Writing a bot for Programmable Chat in Node.js
Time to read: 3 minutes
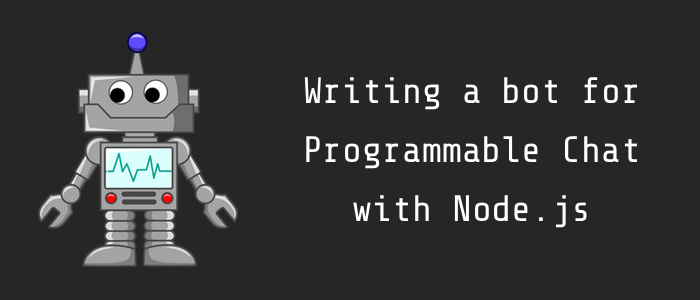
It seems like bots are the new hot thing that every chat supports. They usually augment conversations or they can perform tasks for the user. We will add to an existing Programmable Chat chat a simple bot that will return us a GIF whenever we ask for it.
Getting Your Initial Chat Up And Running
We will be working off an existing chat application. Before we get started with our bot we need to get a couple of things ready.
First of all, make sure you have:
- Node.js and npm which you can get here
- A Twilio Account to set up Programmable Chat – Sign up for free
- ngrok – You can read more about ngrok here
The existing chat application that we will base our work off is this quickstart project. It only takes 5 minutes to set this project up. Clone the repository by running:
Configure it according to the README.
Intercepting Messages
Twilio Programmable Chat will handle the sending of chat messages from client to client. The product also has webhook notifications for when new messages in our chat are sent. Using these webhooks we can control whether a message should be delivered or blocked. All this can be done simply by returning different HTTP status codes. Status code 200 (OK
) means continue delivering this message and 403 (Forbidden
) means block this message.
Before we decide whether to block or forward a message we will need to access the data that Twilio sends to our application. To do that we first need to parse it first by adding body-parser
to our project using npm:
Once the package is installed add it to index.js
:
Add a very basic endpoint to index.js
that will print the data received and return the status code 200 to tell Twilio to continue with delivering the message:
Restart the server by running:
We need to expose our application to the public so Twilio can deliver the webhook. For this execute in a different terminal:
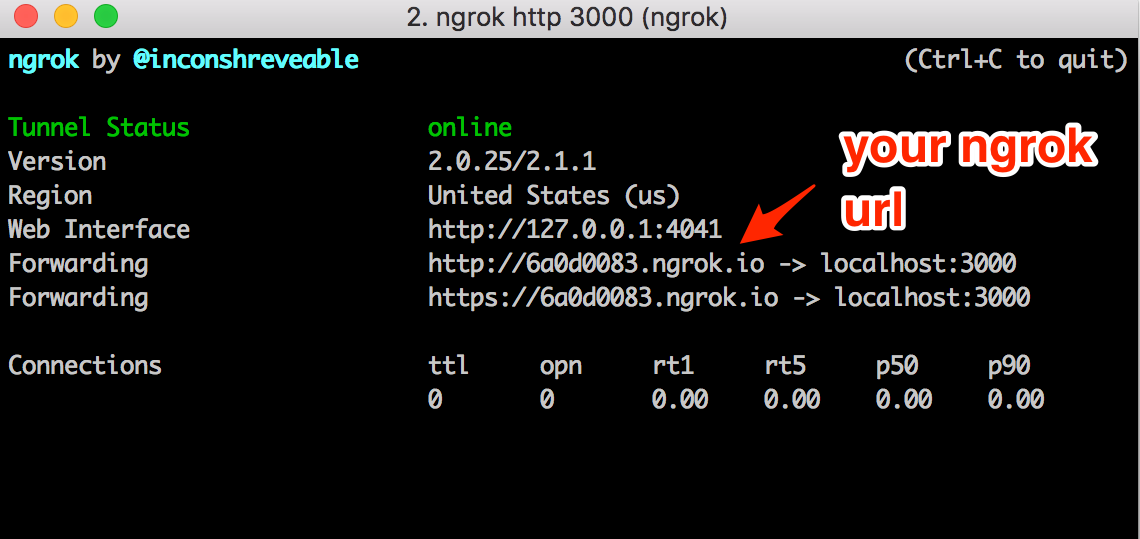
Afterwards go to your messaging service in the console and configure the webhook URL as:
http://.ngrok.io/message
.Make sure that the HTTP method is
POST
and that OnMessageSend
is activated.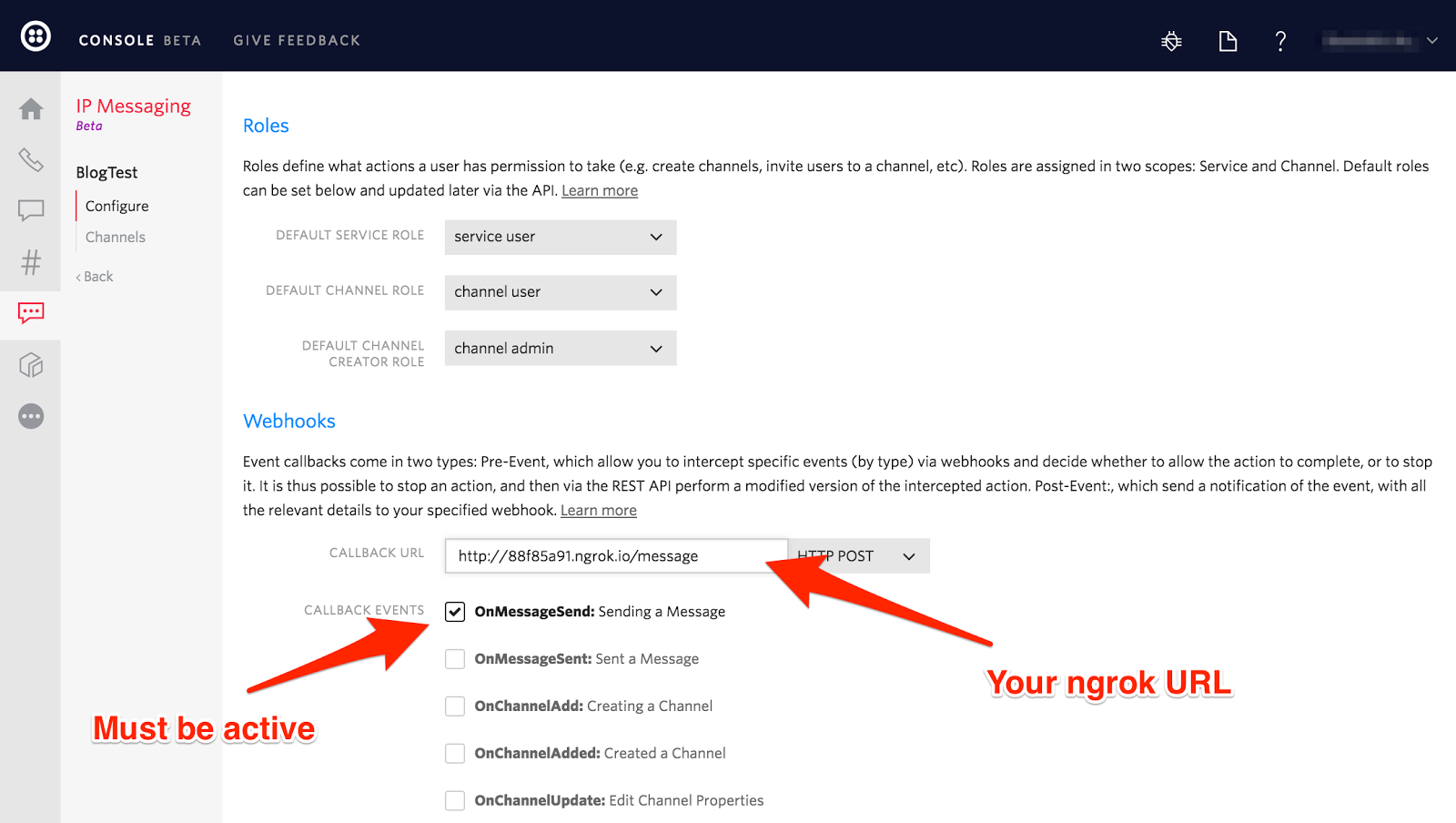
Navigate to http://localhost:3000 and start writing messages. You should see them start appearing on in your terminal with additional information for the message.
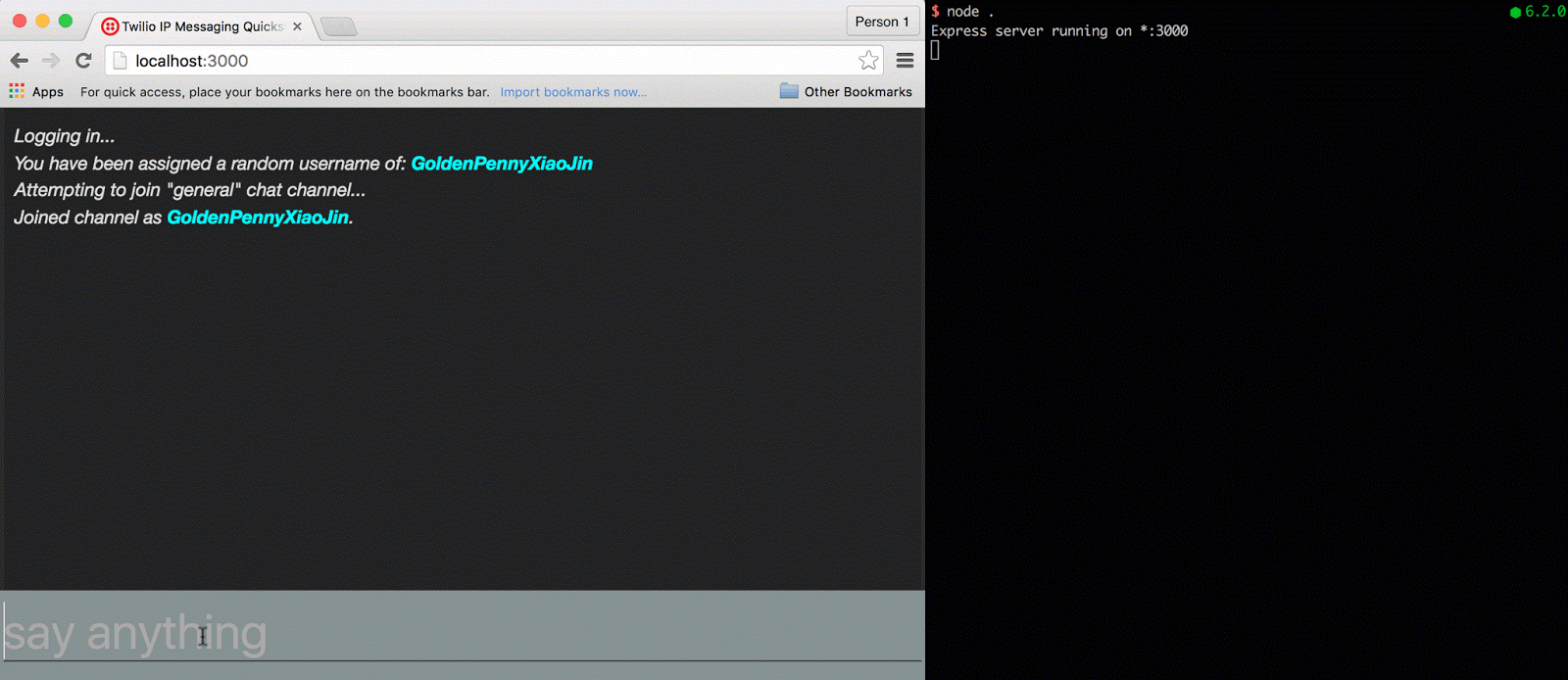
Controlling The Conversation
Now that you are receiving all the necessary information, we can dive deeper into the control that these webhooks provide. If we return the status code 403 instead of 200 we can block the respective message. Let’s alter the route to intercept and not deliver messages that start with /gif
:
Restart your server and send a message with /gif
. You should see that it’s not showing up. All other messages will normally appear.
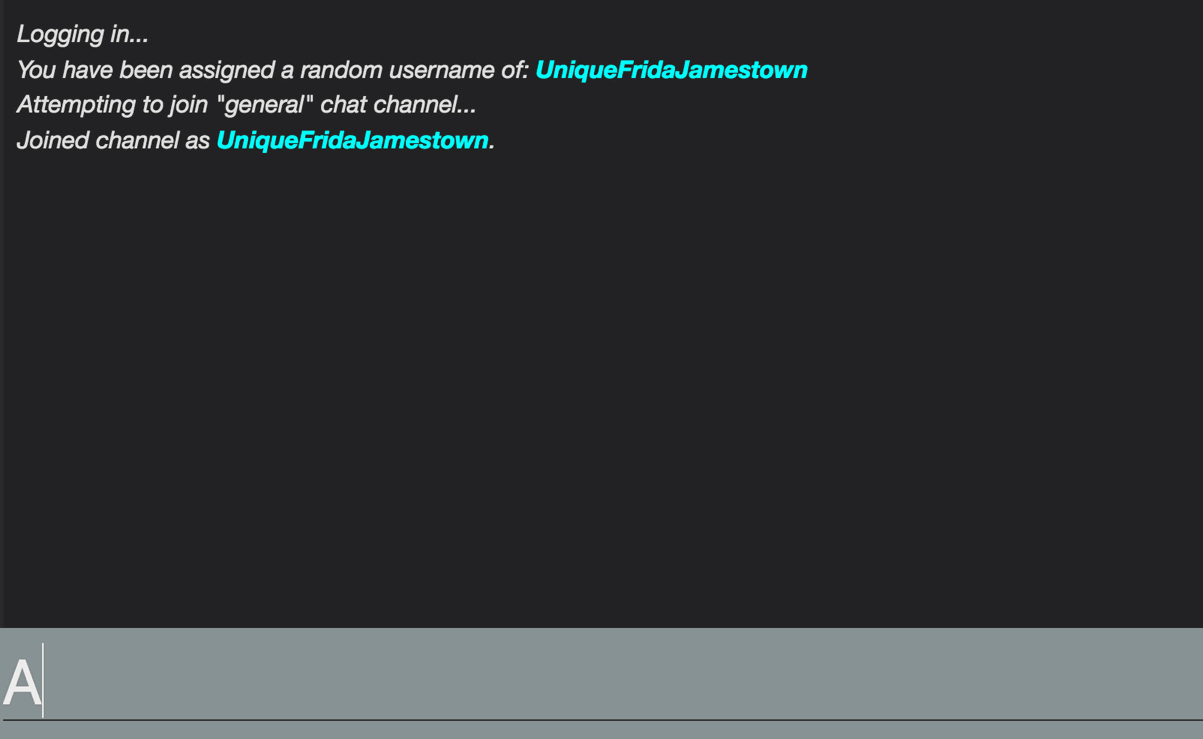
Let The Bots Join!
So far we can intercept the content that is being sent but we want to actually send our own messages. We want our bot to actually participate in the chat. For this we will forward the message at all times and then use the information about the channel to place the bot in the channel and write a message to it using the Twilio helper library.
Modify the index.js
file accordingly:
Once you restarted the server try writing /gif
in the chat again. Now you should see the bot write the actual message and your message staying preserved.
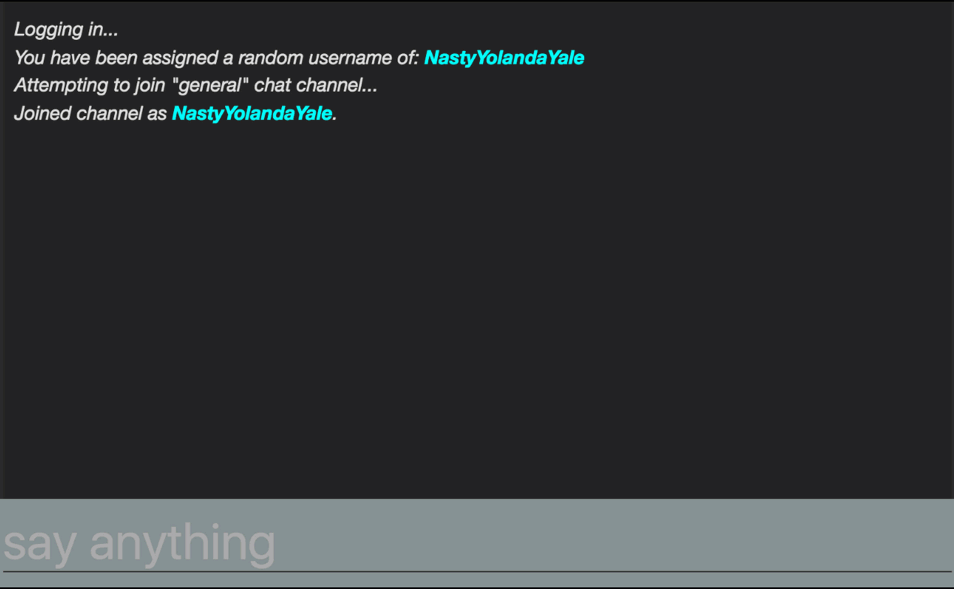
Upgrade Your Bot
So far we are only sending text with our bot. We can use the new message attributes to add additional contextual information to our message, such as information that this is actually a picture.
For this send an object as attributes that specifies the type as 'image'
when creating the message:
Now that we are sending this information we need to update our front-end to display the image. Open public/index.js
and update the printMessage
method as well as the event listener for messageAdded
to wrap an image-tag around the content:
Now restart the server and reload your browser and you should be able to see the GIF properly appear the next time you write /gif
!
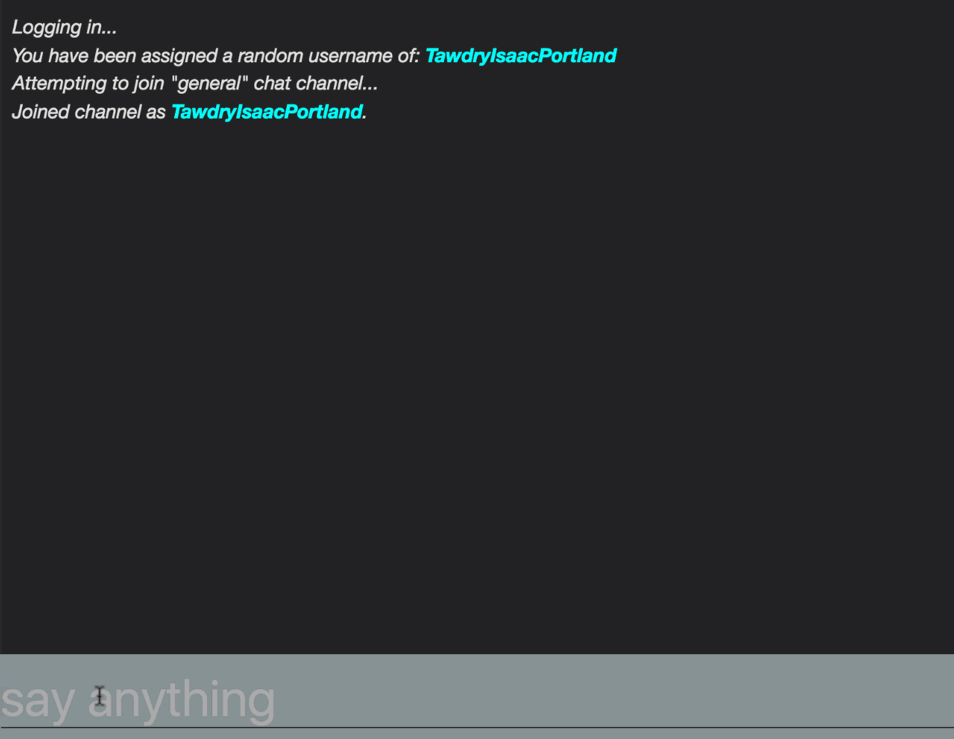
Rise Of The Bots
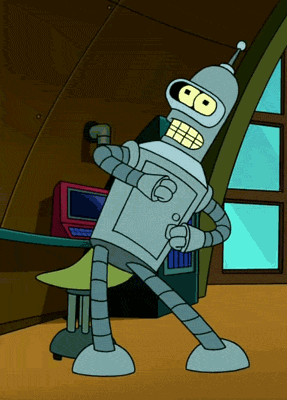
Amazing! You just finished your first bot!
But you don’t have to stop here. You could extend the bot to return a random GIF using the Giphy API or come up with your very own bots. How about a bot that recommends a restaurant whenever you talk with your colleagues about lunch? Now that you have the tools you are only limited by your imagination!
Let me know what you came up with by sending me a message or tell me in person at SIGNAL London. You can use DKUNDEL20 to get 20% off.
We can’t wait to see what you build!
- Email: dkundel@twilio.com
- Twitter: @dkundel
- GitHub: dkundel
- Twitch (streaming live code): dkundel
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.