Celebrate Anniversaries With Your Loved One Using Twilio Voice Calls
Time to read:
As humans, we generally tend to celebrate anniversaries in the life of a loved one. Examples of such anniversaries can include weddings, birthdays, or job anniversaries. And the frequency at which they occur varies, from yearly, to quarterly and even monthly for some of them.
In this tutorial, we’ll be building a NodeJS app that allows you to celebrate special anniversaries in the life of a loved one using Twilio Programmable Voice.
Prerequisites
To follow along, you’ll need the following:
- A Twilio account (free or paid). If you're new to Twilio, click here to sign up.
- A Twilio phone number
- Node.js version 14.16 or above is installed on your machine
Getting Started
First, create a directory where the project will reside. From the terminal, run the following command:
Next, change into the project directory and run the following command to create a new package.json file in the root of your project directory.
Next, install all the dependencies the project will need. These are:
- dotenv: A library for importing environment variables from a
.env
file. - twilio-node: A Twilio helper library for interacting with Twilio’s REST API.
- moment: A Javascript date library for working with dates.
- node-cron: A library that allows you to schedule task in Node.js
Run the following command to install all of the dependencies at once:
Set up Twilio
You will need to obtain the Account SID, Auth Token and phone number associated with your Twilio account. These details can be obtained from the Keys & Credentials section on your Twilio Dashboard.
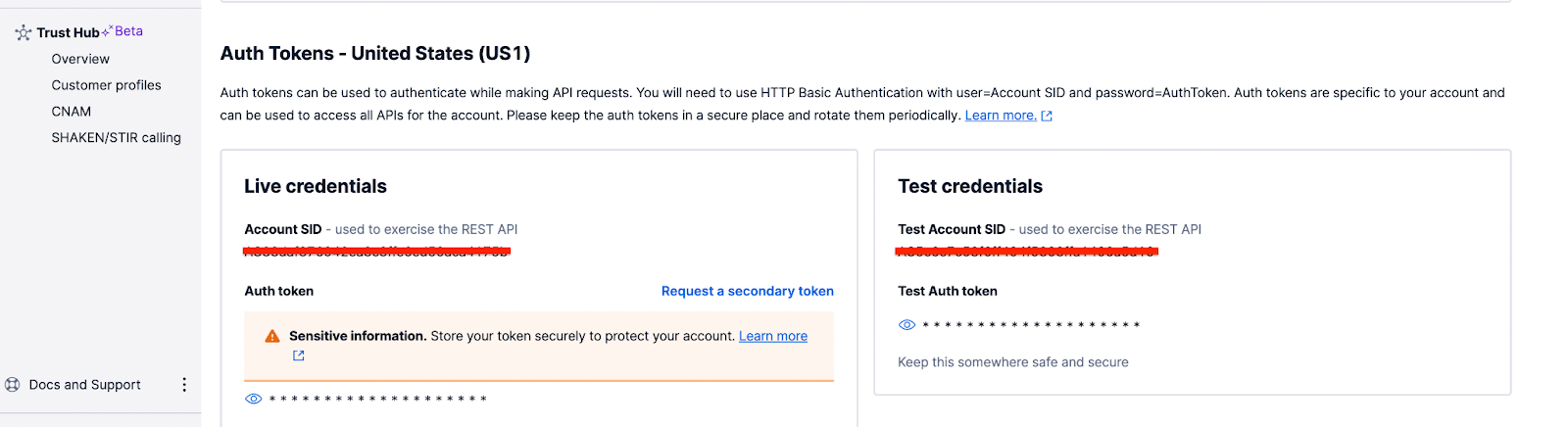
Head back to the project’s directory and then create a file named .env. Then, add the following details to the file:
Don’t forget to replace "xxxx" with their corresponding values. The PARTNER_NUMBER
field is the phone number of your partner or loved one you’ll be placing a phone call to.
Create an anniversary list
For the purpose of this tutorial, you’ll use a simple JSON file to keep track of all the important anniversaries you need to celebrate with your loved one. In your project directory, create a new file named anniversary.json, and paste the following into the file:
Each anniversary entry contains the following details:
anniversary
: The title of the particular anniversary you’re celebratingday
: The date for which this anniversary occursfrequency
: The celebration frequency for the anniversary, which can bemonthly
oryearly
. Later down the line, the value of this field will be used to update the anniversary's datemessage
: The anniversary message you would like to be said to your partner during the voice call
Creating Outbound Voice Calls
In the root of your project’s directory, create a new file named app.js and paste the following code into the file:
At the top of this file, all the dependencies the project will need are imported.
The require("dotenv").config()
function loads the environment variables from the .env file you created earlier.
The celebrateAnniversary()
function fetches all the anniversaries available in the anniversary.json file. Next, it loops through each of them. If there’s a match with an anniversary date and the current date, the placeCall()
function is invoked, and then subsequently, the anniversary day is updated based on the anniversary’s frequency.
The fetchAnniversaries()
and writeAnniversaries()
functions are used to read from and write to the anniversary.json file respectively.
The placeCall()
function is responsible for making the voice call to your loved one. A predefined message will speak to the receiver using the Twilio Markup Language (TwiML). At its core TwiML is an XML document with special tags defined by Twilio. The message to speak to them is obtained from the message
attribute on the anniversary
object.
Automate the application with Cron
Next, you're going to automate app.js to run everyday. Just below the updateAnniversaryDay()
function, add the following function:
Using the node-cron library which you installed earlier, you’ve defined a Cron schedule that calls the celebrateAnniversary()
function everyday at 8am.
Testing
To test that the app works as expected, you’ll need to make a few changes.
- Update any of the anniversaries in the anniversary.json file to reflect the current date
- Update the
PARTNER_NUMBER
environment variable to be your own, personal, phone number so you can receive the phone call - Update the Cron schedule in app.js to run every two minutes, by updating the call to
cron.schedule
to match the code below:
Finally, run the following command to start the application:
Within two minutes, an outbound phone call should be placed to your number with the anniversary message being read to you.
Conclusion
In this tutorial, using Twilio Voice, we’ve seen how you can build an application that celebrates special anniversaries in the life of a loved one. This tutorial can serve as a starting point for you, if you’re looking to build something special for a loved one using any of Twilio’s product offerings such as Messaging, Video, etc.
Dotun is a backend software engineer who enjoys building awesome tools and products. He also enjoys technical writing in his spare time. Some of his favorite programming languages and frameworks include Go, PHP, Laravel, NestJS and Node.
Website: https://dotunj.dev/
Github: https://github.com/Dotunj
Twitter: https://twitter.com/Dotunj_
Image Credits
- “Anniversary Cake. 3-tier anniversary cake” by Angie Chapman (used in the background of the tutorial's main image) is licensed under CC BY-ND 2.0
- "birthday cake" by waferboard (used in the background of the tutorial's main image) is licensed under CC BY 2.0
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.