Using the Yelp API and Twilio Messaging for Flower Delivery
Time to read: 6 minutes
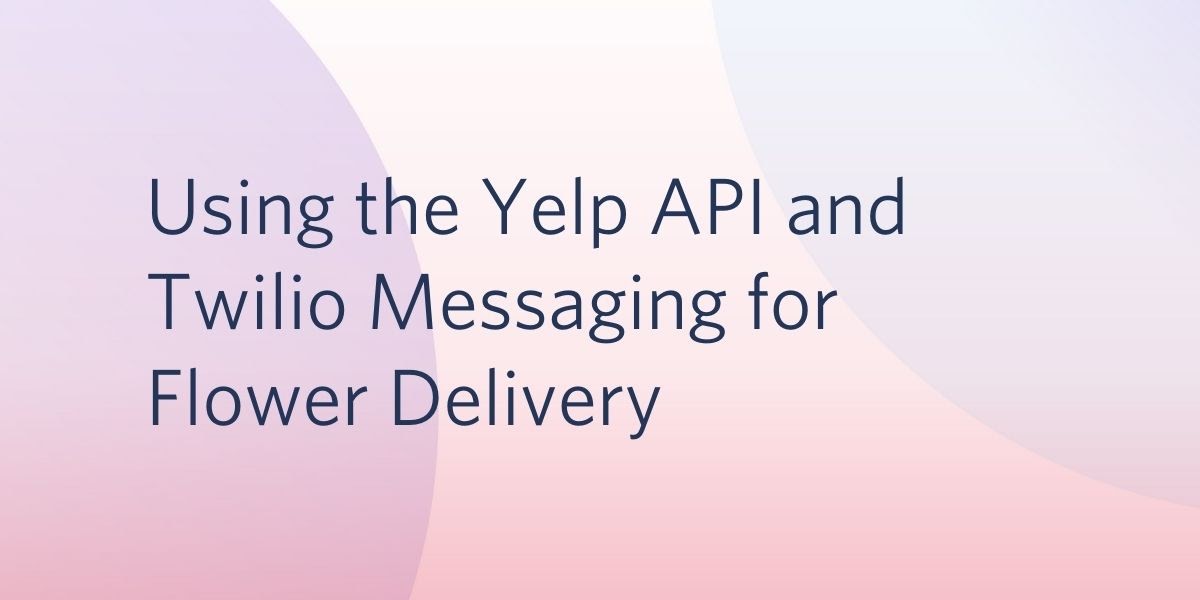
Important dates can easily sneak up on you, and before you know it, it’s only a few days away from a special event like Mother’s Day or a birthday. If you’re like me and need to grab a last minute (but still thoughtful) gift, using the Yelp API is a great way to get localized recommendations fast.
I hooked up the API to a Twilio phone number that you can text to get a list of florists in your delivery area. It will also indicate if the shop is currently open 💚or closed 🔴so you will know if it’s a good time to call. Try it out by texting +1 (815) 551-0M0M, which is +1 (815) 551-0606, to get recommendations based on delivery address zip code.
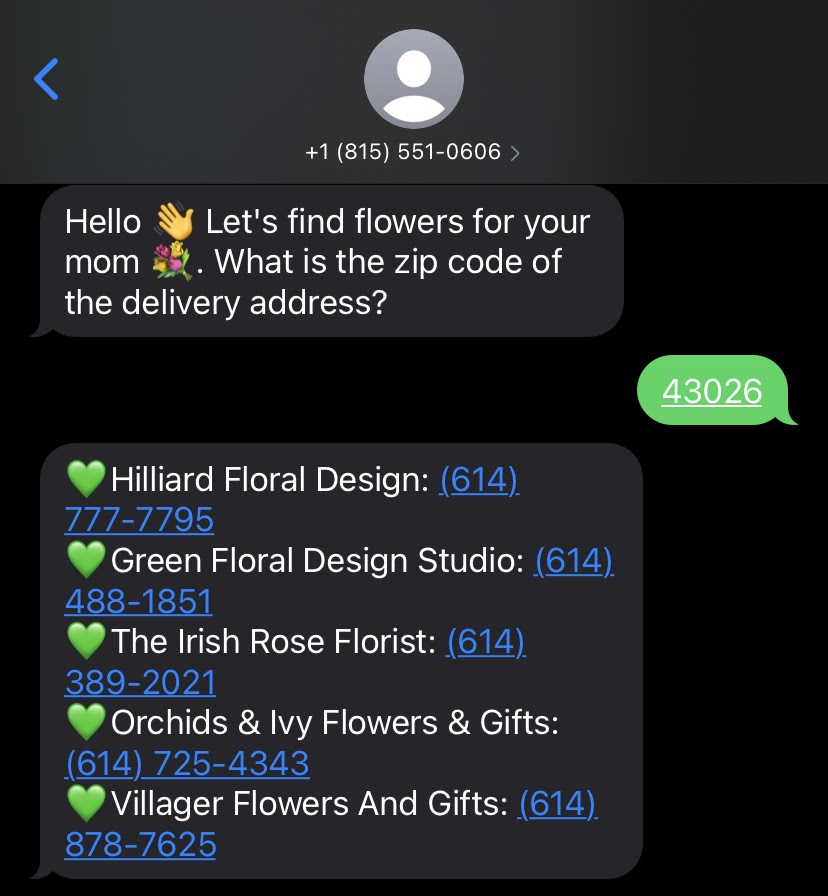
Here’s how you could build your own version of this using the Yelp API, Twilio Programmable Messaging, Studio, and Functions. First, you will set up the Yelp API call using Twilio Functions. Then, you’ll add in the messaging capabilities and set up a Twilio Studio flow to handle the incoming messages. Lastly, you’ll connect it to a Twilio phone number and test it out.
Prerequisites
- A Twilio Account — sign up for a free one here and get $10 when you upgrade your account.
- A Yelp account and Yelp Fusion API Key — Register for a free one here.
- Postman, a free tool for making API calls.
Set up a Yelp App and Get the Fusion API Key
The first thing you need to get started is a Yelp user account. If you already have access to Yelp, you can log and check out their developer documentation. Next, you will need to create an app on the Yelp Developer’s site. You need to fill out some basic information about what you’re building. Once you save your app info, it will generate a Client ID and an API Key.
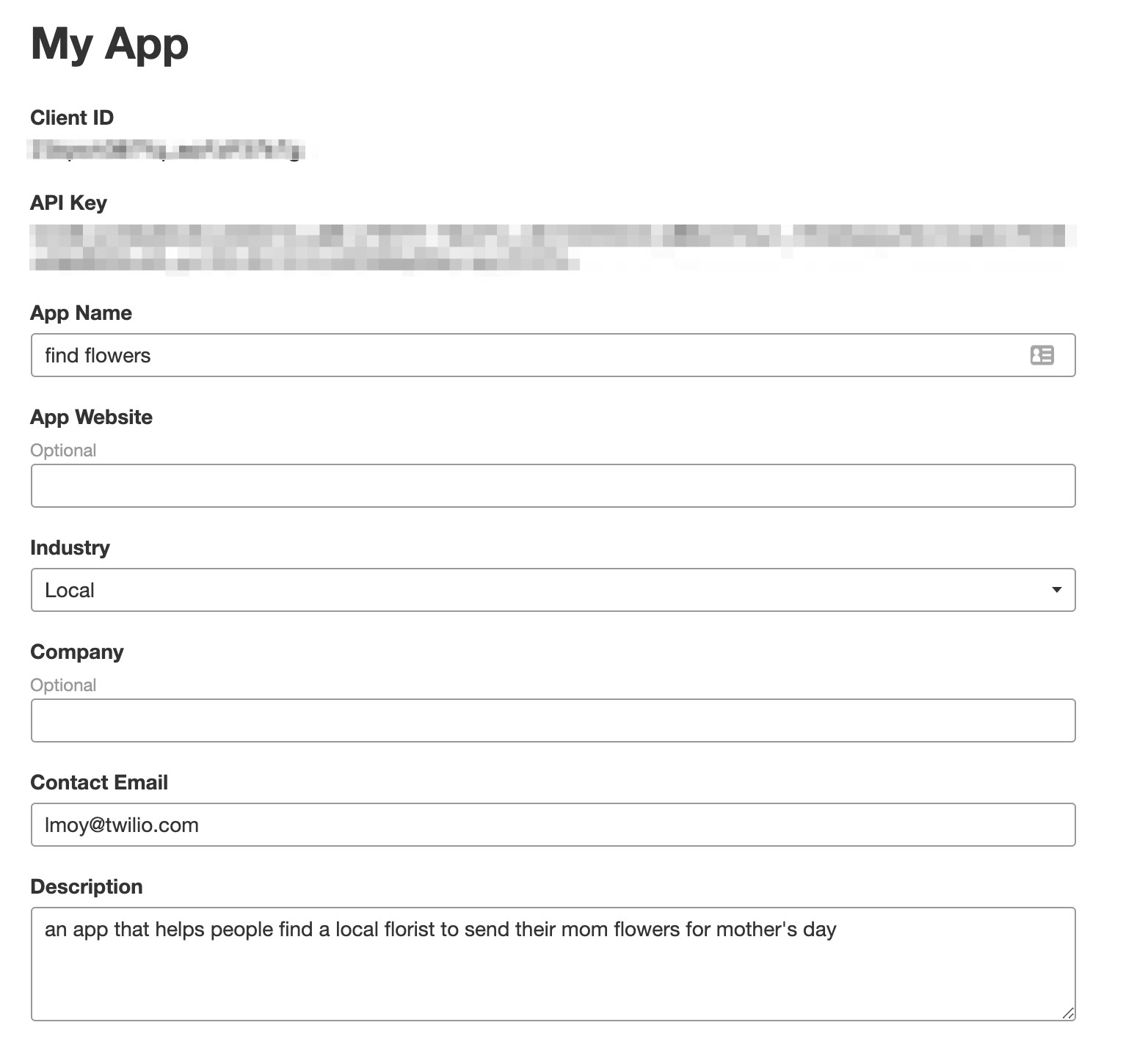
The Yelp API provides accessible data about different businesses and allows you to retrieve this data using a location such as a city name or a zip code. So for the purposes of this project, it fits the bill.
There are two different Yelp APIs that can be used to get the data. I chose to use the Yelp Fusion API for this project since it is stable and has great documentation. Another option is the Yelp GraphQL API. You must be a part of the Yelp Developer Beta Program to try this out, which you can easily join or leave by clicking the Join the Developer Beta button in your Manage App settings.
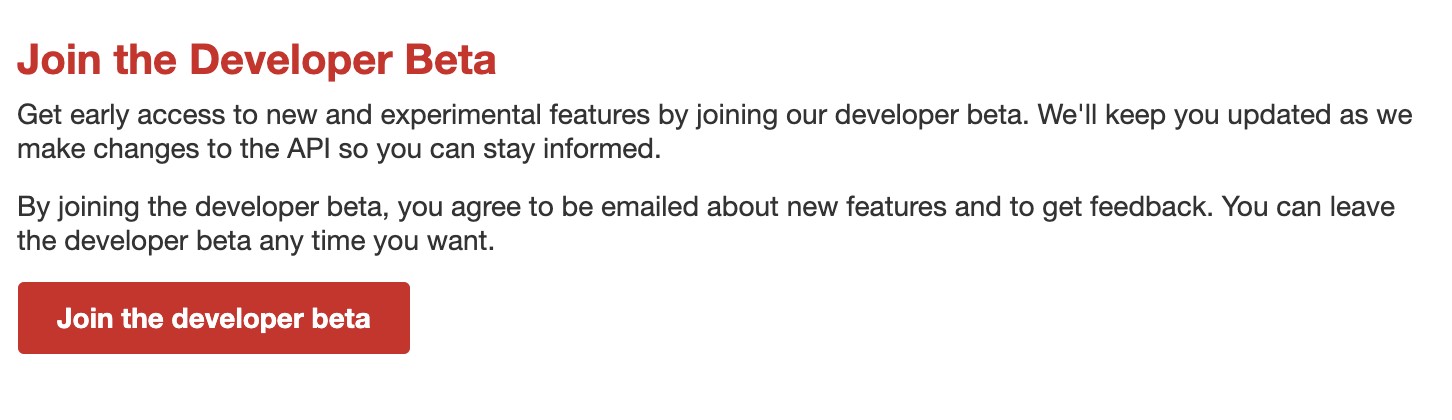
Regardless of which API route you choose, keep the API Key and Client ID handy because you will need them in the next step.
Set up Twilio Function Dependencies and Environment
Next, head to your Twilio console and go to Twilio Functions. Click the blue Create Service button and give it a name (I chose find-flowers
for mine, but if you are making a tool to search for a different type of service feel free to name it accordingly).
Open up the Environment Variables console, which is in the bottom left corner of the Functions console. Here you will need to input the Yelp credentials and give them the names YELP_CLIENT_ID
and YELP_API_KEY
. You will need the API Key to make the call out to Yelp in the function.
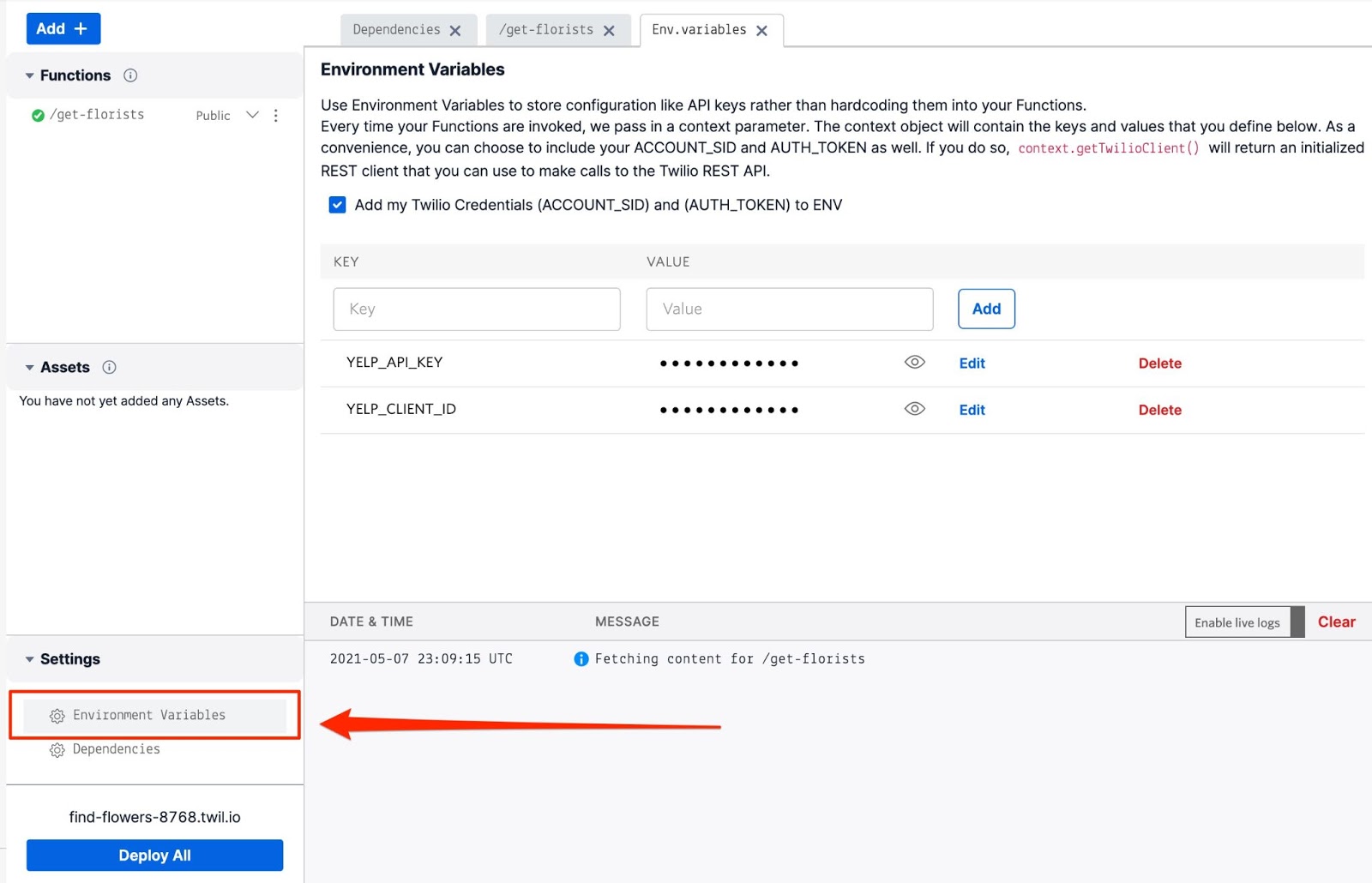
Then, you need to think about how you will want to call the Yelp API (for more ideas you can check out this post on 5 ways to make HTTP requests in node.js from my awesome co-worker Sam). You will need to make a get request to get the list of business names, phone numbers, and information about if they are open. I chose to use axios for this build. In order to use it you need to add axios to the Dependencies console.
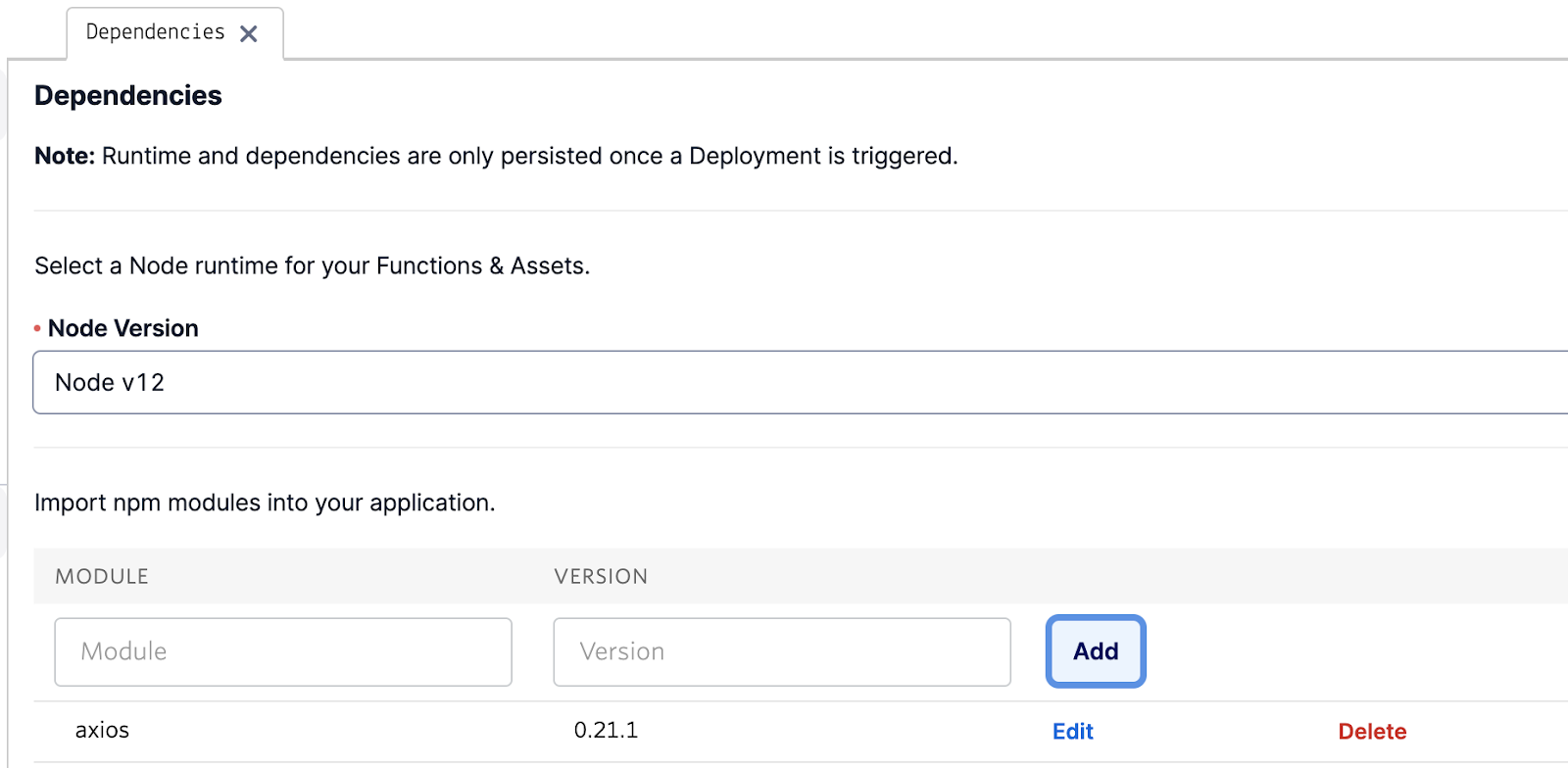
Now you can create a Function and make a call to the Yelp API.
Create a Twilio Function to Fetch Florist Data from Yelp
Click the blue Add button and give your function a name (I named mine "get-florists"). Delete the boilerplate code that is auto-generated in the function, and replace it with the below.
In this code, you are getting the API key from the context, which is where it is stored after you save it to the Environment Variables console. You make the GET request using axios, and need to use the API Key to access your account in the header.
You will specify the parameters you want to search for, including location, the term or type of business, and how many businesses you would like to receive. If you want to check out the other options for parameters, you can find them all in the Yelp Fusion API business endpoint documentation. For now a zip code is hard coded, but in the next section you will set up the Function to receive a parameter from the Studio flow based on what someone texts back.
Next, loop over all of the business data, and format it in a string. This will make the data readable when you send it back as a SMS message.
Change the visibility of the Function to public from the dropdown to the right of it. Then hit Save > Deploy All and then refresh the page. Turn on live logs, and test the endpoint out using Postman. Once you add your Twilio credentials to the Postman Authorization panel, you can copy the URL of the Function by hitting the option menu next to it.
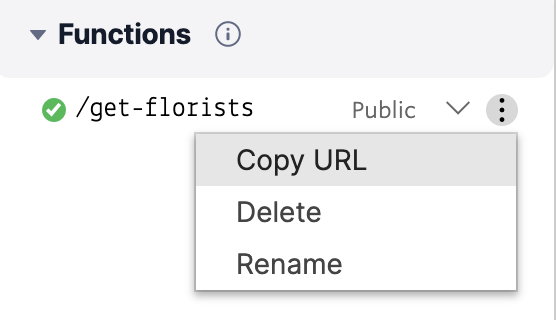
Paste this into Postman and test it as a POST request. You should see something like the below logged in the Functions console.

Add TwiML Messaging Capabilities to Respond to Incoming Texts
In this next part, you will add in TwiML functionality from the node.js helper library so that this Function will send back a response to a texter with the data received from Yelp. You will also use the is_closed
boolean flag from the API so that your message can indicate whether the business is currently open or closed at the time the texter messages in.
Replace the Function code with the block below.
You will use TwiML, the Twilio markup language, via the node.js helper library in order to reply back. You could also send the data back as JSON to the Twilio Studio flow that you are about to set up. Doing it all in the Function saves an additional step.
In this code, you will set the default of a business to be open with the openClosed
variable set to a green heart emoji. If is_closed
is true, you’ll change the variable to be a red circle emoji. Once the string is formatted with openClosed
, and the business information, you will set it as the body of the message. You’ll also want to make sure that the twiml
Messaging Response is in the callback.
Now you can connect this to Studio and a phone number.
Buy a Phone Number and Connect it to Twilio Studio
First, you will need to buy a Twilio phone number. Once you have a phone number, head to the Twilio Studio dashboard and click the blue plus sign to start a new flow.
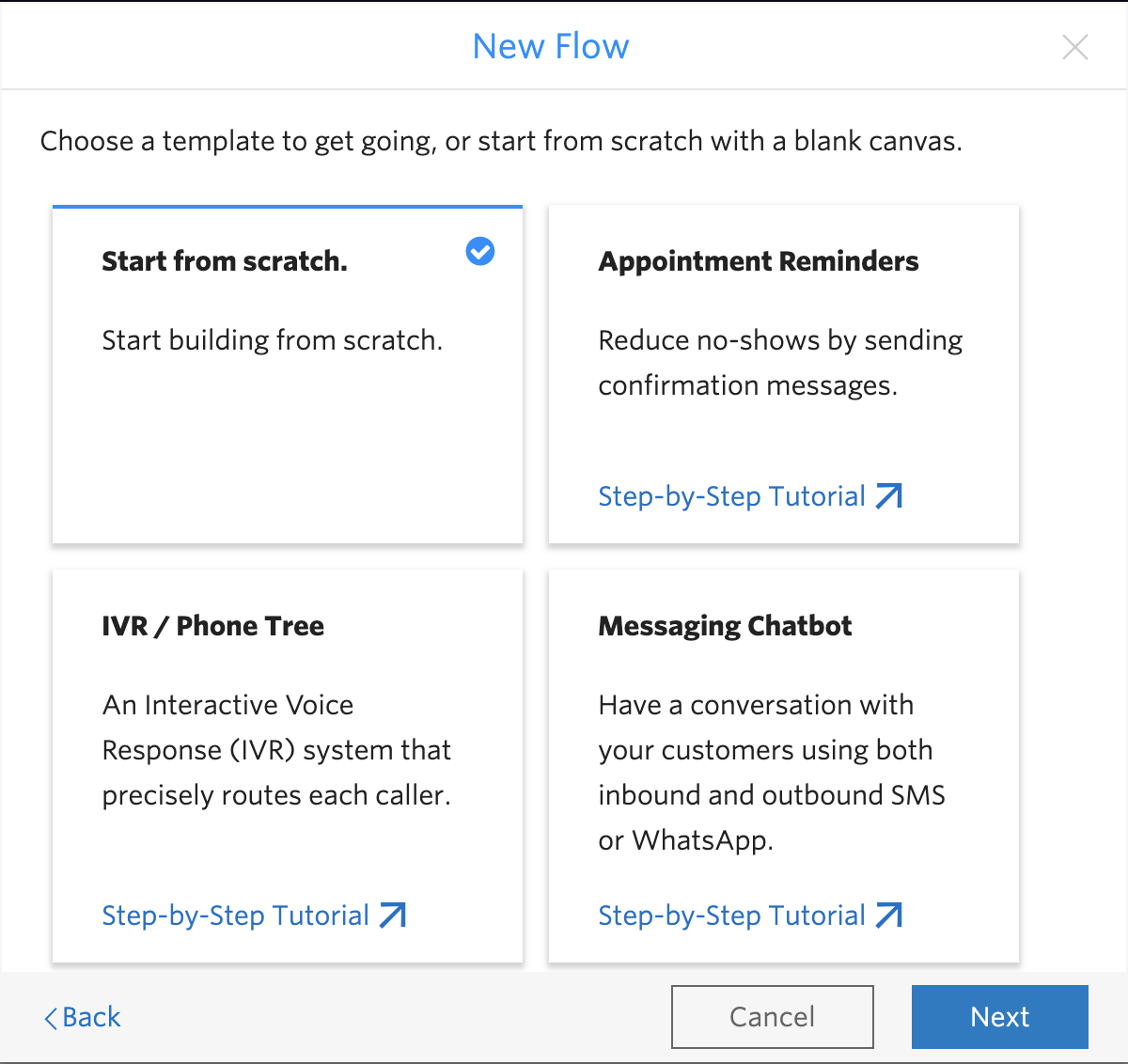
You will start from scratch, and as you will see in a moment, you will need just two widgets to create this: the Send and Wait for Reply widget, and the Run Function widget.
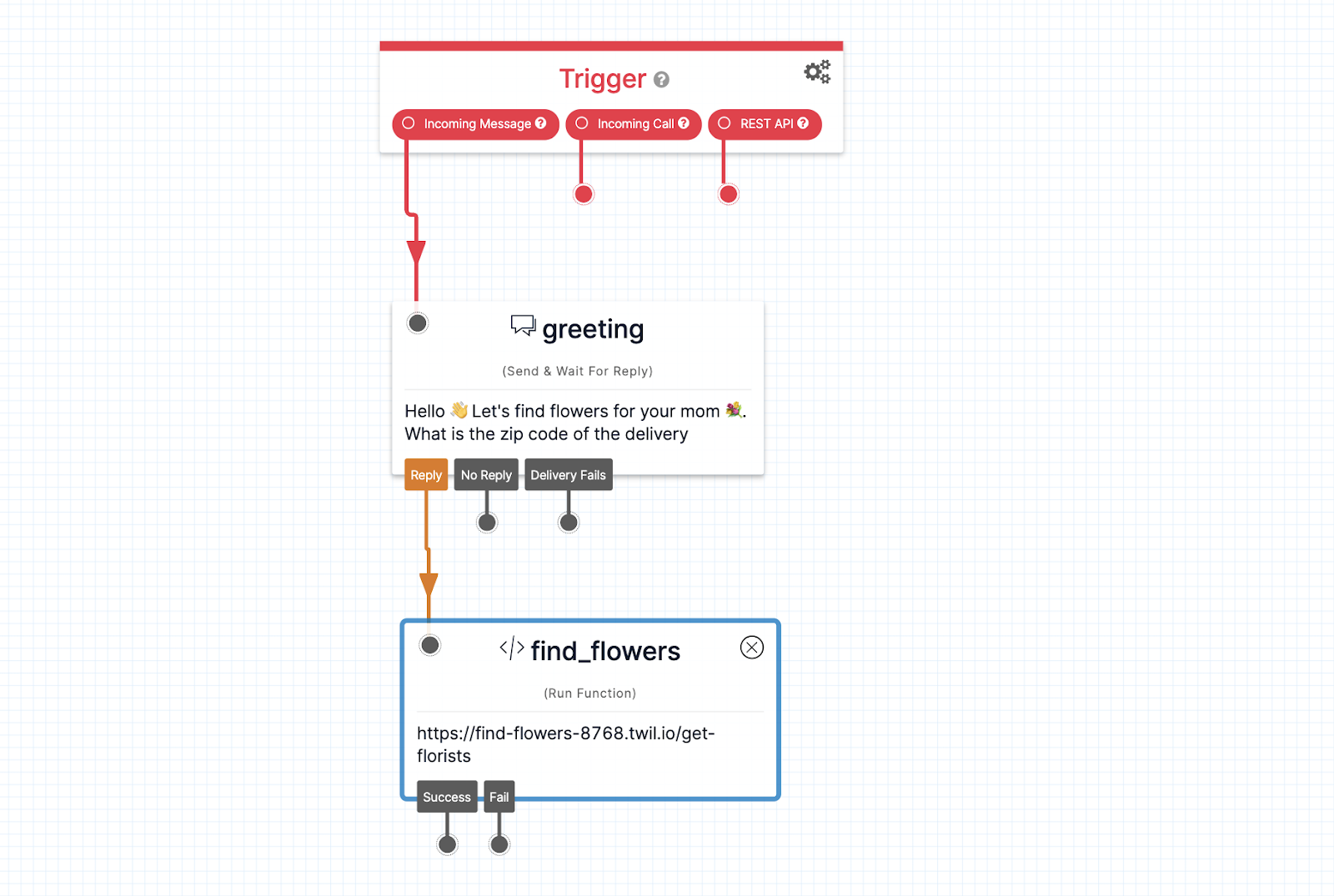
First, choose the Send and Wait for Reply widget. Give it a unique name, and input the greeting you wish to send.
Next, grab the Run Function widget. When you click into the service, environment, and function dropdowns, they should auto-populate with what you created in Functions. You will need to add zipCode
as a key in the function parameters. For the value of zip code, you will use the body of what the texter messaged in. This is retrieved from the Studio context using the liquid templating language and should look something like {{widgets.greeting.inbound.Body}}
. After you’ve configured the Run Function widget, hit the “Publish” button to save your Studio Flow.
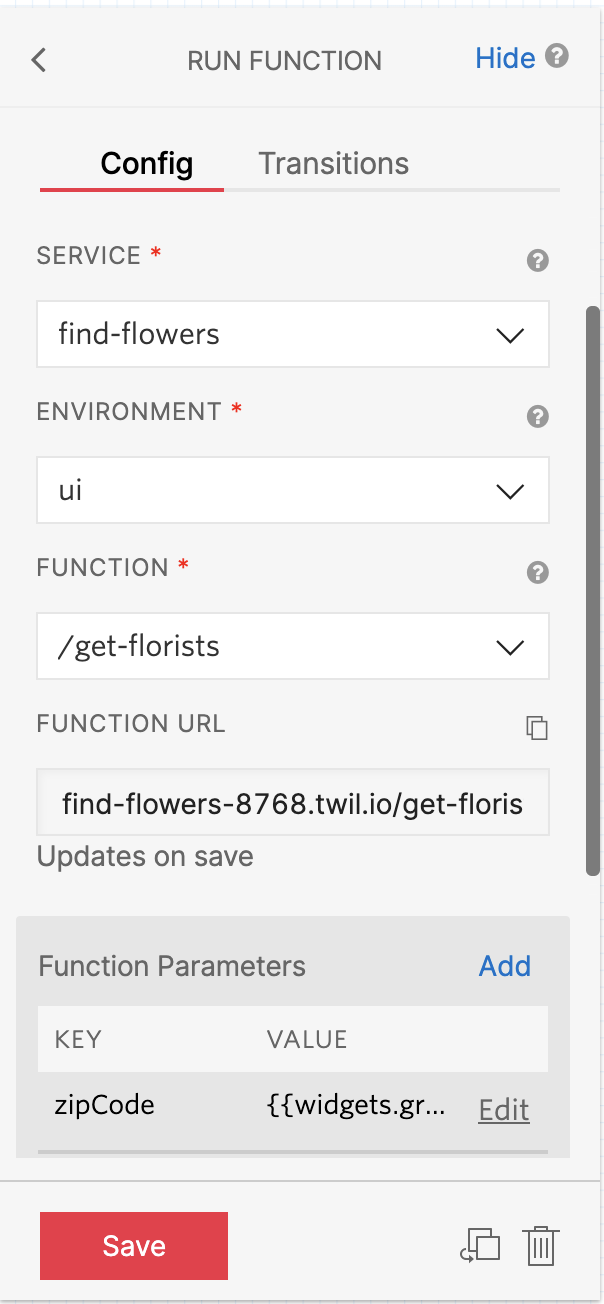
Now head back to your Phone Number. Scroll down to the Messaging configuration, and choose Studio. Find and select your Studio Flow from the dropdowns for when a message comes in.
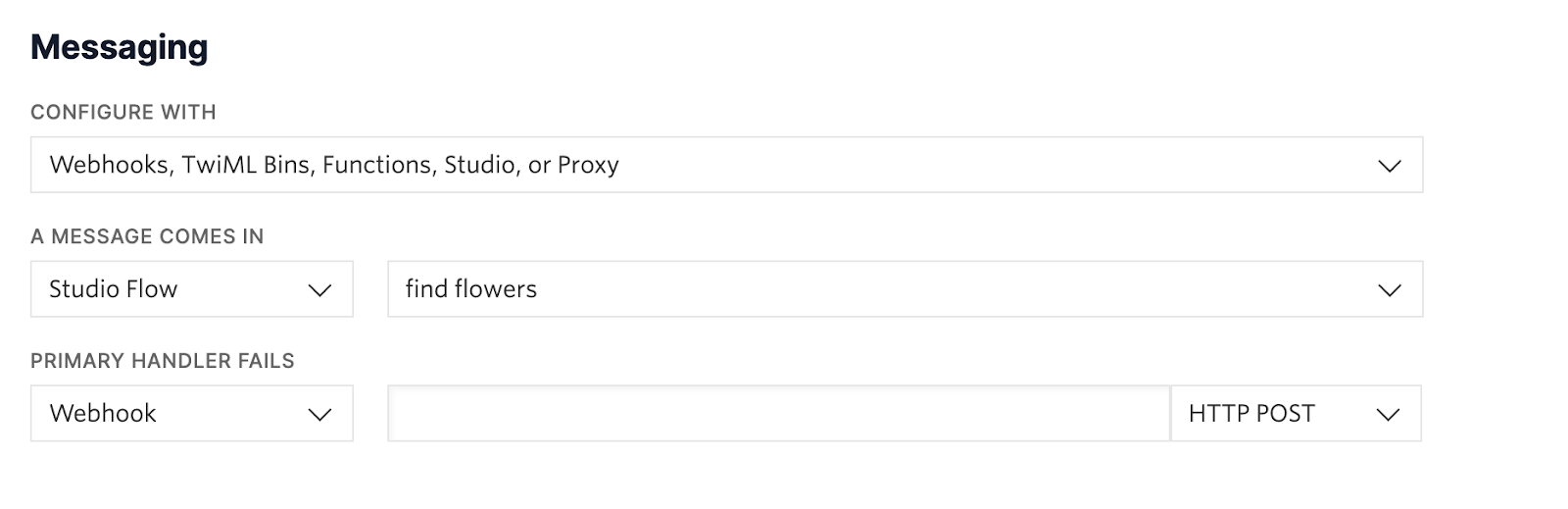
Now, text a message to your number. You should get something like the below:
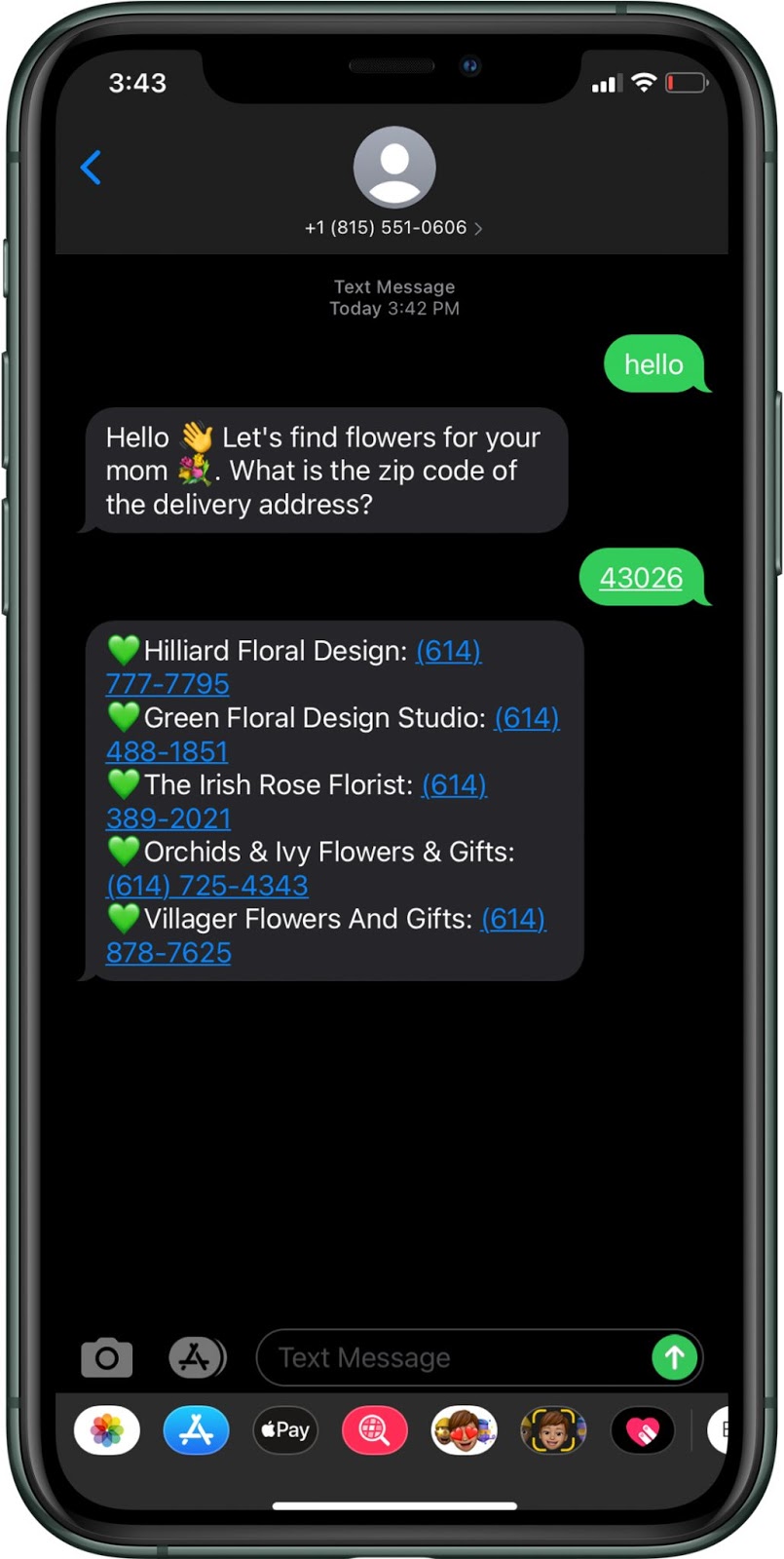
Conclusion
This is just one way that you can implement the Yelp API using Twilio Messaging, but there are so many other applications you could build with it (and you could try the Yelp GraphQL API as well). Maybe something to help choose a restaurant when you’re in a new neighborhood, or even using the API to grab photos from the business pages to have them sent directly to you.
If you decide to make something, or use the number to make a delivery order, I’d love to hear about it. Email me lmoy[at]twilio.com to let me know, and send me a tweet with a photo of what you ended up sending to your loved one.
Liz Moy is a Developer Evangelist on the Enterprise Evangelism team. She loves to talk to developers and does so frequently on Build and Deploy with Liz Moy, a podcast powered by Twilio. You can find her at lmoy [at] twilio.com or on Twitter @ecmoy.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.