SMS Powered Star Wars Trivia with PHP and Twilio
Time to read:
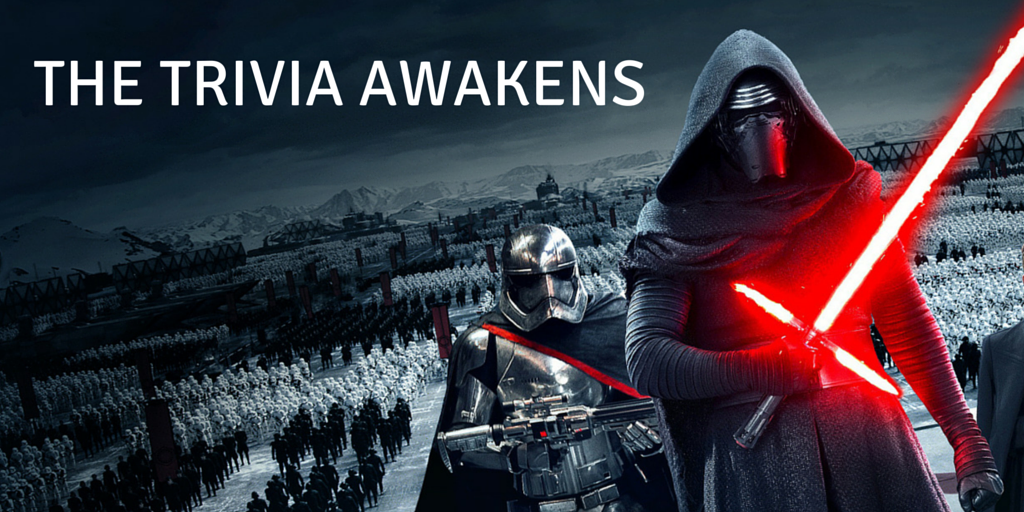
This weekend millions of moviegoers will stand in line and sit in theaters waiting for The Force Awakens. We thought we’d give them some spoiler-free entertainment in the form of SMS powered Star Wars trivia.
If you’d like to play, text your name to 74310.
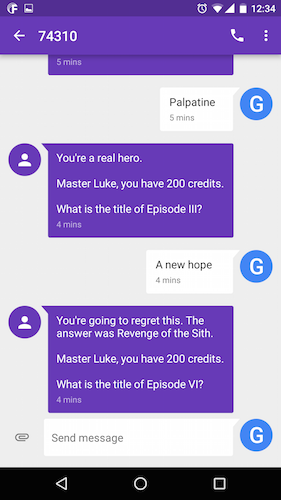
This app is powered by 40 lines of PHP and a text file full of questions. All you need to recreate it is a Twilio account and a server on which you can host a PHP file.
Reply to an SMS
Sign up for a free Twilio account and buy a phone number.
Scroll down to the messaging request URL and punch in the url of your PHP server, appended with trivia.php . It’ll look something like:
On your server, create trivia.php. Add some simple PHP and TwiML to respond to a text message:
Text your shiny new Twilio number and see what happens.
Now that we can reply to a text, let’s change that $message based on the state of the game.
Track the Conversation with Session Variables
Session variables are global variables that persist across requests.
That means that if we set $_SESSION['name'] when a new player texts in, that value will be there for the rest of our “conversation.” We’ll use session variables to track a player’s name, score and the correct answer to the question they were just asked. For more on tracking conversations with session variables, check out:
When a player texts your number, Twilio makes an HTTP POST request to your PHP file. The body of their message is stored passed via $_POST['Body'] . The first time we get a message, we’ll assume it’s the player’s name.
Below the header() , add a function that will initialize the name and score and then return a welcome message. We’ll also replace our previous message with the output of that function:
Text your name to your number and be welcomed by Yoda.
Ask a question from a text file
Create a file called questions.txt and fill it in with values from here.
Above $message = welcome() , add a function to:
- grab a line from questions.txt
- split it based on a | delimeter
- set a session variable with the correct answer
- return the question as a string
Below $message = welcome() , append the question:
Text your number again and you’ll get the welcome message along with a question.
Respond to an answer
Add a function to compare the message body to the correct answer. We’ll lowercase the strings first to make the comparison more forgiving:
Add an if statement to pull our pieces together:
Finally, tell them their score and ask them a question:
And that’s it. Forty lines of code and we’ve got ourselves an SMS based Trivia game.
May the Force Be With You
We’ve found SMS based trivia to be a fun way to engage a crowd on their mobile device without making them install an app. It appeals to people’s competitive nature, it’s super low friction and it’s easy to spin up for different occasions.
If you’re looking to improve upon what we just built, here are some suggestions:
- Add a public leaderboard. It will require a more permanent datastore, but this could be as simple as writing to a text file.
- Accept multiple correct answers. For instance, people get frustrated if they answer “7” and are told that the right answer is “seven”.
- Add picture questions. The TwiML will look like:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.