How to Validate a Phone Number in a Joomla Extension
Time to read: 4 minutes
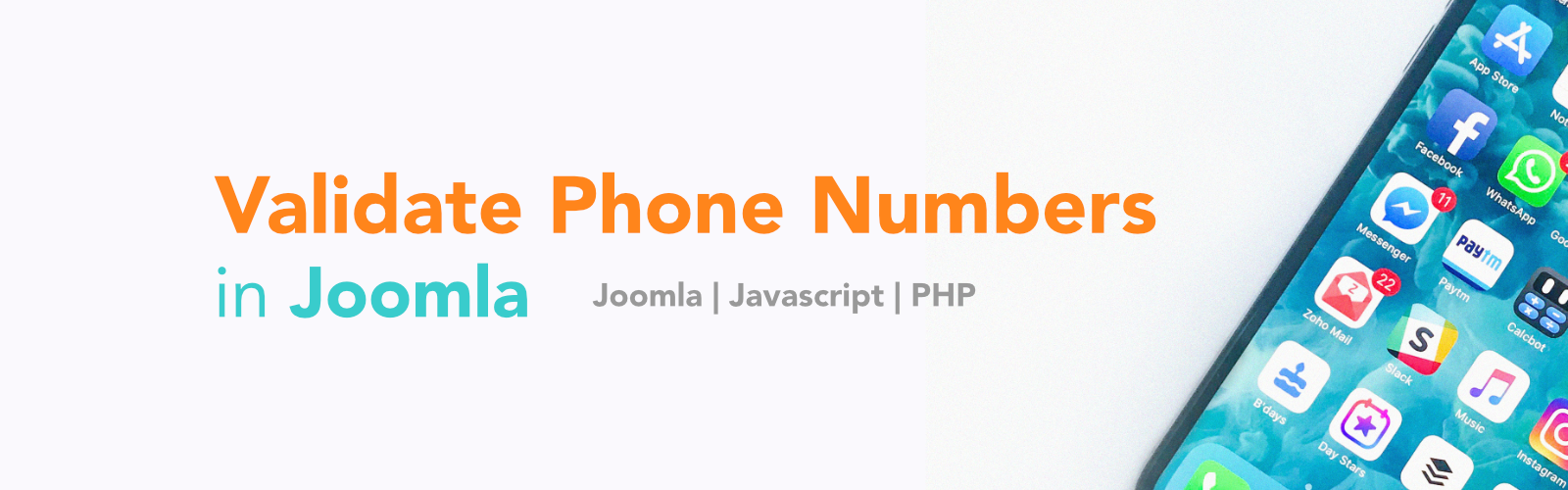
In the article Add a Click To Call Button & SMS Chat to Your Joomla Site, I presented a ready-to-use extension, XT Twilio for Joomla to implement a simple communication channel on a website. The basic extension sends SMS messages (or supports a Click2Call workflow) between users and the sales area. In the second article of this series, How to Customize a Joomla Extension for SMS Chat, I presented a case to add the client name field to the extension and personalize the SMS messaging.
In this tutorial, to tackle the complexity of handling international telephone numbers properly following the E.164 standard, I’m going to introduce the integration of an advanced JavaScript plugin for entering and validating international telephone numbers, jackocnr/intl-tel-input. The plugin adds a flag dropdown to any input, detects the user's country, displays a relevant placeholder and provides formatting/validation methods. For a complete description of the plugin features, please visit https://intl-tel-input.com/.
You will need a basic website configuration:
- Local installation of Joomla (preferably version 3.8 or superior). The full detail of CMS requirements can be found here.
- PHP 5.5 or superior. PHP 7.2 is highly recommended.
- MySQL 5.1 or superior. MySQL 5.5.3 + is recommended.
- A PHP development environment (only if you plan to develop your own extension).
- Optional: NodeJS is only required if you plan to pack your own extension with Webpack.
The source code and the installable package (pkg_xttwilio_v1.1.0.zip) of the extension we’ll be modifying can be found in this repository:
https://github.com/anibalsanchez/XT-Twilio-for-Joomla/releases/tag/1.1.0
Add the Telephone plugin to the Click to Call Module
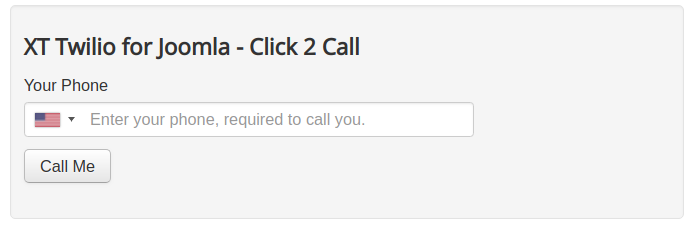
To add the Intl-tel-input plugin in the form, we’ll modify the files that implement the Click to Call module. The XT Twilio for Joomla extension should be installed inside of your modules folder at modules/mod_xttwilio_click2call
and the library in the libraries/xttwilio
folder. If you don’t have it installed, please follow the installation instructions here.
Add the Intl-Tel-Input plugin to the Media Folder
In the Joomla folder, add the Intl-tel-input plugin files at media/lib_xttwilio/intl-tel-input
. From the build directory of intl-tel-input, the following essential files must be copied to the media folder:
- The plugin stylesheet: css/intlTelInput.min.css
- The flags images: img/flags.png and img/flags@2x.png
- The plugin script: js/intlTelInput.min.js and js/utils.js
Additionally, since we are copying the minified (optimized) files, we also include the original source files for documentation purposes.
Declare the Media Installation
To declare the new files that we just copied, declare the new media installation in the library manifest located at libraries/xttwilio/lib_xttwilio.xml
;
In the module JavaScript routine located at modules/mod_xttwilio_click2call/tmpl/default.php
, add the Intl-tel-input plugin initialization and field validation:
Finally, in the module initialization located at modules/mod_xttwilio_click2call/mod_xttwilio_click2call.php
, add the Intl-tel-input plugin JavaScript and Stylesheet files:
The Ajax Communication
The front-end functionality must be supported by a backend element to manage the communication with Twilio. This is a schema of the implemented organization between the front-end modules and Twilio:
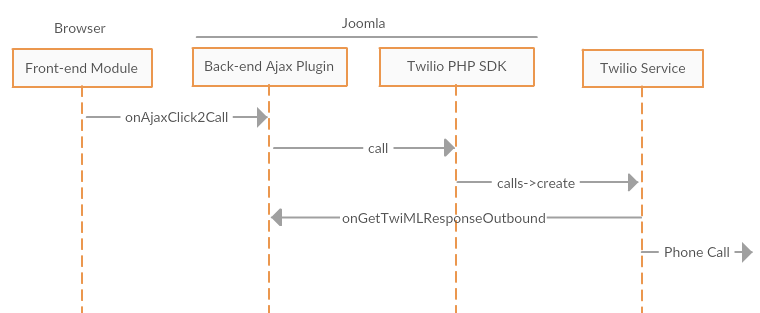
The user can enter the telephone numbers in several ways. In all cases, it is validated and the Backend receives the international telephone number, following the required E.164 standard. For instance, a US number could be +14155552671, or a GB number could be +442071838750. For more information, What is E.164?. Thanks to the plugin implementation, the communication progresses smoothly based on correct phone numbers. Of course the telephone could be a wrong number, but at least it is consistent under the known patterns.
Test the International Telephone Number
Similarly to the steps of the previous article, you must install the modified version of the extension, and complete the steps to send an SMS.
- Step 1 - Install the extension: I have already created the new version with the modification. You can download it for testing from here: https://github.com/anibalsanchez/XT-Twilio-for-Joomla/releases/tag/1.2.0
- Step 2 - The Twilio Account Configuration: After you complete the configuration to open a call, you are going to have the following information:
- Account SID
- Auth Token
- Twilio Phone Number
- Step 3 - Configure the account in the Ajax plugin: In the plugin, you must configure the previous credentials, Twilio’s phone and the Salesman Phone Number (who is going to answer the client call).
- Step 4 - Configure the Click to Call module: To configure the module from the Joomla admin menu, navigate to Extensions > Module > Twilio for Joomla - Click to Call module.
- Finally, visit the page, where you have published the module, and test with several invalid or valid numbers to confirm how the field immediately detects the number pattern.
Test Case: An invalid telephone number
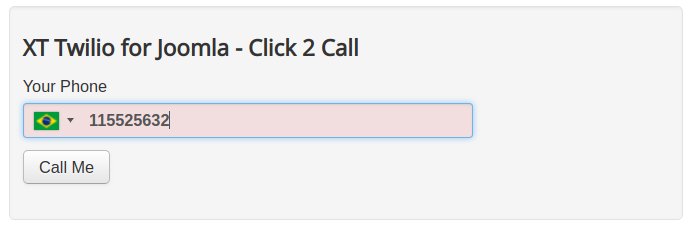
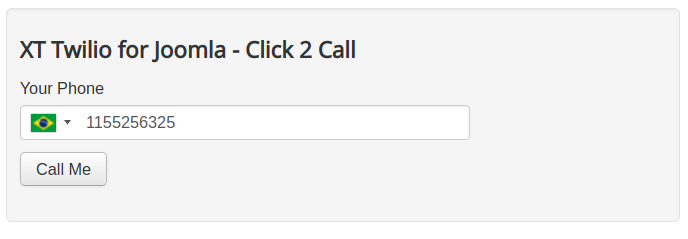
Next Steps
Based on this initial version of a Twilio implementation for Joomla, a world of possibilities is open. In this tutorial, I have explained in detail how to enrich the original modules to fulfill the requirement of a practical system to support international phone numbers.
Receiving the phone number in the right format and validating against the country pattern is the first layer of data verification. In the backend, a layer of validation could also help to reduce fraud, increase lead conversion, and drive message delivery programmatically. For this task, Twilio has the Lookup API. The service provides a formidable database to precisely know who is trying to reach you.
At this point, this solution of two modules and a library is complete and ready to provide further communication services to other extensions of the Joomla ecosystem, such as EShop, J2Store or VirtueMart. In the next installment of these series of articles for e-commerce solutions, we are going to add the Phone Validator API to check the phone numbers.
To the moon!
References
- XT Twilio for Joomla in the extension directory (JED)
- Source code repository: https://github.com/anibalsanchez/XT-Twilio-for-Joomla
- Twilio PHP SDK
- Webpack-automated build files for Joomla! extensions: https://github.com/anibalsanchez/extly-buildfiles-for-joomla
- Joomla! (https://www.joomla.org) is an award-winning content management system (CMS), which enables you to build websites and powerful online applications.
About Anibal
Anibal Sanchez is a technology geek, with a pinch of an entrepreneur. Aníbal is the team leader of Extly Tech. He helps businesses in rapid web development, implementing DevOps processes, quality assurance practices and project management methodologies. Anibal contributes to the Joomla! community as a member of the Leadership Team, JED’s Assistant Manager and Joomla! Magazine author.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.