ETA Notifications with Node.js and Express
Time to read: 2 minutes
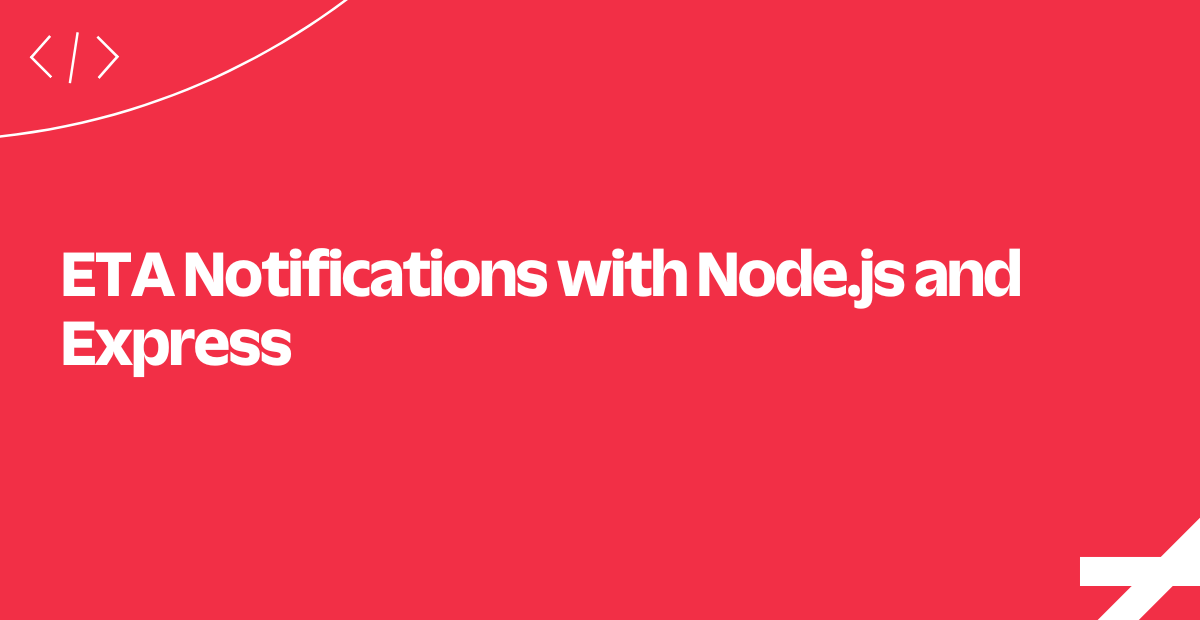
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly wherever we are.
The key to those services? Instant customer notifications when something changes.
On this tutorial we'll build a notification system for a fake on-demand laundry service Laundr.io in Node.js and Express.
Let's get started!
Trigger the Notifications
This delivery driver's screen will show two buttons that allow him or her to trigger notifications: one for picking up an order and one for delivering it. Those buttons will be wired to the appropriate route:
- Delivery person picks up laundry to be delivered (
/pickup
) - Delivery person arrives at the customer's house (
/deliver
)
On a production app we would probably trigger the second notification when the delivery person was physically near the customer using GPS. (In this case a button will suffice.)
On the server we'll use the Twilio REST API Client to actually send out the notifications. Let's go there next.
Setting Up the Twilio REST Client
Here we create a Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
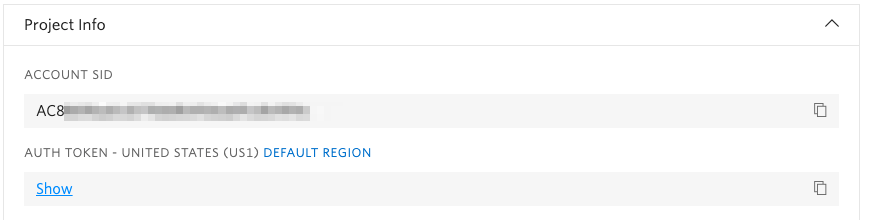
On deck: how we handle notification triggers.
Handle a Notification Trigger
This code demonstrates how to handle a HTTP POST
request triggered by the delivery person.
It uses the sendSmsNotification
method of our Order
model to send an SMS message to the customer's phone number (which we have registered in our database). Simple!
Next, let's take a closer look at how we send the SMS.
Sending the Message
Here we demonstrate actually sending the SMS.
Picture worth 1,000 words? Improve the message by adding some optional media with mediaUrl
:
'mediaUrl' : 'http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg'
In addition to the required parameters (and optional media), we can pass a statusCallback
url to let us know if the message was delivered.
Status updates on message delivery is interesting - let's look there next.
Handle an Incoming Twilio Callback
Twilio will make a POST
request to this controller each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notificationStatus
on the Order
and business logic dictates what we'd do next. This is an excellent place to add logic that would resend the message if it failed, or send out an automated survey after the customer receives their clothes and a delivery SMS.
That's all, folks! We've just implemented an on-demand notification service that alerts our customers when their order is picked up or arriving.
Now let's look at other features Twilio makes it easy to implement.
Where to next?
Node and Twilio are like two peas in a pod. Here are some other tutorials where we demonstrate interesting features:
Increase your rate of response by automating the workflows that are key to your business. In this tutorial you will learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand so two users can communicate without exchanging personal information.
Did this help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.