Shannon Turner Builds A Pet Cam Using Django, Raspberry Pi and Twilio
Time to read:
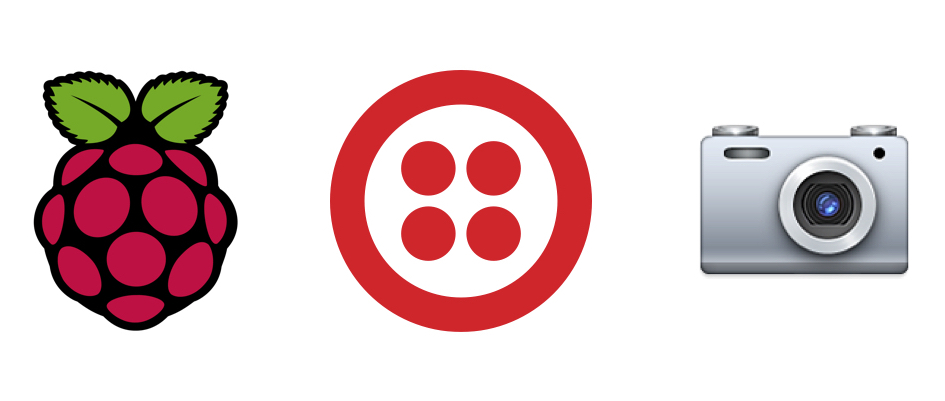
Thanks to the power of code, your pets are never more than a POST request away. Shannon Turner built a Twilio MMS, Raspberry Pi + Django hack so she can see what her winged companion, a lovely parrot, is up to when she’s out.
“Any time I miss my pet, a photo of him playing with his toy is only a text message away,” says Shannon. “I’ve had BudgieCam running for just over a month and I’ve already taken over 200 photos and nearly 100 videos.”
Building BudgieCam
this is what greets me whenever I walk into the room ? ? ? pic.twitter.com/HMIQwsAWsk
— Shannon Turner (@svthmc) August 5, 2016
When Shannon is on the road, she sends a text to her Twilio-powered number. Twilio gets that requests and fires off a POST request to Shannon’s Django site which triggers her Raspberry Pi (complete with Raspberry Pi Camera) to take a photo. The photo is stored on Shannon’s Pi server. Then Django instructs Twilio to send an MMS with the photo of Shannon’s pretty bird back to Shannon. This all takes place in the span of a few seconds.
Shannon usually doesn’t do this. She normally ships civic minded hacks. Hacks that help women learn to code, recommend movies that pass the Bechdel test, or out politicians whose ideals are quite behind the times. “This hack is just for fun,” says Shannon.
If you want to engineer a little fun and a whole lot more pet pictures into your life, here’s the code Shannon used to build BudgieCam on GitHub. Take a look at the Twilio integration below, and the MMS docs that will give you a good foothold in tackling project like this.
Learn more about Shannon and all of the rad hacks she builds right here.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.