Pokemon Faux: Create Fake Pokemon Go Screenshots with Python, Flask and Twilio MMS
Time to read:
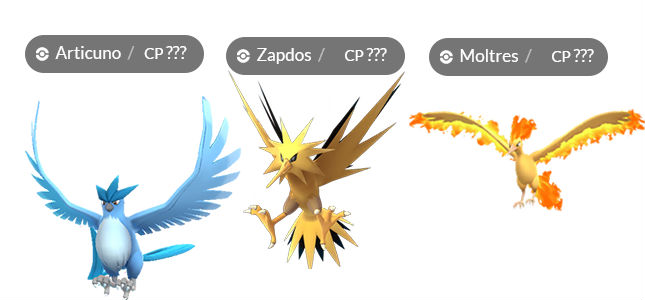
Pokemon Go is awesome and we all want to show off when we catch rare Pokemon. Let’s build a quick hack using Python and Twilio MMS that will allow you to trick your friends into thinking that you’ve encountered legendary Pokemon.
You can continue reading to find out how to build this, or try it out now by texting an image and the name of a legendary Pokemon to:
(646) 760-3289
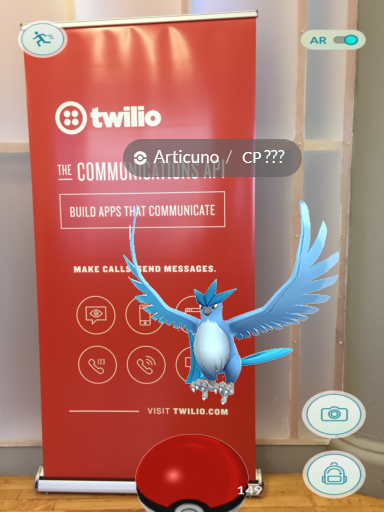
Getting started
Before diving into the code, you’ll first need to make sure you have the following:
- Python and pip installed on your machine
- A free Twilio account – sign up here
- The images we will need to use including screenshots of models of the legendary Pokemon and the overlay for the Pokemon encounter screen. Create a new folder called
pokemon-go-images
in the directory where you want your project to live and save them there.
The dependencies we are going to use will be:
- The Twilio Python library for generating TwiML to respond to incoming messages
- Pillow for image manipulation
- Flask as the web framework for our web application
- Requests for downloading images from text messages
Open your terminal and enter these commands, preferably in the safety of a virtual environment:
Overlaying images on top of each other
Let’s write some code to take the image we want to manipulate and overlay the Pokemon catching screen over it. We will use the Image module from PIL.
We need a function that takes a path to an image and the name of a Pokemon. Our function will resize the images to be compatible with each other, paste the overlay over the background image, paste the selected Pokemon on the image and then overwrite the original image with the new image.
Open a file called overlay.py
and add the following code (comments are included in-line to explain what is happening):
Try running it on your own image. This works best with images taken on phones, but let’s just see if it works for now. Open up your Python shell in the same directory as the file you just created and enter the following two lines:
Now open the new image and see if you are catching a Mewtwo on a train like I am:
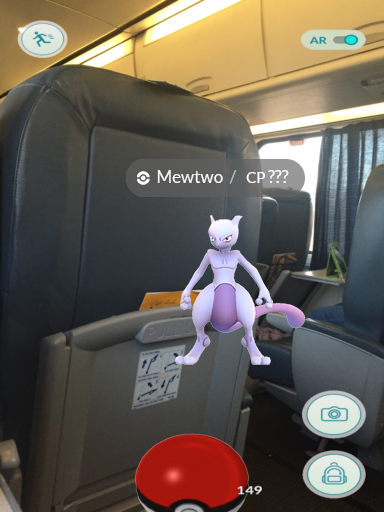
Responding to picture text messages
We need a Twilio phone number before we can respond to messages. You can buy a Twilio phone number here.
Now that we have the image manipulation taken care of, make a Flask app that receives picture messages and responds to them with a Pokemon being captured in that picture.
Open a file called app.py
in the same directory as before and add the following code:
The /sms
route responds to an incoming text message with some Twilio flavored XML called TwiML. Notice that the ‘NumMedia’ parameter is not zero, meaning we received an MMS. Twilio does not return an image itself, they return a URL to the image.
We create a string called filename using the MessageSid
parameter to maintain a unique identifier for each image. Then the program opens the file to write the content of Twilio’s image to the file.
The second route, /uploads/
handles the delivery of the message.media
TwiML using that URL to retrieve the new image.
Your Flask app will need to be visible from the internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it. In your terminal run the following command:
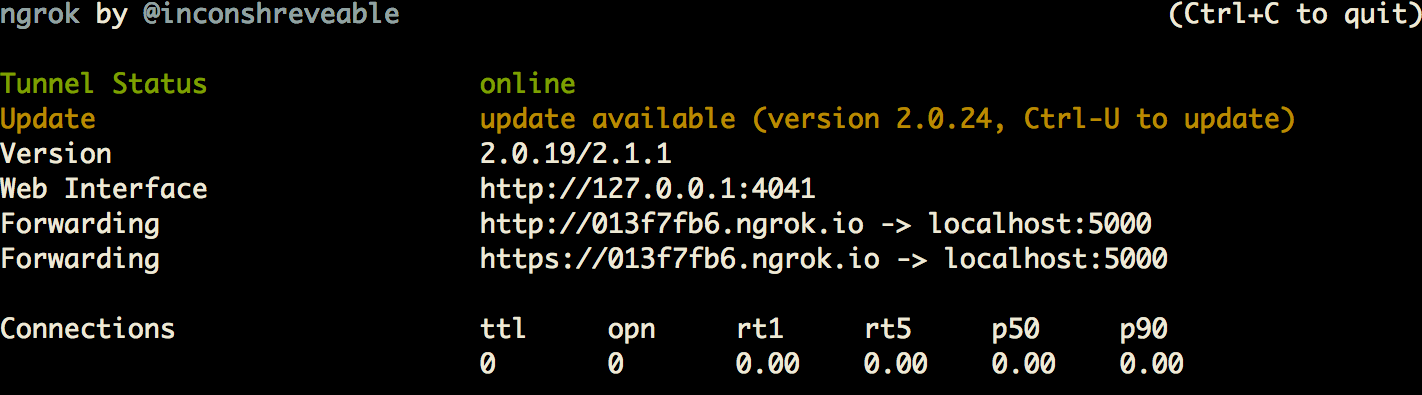
This provides us with a publicly accessible URL to the Flask app. Configure your phone number as seen in this image:
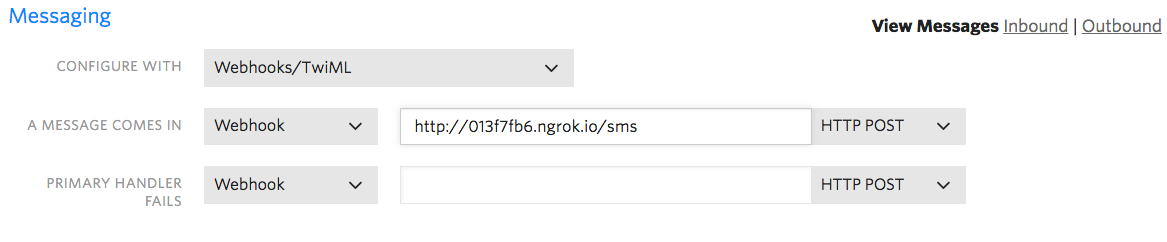
Before testing this out, make sure that you’ve changed the file paths and the URL to reflect your own.
Now try texting an image and the name of a legendary Pokemon to your newly configured Twilio number. Looks like we found a Zapdos in the Twilio New York office!
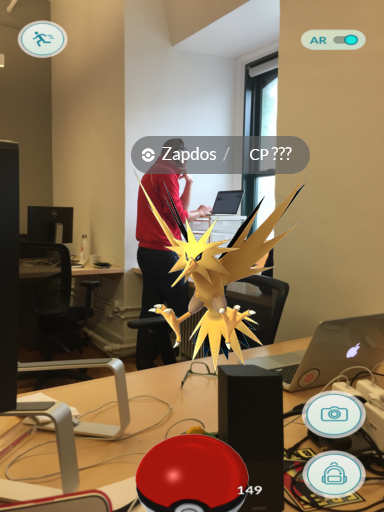
Time to catch ’em all!
Now that you can send messages to make it look like you are catching legendary Pokemon in any arbitrary picture, your quest to making your friends think you are a Pokemon master can truly begin.
The code for this project also lives on this GitHub repository.
For more Pokemon Go-related awesomeness, check out this post that will walk you through setting up SMS alerts when rare Pokemon are nearby.
Feel free to reach out if you have any questions or comments or just want to show off the cool stuff you’ve built.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Thanks to my good friend Shahan Akhter for helping out with image manipulation and tweaking the Python code to make the images look better.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.