Build Your Own API Analytics in Vue.js with PHP, Laravel, and Twilio Sync
Time to read: 5 minutes
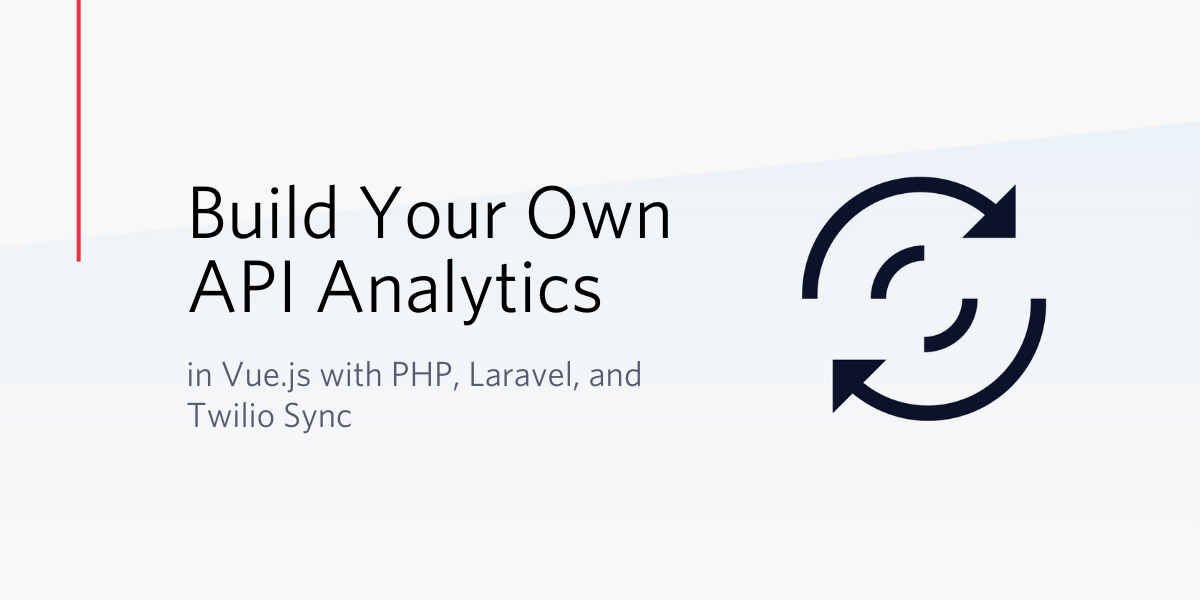
Twilio Sync is a service provided by Twilio that helps you keep your application state in sync across different services. Specifically, it provides a “stack-agnostic” API that helps you add real-time capabilities to your application.
In this article, we will explore how we can build a custom real-time API analytics tool powered by Sync Lists, a Sync primitive type that helps us synchronize individual JSON objects.
NOTE: You can learn more about Sync Lists and other primitives from the Twilio Sync Object Overview.
Prerequisites
To complete this tutorial you will need the following:
- Laravel CLI, Composer, and npm installed on your computer
- Basic knowledge of Laravel
- Basic knowledge of Vue.js
- A Twilio Account
- cURL or Postman installed to test our API endpoints
Creating the Sample Application
We will generate a fresh application with the Laravel installer and enter into the project directory with:
Next, install the Twilio SDK for PHP with the command below:
Now, create a new Sync service in your Twilio console and populate your .env
file with the generated service SID, together with your Twilio API credentials as follows:
Also, remember to update your database credentials in the same file to match your local setup.
Models and Migrations
Our application will contain two models; a Recipe and Hit. They will both be used in setting the conditions and recording the API hits of our users, respectively. Create the Recipe model and its corresponding migration file with:
Now, open the generated recipes migration file and make the following changes:
Our recipes need to contain formatted texts such as lists and headers. We achieve this using Mutators in the getBodyAttribute
method below i.e, the body string is stored in markdown and transformed into HTML using the CommonMark parser which comes bundled with Laravel. Replace the content of the Recipe model class, located at app/Recipe.php
with the following:
Similarly, generate the Hit model and it’s migration file with: php artisan make:model Hit -m
and paste in the following in the migration file that Laravel generates for us:
Now update the $fillable
attribute of the generated Hit
model to the code below:
Apply the migrations we have created with the artisan command:
Seeding our Database
To try things out, we need some dummy data in our recipe table. Create the seeder file by running php artisan make:seed RecipesTableSeeder
from your terminal. Next, replace the content of the RecipesTableSeeder
file at database/seeds/RecipesTableSeeder.php
with the following:
Next, we need to make Laravel aware of our seeder file by opening the DatabaseSeeder class file (database/seeds/DatabaseSeeder.php
) and updating the run method to the following:
Populate the database with the dummy data by running:
Building the API Controller
With our models in place, let’s create the corresponding RecipesController
class. This controller class will handle all recipe-related operations in our API. Open up your terminal and run the command below in your application directory:
This will create the Api
folder in your Controllers directory (if it doesn’t exist) and generate the RecipesController.php
there. Open the created file and add the following to it:
Using Laravel’s After Middlewares
Our core application API is ready now, but we still need to monitor all requests and responses our API is processing. We will achieve this with Laravel after middlewares. After middlewares are regular Laravel middlewares, except they perform their tasks after the request has been handled by the application. Let’s create a SaveEndpointHit middleware for this case with:
This will generate a new file at app/Http/Middleware/SaveEndpointHit.php
. Fill it with the code below:
Next, register the SaveEndpointHit
class as a route middleware by appending it to the $routeMiddleware
array in app/Http/Kernel.php
.
At this point, our controller and middlewares are ready. Let’s proceed to create our application routes. We will be putting our API related routes in routes/api.php
. That way, they’ll become accessible at APP_URL/api
instead of the regular APP_URL
. Open the API routes file (routes/api.php
) and replace its content with the code below:
In the code block above, we have attached the hit.save
middleware (which is an alias to the SaveEndpointHit class) to the recipe route resource. That way, every call to any of the endpoints triggers the middleware..
Setting up our Dashboard with Vue
We will now hook into our API from a Vue-powered dashboard, responsible for displaying the API calls. We achieve this by using the Laravel UI package which makes it easy to work with frontend libraries and frameworks like React and Vue.js. Install and configure the package by running the commands below in your terminal:
For our dashboard, we will create a new Dashboard component for viewing our API hits.
Next, replace the default example component with the dashboard component by replacing the content of the resources/js/app.js
file with the following:
Also, get rid of everything inside the resources/views/welcome.blade.php
template file and replace its content with the following:
Notice that we are passing a token
prop to our dashboard component. This token represents our Twilio Sync token which is passed down from our controller (which we will create next).
Create the controller responsible for rendering the dashboard with:
The command above creates a DashboardController.php
file in app/Http/Controllers
. Open the file and paste in the following code:
Next, register the controller in our web routes by replacing the content of routes/web.php
with the following:
Viewing API Calls in Real-time
Our controllers and Vue components are ready, but when you visit the dashboard URL at http://localhost:8000/admin/dashboard
, it renders a blank page. This is because we have no way of populating the API calls for now. We will use the Twilio Sync SDK to fetch the hits from our Sync service and prepare them for rendering when our dashboard component is created. Install the twilio-sync
package with:
Next, replace the content of the dashboard component file (resources/js/components/DashboardComponent.vue
) with the following:
Testing
We’re now ready to test the application. Your files will need to be compiled by running the following command:
The server will also need to be started in a new terminal:
Now visit (or refresh) the dashboard URL at http://localhost:8000/admin/dashboard
in your browser and make some API calls with curl like so in a separate terminal:
You should see subsequent requests being rendered in real time.
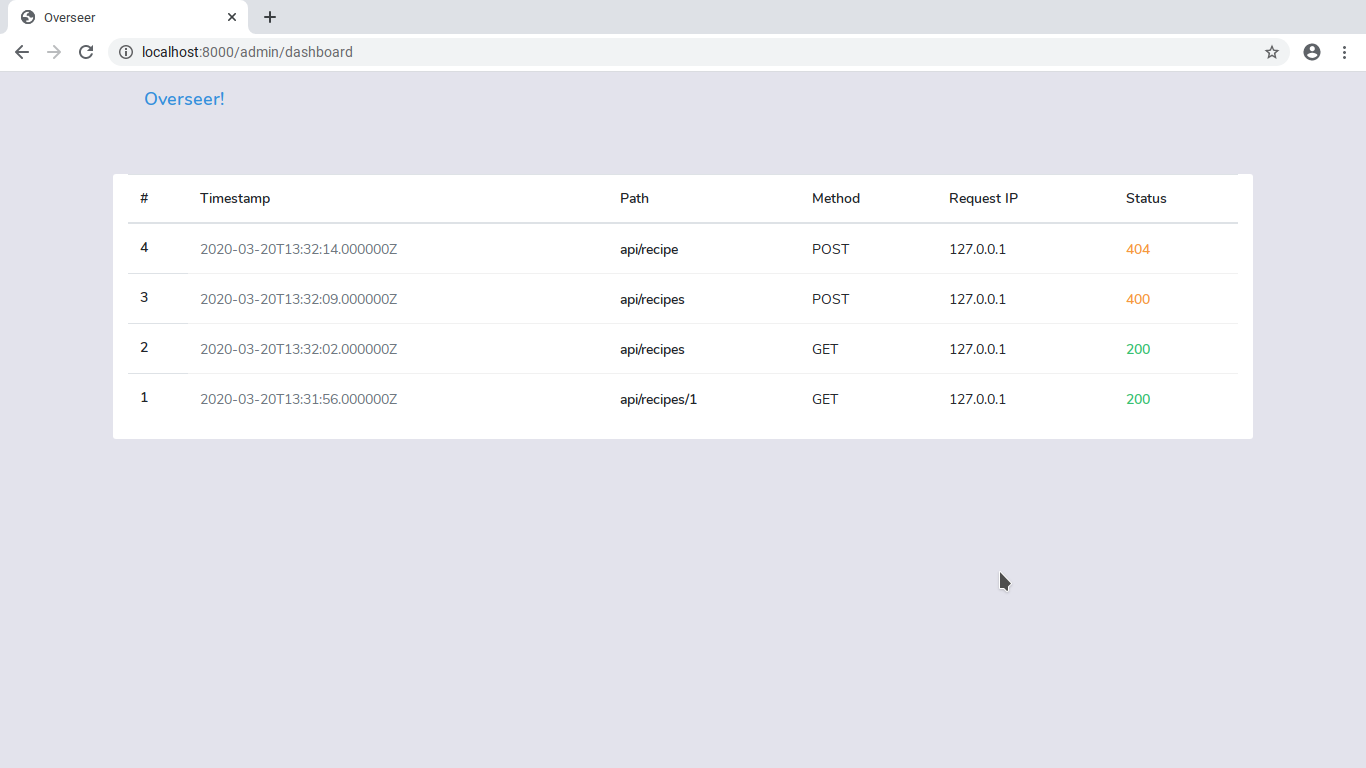
Monitoring Application Crashes
Because our middleware depends on successful responses, it fails in cases where exceptions are thrown before Laravel can finish processing the request. To handle such cases, open the exceptions handler at (app/Exceptions/Handler.php
) and create a new private method responsible for logging the errors as in the code block below:
Next, call the method above in the render
method of the Handler
class as shown:
Build your files again with npm run dev
and start your Laravel server if it isn't running already and visit http://localhost:8000/admin/dashboard
. You should see all API requests being displayed on your dashboard - whether they were completed or not.
Conclusion
Twilio Sync provides a powerful and flexible API that helps us add real-time capabilities to our applications, and in this article, we have seen how it can be used to power a simple API analytics tool. You can find the complete source code on Github, and if you have an issue or a question, feel free to create a new issue in the repository.
Michael Okoko is a student currently pursuing a degree in Computer Science and Mathematics at Obafemi Awolowo University, Nigeria. He loves open source and is mostly interested in Linux, Golang, PHP, and fantasy novels! You can reach him via:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.