How to Receive a POST Request in Node.js
Time to read: 4 minutes
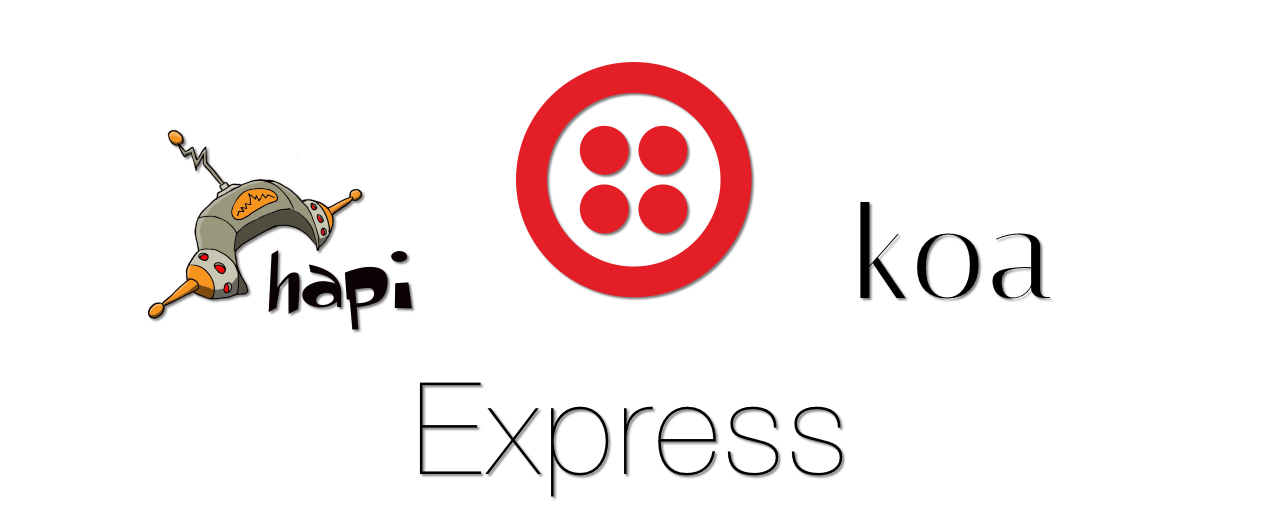
Receiving a POST request is the “Hello, World” v2 of building a web app. Here’s how to receive a POST request with three popular Node.js frameworks – Express, Hapi, and Koa.
Let’s build an app that receives an inbound SMS webhook from Twilio. When a user texts your Twilio phone number, Twilio will make a POST request to the URL that you configure with all the bits of information you care about. Normally you’d want to reply with TwiML, but for simplicity we can reply with an SMS in plain text if we set the Content-Type
header as text/plain
.
What We’ll Need
- Node.js – follow the steps to install for your platform.
- A free Twilio account and phone number.
- Ngrok – download the binary for your platform.
There’s a good chance you’ll be writing this code on your own computer which poses an issue. Your computer can’t receive incoming HTTP requests by design.
Thankfully, Ngrok lets you expose your local development server to Twilio so it can receive HTTP requests. If you haven’t used Ngrok before, my buddy Phil has a great post about why you’ll love using it to test webhooks.
Start Ngrok for HTTP on the port we’ll be using for our servers:
Configure your Twilio phone number to POST incoming messages to http://YOUR_NGROK_URL.ngrok.io/sms.
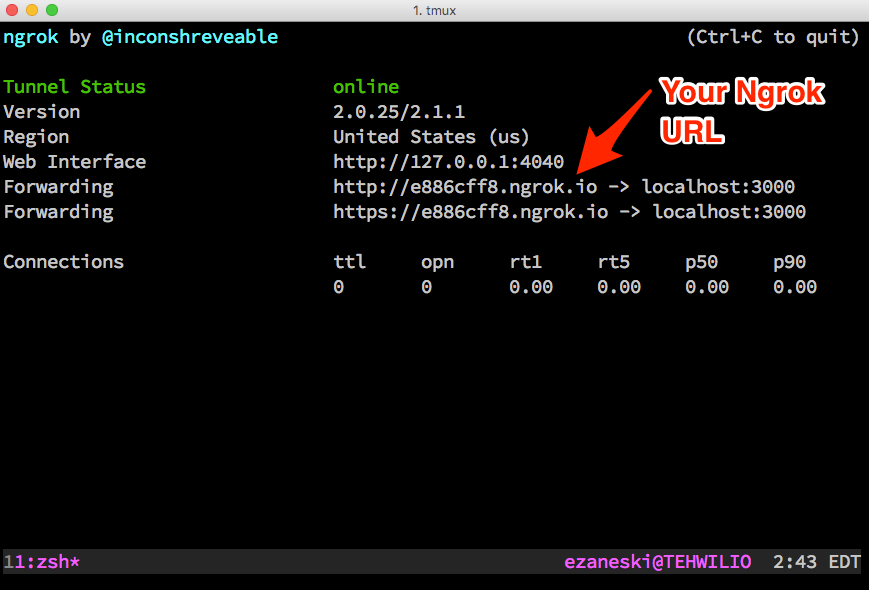
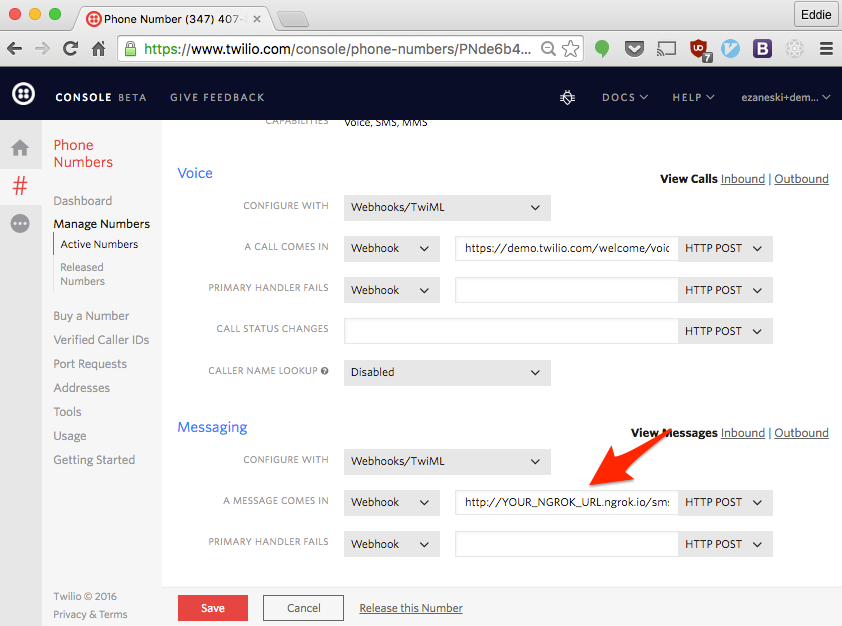
Make sure to keep Ngrok running throughout the post. If you restart it, your generated URL will change and need to be updated in your phone number configuration.
Express
We’ll start with my favorite web framework for Node.js – Express.
Express makes heavy use of middleware — functions that get called on each inbound request. Routing is done through a simple yet powerful interface that passes a request
and response
into a callback function.
We need to install the express library along with the body-parser middleware to handle parsing the body of the POST request. Install the dependencies into your project directory:
Paste the following into a file named express-post.js
to create a simple web server that responds to a POST request at /sms
.
Start the server and send a text message to your Twilio phone number.
Take a moment to leap to your feet and boogie for receiving your first POST request in Node.js.
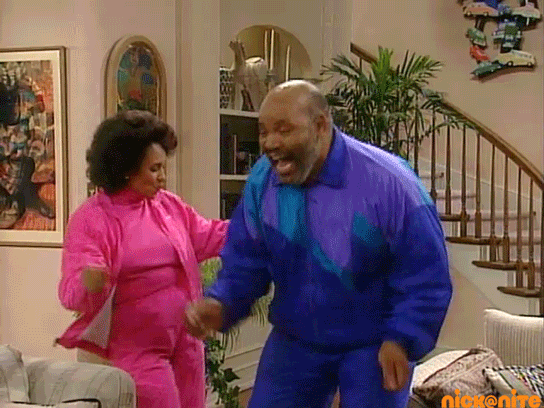
Hapi
Hapi was originally developed at Walmart Labs and is well known for keeping the servers “bored out of their mind” during Black Friday 2013.
Hapi embraces configuration and includes an enormous amount of functionality out of the box like parsing requests. Routing in Hapi is done through clear config objects with handler functions. Like Express, it passes a request
and a reply
.
Install the hapi
dependency by running:
Paste the following into a file named hapi-post.js
Blast off with our Hapi server and shoot a text to your Twilio phone number:
Seriously, if you didn’t get up before jump to your feet now and groove.
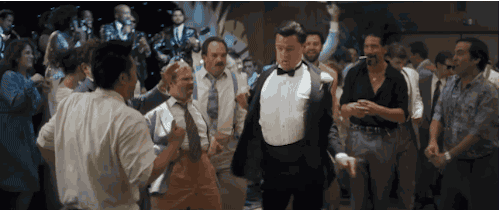
Koa
Koa has a bit of a cultish following and is actually second of the three frameworks on monthly downloads. It’s a super lightweight library that doesn’t even have a router out of the box. Koa is for developers who want total control without decisions being made for them.
The framework was originally based off of generator functions but the latest version (2.0.0) uses async
functions. We’ll be avoiding these for now but check out this blog post to learn more about async/await in JavaScript.
Koa uses middleware for route handlers and are called on every request with a context (ctx
) passed in. The context contains everything needed to interact with a request and send back a response. Like Express, we need to pull in a middleware to parse the body of the request. Install the dependencies with:
Then paste the following into a file named koa-post.js
:
Fire up our final server and send a text message to your Twilio phone number:
This is your last chance to get up. Just do it.
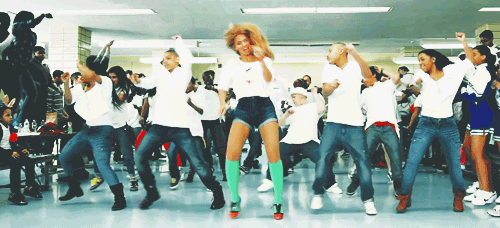
Wrap Up
We’ve seen how to receive a POST request in three popular Node.js frameworks and have hopefully done a bit of dancing. Of the three frameworks Express has always been my go-to but I plan on experimenting with Hapi and Koa further.
The next thing you should do with your newly built web apps is use the Twilio Node.js library to create some TwiML to respond to an incoming phone call.
You should also check out the docs on Express, Hapi, and Koa to take full advantage of all their features.
Be sure to let me know which one is your favorite and why.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.