Search for and buy Twilio phone numbers with Ruby
Time to read:
This post is part of Twilio’s archive and may contain outdated information. We’re always building something new, so be sure to check out our latest posts for the most up-to-date insights.
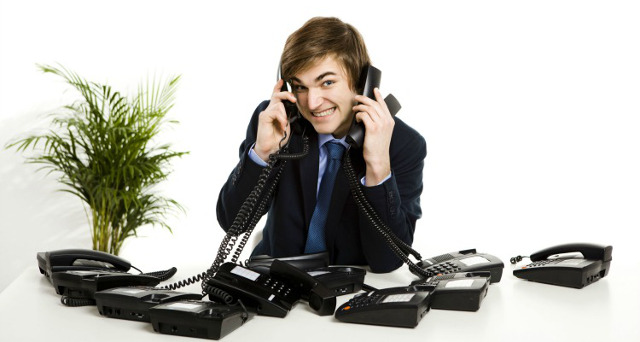
You’re in a hurry and you need a new phone number! All you have is your Twilio Account SID, Auth Token and a command line with Ruby installed. What do you do?
Don’t worry, just grab your keyboard, open your terminal and you’ll have that new number in no time.
Let’s buy a phone number
Make sure you have the twilio-ruby gem installed. This will make things a lot easier to call the REST API (though there are many ways to make an HTTP call with Ruby).
Install the Twilio gem
If you don’t have the gem yet, get it installed now. On the command line type:
Now that the gem is installed, we can get to work. For maximum speed we’re going to write all of our code in irb
, Ruby’s REPL. If you prefer, you can use pry instead.
Prepare your API client
Open up irb
and require the twilio-ruby gem. You can do this in one step with this line:
We need to create an API client that is authenticated to use the API with our credentials. You’ll need your Account SID and Auth Token (found in your Twilio console) for this bit.
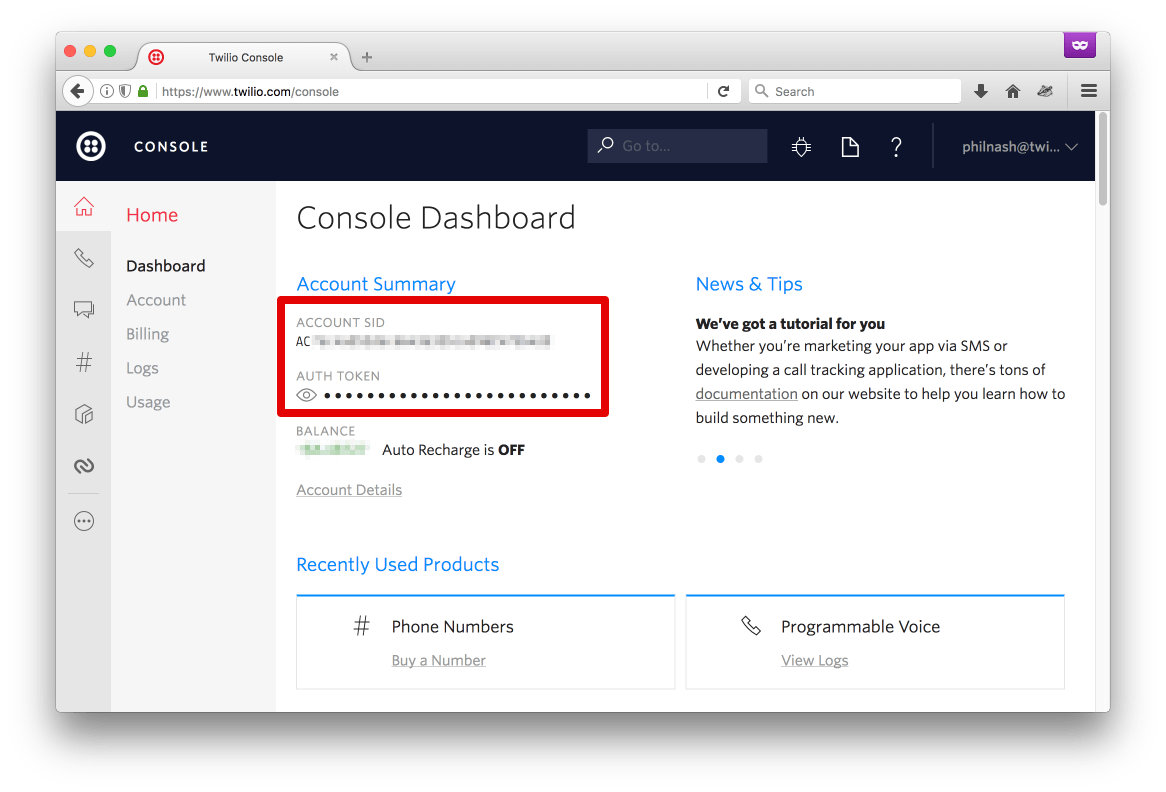
Create the client like so:
Search for the perfect number
Now that we have the authenticated client we can start searching for numbers to buy. We need to pick a country in which to search for numbers. Since I’m in the UK, I’m going to search for a nice local British number. If you want to search in a different country, substitute “GB” with the two letter country code that you want.
This has given us a list of AvailablePhoneNumberCountryContext::LocalInstance
objects, but we want to see the numbers themselves.
I got a list of numbers from Cardiff when I performed this search. I’m in London though, so let’s try to find some London numbers. We’ll do so by searching for numbers that contain “4420”.
We have bought our phone number!
The only thing is, it doesn’t do anything interesting yet. It’s going to need some URLs that Twilio will make requests to when the number receives an incoming call or SMS. The URLs need to return TwiML, a subset of XML, that tells Twilio what to do with the call or message.
Adding behaviour to the number with TwiML Bins
You can generate TwiML however you like, but for the purposes of this phone number I’m going to use TwiML Bins which let you edit and then host TwiML from your Twilio console. TwiML Bins are more powerful than hosting plain XML too as they support Mustache templates.
Open your console and create two TwiML Bins, one for voice and one for SMS. Here’s an example you can use for voice that uses TwiML’s <Say> to read out a message to the caller:
Here’s an example for SMS that echoes back the message you send to the number:
Save those TwiML Bins and grab the URLs. Update the phone number, adding a voice_url
and sms_url
parameter:
You have bought and configured a new number! Give it a call and you’ll hear the message from the TwiML Bin. Send it an SMS message and it will echo that message back to you.
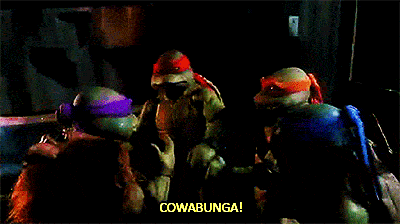
Numbers bought
That’s all you have to do to buy yourself a new Twilio number. It took us only 8 lines of Ruby!
Now you can use this in your applications to provide numbers for your users. This is particularly useful when you are doing phone number masking. You could also use this code to create local phone numbers in a call tracking campaign.
Let me know what you get up to with your new found ability to buy phone numbers whenever you want. Shoot me an email at @philnash or leave a comment below.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.