Create an Eventbrite App that Notifies You of Local Events with Twilio SMS and Laravel PHP
Time to read:
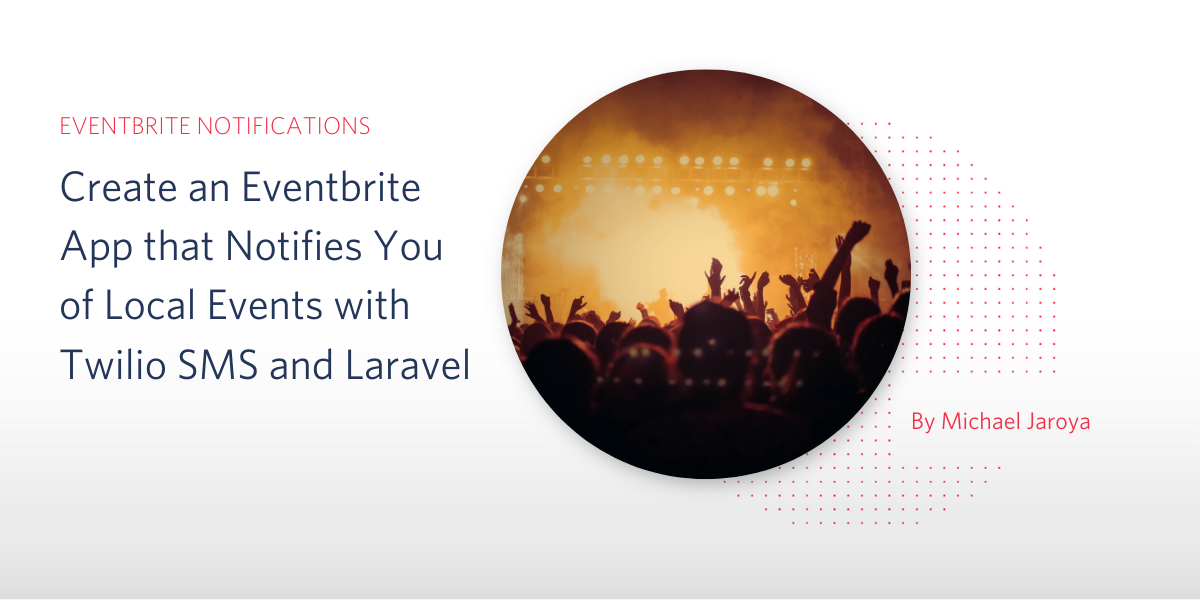
Networking is a skill that everyone needs, especially if you are an entrepreneur or plan on being one. A strong professional connection can help take your business to the next level. Those connections can provide solutions to the complex problems you’re facing, expand your customer base, and much more. Even if you’re not an entrepreneur, networking is a proven method to career and economic advancement.
The easiest way to create this connection is by attending local events in your city and interacting with people physically. I can’t count the number of times I have googled the keywords “events near me” only to see a lot of irrelevant results, not to mention the noise of ads. The events that are relevant are either in a faraway city or in another country altogether.
In this tutorial, we are going to implement a simple app that sends daily SMS notifications of events happening near you using Twilio SMS and the Eventbrite API.
Getting Started
To complete this tutorial, you will need the following:
- Twilio Account
- Eventbrite Account
- PHP version 7+
- Composer globally installed
Create a New Laravel Project
Create a new Laravel project by running the following command in your terminal:
Set up the Twilio SDK
First, we need to install the Twilio PHP SDK using Composer. This will allow our application to easily connect to the Twilio API and programmatically generate SMS. In your terminal, run the command below:
Creating an Eventbrite Personal OAuth Token
Our application will be pulling its data from the popular event management platform, Eventbrite. In order to request data from the service and use the Eventbrite API, you must have a personal OAuth token. If you don't have a token, create one by following this guide.
After you have completed your token setup, install Guzzle to execute the HTTP API requests. Guzzle is a great alternative to writing your server-side requests in cURL. Run the command below to install the latest version:
Once these steps are complete, update the .env
file with the credentials from your Twilio and Eventbrite dashboards as shown below:
Create the Event App
We will create a model and extend it with custom methods to fetch events from Eventbrite based on location and time filters. Once filtered, the events will be sent to a list of subscribers using Twilio SMS.
Create the Event Model
Run the command below to create the Event
model:
Create Logic to Fetch and Send Events
We will use a function to fetch events from Eventribe and pass location and time filters. Let's make the API to return events happening this week within the locality of the subscriber.
You can always change these filters to fit your needs. More information on event filters can be found in the Eventribe docs. Open the app/Event.php
file generated by the previous command and add the following method.
The fetchEvents()
function accepts five parameters:
$location
- the location of the subscriber e.gNairobi
$date
- the start date keyword-
$within_radius
- the radius covered from the location specified $limit
- the maximum number of events to be returned$sort_by
- how to sort the events
The start $date
keyword returns events with start dates within the given keyword date range. Keyword options are "this_week", "next_week", "this_weekend", "next_month", "this_month", "tomorrow", "today".
The $sort_by
parameter supports three options; "date", "distance", and "best". Prefix with a hyphen to reverse the order, e.g. "-date".
By default, the app will return events within a 10 Kilometres radius and sort by “best”.
Once we receive the response from Eventbrite with the list of events, we transform it into a format that can easily be consumed through SMS and remove unnecessary details.
Next, we need to create a function to send the fetched events to subscribers via Twilio SMS. Add the code below to the Event model:
The sendSMS()
function accepts two parameters; $to
the number of the recipient and the $events
which forms the SMS body.
Consolidating the App
Having all of the components required to build the app, let’s put everything together. Reopen the Event
model in app/Event.php
and add this final method:
The Event Artisan Command
Lets create an artisan command that we will call from Laravel Scheduler to automate the process of sending events to the subscribers.
Run the artisan command below to generate the artisan command:
Open the file app/Console/Commands/sendEvents.php and update the
handle method as shown below:
Laravel's command scheduler allows you to fluently and expressively define your command schedule within Laravel itself. When using the scheduler, only a single Cron entry is needed on your server. Your task schedule is defined in the app/Console/Kernel.php
file's schedule
method.
Next, update schedule
method with this single line of code.
The command will be executed every Monday and Friday at 6:30 AM.
If you need help, you can use http://corntab.com/ to create cron rules.
Finally, add the following Cron entry to your server.
This Cron will call the Laravel command scheduler every minute. When the schedule:run
command is executed, Laravel will evaluate your scheduled tasks and runs the tasks that are due.
Testing
To test the app, navigate to your project directory and run the artisan command below:
You should get an SMS as shown below:
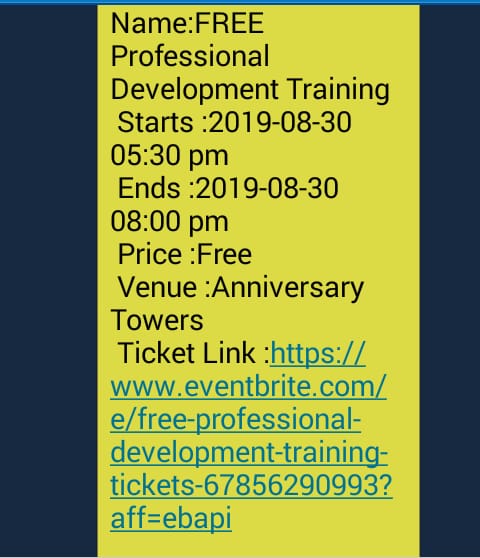
Wrap Up
To build upon this app, you can tailor the events received by each subscriber to be based on their interests and biases and also give them the liberty to choose the frequency in which they want to get notified. You can achieve this by providing your subscribers with a platform where they can set their preferences. The code is available on Github.
Have some ideas to better improve the app? Let me know. I can’t wait to see what you build.
Feel free to reach out to me:
- Twitter: @mikelidbary
- GitHub: mikelidbary
- Web: www.talwork.net
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.