Learn How to Code: A Beginner's Guide
Time to read: 8 minutes
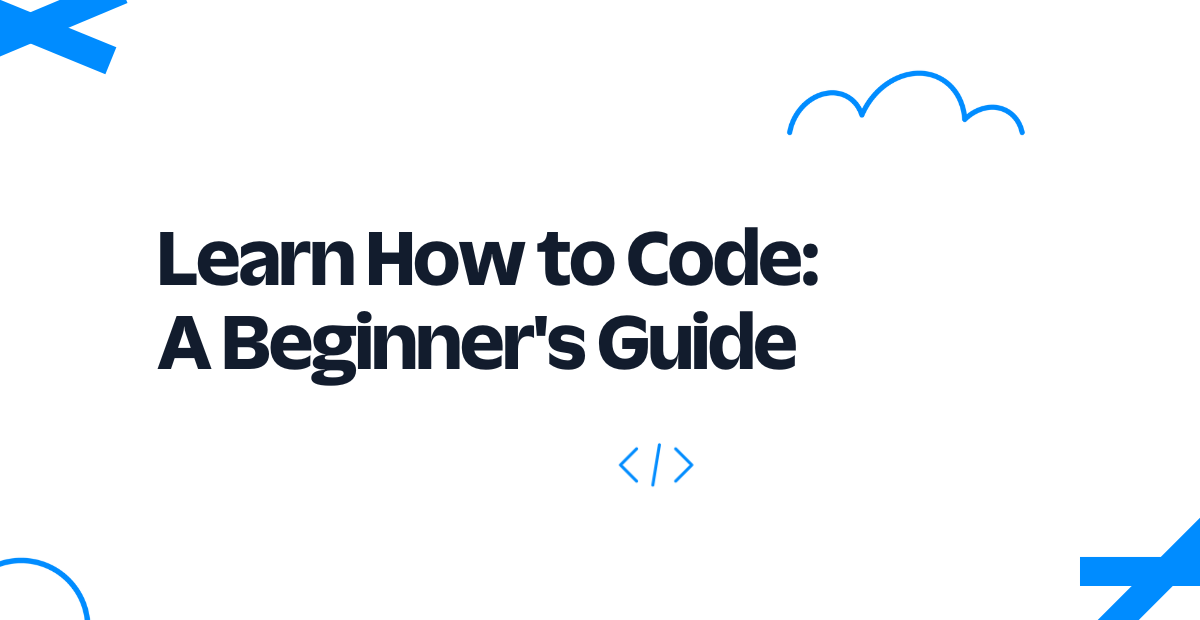
Learning how to code can be challenging, and getting started may seem overwhelming.
But Twilio is here to help. We’ve put together this guide to get you started on the right foot.
We’ll take you step-by-step from where to begin, through the essential tools for coding, to a few foundational programming concepts. By the end of this article, we hope you feel confident starting your coding journey.
Where do I begin learning how to code?
The key to learning to code is taking the process step-by-step. In this post, we’ll look at:
- Which language should I learn?
- Which development tools should I use?
- What are some basic coding concepts I should know?
Before you jump in, however, we recommend thinking through your goals. Do you want to acquire new skills for your current job? Are you looking to start a career in software development? Or are you curious and want to pick up a new hobby? A clear understanding of your purpose and goals for learning to code will help inform the approach as you move through this guide.
There are many resources available to help you learn how to code for free or at an accessible price—online tutorials, coding boot camps, and even textbooks. Online learning platforms are an excellent place to start. Examples include:
These platforms offer interactive courses that guide you through the basics of programming and allow you to practice what you learn in real time.
Let’s start with languages and which one is right for you.
Which coding language should I learn?
With so many options out there, choosing a language can seem daunting. The good news is many of the concepts of coding are the same from language to language. So don’t stress too much over your choice. Let’s walk through some of the modern, popular languages that would all be appropriate starting points, plus the pros and cons of starting with each.
The most accessible type of coding language for a beginner to learn is an interpreted language, sometimes referred to as a scripting language. Scripting languages can be run immediately without the need for additional tools to package them up and make them executable. Interpreted languages like Python, JavaScript, and Ruby are a good start for those new to coding, so let’s take a closer look at them.
Python
Python has a clean syntax that’s straightforward to read and write. It’s also a versatile language used across many domains, including web development, data analysis, AI, and machine learning. Thanks to this versatility, beginners can apply their Python skills in various domains as their interests evolve. Many Python developers also use frameworks like Flask to help them in their development.
Here are a few resources for learning Python:
- Learn Python 3 from Codecademy
- Interactive Python tutorial from LearnPython.org
- Programming for Everybody (Getting Started with Python) from Coursera and the University of Michigan
JavaScript
JavaScript is the primary language for web development, making it an essential skill for aspiring web developers. Starting as a client-side (or browser) scripting language, it evolved to form the basis for Node.js. Node.js is a runtime environment for building full-fledged web applications using frameworks like Express, React, Vue.js, and more.
Here are a few resources for learning JavaScript:
- Learn JavaScript from Codecademy
- JavaScript First Steps from Mozilla Developer Network
- JavaScript Programming video course from freeCodeCamp
Ruby
Ruby has an elegant and human-readable syntax, and web developers use it widely, particularly with the Ruby on Rails framework. This framework provides a solid foundation for building web applications and helps beginners get up to speed quickly.
Here are a few resources for learning Ruby:
- Learn Ruby from Codecademy
- The Definitive Ruby Tutorial for Complete Beginners from RubyGuides
- Full Stack Ruby on Rails from The Odin Project
These three programming languages have a reputation for readability and accessibility for beginners and enjoy broad and supportive developer communities. Plus, these languages have rich ecosystems of libraries and tools to help new developers.
Which development tools should I use?
Once you’ve selected a language and are ready to get started, you’ll need some tools to do your job. As you begin coding, you’ll write your code in a text editor and likely test it within a web browser. So you’ll need to install a good text editor (such as VS Code, Sublime Text, or Atom) and have access to a web browser.
Most web browsers have a developer tools feature (like Chrome and Safari) with a JavaScript console and detailed network request activity. These will be helpful once you begin coding and testing.
In addition to a text editor and a web browser, we recommend the following tools.
Terminal window or command line interface
A terminal window or command line interface (CLI) allows you to interact with your computer's operating system using text commands. If you’ve never coded before, you might only be familiar with your desktop interface with its windows and double-clickable icons. Once you enter the world of coding, you’ll find yourself typing in a terminal window instead.
The CLI is an essential tool for running scripts, managing files, and working with your version control system.
Version control system
A version control system (VCS) allows you to track changes in your code, helping you follow the evolution of your code and see where you may have introduced issues (also known as bugs). A VCS helps prevent the loss of work by maintaining a history of changes and lets developers revert to previous versions of their code if needed.
Using a VCS also encourages collaboration, as multiple coders can work on the same project. Coders can save changes to their files and push those changes to a central location to share with the rest of the team. Two coders can even make changes to the same file, and a VCS will sort out and merge any conflicts between the edits. This approach allows beginners to collaborate with others on projects and learn from their peers.
Git is the most widely used VCS. GitHub and GitLab are popular platforms for hosting your Git repositories online.
Integrated development environment
An integrated development environment (IDE) is something many programmers spend their entire day working inside. It's an application that provides a full-fledged environment for writing, editing, testing, and debugging code. As a coding toolbox filled with helpful features, an IDE can help smooth the initial development process for beginners. IDEs offer code completion, syntax highlighting, and error detection, helping new programmers write and understand code.
Some IDEs even include built-in tools for version control and code refactoring. This helps beginners manage their projects and improve their code more efficiently.
Examples of popular IDEs include Visual Studio and PyCharm. Choose an IDE that supports the programming language you’re learning.
Now that we’ve covered some of the essential tools for coding beginners, let’s wrap up our guide with some helpful pointers for aspiring coders.
What are the basic coding concepts I should know?
No matter the language you choose, you’ll encounter several foundational coding concepts. It’s vital to understand these as you’ll run into them daily. Let’s introduce them briefly.
Data types
Data types represent the different kinds of data a programming language can handle. Common data types include:
- Integers: Whole numbers, such as
-123
,42
,5000
. - Floating-point numbers: Decimals with varying degrees of precision (also known as floats), such as
-0.0001
,3.14
,99.9
- Strings: Sequences of characters usually denoted through single or double quotation marks, like '
hello world
' and '3.14 is an approximate value of pi
'. - Booleans: These hold the value of either
true
orfalse
. - Arrays: These store an ordered collection of data types, such as a list of integers or strings. For many languages, the collection can be a mix of different data types. Arrays are usually represented by square brackets
[]
. For example,['alice', 'bob', 'charlie']
is an array with three elements, all strings. - Hashes: Sometimes referred to as associative arrays, these store a collection of pairs—a unique key and the associated value. Hashes are usually represented by curly brackets
{}
. For example,{ 'alice': 30, 'bob': 42, 'charlie': 26 }
is a hash with three keys, each associated with an integer value.
Variables
Variables are placeholders for data values that coders use to store and manipulate data through the execution of a program. For example, let’s say we have a variable called subtotal
, and it represents a number. We might assign it a value of 60.
Then, we might have another variable called tip
, which is 15% of the subtotal
.
Finally, we have a third variable called total
, and we assign its value to be the sum of subtotal
and tip
.
Coders can use variables to manipulate data values and make computations. In our above example, tip
and total
are always calculated the same way, but the resulting value depends on the value of subtotal
. By changing just the value for subtotal
(for example, to 100), we would change the computed values of tip
and total
too.
In some programming languages (such as C++ or Java), you must declare the data type of a variable before using it, and you can’t change that data type afterward. In others (such as JavaScript or Python), you don’t need to declare a variable’s data type at first use, and you can change it whenever you want. The latter approach is more flexible for beginners.
Functions
Functions are reusable blocks of code that perform a specific task. Functions can accept inputs (called parameters or arguments) and typically return a result. For example, the following JavaScript function takes 2 numbers as arguments, then returns the sum:
If we call this function within a JavaScript console, it looks like this:
Functions help make code more modular, maintainable, and readable.
Conditional statements
Conditional statements allow your code to take different paths based on specific conditions, using logical expressions to determine which subsequent block of code to execute.
In many programming languages, you begin conditional statements with an if
condition, followed by a block of code to execute if that condition is met. Then, you may use an else if
condition, followed by another block of code. This section basically says, “If that first condition wasn’t met, but this one is, then do this instead.” Finally, you may use the keyword else
, followed by another block of code. This last section says, “Since none of the above conditions were met, do this as a last resort.”
In JavaScript, an example of a conditional statement (which also uses variables) looks like this:
Loops
Developers use loops to execute a block of code repeatedly until a specific condition is met. Common types of loops include:
for
loops iterate over a sequence of values.while
loops execute repeatedly as long as a certain condition is true.
An example of a while
loop in JavaScript looks like this:
When the above code is executed, the result is:
Learn how to code with Twilio
Taken step-by-step, you’ll be coding confidently in no time. Keep the following in mind as you begin your journey:
- Focus on the basics. Begin by learning the fundamental concepts of programming. Learn one concept at a time, then add on other concepts as your comfort and confidence grow.
- Practice regularly. Consistency is key when learning a new skill. Set aside dedicated time each day or week to practice coding and work on small projects to apply your knowledge.
- Get your hands dirty. If you take an online coding course, don’t just let it play in the background. Your mastery of coding concepts and skills will come much faster if you write code while going through a course.
- Divide problems into smaller tasks. When faced with a complex problem, divide into more manageable tasks. This will make it easier to understand and solve the problem.
- Learn from others. When you’re stuck (or curious), don't hesitate to seek help from online forums, documentation, or peers. Learning from others is an essential part of the coding journey.
- Embrace mistakes. Making mistakes is a natural part of any learning process, and that includes learning to code. The more you mistakes you make, the more you learn.
Ready to begin learning how to code? Twilio Education can kick-start your journey with practical developer training for students, educators, and professionals. Get started today with a free on-demand course or live event.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.