Using Bots To Route Customer Requests Based On Sentiment and Emotion
Time to read:
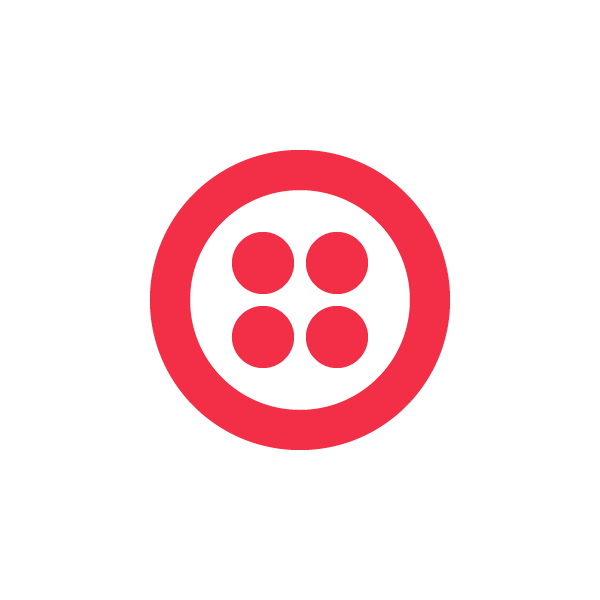
2016: the year where no strategy or vision pitch was complete without mentioning bots.
You can’t watch a tech keynote, scroll through your newsfeed, or be anywhere online without reading how bots are replacing apps, or replacing humans.
Assuming though for just a moment that we don’t turn our every human interaction, from wedding vows to childcare, into an AI driven chat based interaction… we have a question to answer: what is a realistic view of how companies could be using bots today? I’m particularly interested in the possibilities for using bots within a call center (But not as a replacement for humans – despite the hype we’re not a fully virtual society quite yet).
Sentiment driven routing
To explore these ideas, I built a call center prototype to look at ways to merge human and bot interaction together. I’ve been chewing on a few questions: Could you have customers chat with a bot first to better determine their intent, and even emotional state, and use that information to connect them to a better matched agent? Could you save the agent time by having the bot capture key information first and inform the agent when they take the interaction? What about handling self-service questions entirely automatically without ever passing the chat to a human agent?
My prototype handles inbound interactions coming in over both SMS and from Twilio’s new Facebook integration, all routed by TaskRouter. I also used Marketplace AddOns for details about the users texting in, along with Meya.ai for the bot platform, and Firebase. The code for this is all available in github, so you can follow along as we go through the architecture.
A customer messaging in ‘hey I could do with some help’ will get routed in a completely different way to someone messaging ‘You guys really suck I can’t believe you still haven’t fixed this’. And someone messaging ‘what are your opening hours?’ doesn’t need to be routed to an agent at all.
Customers messaging in first cause a task to be created in TaskRouter. The Task serves as the primary key for the entire lifecycle of the customer interaction. When the task is created, it sits in a queue waiting to be bot qualified, and my app server connects the messages back and forth with the bot platform. The customer first chats with a bot, which determines their intent and emotional state.
Sending messages related to unqualified tasks to the bot:
The Meya bot platform uses an easy scripting interface to storyboard the interactions. It starts by gathering the intent of the first message, and then transitions between different states from there depending on what’s said – the sequence will flow through to the next state unless you specify a transition to a different state.
When a bot gets an answer to the question ‘how can i help’, it uses Wit to determine sentiment. Wit is really easy to train from a data set of responses what the intent of the interaction is. The more you train it, the better is is at handling different variations of what the customer might say.
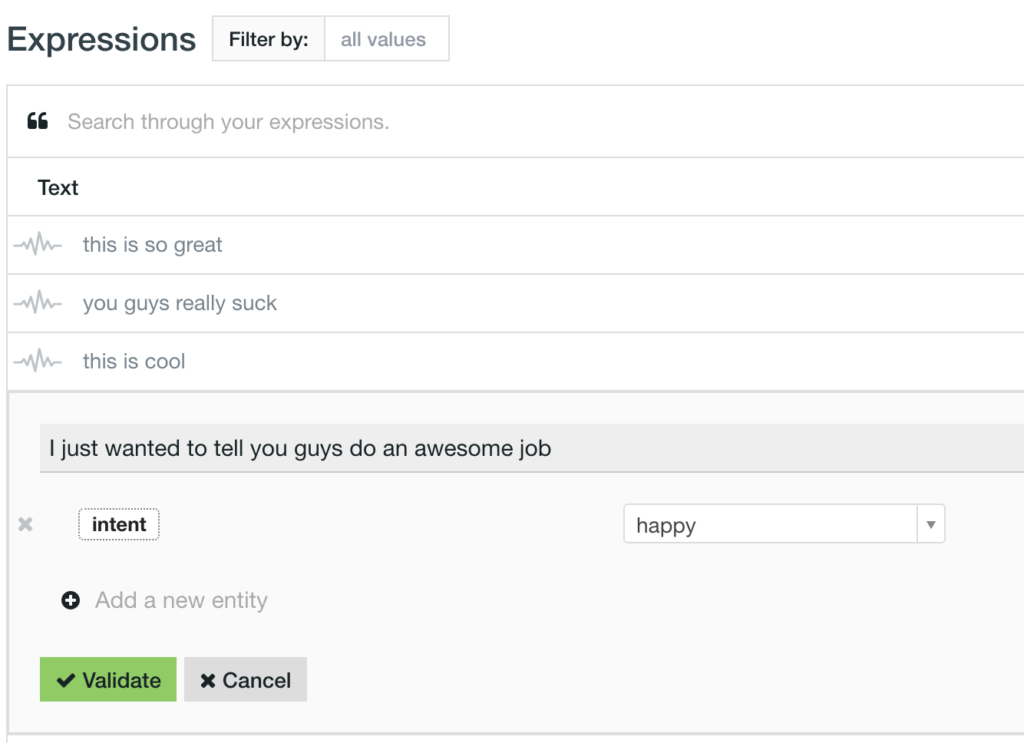
Once the bot has determined the intent, we’re ready to update the task attributes in TaskRouter to say that the task has been bot qualified, and mark their intent. In this case, it assigns each task to one of the following states: angry, happy, needs_help, problem, service_question, or unsure. To do this, I used the native Twilio integration available within the bot platform Meya.ai, so that my bot logic directly calls the update task API with the new attributes.
With the task updated, TaskRouter then moves the task into the appropriate queue so the best agents can handle the query. The gif below shows a realtime visualization of a TaskRouter workspace (code for this is also in github).
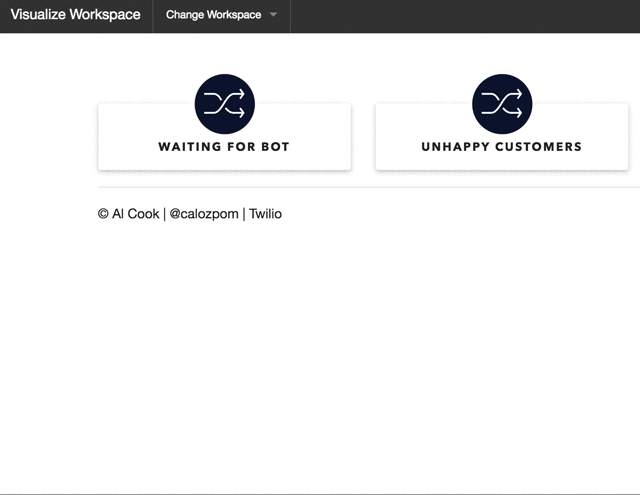
Once a task is bot qualified, and a suitable agent becomes available based on the determined intent, TaskRouter will push a reservation request to the agent. At this stage the agent gets to see all of the conversation history so they can get up to speed immediately and continue the conversation.
One of the key benefits of using messaging for customer service is that agents can handle multiple messaging interactions simultaneously. So I used the new multitasking feature of TaskRouter to be able to specify how many tasks the agent can handle concurrently. As you change capacity of the worker, they get additional task reservations:
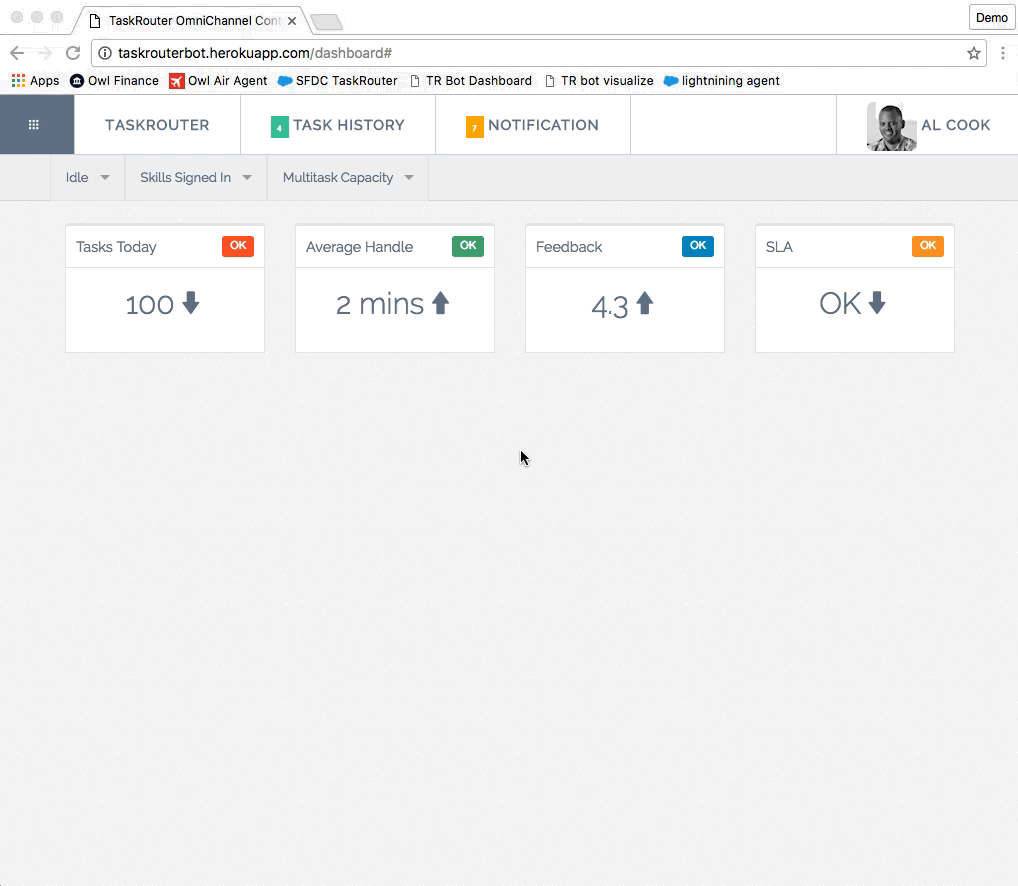
And finally I also used Marketplace AddOns to get the name and address of folks texting in:
So now we have a customer service solution where users can message in through SMS or Facebook, and based on what they say they need help with (and how they say it), they will get routed to the best qualified agent. The agent will have all the context of the conversation so far, and details about the customer from the Marketplace AddOns lookup of their phone number. The agent can choose how many customers they want to handle concurrently using the new multi-tasking capability of TaskRouter.
You can hear me talk more about this demo in this video from SIGNAL. All my code is available in github. The back end is all Node, the front end uses Foundation, and the bot platform used was meya.ai (who are phenomenally helpful, nice people). Disclaimer: I hadn’t coded anything in ten years, and had never used Node or any of these tools before, so in no way is this production code. Pull Requests welcome!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.