How to Receive and Respond to a Text Message with Python, Flask and Twilio
Time to read: 3 minutes
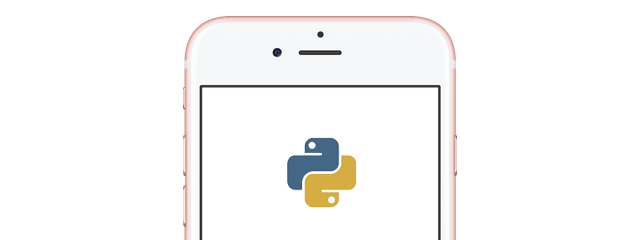
Here’s all the code you need to receive an SMS message and to send a response using Python, Flask, and Twilio:
If you’d like to know how that works, check out this short video:
Can you walk me through this step by step?
When someone texts your Twilio number, Twilio makes an HTTP request to your app. Details about that SMS are passed via the request parameters. Twilio expects an HTTP response from your web app in the form of TwiML, which is a set of simple XML tags used to tell Twilio what to do next.
First make sure you set your local environment up and have a directory where the code will live.
Open your terminal and install Flask, the popular micro web framework, which we’ll use to receive Twilio’s request:
Install the Twilio Python library to generate the response TwiML:
Create a file called app.py
, and import the Flask and request objects from the Flask library. Also import the Twilio Python library and initialize a new Flask app:
We need a route to handle a post request on the message endpoint. Use the @app.route
decorator to tell our app to call the sms function whenever a POST
request is sent to the ‘/sms’ URL on our app:
Details about the inbound SMS are passed in the form encoded body of the request. Two useful parameters are the phone number the SMS was sent From
and the Body
of the message:
Next we’ll use the Twilio library to create a TwiML <Response> that tells Twilio to reply with a <Message>. This message will echo the phone number and body of the original SMS:
And don’t forget to tell the app to run:
In your terminal, start the server which will listen on port 5000:
But how does Twilio see our app?
Our app needs a publicly accessible URL. To avoid having to deploy every time we make a change, we’ll use a nifty tool called ngrok to open a tunnel to our local machine.
Ngrok generates a custom forwarding URL that we will use to tell Twilio where to find our application. Download ngrok and run it in your terminal on port 5000
Now we just need to point a phone number at our app.
Open the phone number configuration screen in your Twilio console. Scroll down to the “a message comes in” field. You should see something like this:
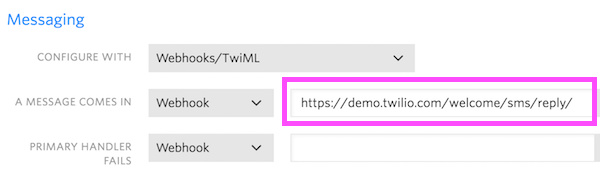
Punch in the URL for our message route that was generated by ngrok. It should look something like http://your-ngrok-url.ngrok.io/sms
.
Click save, then text your number to get a response!
Next steps
To recap, when the text hits Twilio, Twilio makes a request to our app, and our app responds with TwiML that tells Twilio to send a reply message.
If you’d like to learn more about how to use Twilio and Python together, check out:
Feel free to drop me a line if you have any question or just want to show off what you built:
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.