Improving your pun game with Python, Rhymebrain and the Twilio API for WhatsApp
Time to read: 3 minutes
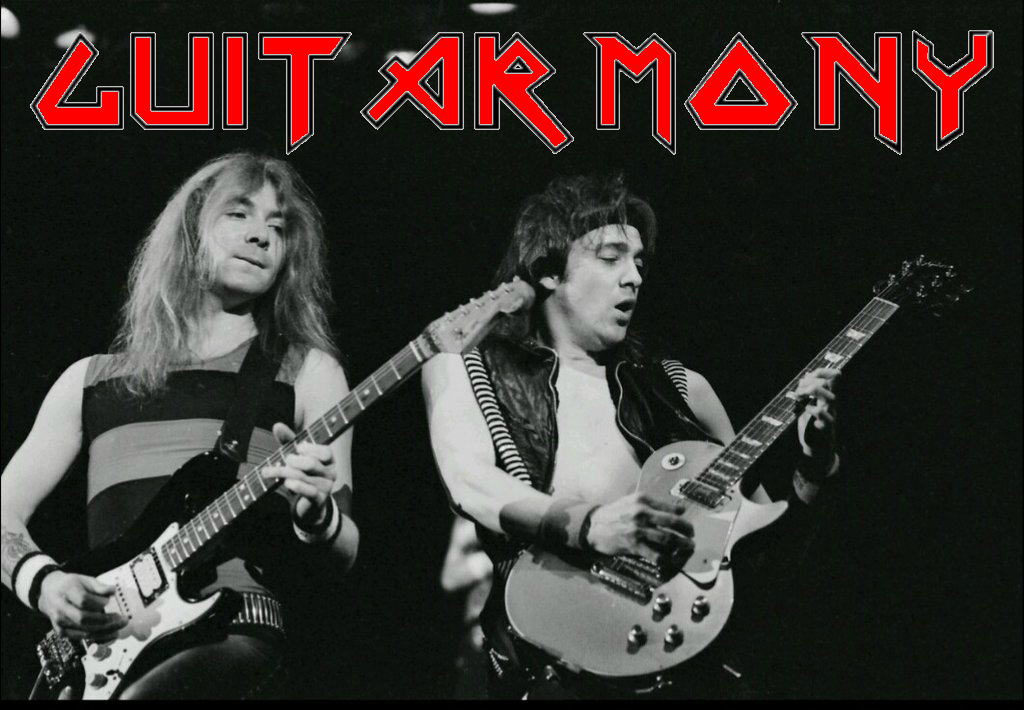
Puns are awesome and one easy way to come up with puns is to combine two different words. This is called a portmanteau. Let’s build a WhatsApp bot that gives you portmanteaus for certain words that you message it, so you can come up with better puns on the fly!
We’ll be using the Rhymebrain API for the portmanteaus and the Twilio API for WhatsApp to program our WhatsApp bot.
Setting up
To get started make sure you have:
- A Twilio account (sign up for a free Twilio account here)
- The WhatsApp Sandbox Channel.
- Follow these instructions to install the WhatsApp Sandbox Channel in your account.
- You will also need to connect your own WhatsApp account with the sandbox
- Python 3 installed, and your development environment set up.
Like with Twilio Programmable SMS we’ll need a webhook that will respond to incoming HTTP requests with every message sent to our WhatsApp bot. The webhook can then reply with TwiML to respond to messages.
We’ll be using the requests module to make HTTP requests to the Rhymebrain API, the Twilio module for generating TwiML, and Flask as our web framework to respond to incoming requests.
Install these requirements with the following command, preferably in a virtual environment:
Working with the Rhymebrain API
The Rhymebrain API is amazing for developers wishing to moonlight as poets, such as my friend Bilal who first showed me this inspiring literary-assistance technology. You can interact with the API by sending a simple GET
request. As its name implies, you can use the API to get rhymes and word information, but for this project we are going to be getting portmanteaus of words.
Create a file in the directory where you want your code to live called portmanteau.py
. Insert the following code:
This code contains a function that takes a word and uses that to make a request to Rhymebrain which will return a text-message friendly string containing all the portmanteaus of that word. Perfect for coming up with puns!
If you want to test this code, try opening your Python shell and running the following code:
You should see a bunch of word combinations. My favorites are Guitarmony and Guitargument. I imagine “Guitargument” is a great word to describe a shredding guitar solo duel!
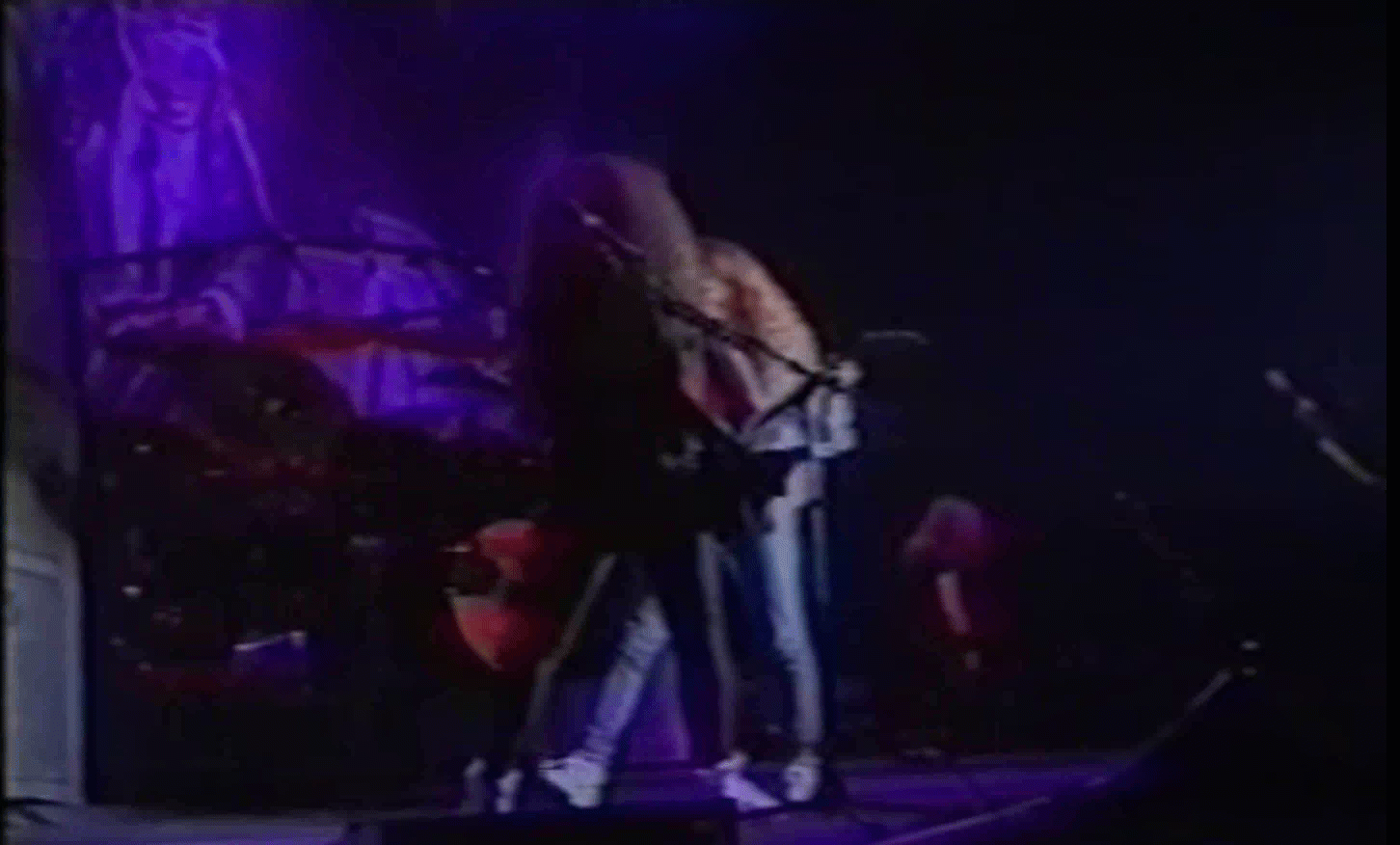
Jamming with Whatsapp
Before being able to give Twilio a URL to send Whatsapp requests to, we will need a web app to respond to those requests. For this, we’ll create a Flask app that uses the portmanteau module we just wrote.
Create a new file in the same directory called app.py
, and insert the following code:
This code is identical to code that could respond to an incoming text message to a Twilio number. The only difference is where the message is coming from. If you want to read more about how this works conceptually, you can check out this blog post.
In the /whatsapp
route, we are grabbing the first word from the message we receive and sending that to the get_portmanteaus
function to get a response string.
Now run your Python app by entering the following terminal command in the same directory as your code:
Your Flask app will need to be visible from the Internet for Twilio to send requests to it. We will use ngrok for this, which you’ll have to install if you don’t have it. In your terminal run the following command:
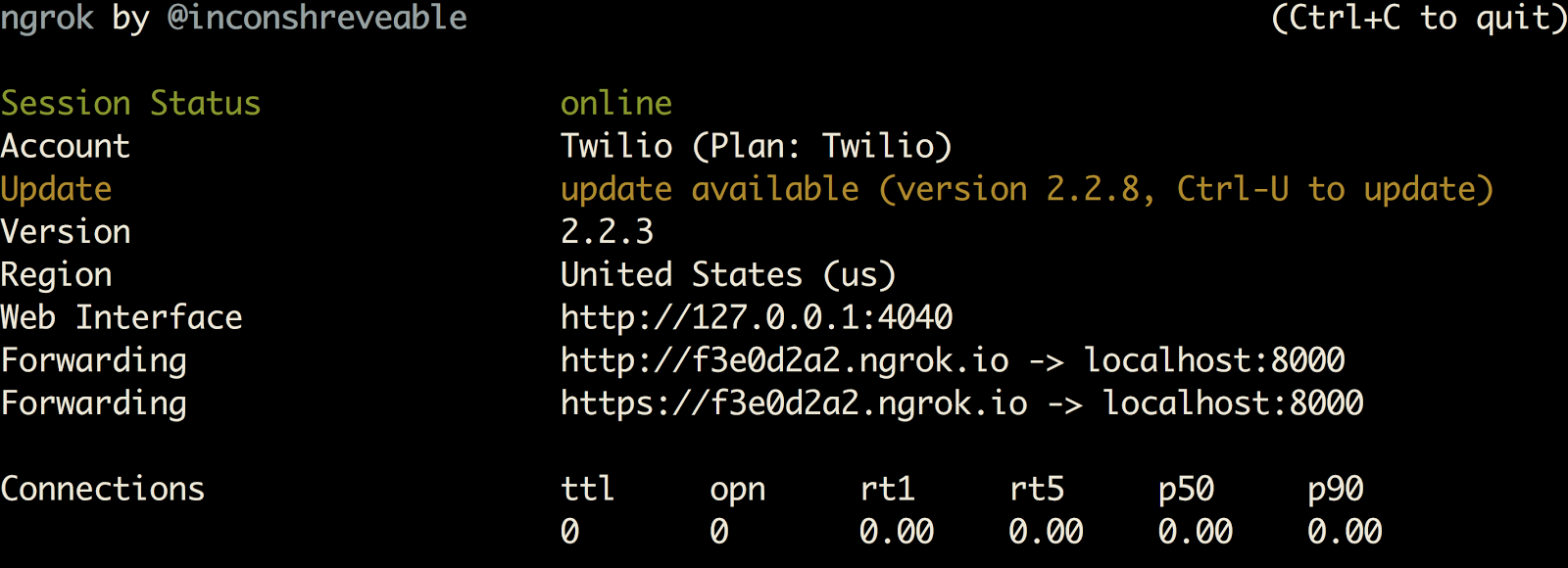
This provides us with a publicly accessible URL to the Flask app. Configure your Whatsapp bot as seen in this image by adding your ngrok URL with a /whatsapp
route appended to the “A MESSAGE COMES IN” section:
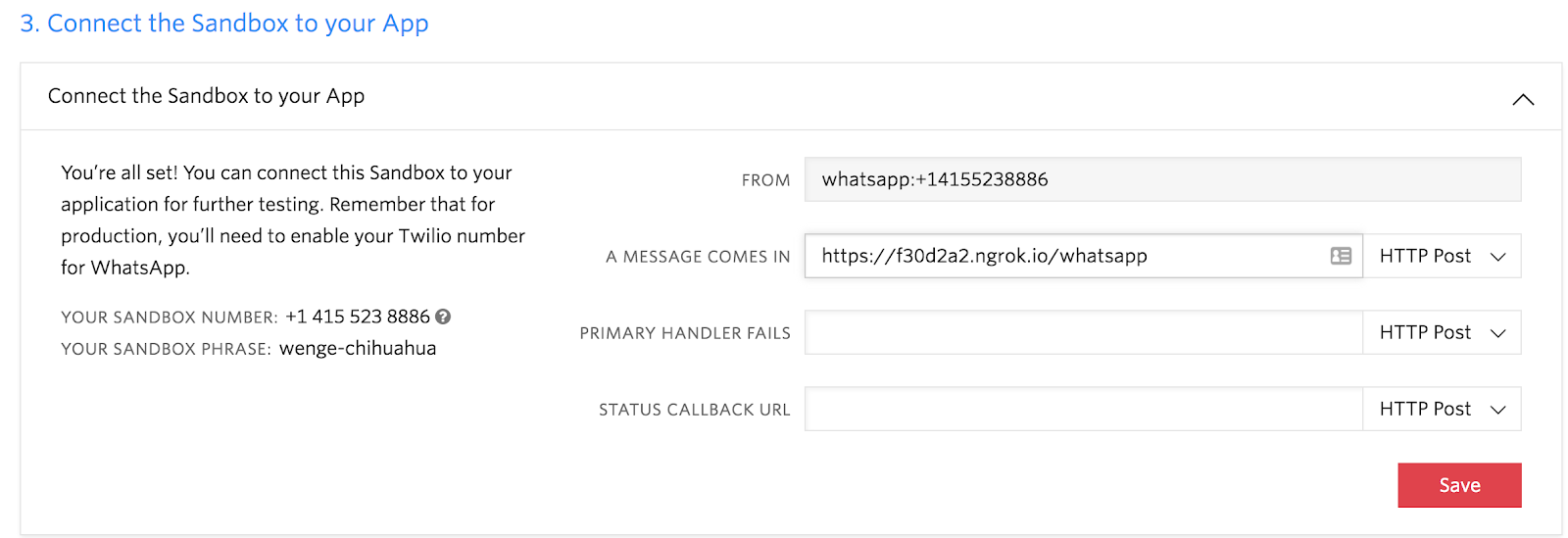
Save the changes and open Whatsapp to send a text message to your Sandbox with a word of your choice. You should get some pretty cool portmanteaus!
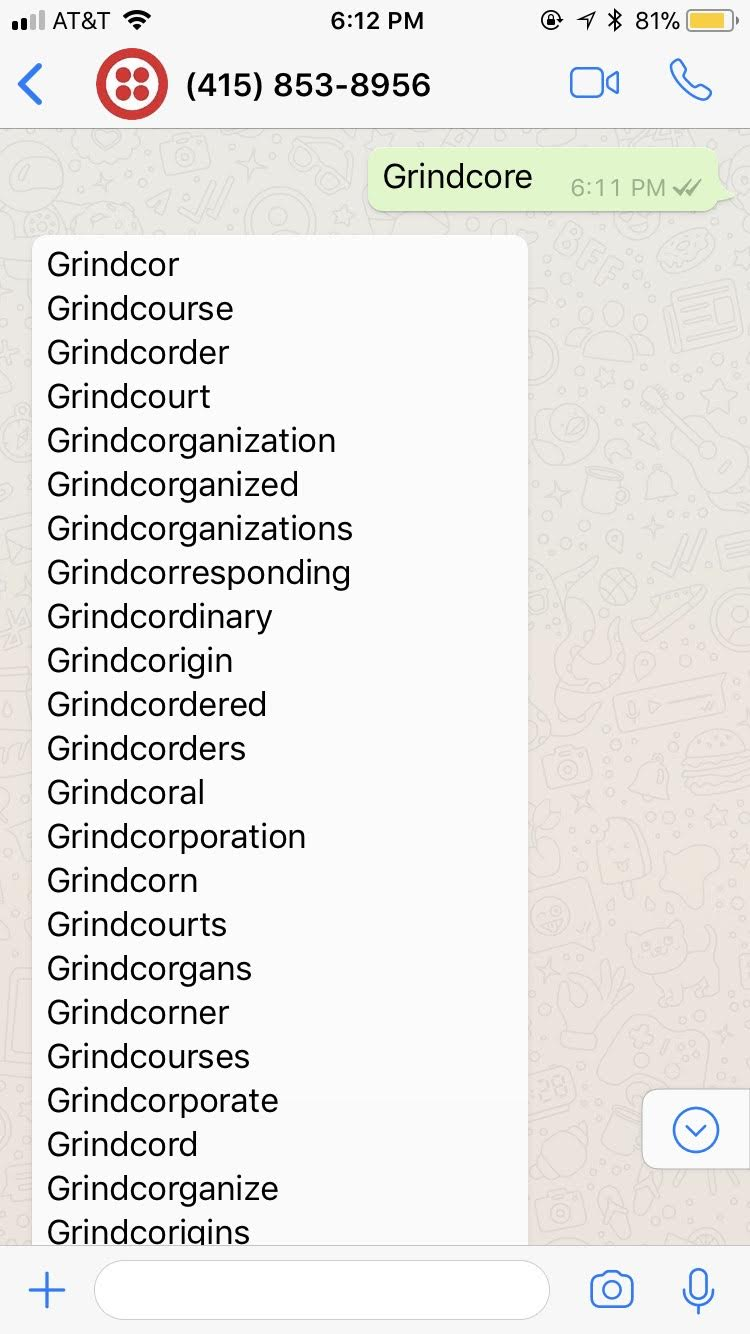
What if I want to bring this wonderful poetry to other channels?
The cool thing about using WhatsApp with Twilio is that the code for responding to other channels like regular SMS is identical! You can use this same code to respond to an SMS sent to a Twilio number just by using your web app’s URL as the webhook request for that.
If you want to learn more about Twilio & WhatsApp check out the following resources:
- Learn how to send a message to WhatsApp in the Docs
- Building an npm search bot with WhatsApp and Twilio
- Building an emoji translation bot with WhatsApp
If you have any other cool ideas, feel free to reach out and let me know or ask any questions:
- Email: Sagnew@twilio.com
- Twitter: @Sagnewshreds
- GitHub: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.