How To Build Your Own MMS Enabled Motion Activated Security Camera With Linux, Python and S3
Time to read:
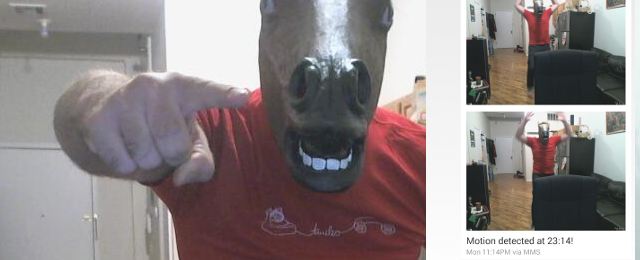
Holy biscuits was last week a barrel of monkeys. All of us at Twilio have had a beastly boatload of fun seeing the stuff you’ve started building with Twilio MMS. Many of you blazed through Kevin’s Getting Started with MMS tutorial over the weekend to get started on your hacks and, of course, seeing all your mustached faces with the example project we built last week remains a source of never-ending laughs.
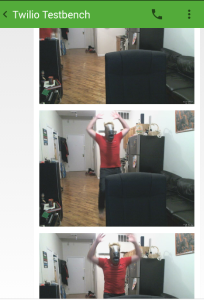
This go round, I thought we would have a look at something a little more real world. Working on the devangel crew, I get to travel around the world meeting all kinds of developers producing rad work. While on the road, keeping an eye on the apartment back in Brooklyn definitely provides some peace of mind. For my second hack, I thought I might provide that in real time using MMS. These kinds of cameras are available on Amazon for $180, but I wanted to see if I could build my own for $0 with my Linux desktop, a webcam I already own and a little Python code.
What does it look like? Let’s take a look at what happened when a clearly agitated mutant horseman broke into my apartment after deploying the Twilio-powered camera.
Yeesh. Harrowing.
For this project, we’re going to build a security camera that sends a photo whenever motion is detected using the tools below.
What You Will Need
For this project, we’re going to need a few things before we get started.
- A Twilio account – you can sign up for one here for free.
- A MMS-enabled Twilio phone number (available in the United States and Canada).
- Python
- pip
- Twilio Python module
- A free Amazon Web Services account – you can sign up for one here.
- boto, the official Amazon Web Serivces Python package.
- motion, a software motion detector for Linux.
- A Video4Linux supported webcam – I used the $70 Logitech C920.
How It Works
We’ll leverage a few different technologies to build our MMS enabled security camera. We’ll use an excellent software motion detector for Linux (aptly called Motion) to watch for any movement in the frame. When Motion detects some movement, it’ll launch our Python app which will look for an arbitrary number of images over a few seconds to craft into an alert.
Now, if our machine connected to the camera was available on the public Internet, the images would be easily available for Twilio to see. However, as a quick Google search will show, having your security camera available on the Internet may not be a great idea. So instead, our script will upload that photo to Amazon S3 so that Twilio can get it. We’ll then use Twilio MMS to send ourselves a message with the S3 public link as the MediaUrl.
Getting Started
Let’s begin by getting our dependencies installed. First, we’ll need to install some Python modules to interact with Twilio and with Amazon S3. Note, if you are not using virtualenv, you will need administrator privileges to install.
If you prefer, you can use a requirements.txt file to do this.
Next, we’ll install Motion to handle our video. Installation options for his open source motion detector are here for the Linux distribution of your choice – for this tutorial, we’ll use Ubuntu.
Finally, we’ll create a new S3 bucket to house our camera. First, we’ll log into the AWS Management Console and navigate to S3 administration panel. Then we’ll click the Create Bucket button in the upper left hand corner.
Next, we’ll name our bucket.
Then we’ll open the properties of our newly created bucket.
Then add a new bucket policy in the Permissions dialog:
Click “Add a new policy”.
Next, we’ll write a new policy to make the photos we upload publicly accessible so Twilio can reach them for our MMS alerts. Bucket policies are written in JSON and you can use this template as a guide:
Finally we’ll click “Save” to apply the policy making sure all the images we upload will be available on S3.
For one last optional step, we can create a new access key for our app. First we’ll go to the Security Credentials page of our management console and click on “Access Keys.”
And then we can generate a new key.
Click Show Access Key and we can keep our values for our AWS credentials or download the root key csv file.
With our AWS setup completed, we’re ready to get Motion configured.
Configuring Motion
The first piece we need to set up is the configuration of Motion to use our camera. This will involve manipulating a few of the configuration settings to execute the script that we are going to write together. We can copy the example below into a file we’ll call local.conf
.
Here’s an example with the important values we need:
With this configuration, each time period during which Motion detects movement in the frame, it will execute a Python app twice – once at the beginning of the movement and once at the end. Now all that is left is to write the app itself.
Connecting Motion To Your Phone With Twilio
With our bucket and motion detector configured, we can now cut some code. Our app will need to do three things:
- Get an image that Motion has recorded.
- Upload that image to a place where Twilio can retrieve it.
- Create a new Twilio message with the location of that image as the MediaUrl.
Let’s create that app together by opening a new file named motionalert.py
.
First up, we will import everything we need for the app and create a class to serve as basis for the alerts we want to send from Motion through Twilio to our phones. We’ll also create an Exception handler so we can gracefully return error information in the command line with a hint to use our command line interface’s --help
flag.
Next, we’ll define a function to get an image that Motion recorded. As Motion operates on a buffer for the motion analysis, we can just grab the most recently created image in the target directory to be reasonably assured it will catch something meaningful in frame.
Now that we have code to find images, we need to upload them to S3 so Twilio can find them for the messages we are about to create. Now, here’s where we start using that S3 bucket we set up earlier in the tutorial. Let’s define a function on our MotionAlert
class to help us upload our images to that bucket.
Now that we have the image in a place where Twilio can see it, let’s add another function to our MotionAlert
class to send an MMS to our phones.
Now, let’s tie all that together with a send
function to string our three functions together.
Lastly, let’s create a command line interface for our class by using argparse
.
With the pieces of functionality in place, let’s put it all together to see what it looks like together in one copy/pasteable snippet.
Giving It A Try
With our S3 bucket and Motion configured as well as our Python app written, now we get to give it a go and see what happens.
Let’s do that by executing this command using the local.conf
file we made towards the beginning of the tutorial.
motion -c local.conf
We should get some output indicating Motion is firing up:
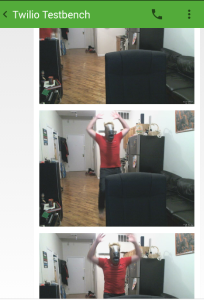
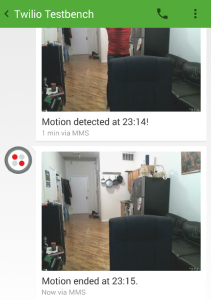
Wonder why that guy had such a long face. BOOM.
Next Steps
Now that we’ve got a rudimentary motion camera sending MMS alerts, we can build on even more functionality in the future. For example, we could:
- Send MMS alerts to multiple phones.
- Spin up and spin down the camera based on an SMS command.
- Convert recorded video into a single animated GIF instead of still frame pictures.
- Alert only when there is movement in certain sections of the frame to reduce false positives.
- Install this rig with a low-power Linux device like a Raspberry Pi.
Playing around with hardware like cameras in creative ways can unlock even more potential for Twilio MMS. If you’re hacking your cameras in creative ways, I definitely want to hear about it – you can find me on Twitter @dn0t or via email at rob@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.