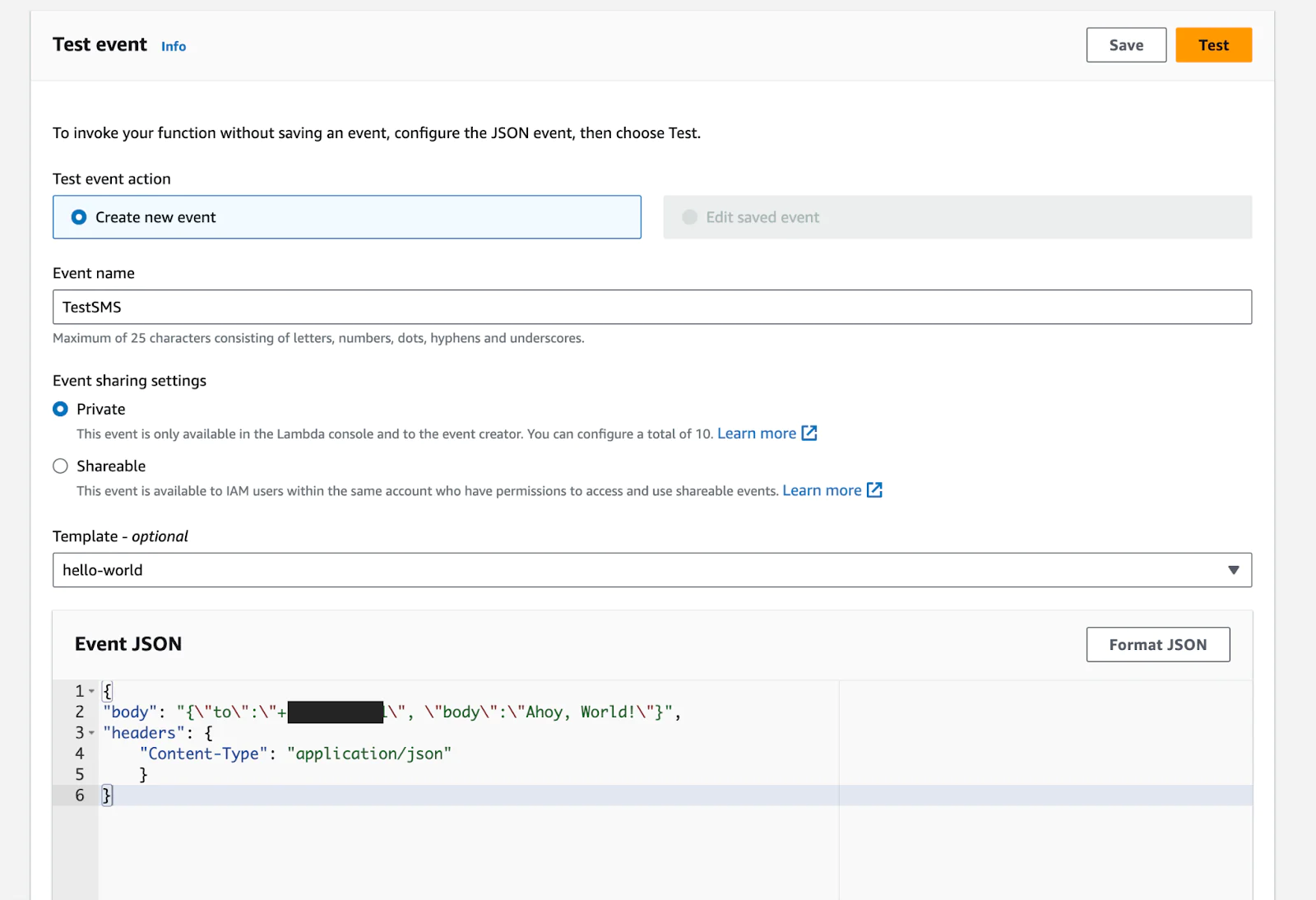
Click on Save and then Test.
After you hit Test, the function executes the code and you should see the log output.
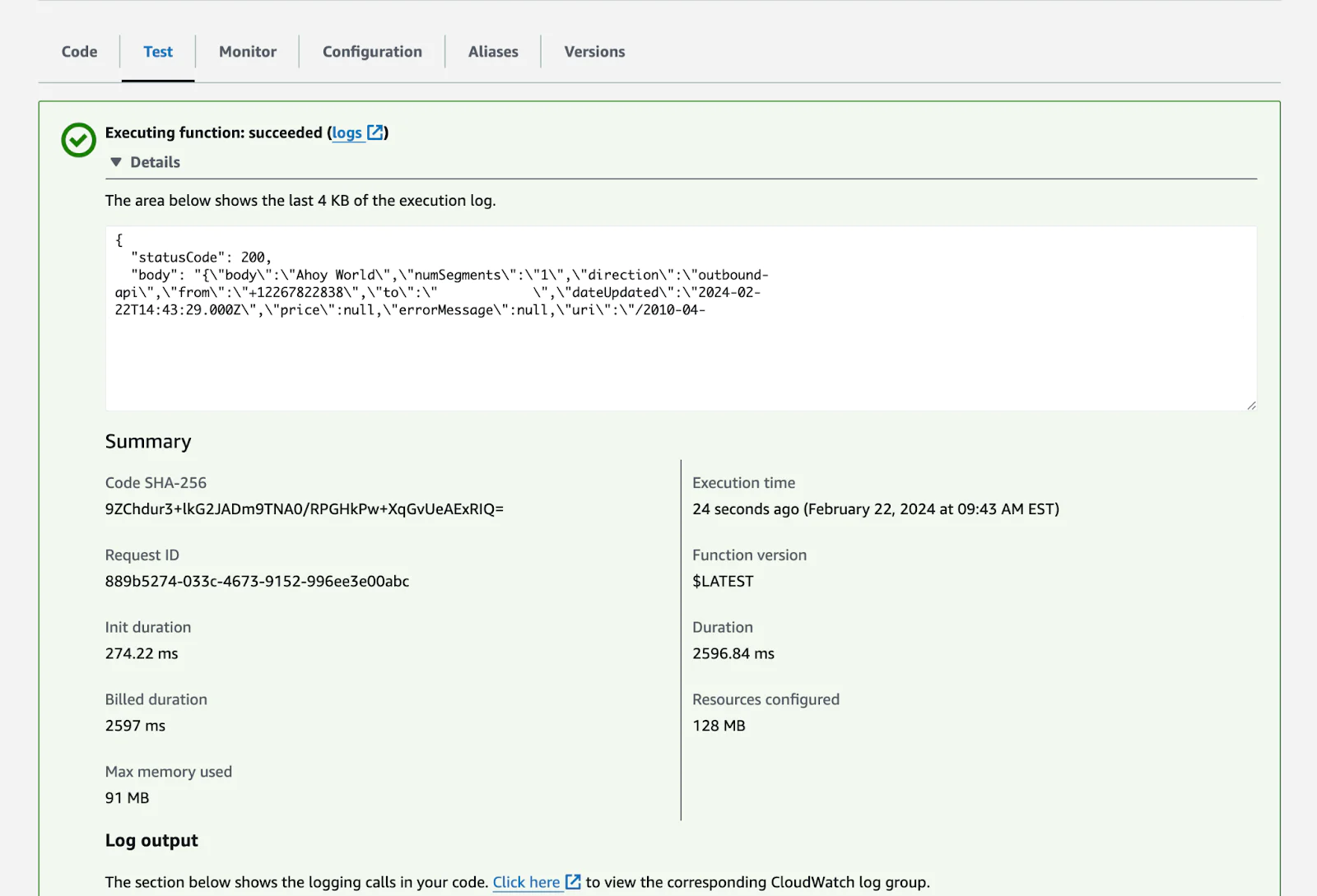
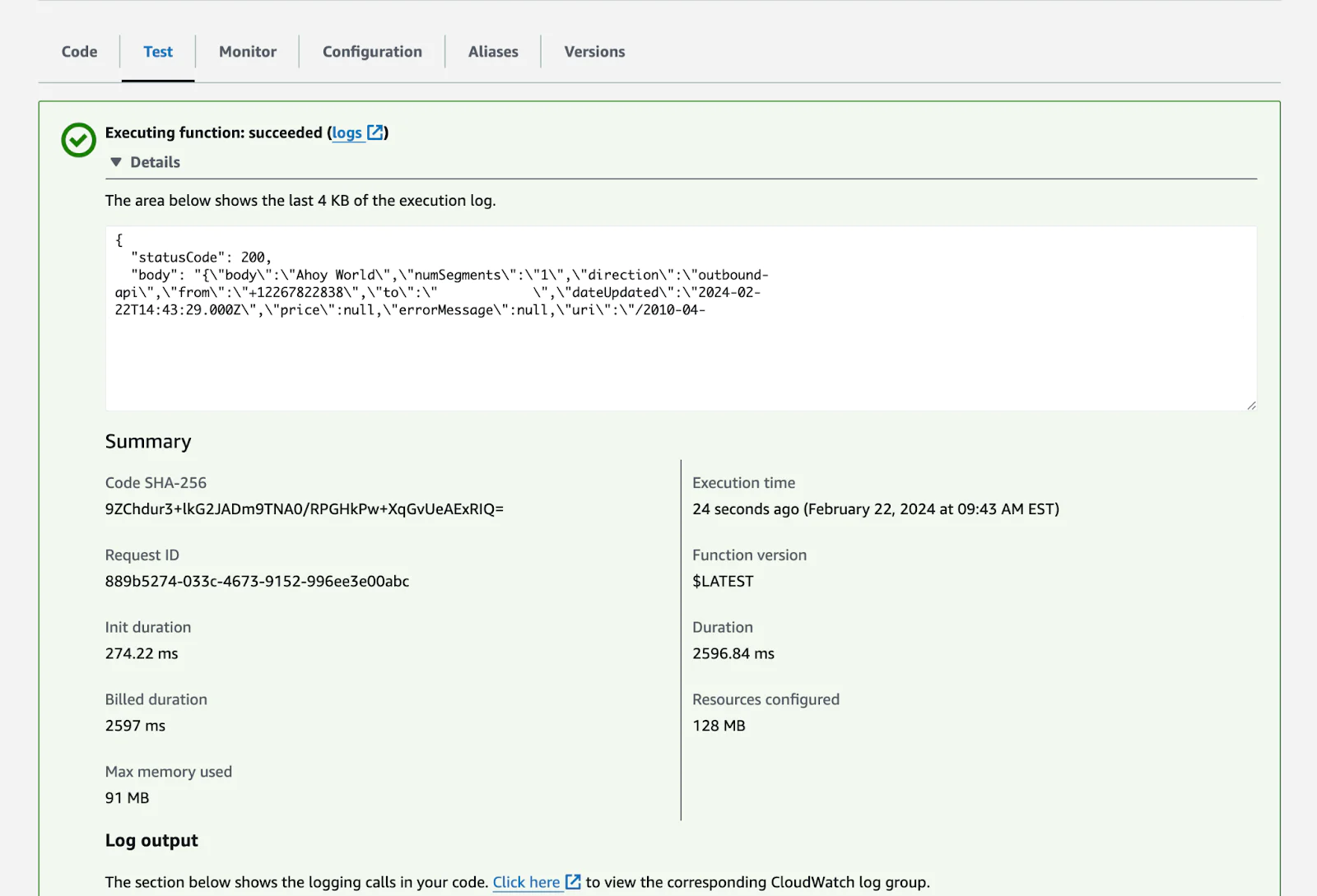
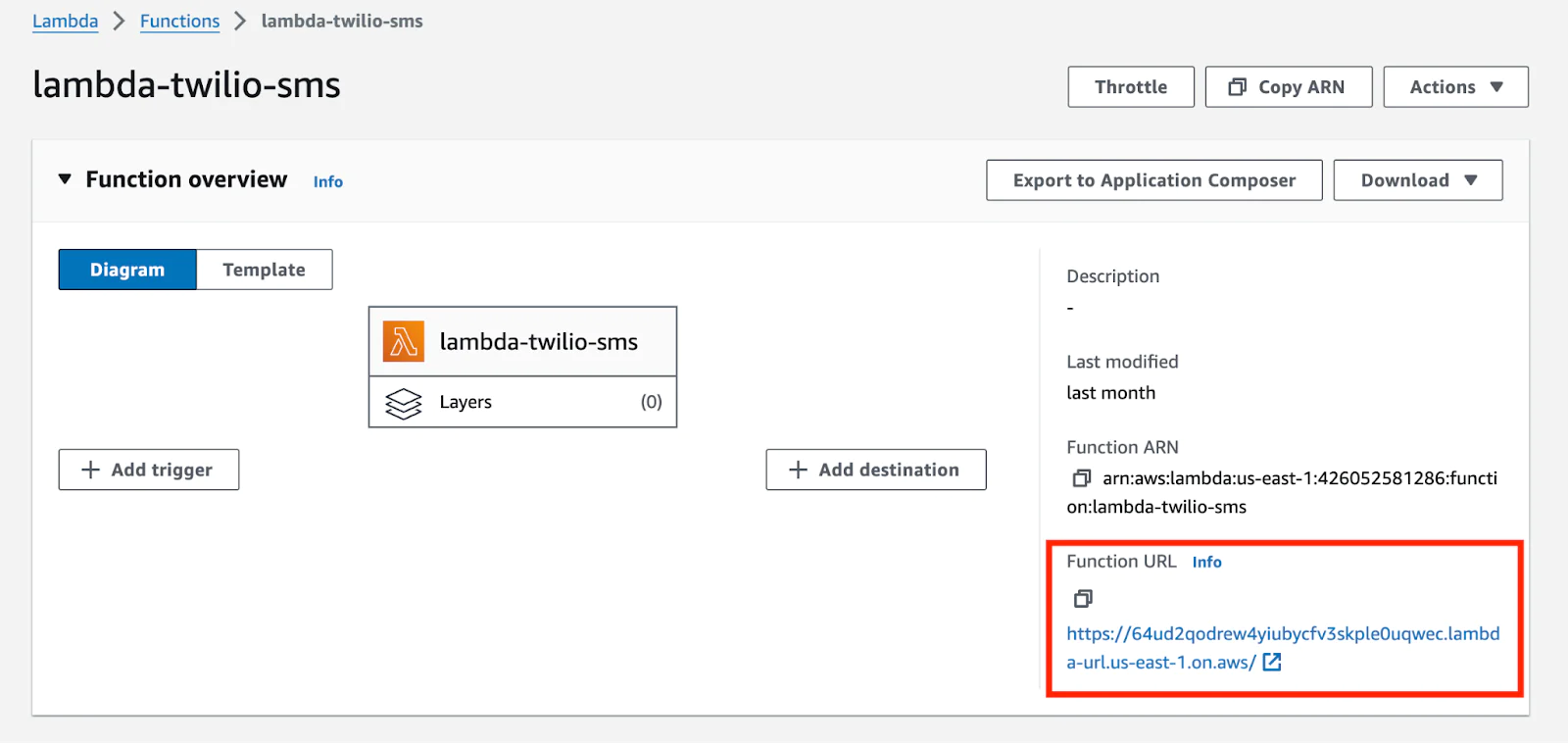
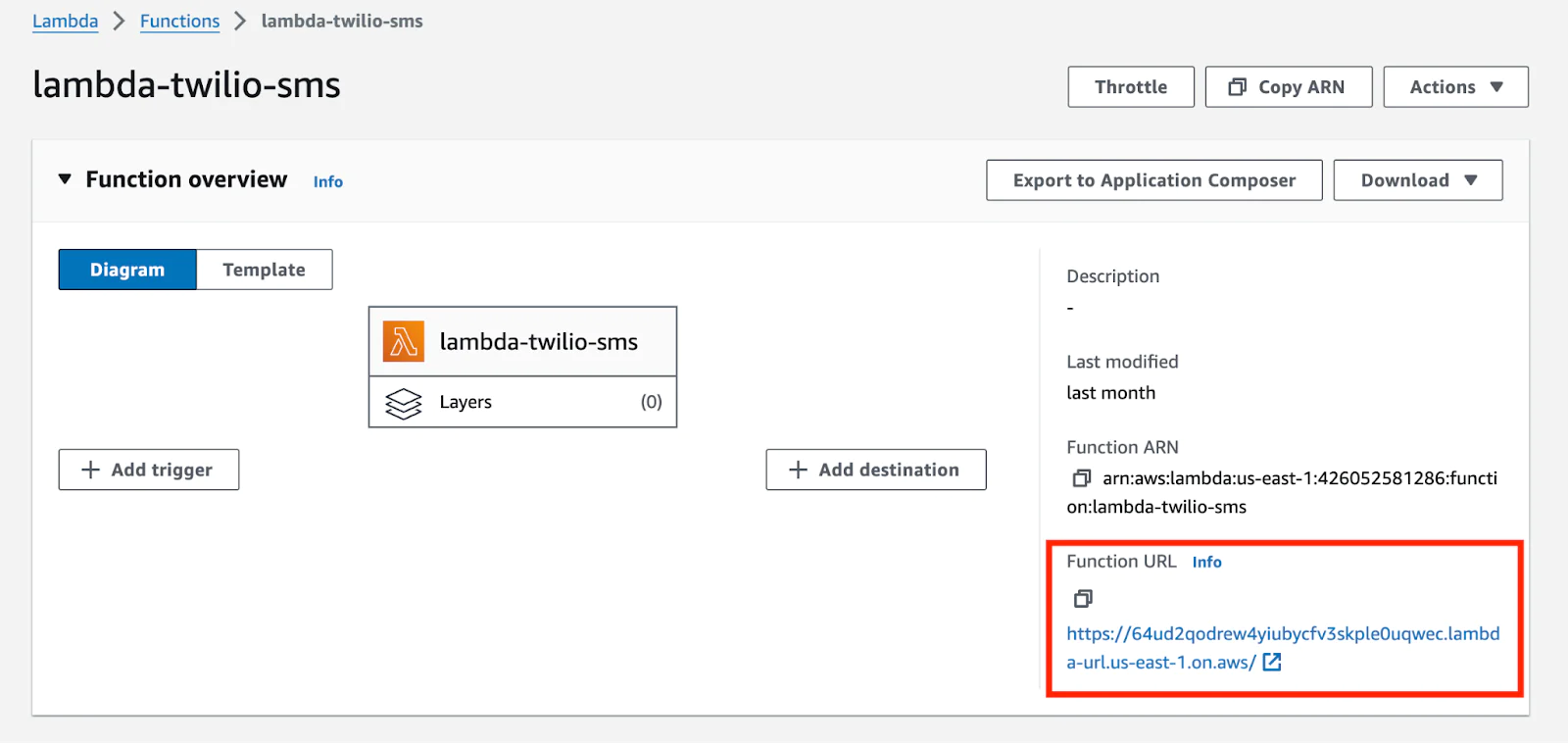
Here is a curl request example:
And Voila! You have built an AWS Lambda Function that you can send SMS with by sending an HTTP request with a valid to
phone number and message as body
.
Conclusion
In conclusion, integrating AWS Lambda with Twilio to send SMS messages using NodeJS offers a powerful, scalable, and cost-effective solution. This combination leverages the serverless cloud infrastructure of AWS and the seamless communication capabilities of Twilio, providing a reliable and efficient way to send SMS. With the simplicity of NodeJS and Serverless, developers can easily implement and maintain this solution without the need for extensive infrastructure. This integration not only streamlines the process of sending SMS messages but also opens up possibilities for other innovative applications. I can’t wait to see what you build!
Rishab Kumar is a Developer Evangelist at Twilio and a cloud enthusiast. Get in touch with Rishab on Twitter @rishabincloud and follow his personal blog on cloud, DevOps, and DevRel adventures at youtube.com/@rishabincloud.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.