SMS Sentiment Analysis in Python with Flask and the IBM Watson Twilio Add-on
Time to read: 3 minutes
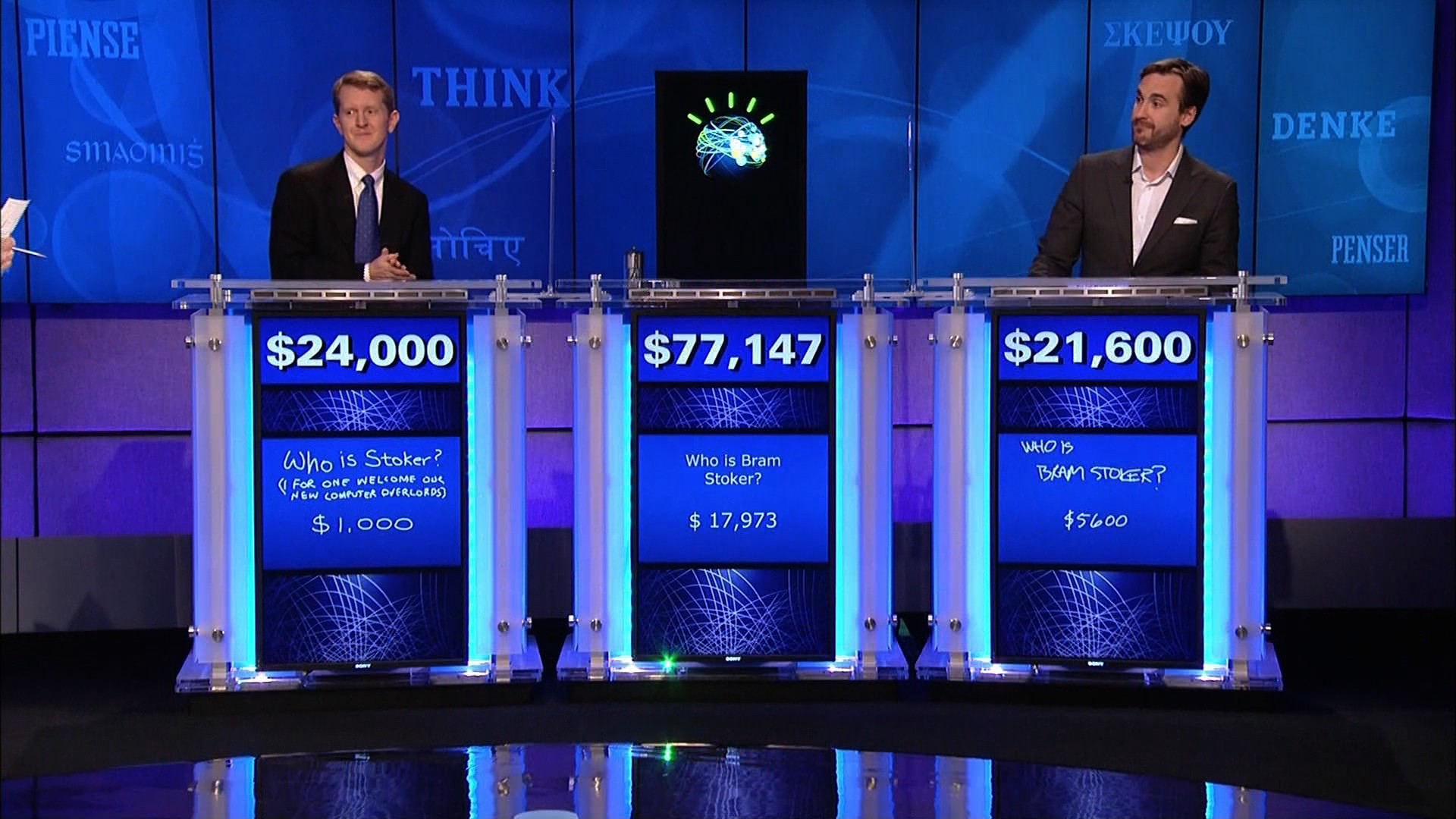
With Twilio’s new Add-ons marketplace developers can reap the benefits of third party APIs with the flip of a switch. The IBM Watson Message Sentiment Add-on adds sentiment analysis information to every SMS request sent to your web application.
Let’s build a Flask app that will determine the sentiment of text messages sent to your Twilio number.
Getting started
Before writing any code make sure you have:
- Python and pip installed.
- A Twilio Account – Sign up for free
- Your Account SID and Auth Token handy from the Twilio dashboard
Now let’s install the necessary third party libraries.
Run the following commands in your terminal to install Flask and the Twilio Python module, preferably in a virtual environment:
Responding to Incoming text messages
Before being able to respond to messages, you’ll need a Twilio phone number. You can buy a phone number here.
Now let’s create a web app that responds to text messages sent to this number. Open a file called app.py
and create a Flask app with one route:
When a text comes into Twilio, Twilio makes an HTTP request to your webapp and expects instructions back in the form of TwiML. The single route on this web app uses the Twilio Python library to generate and return TwiML that responds to the with another message.
Your Flask app will need to be visible from the Internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it. In your terminal run the following command:
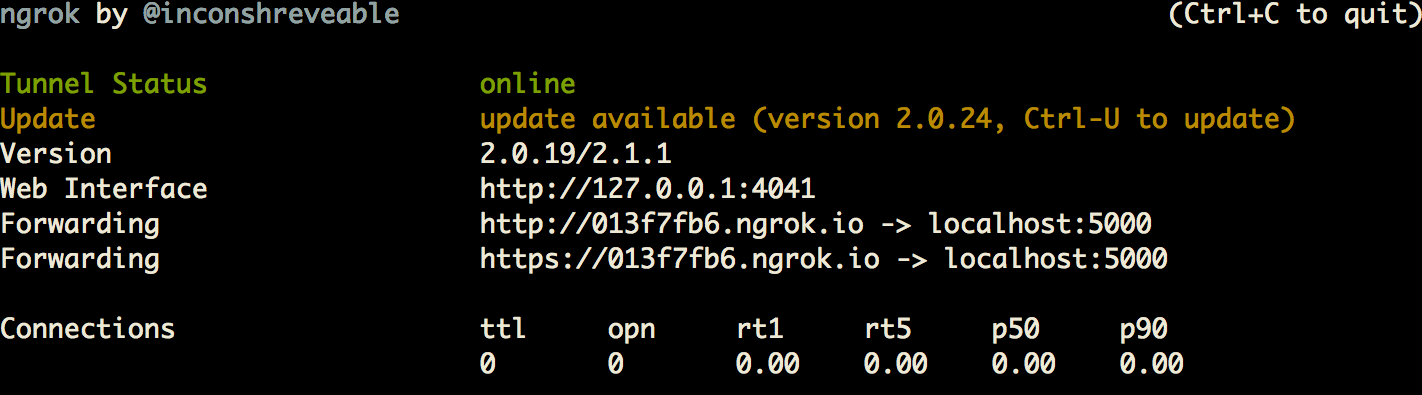
This provides us with a publicly accessible URL to the Flask app. Configure your phone number as seen in this image:
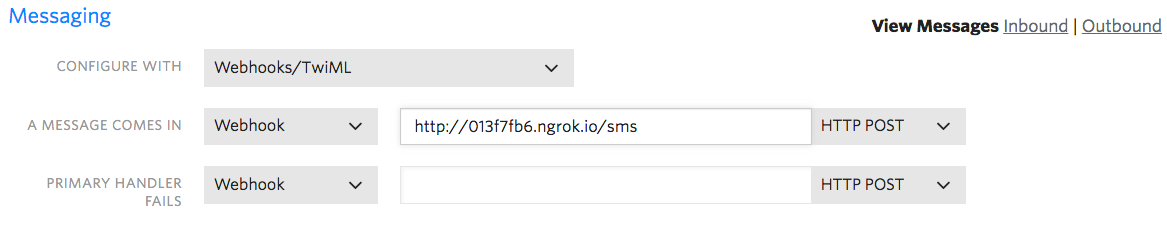
You are now ready to send a text message to your new Twilio number.
SMS Sentiment Analysis with IBM Watson
To add sentiment analysis to all of your , you just need to activate the IBM Watson Message Sentiment Add-on by clicking the “Install” button in your console.
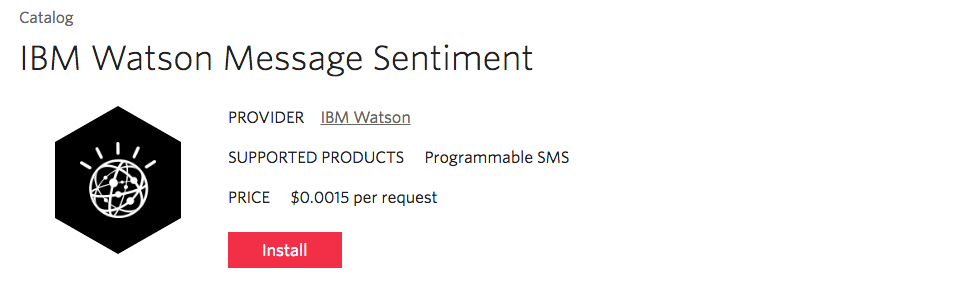
In the POST request sent to your application, there will be a new AddOns field, inside of which you can access the data from the IBM Watson API:
This AddOns
object will be JSON that you will need to parse. Open app.py
again and add the following line to the top of your code:
To access the sentiment of the incoming messages, you just need to add a few more lines of code. Open app.py
again and rewrite your /sms
route:
Now send some happy and sad text messages to your Twilio number!
Looking Ahead
With some configuration and a few extra lines of code, you can get the sentiment of all messages sent to your Twilio phone numbers, whether you’re using it to improve customer experience or to decide which GIFs to send to your users.
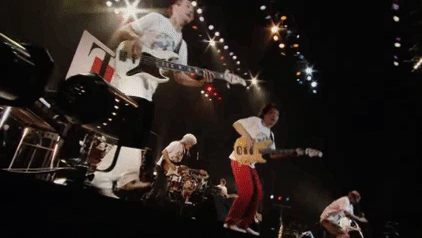
Feel free to reach out if you have any questions or comments or just want to show off the cool stuff you’ve built.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.