How to send WhatsApp Media Messages with Python
Time to read: 3 minutes
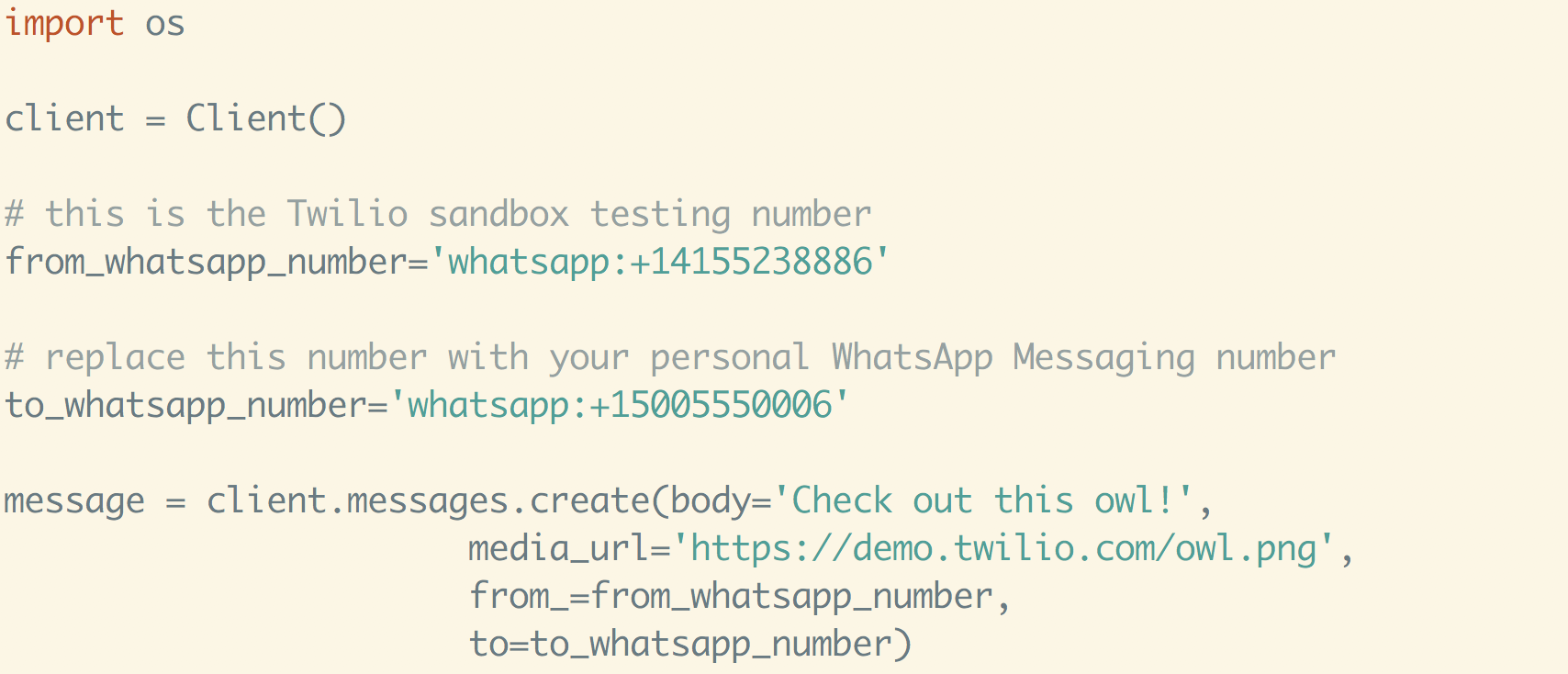
WhatsApp is a messaging service used by people all over the world. With Twilio's Messaging API you can programmatically send WhatsApp messages. Let's walk through how to use Python to send a media message over WhatsApp.
Development Environment Setup
Make sure we have the right software installed and set up that we'll need to use for the rest of this post. Throughout this post, you will need:
- Python 2 or 3 installed (do this first if you haven't already)
- A free Twilio account with an activated WhatsApp Sandbox
- The Twilio Python library
Here is a good guide to follow in general if you are going to be doing more with Twilio and Python.
Sign up for Twilio and activate the Sandbox
Before you can send a WhatsApp message, you'll need to sign up for a Twilio account or sign into your existing account and activate the Twilio Sandbox for WhatsApp. The Sandbox allows you to prototype with WhatsApp immediately using a shared phone number, without waiting for a dedicated number to be approved by WhatsApp.
To get started, select a number from the available Sandbox numbers and follow the instructions to activate your sandbox.
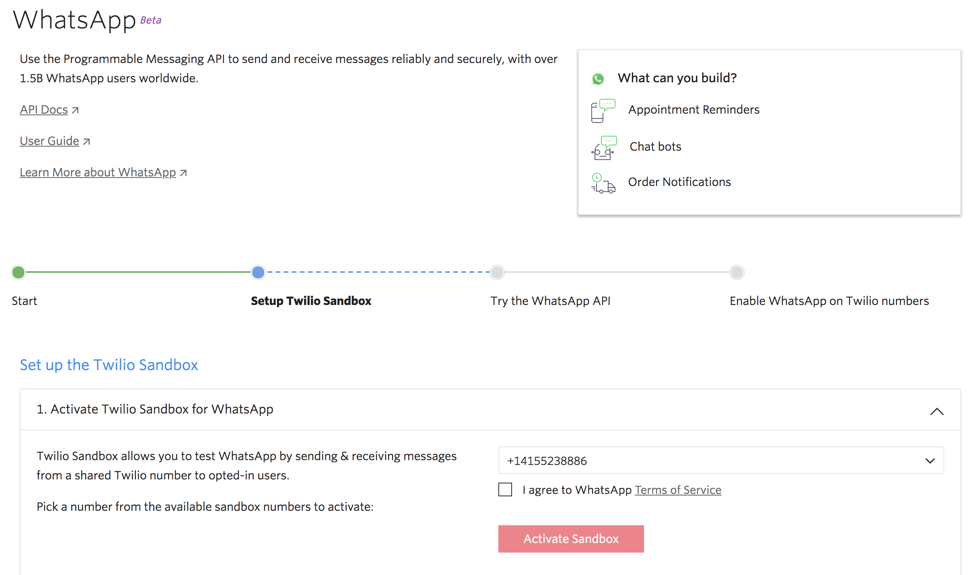
Take note of the phone number you choose in the Sandbox. You'll need this later when we're ready to send some messages.
In order to use the Sandbox, you need to opt in by sending the phone number you chose a message from WhatsApp. Send "join <your sandbox keyword>” to your Sandbox number in WhatsApp, and you will receive a confirmation that you’ve joined. Your sandbox keyword can be found in the console.
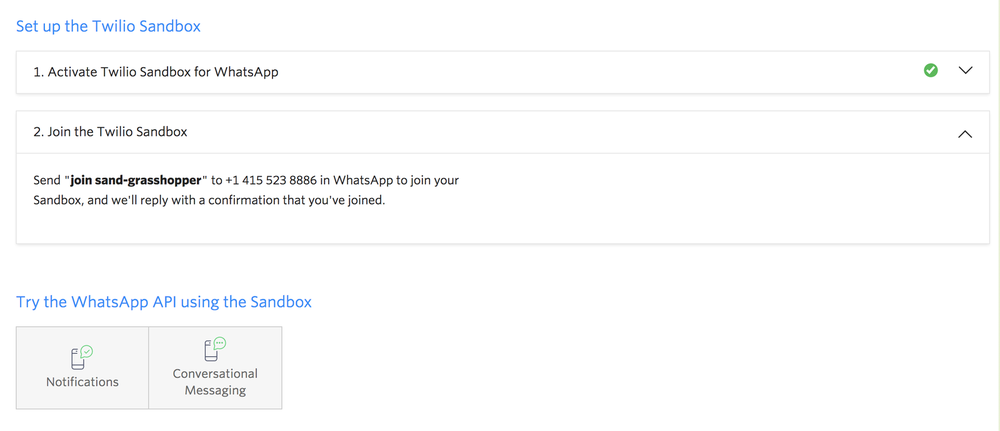
Sending a WhatsApp message with Python
Now that you have a Twilio account and have activated the WhatsApp Sandbox, you're ready to dive into some code and send messages! Let's use this owl picture for the media we are going to send, because owls are cool. Start by opening your terminal and navigating to the directory where you want your project to live.
First install the virtualenv package then run the following command:
After either of those steps, activate the virtual environment:
Install the Twilio Python helper library into the virtualenv:
With that out of the way, create a file called whatsapp.py
in this directory and add the following code to it:
Before running this code, make sure you set the environment variables TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
with their respective values from your Twilio account credentials, which can be found in your Twilio Console. The Twilio Python library will automatically look at those values, so you can avoid hard coding them in your whatsapp.py
file! Here's a useful tutorial if you need help setting environment variables.
Finally, in your terminal run the following command to run this code to send yourself a picture of an owl on WhatsApp:
Check your messages and you should see something like this!
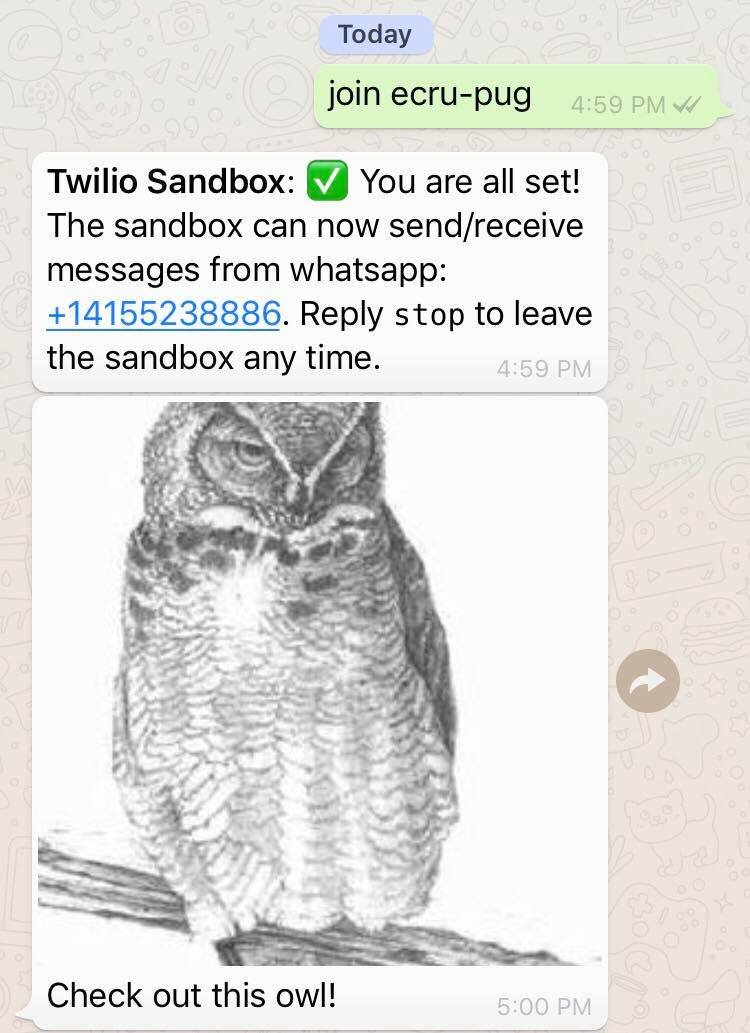
Keep in mind that you will have to use a pre-approved template if you want to message someone more than 24 hours after their last incoming message. And note that WhatsApp media messages don't support some of the file types that MMS does. For more information on file type support check out the FAQs.
What next?
You just successfully sent your first WhatsApp media message using Python, but what if you want to do this in Node.js or C#? We have other blog posts you can check out for that!
- Sending WhatsApp messages with Node.js
- Sending WhatsApp media messages with C#
- Sending WhatsApp messages with Java
- Sending WhatsApp messages with PHP
I’m looking forward to seeing what you build. Feel free to reach out and share your experiences or ask any questions.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.