Create AI-Generated Valentine's Day Poems with Anthropic, SendGrid, Replicate, Exa, and Replit
Time to read: 4 minutes
Create AI-Generated Valentine's Day Poems with Anthropic, SendGrid, Replicate, Exa, and Replit
I've been so busy leading up to Valentine's Day, I've had no time to write florid poetry (as I usually do). I decided to use AI to help me generate poetry via a Streamlit web app using Anthropic , Twilio SendGrid , Exa , and Replicate in Python.
Test it out here on Replit or modify the code directly on Replit here !
Prerequisites
Before you can join me in penning poetry with AI, you’ll need to have a few things in place. Register (or log into) some accounts and meet me back here.
- Twilio SendGrid account to send an email - make an account here and make an API key here
- An email address to test out this project
- A Replit account for hosting the application – make an account here
- Anthropic account to use their Completions API - get on the waitlist to use their APIs here so you can make your API key her e
- Exa account to crawl the web via an API - make an account here and grab an API key here
- Replicate account to use their hosted LlaMA 2 and Stable Diffusion models – make a Replicate account here and grab an API key here
Get Started with Replit
Log in to Replit , then click + Create Repl to make a new Repl. A Repl, short for – or maybe based on – a "read-eval-print loop", is an interactive programming environment that lets you write and execute code in real-time.
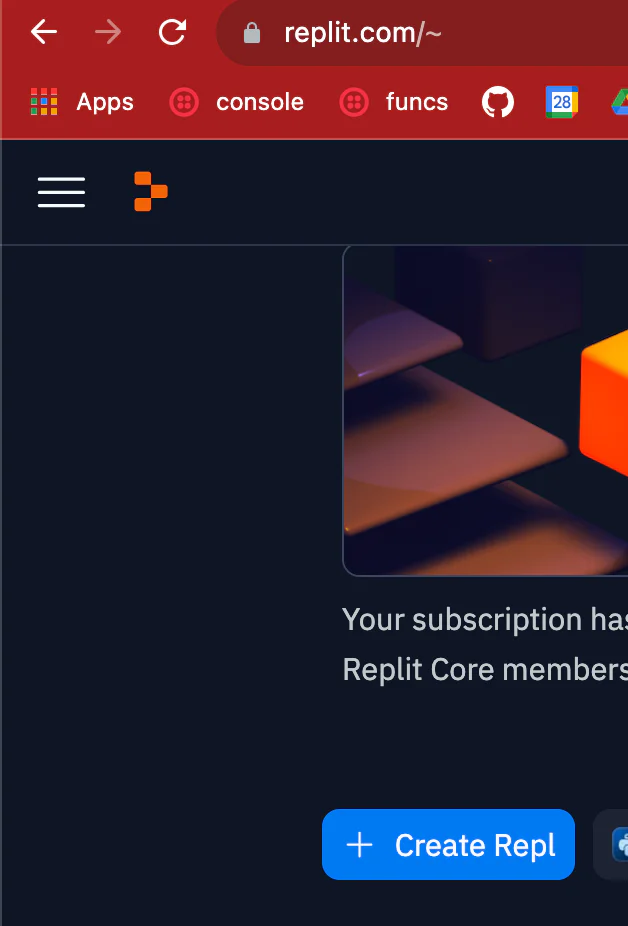
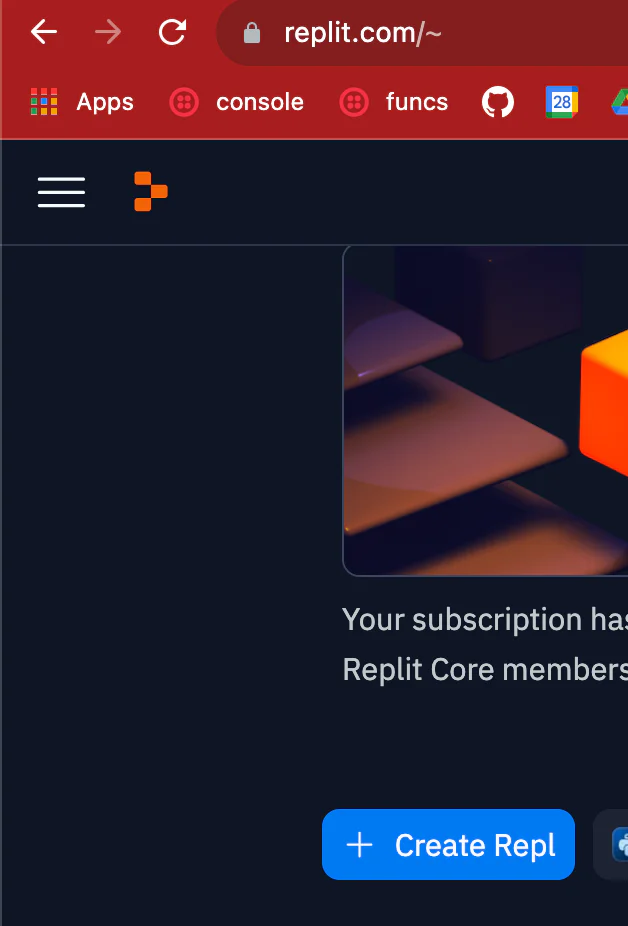
Next, select a Python template, give your Repl a title like stephensmithify-openai-vision, set the privacy, and click + Create Repl.
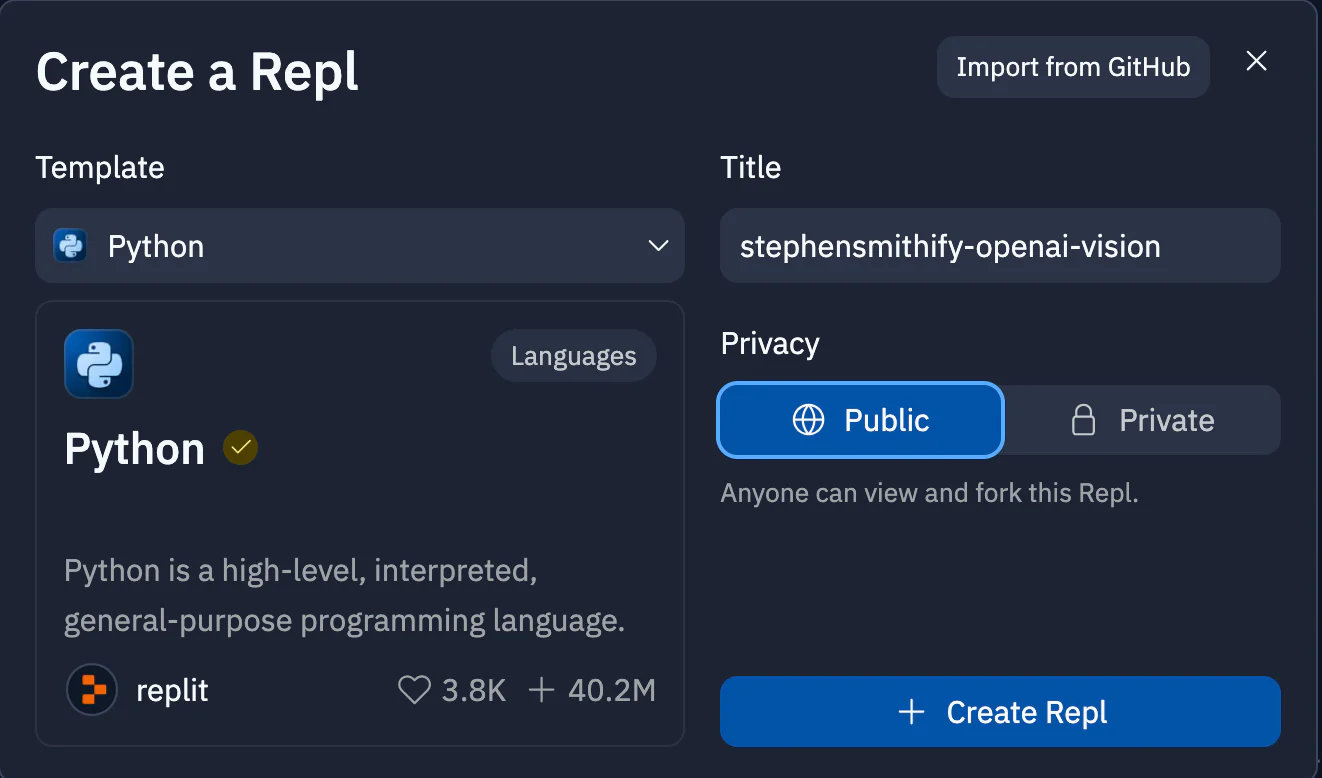
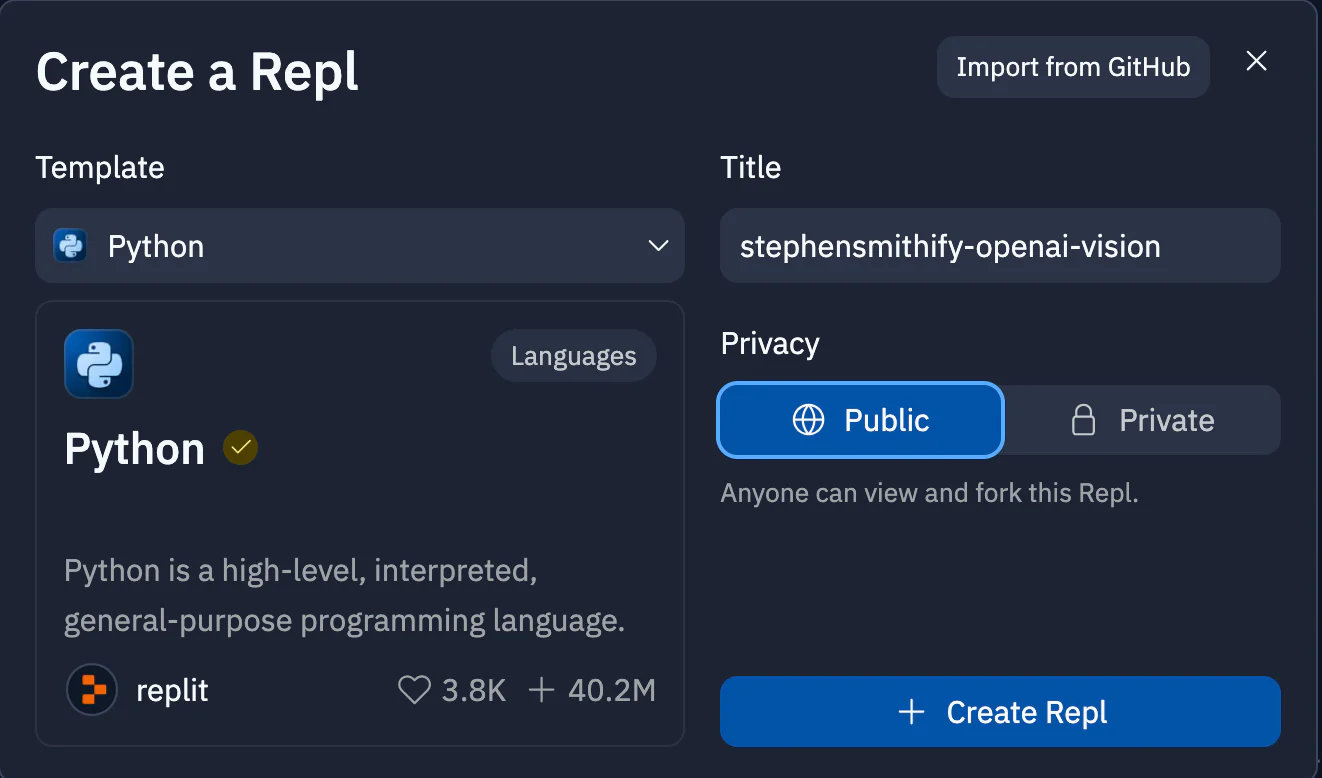
Under Tools of your new Repl, scroll and click on Secrets.
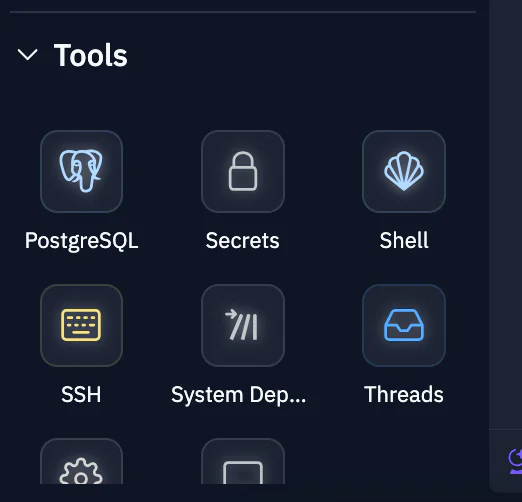
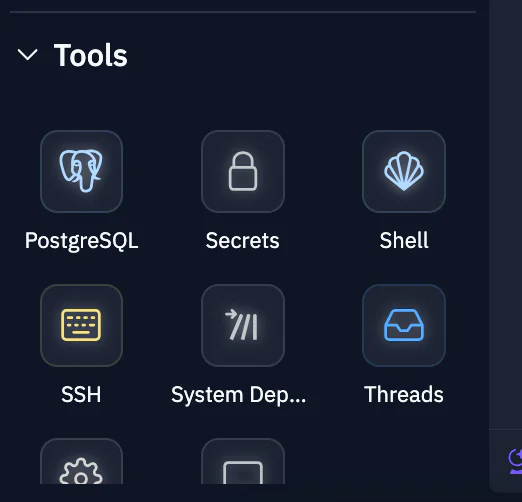
Click the blue + New Secret button on the right.
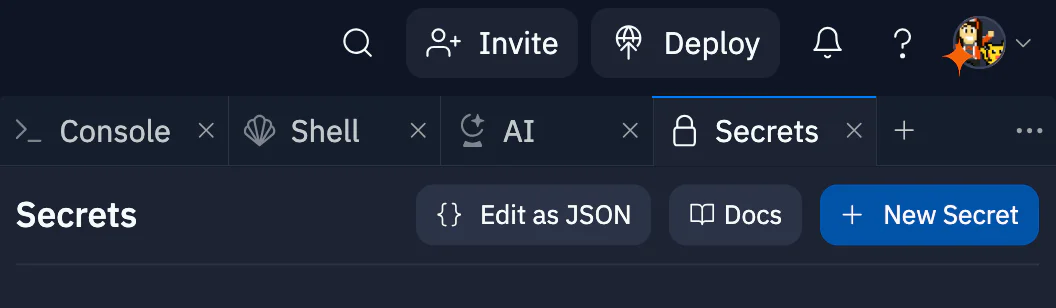
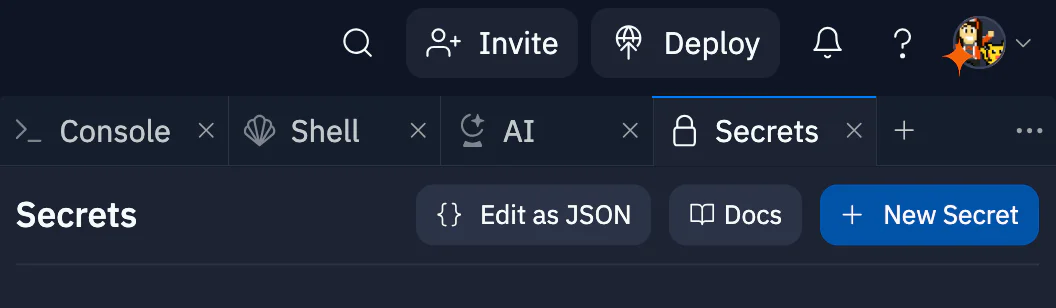
Add three secrets, where the keys are titled ANTHROPIC_API_KEY, SENDGRID_API_KEY, EXA_API_KEY, and REPLICATE_API_TOKEN and their corresponding values are those API keys. Keep them hidden!
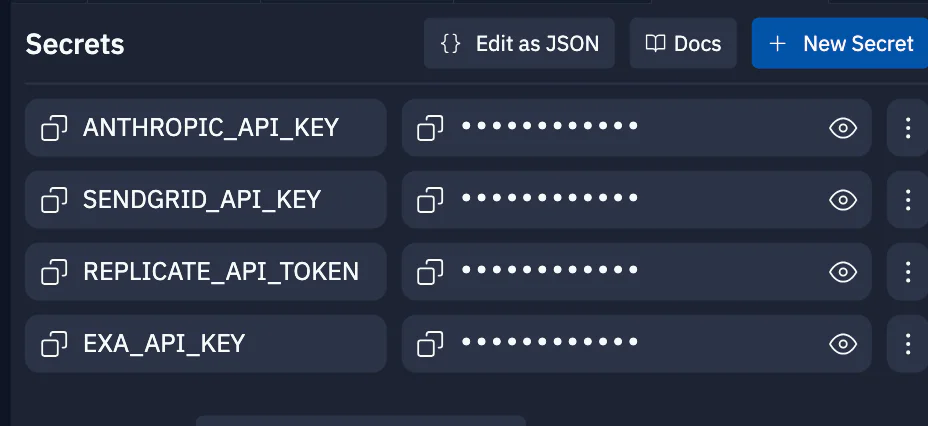
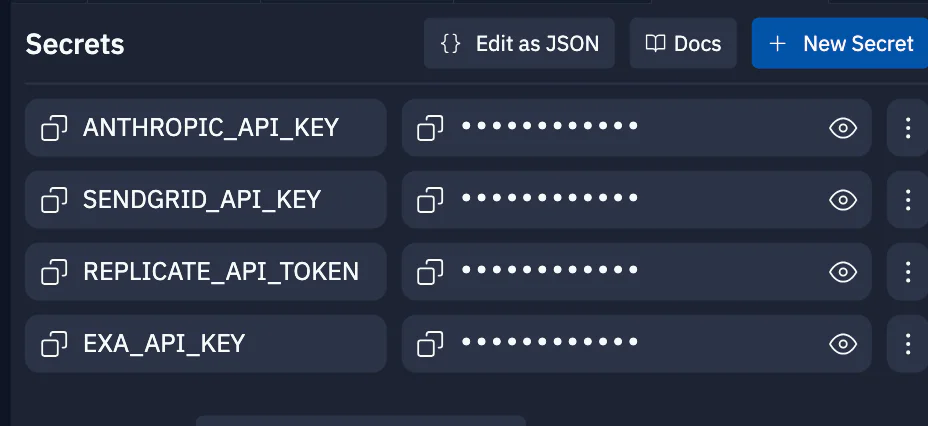
Back under Tools, select Packages.
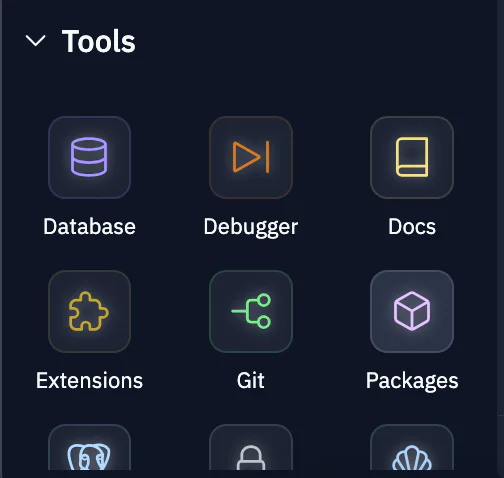
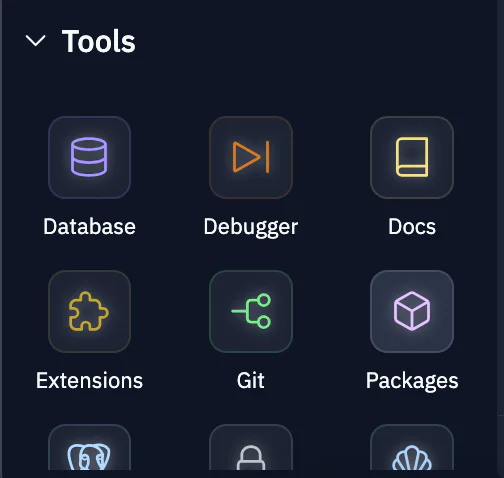
Search and add the following:
- anthropic@0.15.1
- replicate@0.23.1
- exa-py@1.0.8
- re101@0.4.0
- streamlit@1.31.0
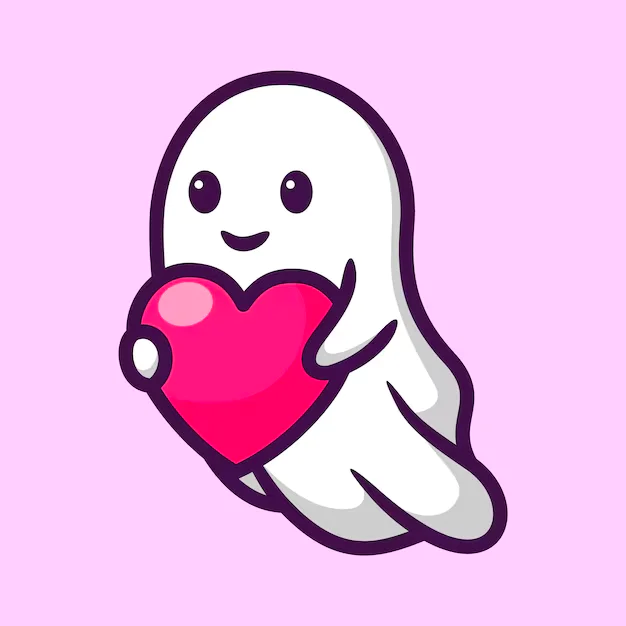
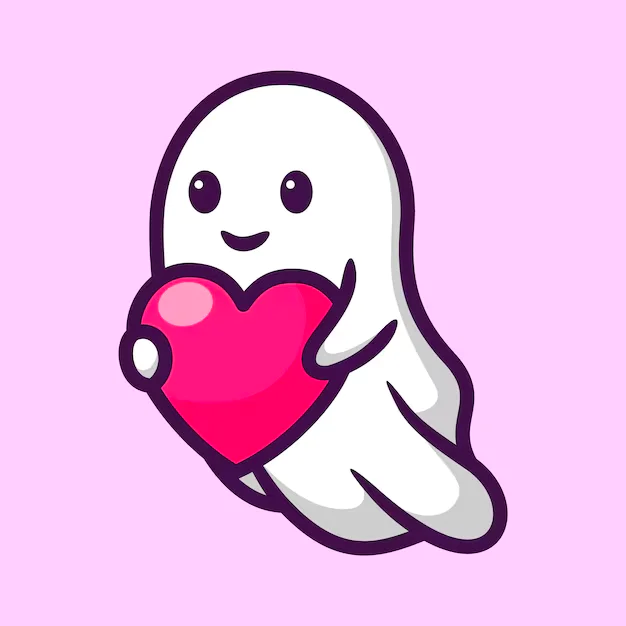
Upload it by selecting the three dots next to Files and then clicking the Upload file button, as shown below.
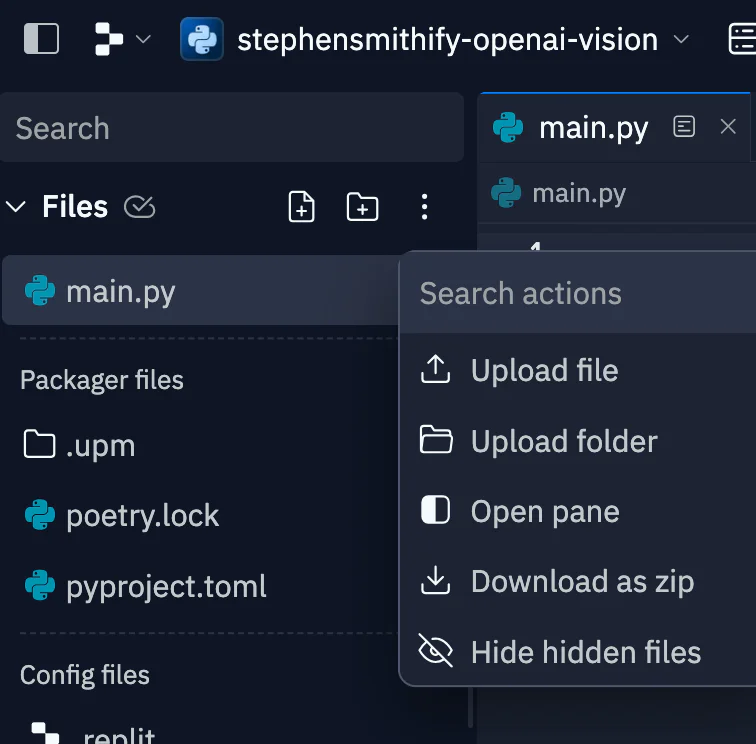
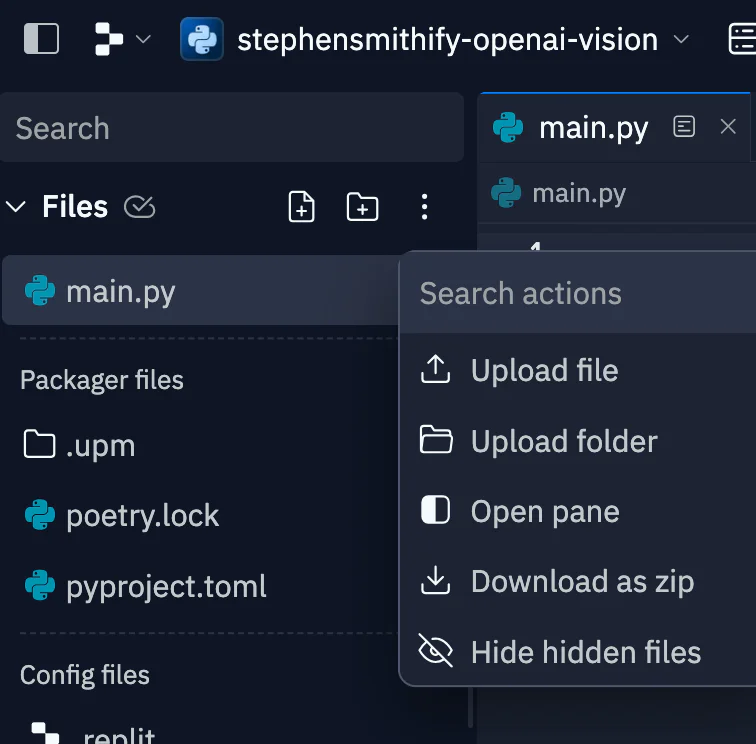
Next, add a new folder called style.
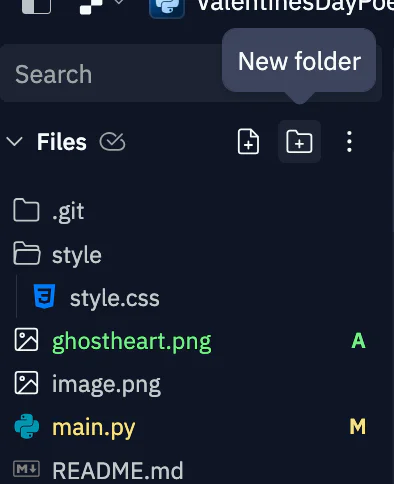
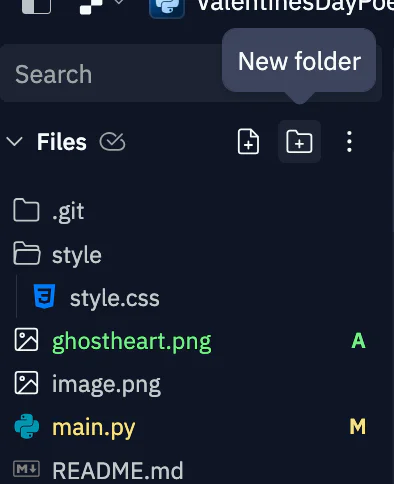
In it, add a new file style.css containing the following code:
Lastly, open the .replit file and replace it with the following so whenever the app is run, `streamlit run` is also run.
Now we're going to write some Python code to generate poetry with Anthropic's Claude model along with a personalized image with a Replicate-hosted Stable Diffusion model!
Create a Streamlit App to Receive User Input
At the top of the main.py file in Replit, add the following import statements:
Next, add the CSS styling to our Streamlit web app and create variables for our API Keys which are hidden as Replit's Secrets.
Now, create a `main()` function containing the app title and images, plus text boxes and radio buttons to receive user input. The app asks for the poem receiver name, a slider to gauge how much the user likes the receiver, what the receiver is like, what LLM to use, some optional customizable add-ons like a movie quote or lyric, the receiver’s astrology sign, and an email address to send the poem to. After all that, we create two empty variables for the poem and the gift suggestion ideas.
Generate Personal Gift Ideas with Exa
The next code runs if all of the user input fields are filled and a button is clicked. A Streamlit spinner object is displayed to let the user know the next steps could take some time.
We then make an Exa search, asking it to return three gift ideas for someone based on their astrology sign and how they were described above. You can read more about searching with the Exa API here.
The Exa output is cleaned up with regex and we make a COPY_PROMPT variable to modify pronouns in input text.
Make an AI-Generated Poem with Anthropic's Claude Model
If the user selected the Claude model, we generate a poem using Claude's completions API as shown below. You can read more about using Anthropic's Claude's Completions here.
If the user selected using the LLaMA 2 model, the following code would be run instead. You can read more about using LLaMA 2 hosted on Replicate here.
To accompany the AI-generated poem, create an image with Stable Diffusion.
You can view the generated image at the URL printed out.
Send the generated poem and image as an email with SendGrid
Now, what should you do with this poem and image? Send them via email using SendGrid in Python with the code below, of course!
The complete code can be found here on GitHub.
What's Next for Anthropic, SendGrid, Replicate, and Replit?
So much fun can be had with AI and LLMs! Models like Anthropic's Claude let developers create personalized stories, poems, and more–and it's wild how the better your prompt is, the better the output can be. Model hosts such as Replicate make it straightforward to use a variety of models such as Stable Diffusion. Besides generating images, you can also generate videos or edit images!
Let me know online what you're building with AI–check out these related Twilio tutorials to Generate an AI Competition Report with Exa, Anthropic, and Twilio SendGrid and Create an AI Commentator with GPT-4V, OpenAI TTS, Replit, LangChain, and SendGrid!
Twitter: @lizziepika
GitHub: elizabethsiegle
Replit: @LizzieSiegle
Email: lsiegle@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.