Hosting Live Webinars with JavaScript, Node.js, AdonisJs and Twilio Programmable Video
Time to read: 5 minutes
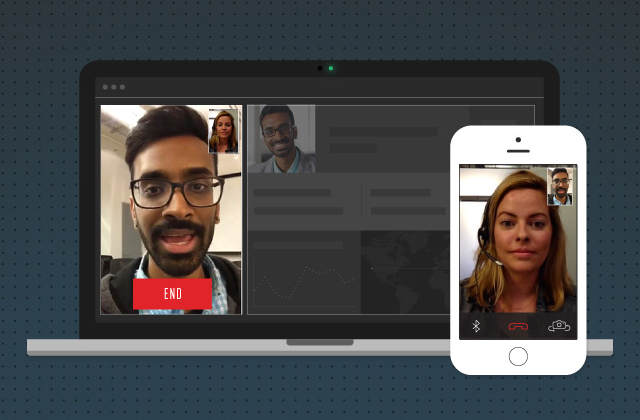
We’re going to use Twilio Video with the AdonisJs framework to create a system where a user can host a video, and viewers can watch their presentation. AdonisJs is a full-stack, open-source MVC framework for Node.js that was inspired by the Laravel framework and borrows some of its concepts. AdonisJs saves you time and effort becauses it ships with a lot of features out of the box.
This system can be extended that users can sign up and schedule talks on, even pay to use. But we’re going to keep our project simple so it is easier to build your initial application.
What is Twilio Video?
We will use Twilio Programmable Video to build our app. Twilio’s Video SDK is a fast way to build a full featured WebRTC video solution across web, Android and iOS. We’ll be using the JavaScript SDK but you can also use the iOS and Android SDKs to extend this project to mobile apps later on.
Twilio Video and WebRTC is best used for small groups. Peer to peer Twilio Video Rooms can have a maximum of 10 participants and Group Rooms can have up to 50 participants. If you are hoping to host webinars larger than 50 participants then for now I would advise against this solution due to current WebRTC limitations.
To get started, you’ll need a Twilio account so sign up for one now if you haven’t already.
Once you get signed up or are signed into your Twilio account, go to the Twilio Console. Go to Programmable Video
under the sidebar navigation panel. Under Tools
you’ll see an option for API Keys.
Create a new API Key, copy the API Key and the API Secret to a safe place so you can use them in your app.
Install Node.js and AdonisJs
You can follow along or copy and paste code from this open source repository named groupinar.
Make sure you have Node.js and npm installed, I’m using Node.js version 8 at the moment.
Install the AdonisJs CLI:
Create our video app:
This will create a folder named group-video
which will contain our application’s code. You can now test it:
You should see output similar to this:
Open http://localhost:3333 in your web browser and you’ll see the welcome page.
Now that we’ve got the barebones, let’s add Twilio Video to our app.
First install the following modules:
Add our Twilio configurations, so open .env
from the project directory and add the following three lines and add your account sid and API key and secret you created earlier:
We can now create our Controller and set up our Routes using the adonis command:
It will prompt you to choose the controller type:
Choose Http Request.
If you look in the app/Controllers/Http/
folder, you’ll see you now have a file called TalkController.js
.
In our editor, add the following two lines directly below the 'use strict'
line:
Inside the TalkController
class add three new functions:
In the token
function, we called:
This will assign each user, regardless of being a host or a guest with a random 10 digit name.
The other two functions are close in what they do, the difference is the host
function sets the hostOrGuest
variable to “on”, and the guest
function sets the hostOrGuest to “off”. This will be used later in our view.
Within those functions we also retrieve a group room using the slug from the URL. If the room doesn’t yet exist, we create it.
This lets us add up to 50 people to a room rather than the usual limit of 10.
Open the start/routes.js file, and look for this line:
Add these three new routes directly below it:
We’ve created a route group, which tells our app that any route beginning with /talk is part of the group. The routes inside this group then line up with the three functions we created earlier:
/talk/token
will call thetoken
function inTalkController
and return our token for each user./talk/host/:slug
will call ourhost
function, creating a room based on theslug
we passed./talk/:slug
will call ourguest
function and display the room.
Run your app using:
Go to http://localhost:3333/talk/token and you will see a generated token.
Creating our Views
We need to create our views
to present something to the user and make our video system do something. Run:
This will create a new file called talk.edge
inside the resources/views
folder. Edge is the template language used by AdonisJs.
We’re only creating one view that will be used by both hosts and guests, the difference will be if we broadcast video or not.
This view sets up how we want our site to look, it will display videos on the left, and a list of attendees on the right.
Towards the bottom, you can see where we specify an object called talk
, in this object we pass the chat room as slug
and whether we are in host mode or not.
Ok, quick recap:
- We created our controller, and set up routing to said controller
- We set up our view to display our videos
We are almost done, all we’ve got left is to set up the final piece of our application to establish the actual connection.
In the public
folder, create a file called app.js
:
In the first piece of our file, we’re calling that /talk/token
route and getting a token that is allowed to use video, then we’re checking if talk.host
was set to "on"
for host mode, or "off"
for guest mode.
If host
is set to "on"
, then we don’t enable video broadcasting and we just watch instead.
Next we tell Twilio to connect to the room that is specified by talk.slug
.
As part of this, we display any other video windows, and participants, and set up listeners to watch for connections and disconnections. Now for the last part of the script, which are the functions we call when participants join or leave:
That’s our video app, this can be used to host webinars pretty easily, or any other type of video chat where you want to use a one-to-many type situation.
Make sure your application is still running with:
Hosting and attending a talk
Hosting a talk is pretty straight forward, you just open the URL with a slug for it:
http://localhost:3333/talk/host/slug
This will create a webinar with the slug of slug, if you want other users to broadcast a video feed as well, then you can share this url to them.
To attend a talk, you would just share the guest URL:
http://localhost:3333/talk/slug
Any visitors who use this URL would see all video hosts on the talk and can watch the broadcast while not sharing video.
What’s next?
You’ve gotten a nice intro to both AdonisJs and Twilio Video, and we’ve made video chats a little unconventional with the host and guest mode.
This app can be extended pretty quickly to include user accounts, Q&A, or chat directly so that guests can interact with hosts using Twilio Programmable Chat.
You can find the sample code here on GitHub. If you encounter errors while working through this post or with the finished source code, please leave a comment or open an issue on GitHub.
You can extend it and if you’d like to show me what extra functionality you added, got questions, or want to send a thank you tweet, you can reach me via email at roger@datamcfly.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.