Send SMS Messages in Delphi
Time to read: 1 minute
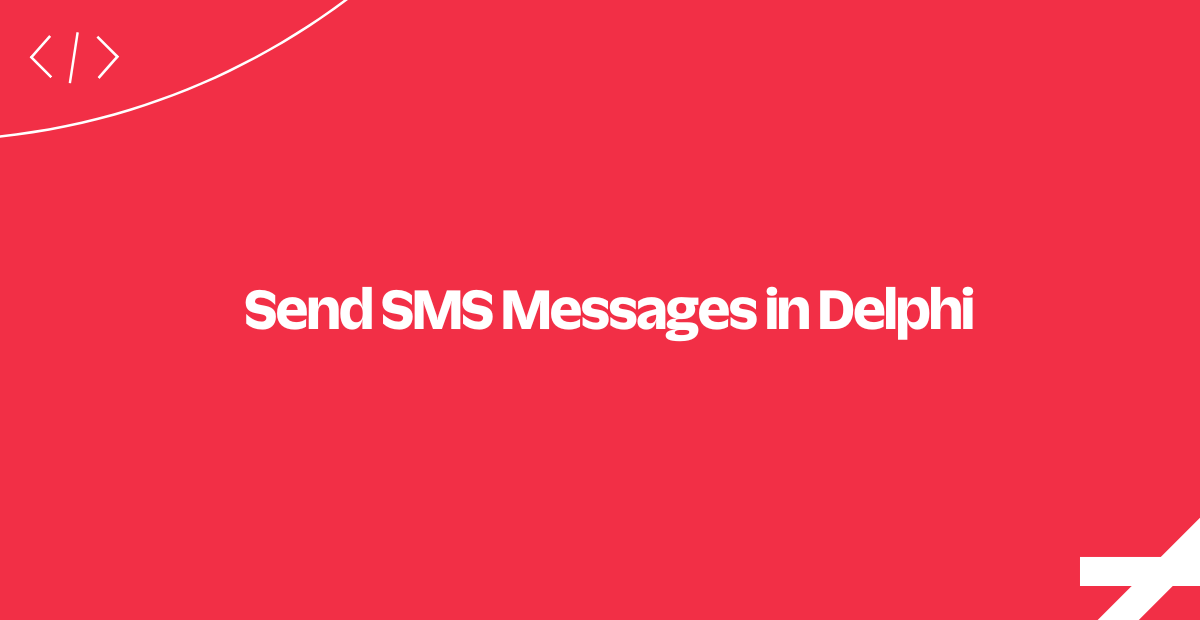
In this guide, we'll show you how to use Programmable SMS to send SMS messages in your Delphi application. The code snippets in this guide were written and tested using Delphi 10.2.3. You can view the complete sample project on GitHub.
Let's get started!
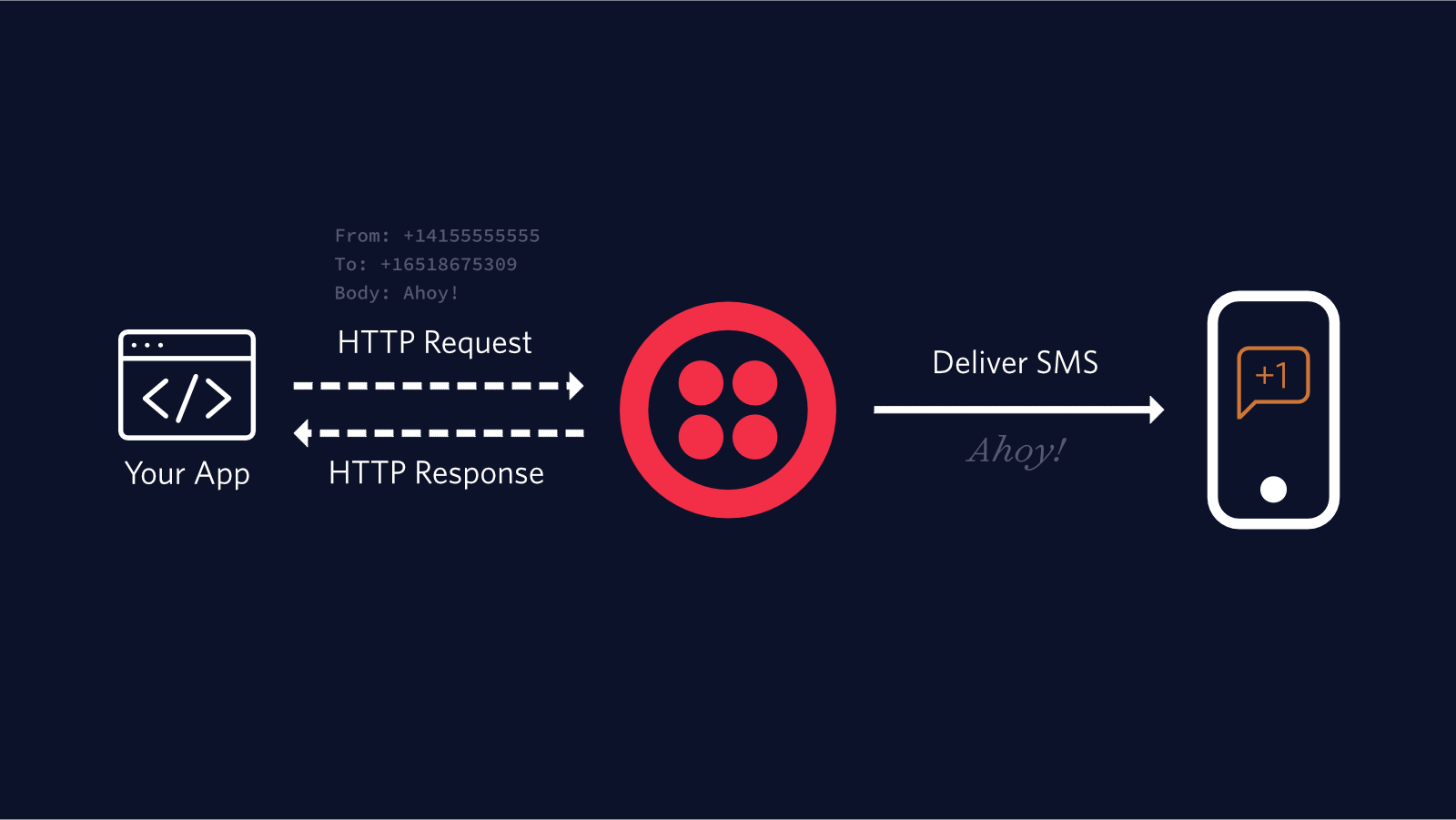
Sign up for a Twilio Account
To use the Twilio REST API, you need an account. Signing up for a free Twilio account is easy. Once you've signed up, head over to your Console and grab your Account SID and your Auth Token. You will need those for the code samples below.
Purchase an SMS Capable Phone Number
Sending SMS messages requires an SMS capable phone number. You can browse the available phone numbers in the Console. Be sure that the phone number you buy is SMS capable. When you search, you can check the box to filter available numbers to those that are SMS capable:
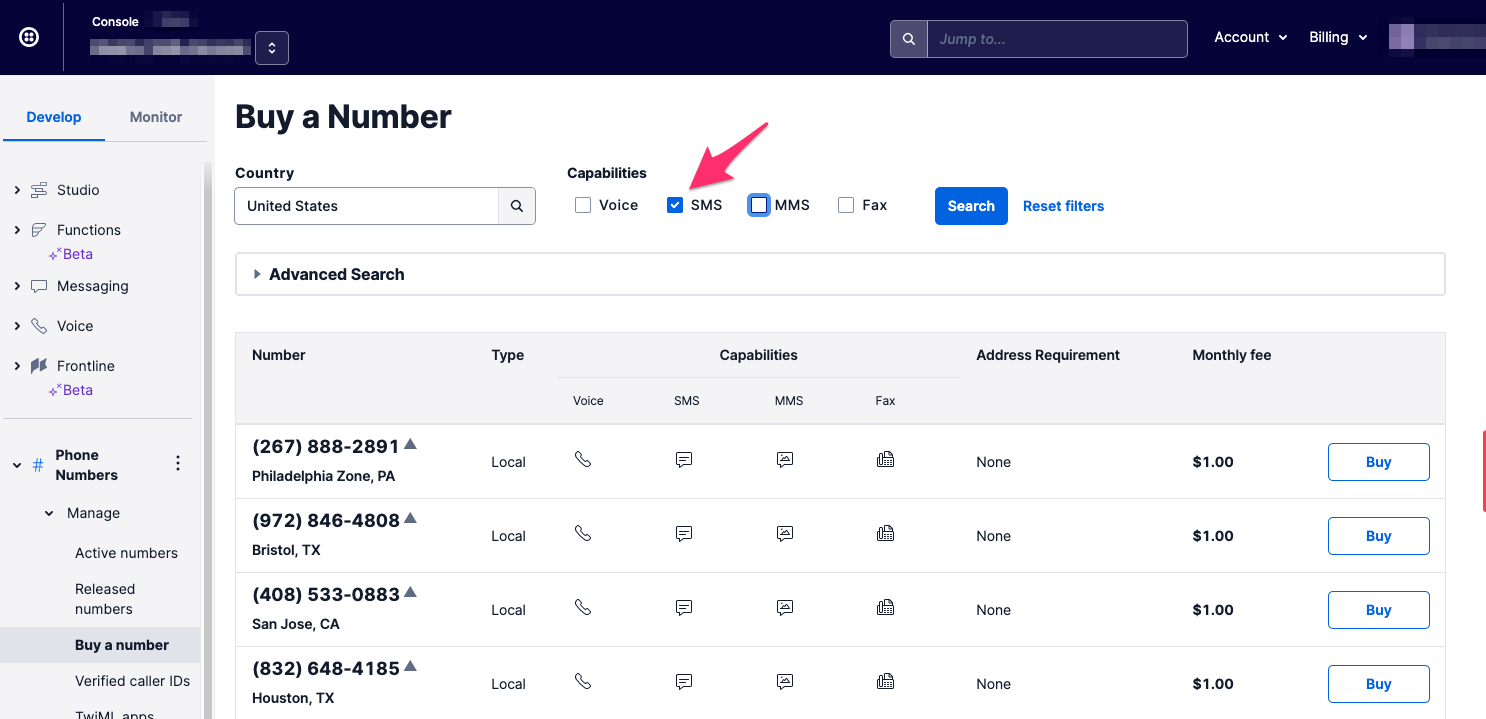
When viewing the search results, you can see the capability icons in the list of available numbers:
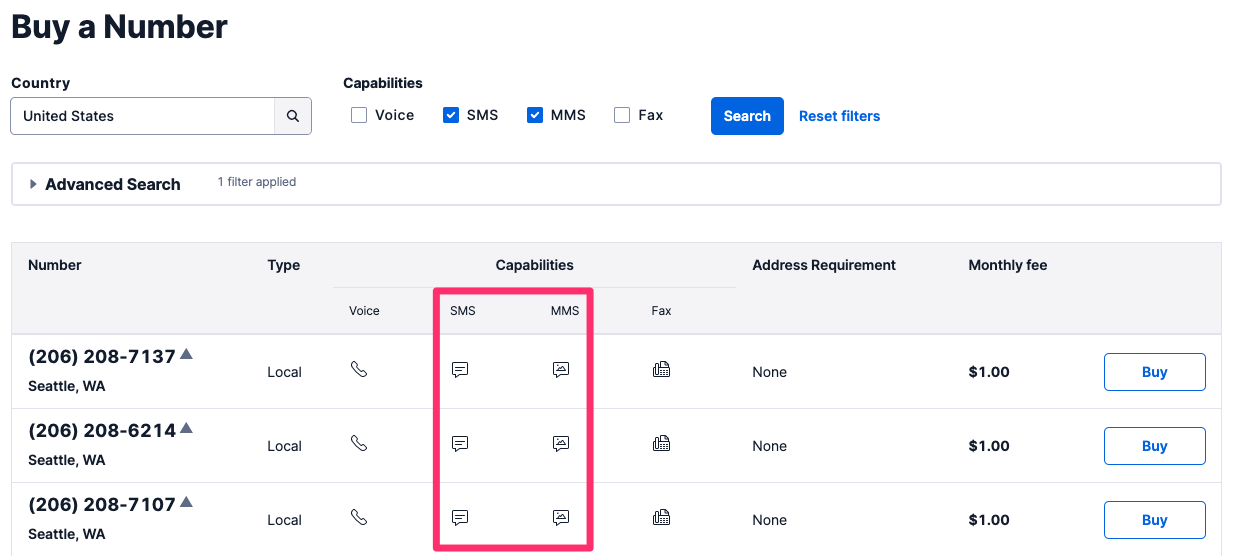
Armed with a Twilio phone number, you can now start sending messages to mobile devices.
Send an SMS Message via the REST API
Sending an outgoing SMS message requires sending an HTTP POST to the Messages resource URI. Using the provided TTwilioClient
, you can post to the Message resource and specify the To, From, and Body parameters for your message.
If you want to send a message to several recipients, you could simply create an array of recipients and iterate through each phone number in that array. You can send as many messages as you like, as fast as you like and Twilio will queue them up for delivery at your prescribed rate limit. See our guide on how to Send Bulk SMS Messages for more tips.
For more information about sending SMS and MMS messages, see the REST API Reference.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.