Send an SMS message During a Phone Call with C# and .NET Framework
Time to read: 6 minutes
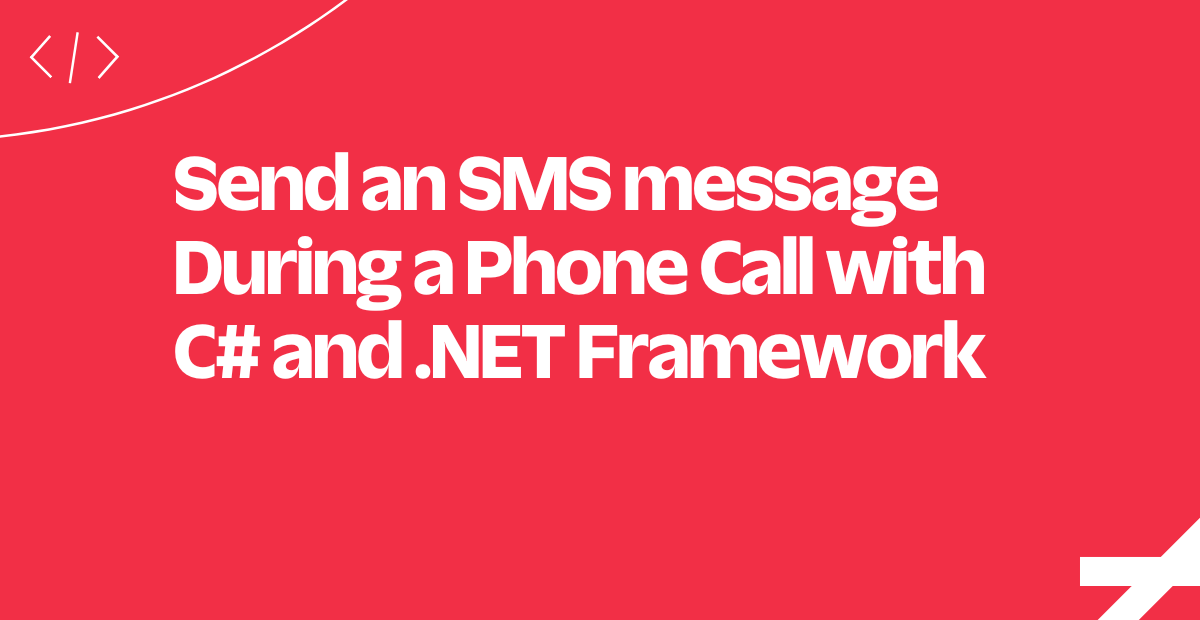
In this tutorial, we’ll show you how to use Twilio's Programmable Voice and SMS to send a text message during a phone call.
We’ll write a small C# web application that:
- Accepts an incoming phone call
- Says something to your caller
- Sends the caller a text message
The code we’re going to write uses the .NET Framework and Twilio’s C# and .NET Framework helper library.
Ready? Let’s get started!
Sign up for a Twilio account and get a phone number
Before you can receive phone calls and send messages, you’ll need to sign up for a Twilio account and buy a Twilio phone number.
If you don’t currently own a Twilio phone number with both SMS and Voice capabilities, you’ll need to buy one. After navigating to the Buy a Number page, check the "SMS" and "Voice" boxes and click "Search":
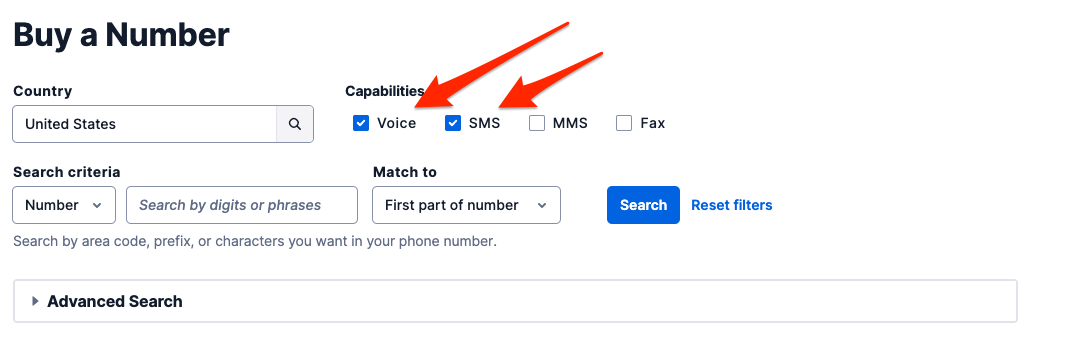
You’ll then see a list of available phone numbers that meet your criteria. Find a number that suits you and click "Buy" to add it to your account.
Create a .NET app that accepts incoming calls
We’ll be building a small .NET web application that accepts incoming calls and sends the caller an SMS. Let’s start by building out the code that receives the call and says something to the caller.
Set up your development environment
To download the code used in this tutorial and run it, you can clone this GitHub repository and follow the setup instructions in the README.
If you’d prefer to build this app from scratch, start by creating a new directory named twilio_calls
. Within that directory, create a new .NET Framework project, You can see how to do it here.
In the Visual Studio, select "Tools," "NuGet Package Manager," and "Package Manager Console" from the main menu and type the following command:
$ Install-Package Twilio.AspNet.Mvc -DependencyVersion HighestMinor
Handle incoming calls
Now that our environment is all set up we can create an empty controller named AnswerController
. We’ll create a small /answer
route that can say something to someone who calls our new Twilio phone number.
In the code sample above, we leveraged Twilio's C# library to create a VoiceResponse
that says some text to a caller. We can ignore the blurred lines for now: those will come into play later when we're ready to create an SMS from this call.
You can now run this .NET Framework application by clicking the play button in Visual Studio. This will open your browswer at the page http://127.0.0.1:<port>
. Note that by default .NET assigns a port dynamically to your project, so you should use the same port number every time we use the <port>
statement here.
You can check that your app is running by sending a POST request to the http://127.0.0.1:<port>/answer address, using tools like curl or Postman. You should see some text that says "Thanks for calling! We just sent you a text with a clue."
But how do we tell Twilio to use this response when someone calls our Twilio phone number?
Allow Twilio to talk to your .NET application
For Twilio to know how to handle incoming calls to your phone number, you’ll need to give this local application a publicly accessible URL. We recommend using ngrok.
If you’re new to ngrok, you can find more information here about how it works and why we recommend using it when developing locally.
Once you’ve downloaded ngrok, make sure your .NET application is running. Then, open a new terminal window and start ngrok:
$ ./ngrok http <port> -host-header="localhost:<port>"
Remember to replace the <port> statement to your correct port number.
You should see some output that tells you your public ngrok URL.
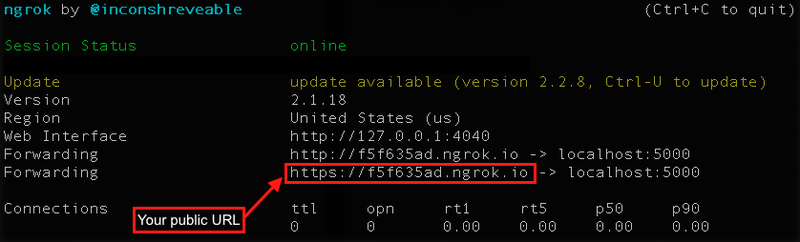
Now you can configure your Twilio phone number to use this app when someone calls you:
- Log in to twilio.com and go to the console's Phone Numbers page.
- Click on your voice-enabled phone number.
- Find the "Voice & Fax" section. Make sure the "Accept Incoming" selection is set to "Voice Calls." The default "Configure With" selection is what you’ll need: "Webhooks/TwiML...".
- In the "A Call Comes In" section, select "Webhook" and paste in the URL you want to use, appending your
/answer
route:
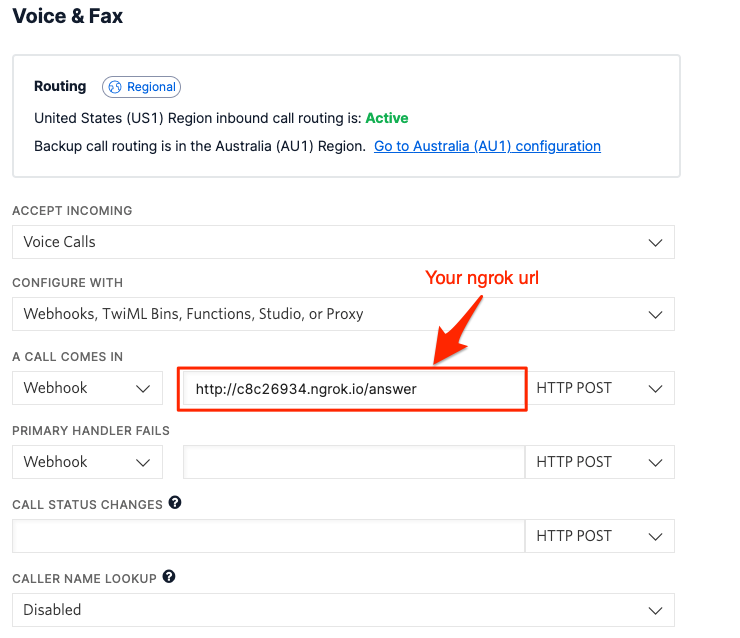
Save your changes. Now you're ready to test it out!
Call the Twilio phone number that you just configured. You should hear your message and the call will end.
Great! Next, we'll get some information about the caller so we can send them a follow-up SMS.
Get your caller's phone number from Twilio's request
When someone dials your Twilio phone number, Twilio sends some extra data in its request to your application.
While Twilio sends a lot of data with each inbound call, the two pieces of information we need are:
- Our incoming caller’s phone number:
From
- Our Twilio phone number:
To
To send our caller an SMS, we'll need to access that To
and From
information. Update your AnswerController
controller to include the following:
var caller = bodyData.From;
var twilioNumber = bodyData.To;
The code should now look like this:
Getting the Twilio phone number from the request allows us to connect this app to multiple phone numbers and route our responses appropriately.
Now that we have our Twilio phone number and our caller’s phone number, we can send our SMS reply!
Send an SMS to your caller from your Twilio number
Now we can create a new method in our application that sends our caller a message with Twilio's SMS API. To do that, we'll first need to include our Twilio authentication information.
Manage your secret keys
To send an SMS via the API, you’ll need to include the Account SID and authentication token for your Twilio account.
However, we don’t want to expose this information to anyone, so we’ll store that information using the project's Web.config
file. In it, change the TwilioAccountSid
and TwilioAuthToken
keys to your own information:
<add key="TwilioAccountSid" value="ACXXXXXXXXXXXXXXXXXX" />
<add key="TwilioAuthToken" value="your_auth_token" />
You can find your unique Account SID and Auth Token in your Twilio console. Replace the placeholder values with your SID and token and save the file.
Now your application can safely access your Twilio authentication information.
Programmatically send an SMS from the call
Now it’s time to send a message to our caller!
Update your AnswerController
file to include a SendSmsAsync
method that sends the caller a clue:
Now, update the AnswerController
route to execute this new SendSmsAsync
function. We’ll pass along the From
and To
values found in the initial request.
Save your file, run your app, and double check that ngrok is still running. If you restart ngrok, you’ll need to reset your webhook for your Twilio phone number via the console.
Try calling your Twilio phone number again. Now you should hear your updated message and get an SMS with a super-secret password!
What about landlines?
This app doesn’t know if our caller is dialing from a phone that accepts SMS or not. What happens if someone calls from a landline and can’t receive an SMS?
Twilio handles an attempt to send an SMS to a landline differently depending on the location of the SMS recipient.
If you try to send an SMS to a landline in the US, Canada, or the UK, Twilio will not check if the number is a landline first. Instead, it will attempt to send the message to the carrier for delivery. Some carriers (especially those in the UK) will convert the SMS to a text-to-speech message via a voice call.
If your recipient is located elsewhere, the Twilio REST API will throw Error 21614, and the message will not appear in your logs.
To better handle exceptions on a call from someone who used a landline to dial our Twilio number, we’ll build some error handling right into our app:
With this exception handling, our caller will still hear our voice response but won’t receive the text.
In our sample app, we’re printing the error message and gracefully exiting. If you were running this app in production, you could handle this exception any way you like: you might track these occurrences in your application logs or spin up a response voice call to your caller.
What's next?
Now that you know how to leverage both Twilio’s Voice and SMS APIs to send a text message when someone calls your Twilio number, you may want to go deeper:
- Take this app a step further by tracking the delivery status of your messages
- Check out our in-depth API reference for Voice and SMS
- Learn how to create conference calls with C#
- Learn how to create true SMS conversations with C#
We can’t wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.