ETA Notifications with Python and Flask
Time to read: 2 minutes
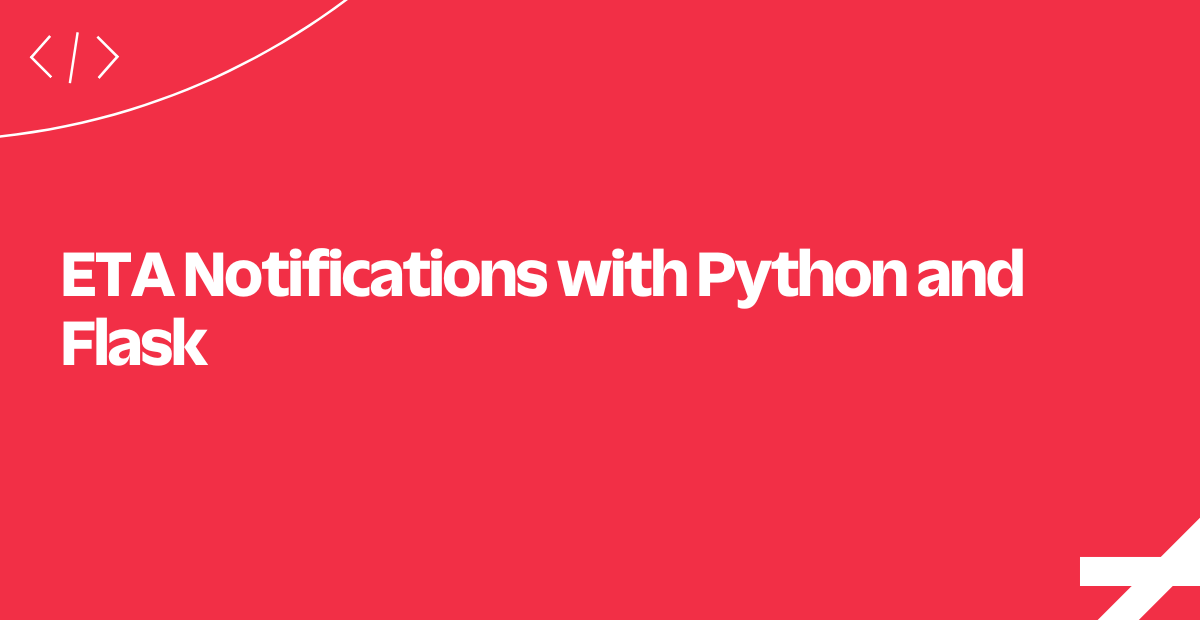
There are now many successful businesses that deliver goods or services to their customers on-demand.
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly, wherever we are. The key to those services working is notifying customers instantly when things change; customers need to know if there's a last second audible.
In this tutorial we'll build a notification system for our made-up on demand laundry service Laundr.io, and we'll show you how to implement ETA Notifications with Python and Flask.
Let's get started!
Trigger Notifications
Here we show a couple of routes which will be exercised by our delivery person. There are two cases we'd like to handle:
- Delivery person picks up laundry to be delivered (
/pickup
) - Delivery person is arriving at the customer's house (
/deliver
)
In a production app we would probably automatically trigger the second notification with GPS, but a button should show you the basics of how it's done.
Now that you've seen the views, let's look at the Twilio REST Client, which we'll use to send the notifications themselves.
Set up the Twilio REST Client
We instantiate a Twilio REST client with our Twilio Account Credentials stored as environment variables.
You'll need a number you own through Twilio: TWILIO_NUMBER
, as well as an Account SID and Auth Token: TWILIO_ACCOUNT_SID
and AUTH_TOKEN
. These can be found in the Twilio Console.
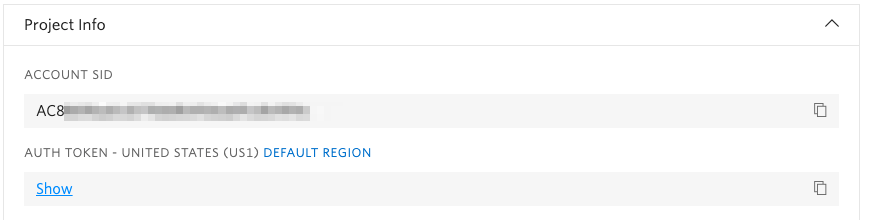
Next up, let's look at what happens when a notification is triggered.
Handle a Notification Trigger
When a notification is required, we extract the phone number stored in each order and then simply send an SMS message with an appropriate body. Easy!
Next, let's look closer at how we send the SMS.
Send the Message Out
This code shows how we actually send the SMS out to the customer.
Think it would be better with an image? You're probably right - you can add an optional media_url
to make it an MMS:
media_url='http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg'
In addition to the required parameters (and the optional media_url
), we can pass a status_callback
url to let us know if the message was delivered.
The status update handler is interesting - let's zoom in on that next.
Handle a Twilio Status Callback
Twilio will make a post request each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notification_status
on the Order
so that we can decide what to do next with the Order
. This is a great place to add logic that would resend the message in the event of a failure, or send out an automated survey when the delivery message is successful.
That's it! We've just implemented an on-demand notification service that alerts our customers when their order is picked up or arriving. You should now be able to add ETA Notifications to your own application very simply.
On the next pane we'll take a look at some other tutorials you might enjoy.
Where to Next?
Like Python? Like Twilio? Try these other tutorials:
Increase your rate of response by automating the workflows that are key to your business. In this tutorial, learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand, so two users can communicate without exchanging personal information.
Did this help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.