Eurovision Sweep with Twilio Functions
Time to read: 2 minutes
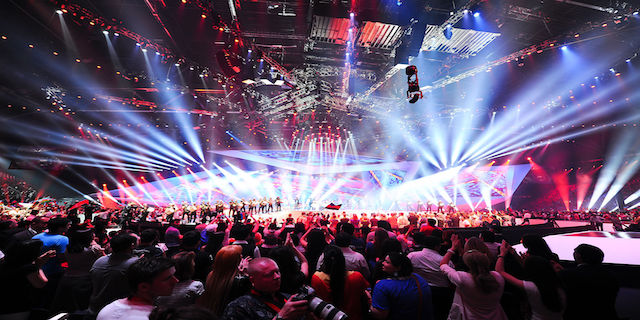
As an Australian, I never got the buzz about Eurovision. For those of you not familiar, it’s a huge annual televised song contest involving the nations of Europe where each country submits an original song and performs live. But now that I live in London it seems appropriate to be getting excited about my first one since I moved!
My wife came up with the idea of having a Eurovision party, involving a sweep where attendees would be randomly assigned a country, bet a nominal amount, and bring a national dish from that country. The app won’t do the cooking, but it will conveniently provide a link to Wikipedia to provide the details and link to the voting page to see if you are in the money.
She was too busy to sit down and work out a sweep, so I figured, working at Twilio, there must be an easy solution. And there is!
First, I had to get all the finalists. I installed requests and beautifulSoup libraries, and then used them to web scrape all the info I needed. I dumped this into a JSON file called euroSweep.json. The code below shows how requests gets a web page and then use beautifulSoup to get the elements of the page that I needed to get the country names.
Next, I needed somewhere to host everything. I was out of time to run up my own server, so I turned to Twilio Functions and Assets. The first step was to upload my JSON file to Assets. Make sure you upload it as a Private Asset or it won’t be available to your Function!
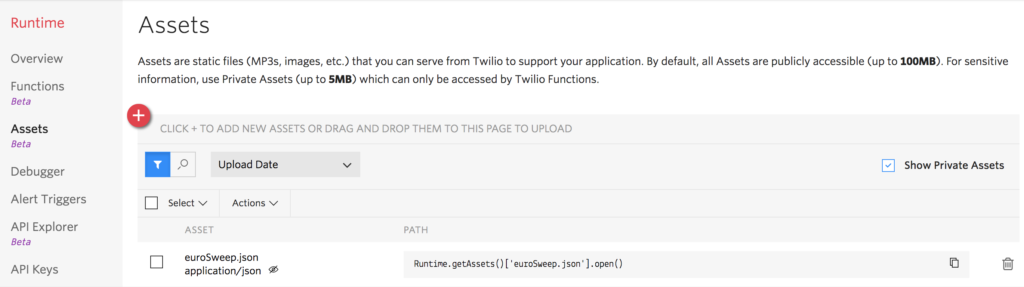
Next, I had to create a Function. Functions are lightweight, event-triggered pieces of code that run in the cloud and scale automatically. Best of all you create them in your own Twilio Console. The Function I created accepted an SMS containing a number of attendees and returned a random, unique country for each person by SMS. The code for this is below. You’ll also need to Name your Function, set the event Dropdown to Incoming Messages and set a path.
The last step was to connect my Function to a phone number. In fact why not make it available across Europe (and Australia, since we are special guest stars after all!)
Through the Console I purchased numbers for my required countries and configured Incoming Messages to point to my shiny new Function!
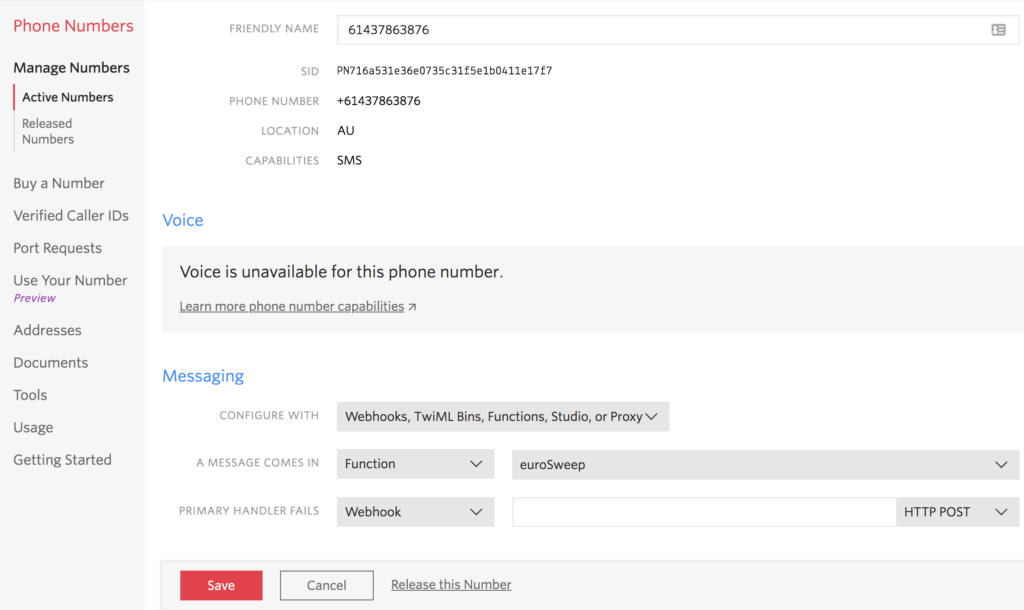
Why not try it out?!
Text eurovisionsweep followed by the number of participants to one of the numbers below, eg eurovisionsweep 5 if there are five people in your sweep
Australia: +61437863876
Estonia: +37259120269
Germany: +4915735981364
Ireland: + 353861800407
Sweden: +46769439050
UK: +447481341033
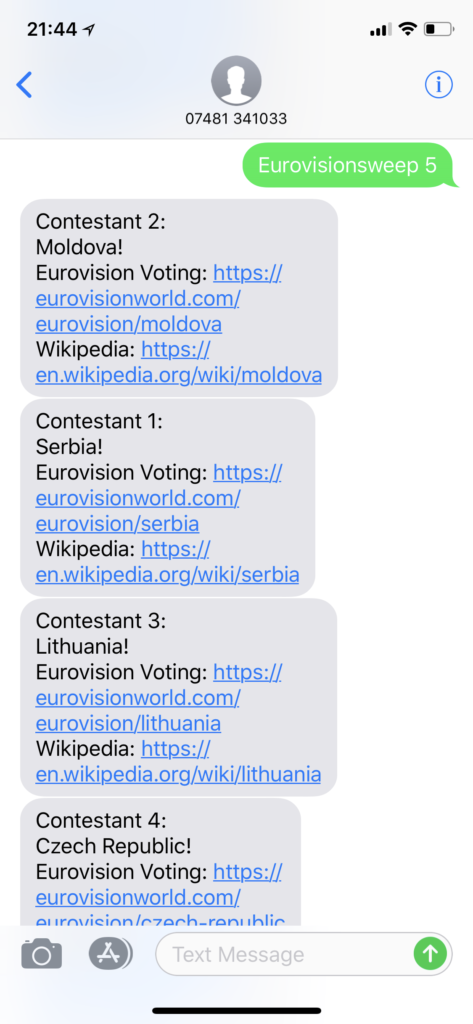
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.