Responding to Incoming Text Messages with Scala and Finatra
Time to read: 4 minutes
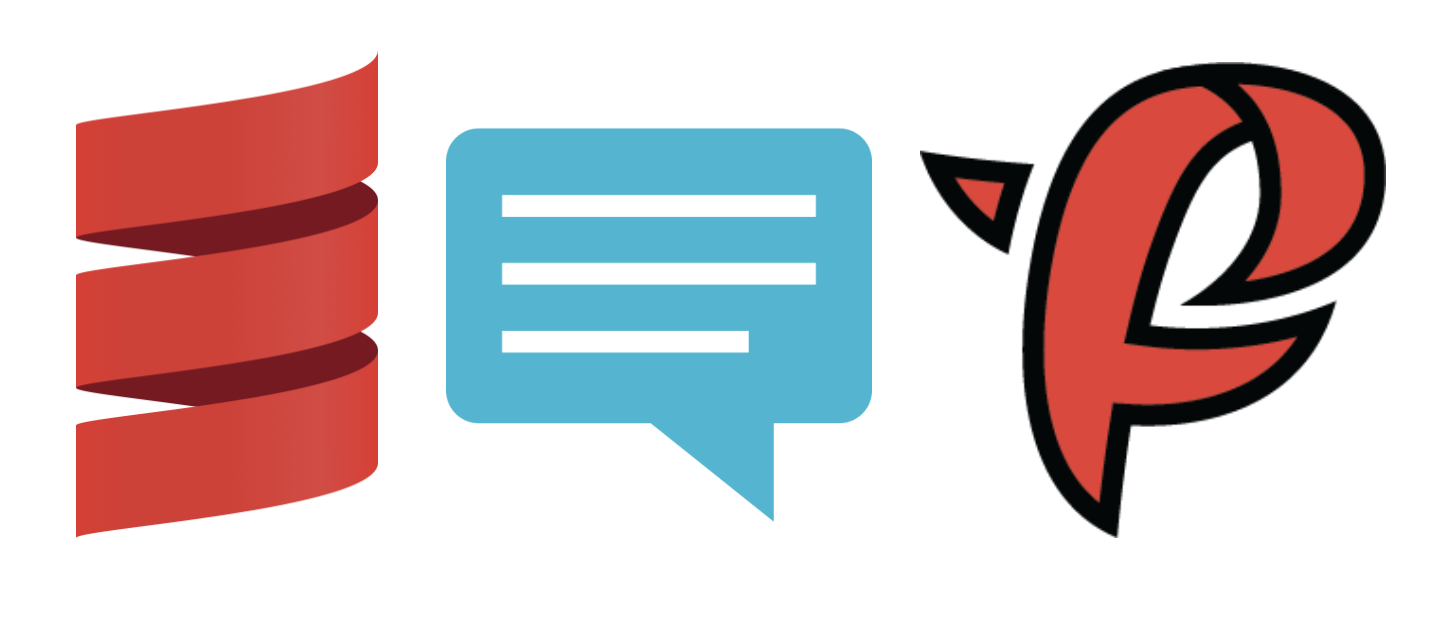
If you’ve ever wanted to create an SMS bot with Scala, you’re in the right place. Perhaps you’re looking for an interactive way to learn Scala or trying to build some automation into your customer interactions. Your SMS bot can be informational or just pure fun. We’ll be building a bot that will respond with your fictional bank balance. In this post, you will learn how to:
- Sign up for Twilio and get your first SMS-enabled Twilio phone number
- Set up your development environment to send and receive messages
- Receive inbound text messages
- Reply to incoming messages with an SMS
Installation
Let’s make sure we’re set up with the necessary dependencies.
Requirements:
- Java JDK 8
- SBT, I’m using version 0.13.6
- Ngrok
- A Twilio account (you can sign up for a free account if you don’t have one)
- A Twilio phone number that can send and receive SMS messages
Check that you have Java and SBT installed by typing the following in a terminal window:
Cloning the project template
We’ll use Giter8 templates to get started with our Finatra application. I created a template that will pull in the necessary dependencies from Finatra and Twilio. In a terminal window type:
You can name your project anything you like or press enter to accept the default name.
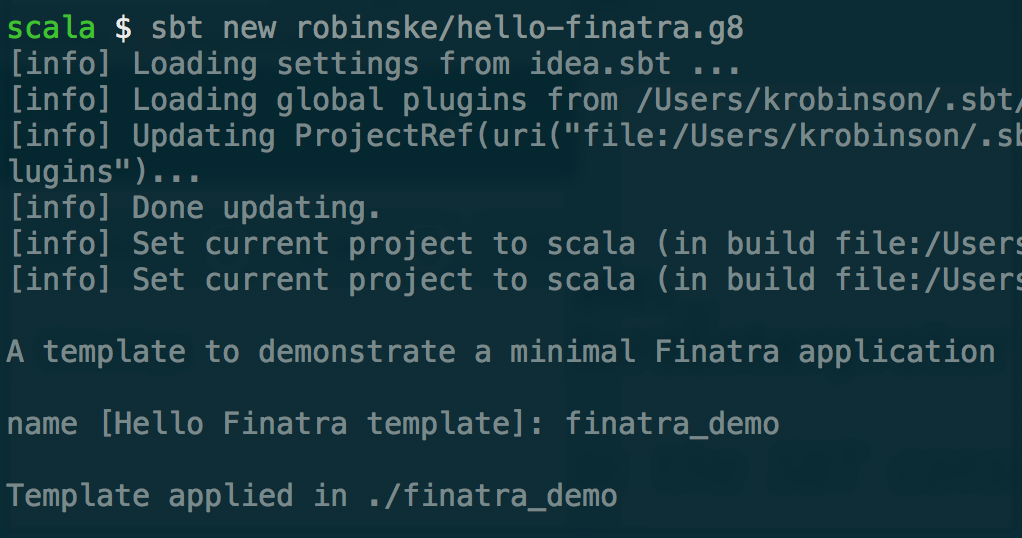
Running ‘Hello World’
Navigate into your directory, in my case:
Then run using SBT:
Finatra will start on port 8888:
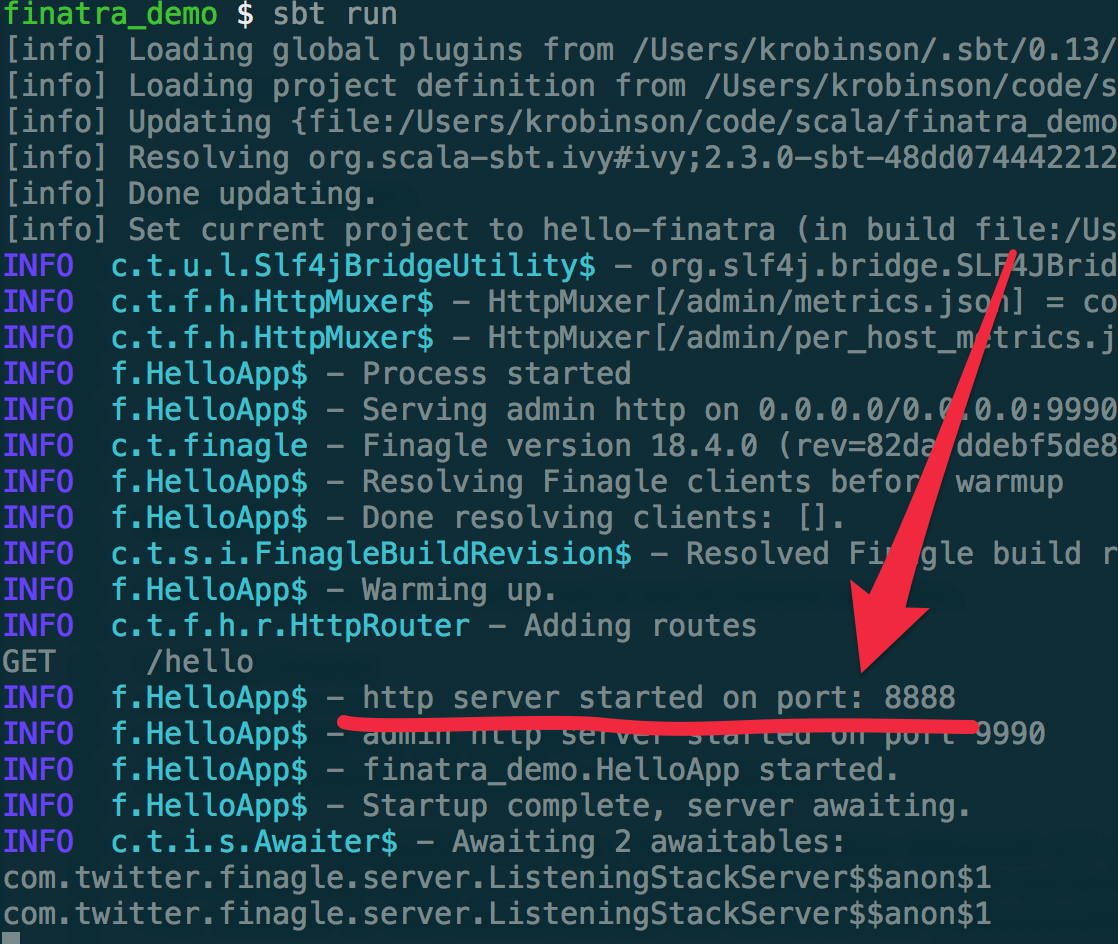
Navigate to http://localhost:8888/hello?name=YourName to see your application in action.
Receiving and Responding to Text Messages with Twilio
We can use our Finatra API to respond to incoming text messages using Twilio. Before you can respond to an SMS with Scala, you’ll need to sign up for a Twilio account or sign into your existing account and purchase an SMS-capable phone number if you don’t already have one.
Get Twilio talking to your application
We’re about to build a small Finatra application to receive incoming messages. Before we do that, we need to make sure that Twilio can reach your application. We’ll use Ngrok to do this. You can read more about how Ngrok works on our docs here. The tl;dr is that we need to let Twilio talk to our locally running application, and Ngrok provides a way to expose our localhost to the public internet.
Make sure your application is running then open a new terminal tab or window and start Ngrok with this command:
You should see output similar to this:
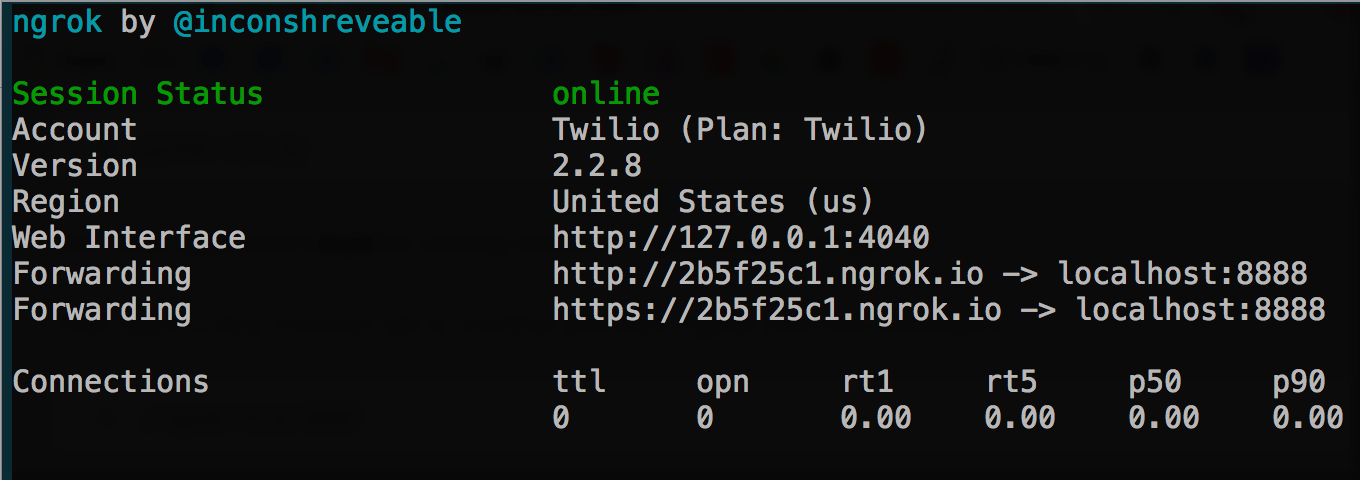
Copy your public URL from this output and paste it into your browser. Add the /hello route for something like http://2b5f25c1.ngrok.io/hello and you should see your Finatra application’s “Hello, World” message.
Receive and reply to inbound SMS messages with Finatra
We’ll have our Finatra app reply to incoming SMS messages with a thank you message to the sender. Twilio has a markup languages that is a set of XML instructions we call Twiml. Luckily for us, Scala has built in XML support so we can write our Twiml as pure XML. Open up Server.scala and update the code in the HelloController class to look like this code sample:
Save this file and restart your app with
Double-check that Ngrok is still running on your localhost port. Now Twilio will be able to find your application – but first, we need to tell Twilio where to look.
Configure your webhook URL
For Twilio to know where to look, you need to configure your Twilio phone number to call your webhook URL whenever a new message comes in.
- Log into Twilio.com and go to the Console’s Numbers page.
- Click on your SMS-enabled phone number.
- Find the Messaging section. The default “CONFIGURE WITH” is what you’ll need: “Webhooks/TwiML”.
- In the “A MESSAGE COMES IN” section, select “Webhook” and paste in your ngrok URL plus /sms.
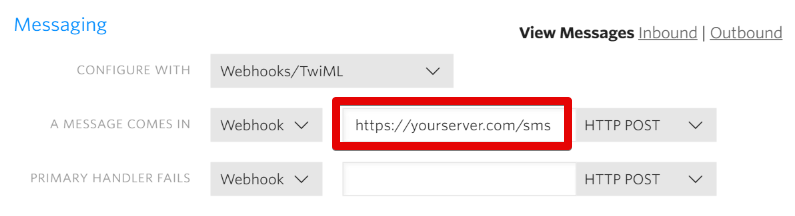
Save your changes – you’re ready!
Test your application
As long as your localhost and the Ngrok servers are up and running, we’re ready for the fun part – testing our new Finatra application!
Send a text message from your mobile phone to your Twilio phone number. You should see an HTTP request in your Ngrok console. Your Finatra app will process the text message, and you’ll get your response back as an SMS.
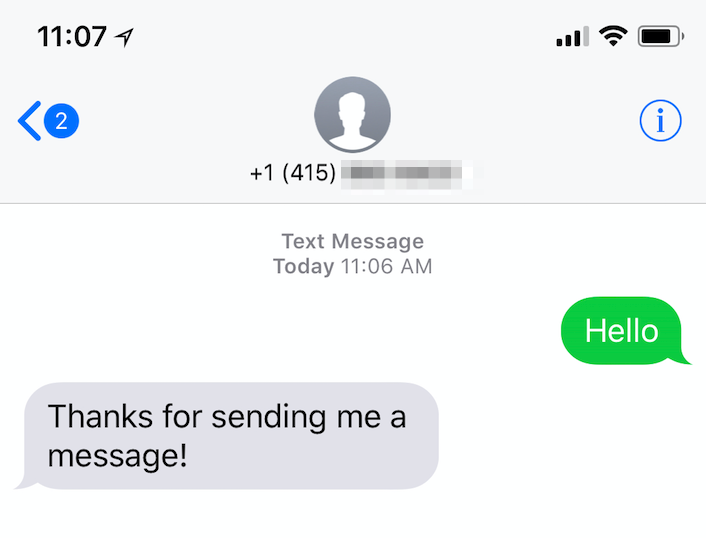
Show me the money
When someone sends an SMS to your Twilio phone number, Twilio makes an HTTP request to your server asking for instructions on what to do next. Once you receive the request, you can tell Twilio to reply with an SMS, kick off a phone call, store details about the SMS in your database, or trigger something else entirely – it’s all up to you!
We can use the incoming message to filter our responses. In this case, we want to respond to a Balance if someone requests it. We’re formatting our Balance as USD, but check out this post for more information on formatting international currency in Scala. Since our application isn’t hooked up to a database, we’ll just use a random number instead. Update your HelloController class to have the following code:
Restart your application with sbt run and send a text message to your Twilio number with the word ‘Balance’. You should see something like this:
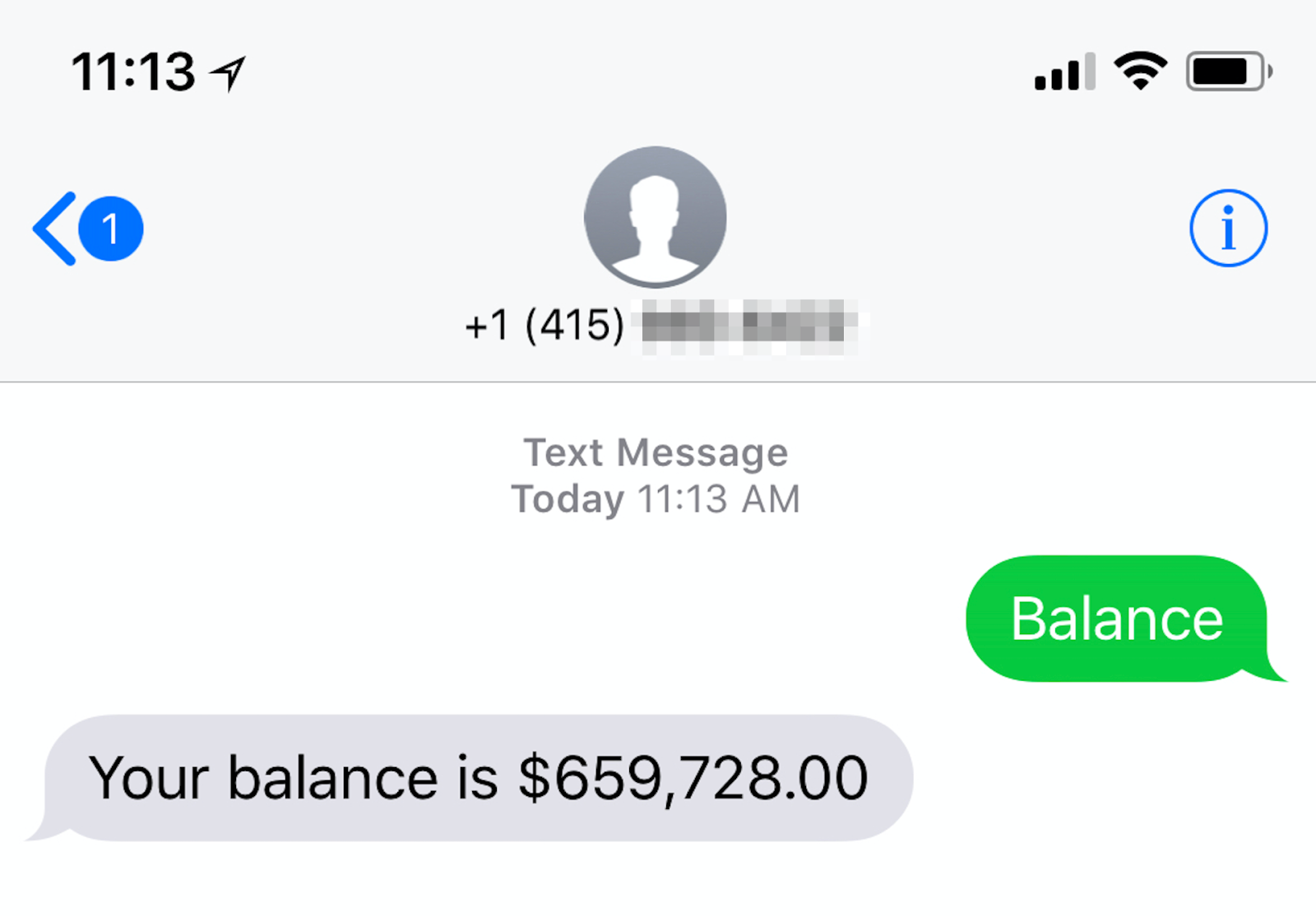
Congratulations, you’re rich! Filtering on the body of the incoming text message will allow you to build interactive bots. You could also filter on the incoming phone number to modify your response.
Where to next?
At Twilio we 😍interactive bots. I’ve had so much fun building Swansonisms and my Where is Kelley bot. Now that you know the basics of receiving and responding to SMS text messages with Scala, you might want to check out these resources:
- Send an SMS message from your application with Scala
- Dive into the API Reference documentation for Twilio SMS
- Learn how to build cool things with TwilioQuest, our interactive, self-paced game that teaches you how to Twilio.
The low overhead of SMS makes bots easy to share with friends and family and show off what you’ve built. If you have any questions or what to share your bot leave me a comment or find me on Twitter. I can’t wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.