SMS and MMS Marketing Notifications with Python and Flask
Time to read: 3 minutes
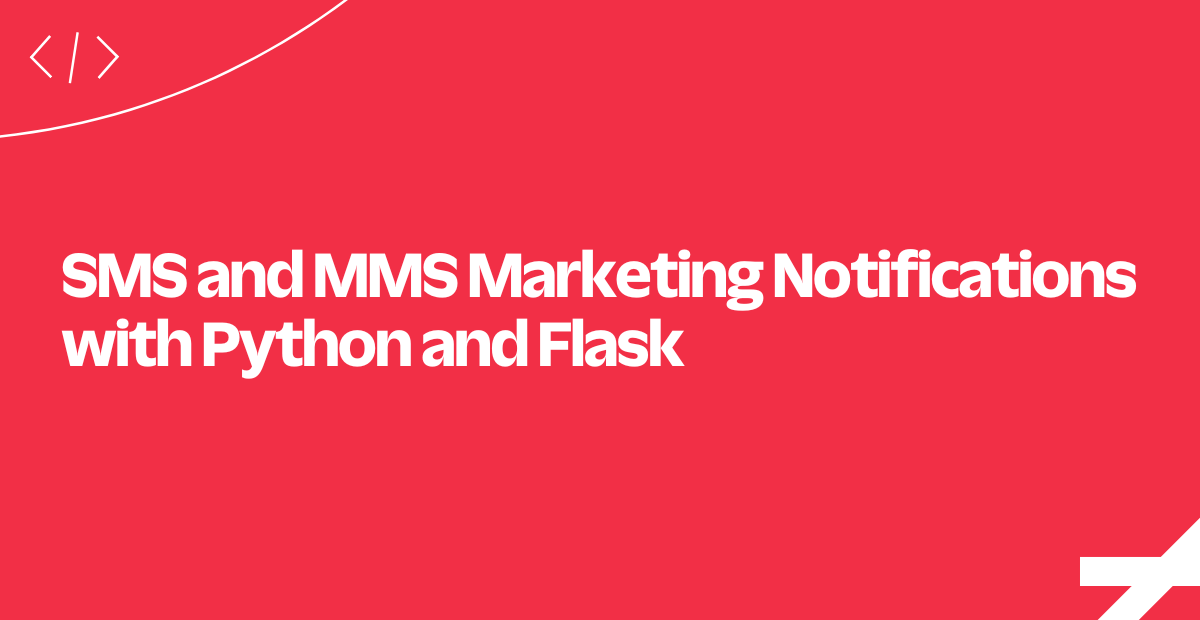
Ready to implement SMS and MMS marketing notifications and see what potentially very high engagement rates will do for you?
Excellent! Today we're going to take you through implementing marketing notifications in Python and the Flask Microframework.
Here's how it'll work at a high level:
- A potential customer sends an SMS to a Twilio phone number you advertise online, on TV, in print, on a billboard, or so on.
- Your application confirms that the user wants to receive SMS and MMS notifications from your application and is added to the subscriber list.
- An administrator or marketing campaign manager crafts a message that is sent to subscribers via SMS/MMS message.
Building Blocks
To get this done, you'll be working with the following tools:
- TwiML and the <Message> verb: We'll use TwiML, the Twilio Markup Language, to manage interactions initiated by the user via SMS.
- Messages Resource: We will use the REST API to broadcast messages to all subscribers.
Let's get started!
The Subscriber Model
In order to send out marketing notifications to a subscriber, we need to provide the right model.
phone_number
stores where to send the notifications.subscribed
identifies which subscribers are active (only active subscribers will receive notifications).
Next up: how to handle incoming messages.
Handling Incoming Messages
This is the endpoint that will be called every time our application receives a message.
- We check if a user is in our database. If the user is not, encourage them to 'subscribe' and return TwiML through the helper library.
- If the user was registered, check that the command has a valid 'subscribe' or 'unsubscribe' in the body.
- If the command is valid, set the user's status to
subscribed
ornot subscribed
, respectively, and return appropriate TwiML through the helper library.
So now we've created a Subscriber
model to keep track of subscribers, unsubscribers, and new customers.
Next, let's take a look at how to reply to received messages.
Replying to Received Messages
We ask Twilio to reply with an SMS by creating a twiml.Response
and calling the message
method on the created object.
Now let's look at how to manage subscriptions.
Managing Subscriptions
We want to provide the user with two SMS commands to manage their subscription status: subscribe
and unsubscribe
.
These commands will toggle a boolean for their Subscriber
record in the database. On the business side, this boolean will determine whether or not they receive messages from our marketing campaign. To respect our user's desires, don't opt them in automatically - rather, they have to confirm that they want to receive our messages.
To make this happen, we will need to update the controller logic which handles the incoming text message to do a couple things:
- If it is a
subscribe
orunsubscribe
command, then create/update their subscription with the right status in the database. - If it is a command we don't recognize, send them a message with the available commands.
Next up, let's look at sending the marketing notifications.
Sending Marketing Notifications
On the server side, we retreive the message text and potential image URL. Next we loop through all Subscribers and call the method send_message
on our TwilioServices
domain object to send messages.
When the messages are on their way, we redirect back to the marketing form with a custom flash
message containing feedback about the messaging attempt.
Let's take an even closer look at how we are sending SMS (or MMS) notifications.
Sending SMS or MMS Notifications
In the method send_message
, we create a Twilio REST client that can be used to send SMS and MMS messages. The client requires your Twilio account credentials (an account SID and auth token), which can be found in the Twilio Console.
Next all we need to do is call create
on the object in order to send our message. The Twilio Message API call requires a from_
, to
and a body
parameters-- the media_url
is optional.
That's it! We've just implemented an opt-in process and an administrative interface to run an SMS and MMS marketing campaign with Python and Flask. Now all you need is killer content to share with your users via text or MMS.
We'll leave that part to you. However, we will suggest a few other awesome features for your application on the next pane.
Where to Next?
We've got lots of Python and Flask guides on the site, but here are a couple of our favorites:
Automate the process of reaching out to your customers in advance of an upcoming appointment to drive down your no-show rate.
Convert web traffic into phone calls with the click of a button on your company's web site.
Did this help?
Thanks for checking out this tutorial! Tweet us @twilio to let us know what you thought or what you're building next.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.