SMS and MMS Notifications with Ruby and Rails
Time to read: 2 minutes
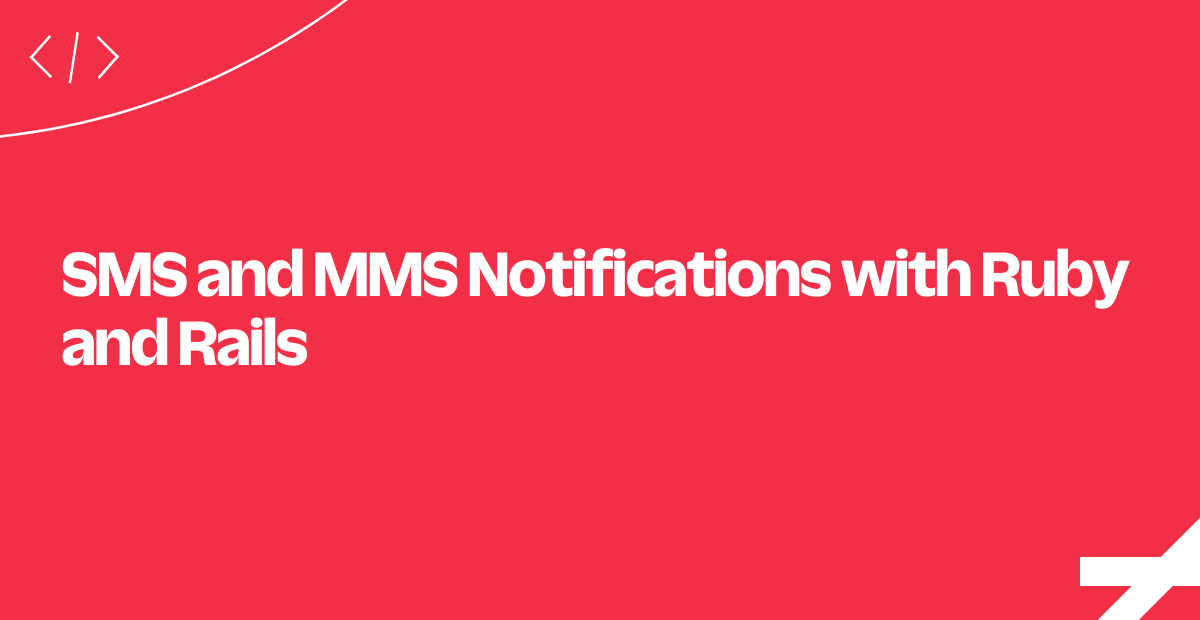
Ruby, Rails, and an automatic ping for all of your server administrators when something goes wrong? We hope you're as excited to build that application as we were when we wrote this tutorial.
We'll use Ruby on Rails and demonstrate how to send SMS (or MMS) alerts to a list of system administrators if something goes wrong on your server. Follow along as we demonstrate the key pieces of code to make it all work.
Clone our repo from Github and use the Readme to get it running in your local dev environment, then you're ready to hop in.
Let's get started!
A List of Your Server Admins... And Whomever Else as Well
In yaml, create a list of administrators who should be notified if a server error occurs.
The only essential piece of data we need is a phone_number
for each administrator.
On deck: configuring the Twilio REST Client!
Configuring the Twilio REST Client
To send a message, we'll need to initialize the Twilio REST client which requires reading our TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
from environment variables. That link shows you how to set environment variables inside *NIX, Mac OSX and Windows - but it may vary based on your choice of shell. If you are on another platform, you'll need to consult the platform documentation.
You can grab those two from inside the Twilio Console:
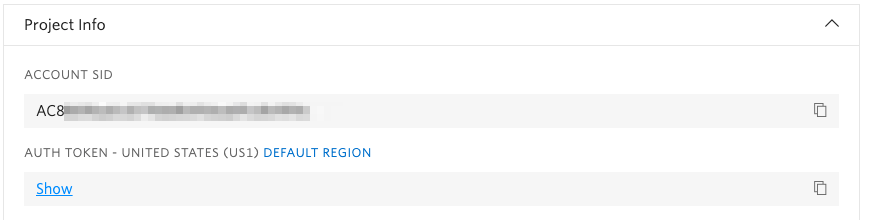
Next, let's take a look at how the application is set up to handle exceptions.
Handling Application Exceptions
We take advantage of the rescue_from
method to rescue any exception raised in controller actions. With those exceptions under control, we can send out a notification when something goes wrong - and get our administrators on the case, quickly.
Next up, let's get creative and see how to create a custom exception alert message.
Creating a Custom Alert Message
Here we create an alert message to send out via text message.
You might also decide to include a picture with your alert message. Perhaps a screenshot of the application when the crash happened? A funny gif? Kid pictures?
Let's continue, and look at loading the administrators from the yaml file.
Reading the Administrators from the YAML File
Next, we read the admins from our YAML file and send alert messages to each of them with the private send_message
method.
Now you've seen the wiring behind the messages, let's deep dive into how we'll send them.
Sending a Text Message
There are the three parameters needed to send an SMS using the Twilio REST API: from
, to
, and body
.
After the message is sent, we print out the phone number we're texting.
US and Canadian phone numbers can also send an image with the message as an MMS (uncomment media_url
). The rest of the world will have image links with automatically shortened links.
And it's as simple as that! You've now got a Ruby on Rails server application which uses the server to notify your server administrators when something goes wrong with the... server.
What else can you do with Twilio? Let's look into it on the next pane...
Where to Next?
If you're a Ruby developer working with Twilio, you might want to check out these other tutorials.
Increase the security of your login system by verifying a user using his or her mobile phone in addition to a password.
Customers missing things scheduled months in advance? Build an appointment reminder application with Twilio and reduce the number of no-shows.
Did this help?
Thanks for checking out this tutorial! Tweet @twilio to let us know what you think, and keep us posted on what you're going to build next!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.