Send vCard with Ruby and Twilio
Time to read:
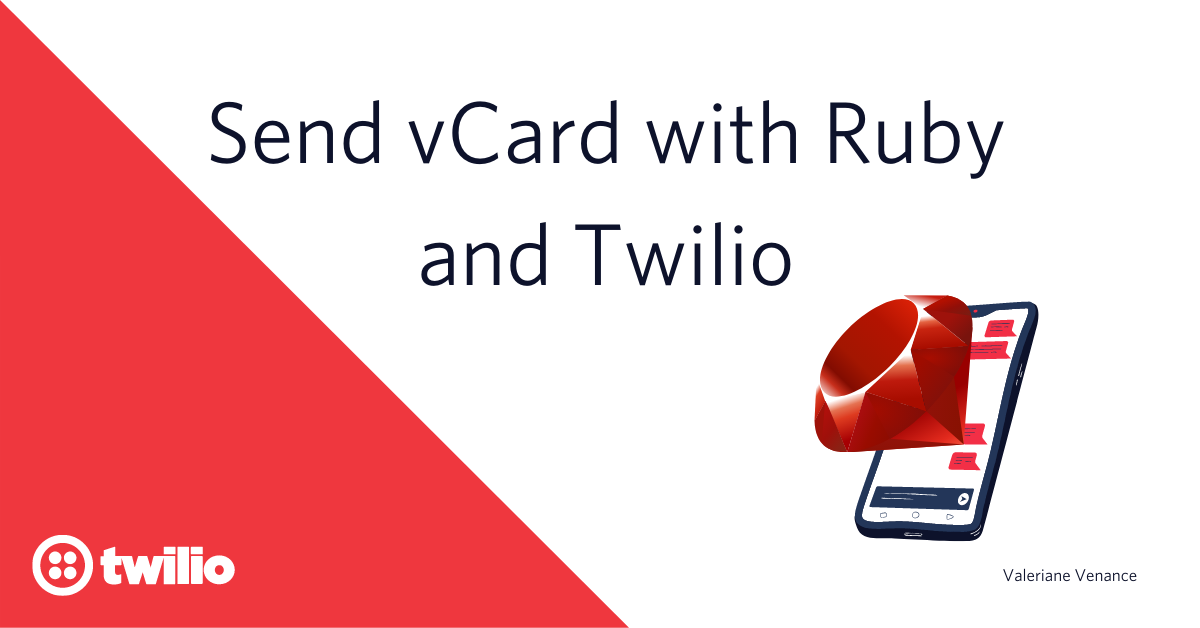
Have you ever sent contact information from a phone to another ? If so, you already have encountered vCard, the standardized format for Virtual Contact Files. These .vcf files are super helpful for contact imports.
In this blog post we will create a vCard with Ruby and send it to our phones with Twilio Programmable SMS. By the end of this tutorial you will have a Ruby script that generates vCard files and another one that sends vCards to your contacts.
I will generate my vCard and send it to my personal phone number. You can add me to your contacts by using your phone number or create your own vCard and send it to whoever you want.
Requirements
To follow along with me, you will need:
- A Twilio account
- A Twilio phone number (we will go through this step together if you need one)
- Ruby’s latest version installed on your machine (I use rvm and recommend you to use a manager but this is not mandatory)
Getting started
Create a new folder vcard-project
in your computer and keep the path to this folder somewhere, we will need it later.
Installing dependencies
We will need the following gems:
- bundler for avoiding permissions error
- vCardigan to generate the vCard
- twilio-ruby for the SMS part
First let’s install bundler:
OSX / Linux
Windows
open a command prompt with administrative rights, and run
Let’s create a Gemfile
in our vcard-project
folder and fill it with
And install the gems with
Generate the Vcard
At the root of your project’s folder, create the file vcard.rb
and open it with your favorite text editor.
vCard creation code
In vcard.rb
add the code below. This code will add me as a contact but feel free to change it with your own information. There are tons of fields you can add to a vCard, here we just cover a few but the full list can be found here.
This code creates a version 4.0 (current) formatted vCard with my full name, phone number, email and their types, and an extra link to all the blog posts I ever wrote on the Twilio blog.
Generate the .vcf file
For this we will use the File default library from Ruby. We need to create a new .vcf
file in our computer, let’s do it now, under the code we have written before:
This creates a new file named vcard.vcf
in the current directory, writes the content of the vcard in it and closes the file.
To generate the .vcf file on your computer, run
If you list the files in your current directory you should see a vcard.vcf
file next to vcard.rb
.
Take note of the path, we will need it right after.
Serve the vCard
Head to Assets in the Twilio Console. Click the blue ‘+’ button and add the vCard from your computer to your Twilio hosted assets. Leave the asset public, you want to share your contacts with the outside world !
Once the upload is complete, you will see a URL for this new asset. Save it for later.
Get a Twilio phone number
From the Twilio console's "Buy a Number" page search for a number with SMS capabilities.
Select the phone number you like and buy it. Then you will be able to give it a friendly name - I chose “vcard sender”.
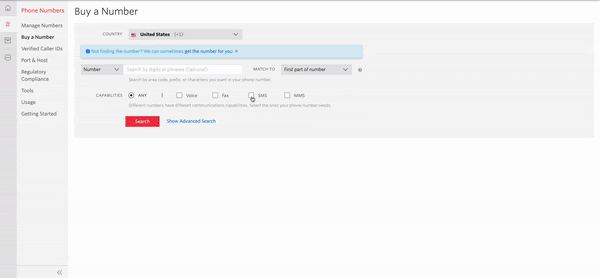
Note that number somewhere. While you’re in the console, go to the main dashboard and grab your Account SID and Auth Token, and add them to your env. Please use ACCOUNT_SID
and AUTH_TOKEN
as names so we’ll use the same you and I.
Add code for sending the vCard
Let’s create a new file called vcard_sender.rb
and fill it with:
- body: can be changed to any text you fancy
- from: must be your twilio phone number in the following format: +15555555555
- media_url: the link to the vCard hosted on Twilio assets
- to: it will be the phone number you want to send the vCard to, in the +15555555555 format. But we will get it from the ruby CLI, you will see how in the next section.
Testing out
Everything is now ready: our code will send an SMS to a phone number of our choice that we will specify. It can be yours so you will have my contacts ! Make sure to write the number in the +15555555555
format.
All’s left is to run the code. Simply do it with:
The recipient will receive an SMS containing your text message and the link to your vCard. Upon clicking the link, a file will download on their phone. Opening the link will add the new contact to their contacts list !
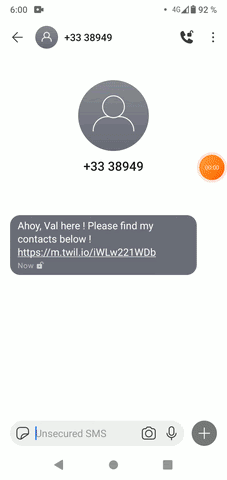
If you have kept the values I wrote, and added your phone number when running the final command, then you will get my business contacts ! Feel free to send an SMS or email if you’ve followed along !
By now you have learnt:
- How to generate vCards / .vcf files using Ruby
- How to host assets on Twilio
- How to send an SMS containing a file using Ruby and Twilio
- How to use arguments from the Ruby CLI in your code, through bundler !
If you’ve found this tutorial cool, you might want to check out some other Ruby tutorials I wrote (or click the link from my contact to see the full list!) :
- 5 ways to make HTTP requests in Ruby
- Building Voicemail with Twilio and Ruby
- Coding a Rails lookup app for my grandparents
I can't wait to see what you build !
Valériane Venance is a Developer Evangelist at Twilio. Leave her a message at vvenance@twilio.com or on Twitter if you’ve built something cool with Ruby. She’d love to hear about it!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.