ETA Notifications with Java and Servlets
Time to read: 2 minutes
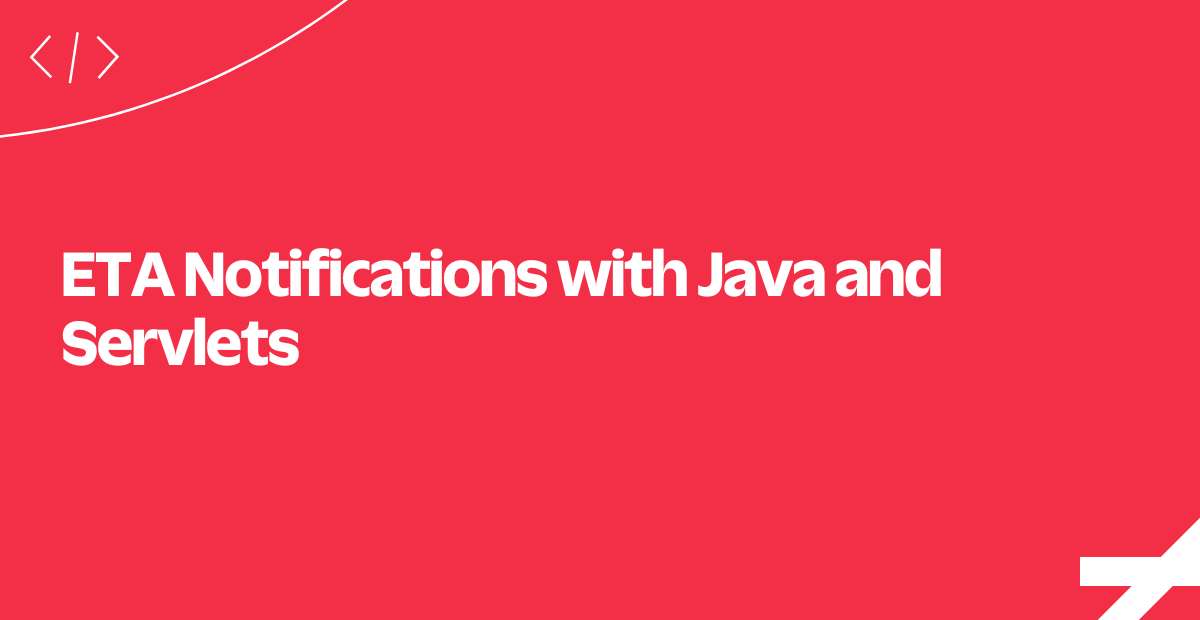
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly wherever we are. The key to those services working? Notifying customers when things change.
In this tutorial, we'll build a notification system for a fake on-demand laundry service Laundr.io using Java and Servlets.
Let's get started!
Trigger a Customer Notification
A driver's screen shows two buttons that allow the laundry delivery person to trigger notifications: one for picking up orders and one for delivering them.
This means we'll have two cases to handle:
- Delivery person picks up laundry to be delivered (
/pickup
) - Delivery person is arriving at the customer's house (
/deliver
)
In a production app we would probably trigger the second notification when the delivery person was physically near the customer, using GPS.
(In this case we'll just use a button.)
Let's look at how to use the Twilio REST API Client to send out a notification.
Set up the Twilio REST Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
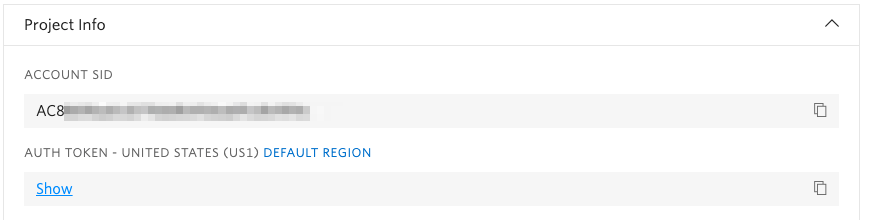
Next up, we'll look at how we handle a notification request.
Handle the Notification Trigger
This code handles the HTTP POST
request triggered by the delivery person.
It uses our MessageSender
class to send an SMS message to the customer's phone number, which we have registered in our database. Easy!
Next let's look closer at how we send the SMS.
Sending the Message
Here we demonstrate actually sending an SMS or MMS.
Think it needs a picture of the clothes? Good idea.
You can pass along an optional media URL:
.setMediaUrl("http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg")
In addition to the required parameters (and optional media), we can pass a StatusCallback
url to let us know if the message was delivered.
Message status updates are interesting - let's take a closer look.
Handle a Twilio Message Status Callback
Twilio will make a POST
request to this servlet each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notificationStatus
on the Order
and let the business logic take over. This is a great place to add logic that would resend the message if it failed or send out an automated survey a few minutes after the customer has clothes delivered.
That's a wrap - and a fold! We've just implemented an on-demand notification service that alerts our customers when their order is picked up or arriving.
Next, let's look at some other easy to integrate features.
Where to next?
Java and Twilio go well together! Here are some other Java Servlets tutorials:
Increase your rate of response by automating the workflows that are key to your business. In this tutorial, learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand so two users can communicate without exchanging personal information.
Did this help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.