SMS and MMS Notifications with PHP and Laravel
Time to read:
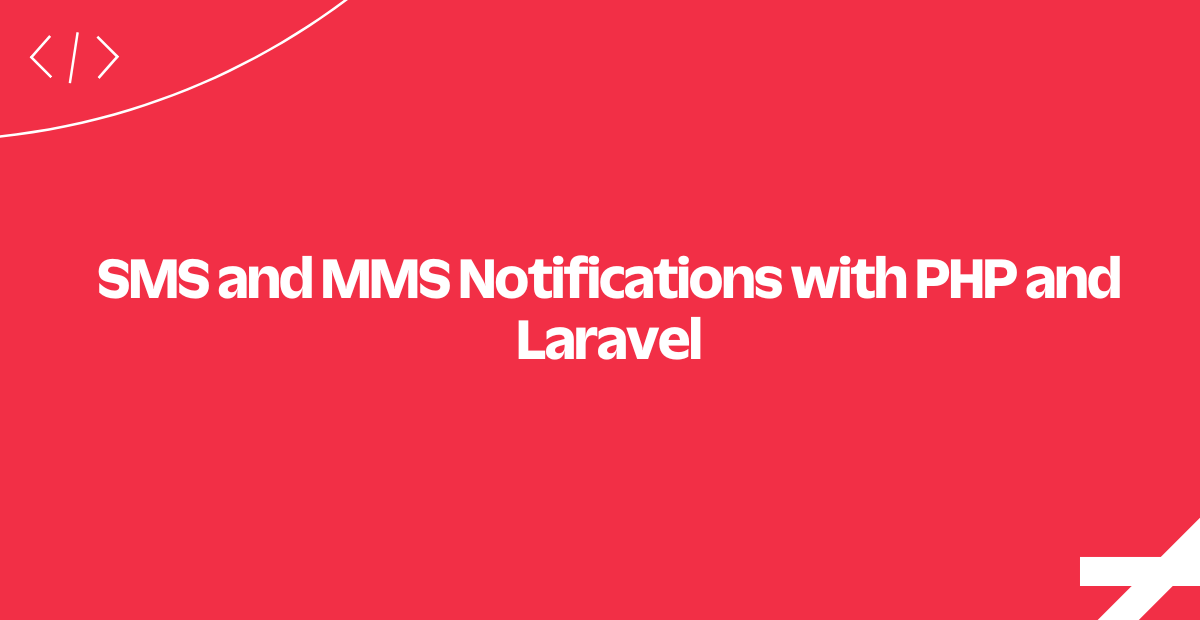
Today we're going to get your server to automatically sound the (textual) alarm when something goes wrong. Using PHP and Laravel, we'll send SMS text message server notifications to the phones of all of your server administrators when there's an exception on your site.
Clone the application and head to the application's README to see how to run the app locally.
Let's get started!
Click the button below to start the journey.
List Your Server Administrators - And Other Lucky Folks
Here we create a list of people who should be notified if a server error occurs.
The only essential piece of data we need is a phone_number
for each administrator.
Next up, we'll see how to set up the Twilio client.
Configure the Twilio Client
To send a message, we'll need to create a Twilio REST client, which requires reading our TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
from environmental variables.
The values for your account SID and Auth Token come from the Twilio console:
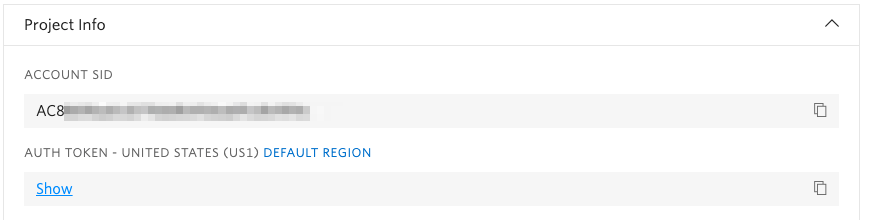
Next let's see how to handle application exceptions.
Handle Application Exceptions
Each time an exception is raised anywhere in a Laravel application an ExceptionHandler
will handle it. (Appropriate name!)
This is where we hook our SMS sending code. It's important to call parent
so the framework can do its regular error handling as well.
Next up let's see how to create a custom message.
Create a Custom Alert Message
Here we create an alert message to send out via text message.
You might also decide to include a picture with your alert message... perhaps a screenshot of the application when the crash happened? A meme image to calm everyone down?
Let's now take a look at how to load the list of administrators.
Read the Administrators from the JSON File
We read the lucky people from our JSON file and send alert messages to each of them with the private send_message
method.
Now let's look at how to send a text message.
Send a Text Message
There are the three parameters needed to send an SMS using the Twilio REST API: From
, To
, and Body
.
After the message is sent, we print out the phone number we're texting. US and Canadian phone numbers can also send an image with the message. (Other countries will have a shortened url appended pointing to the image.)
That's all, folks!
We've just implemented an automated server notification system that can push out server alerts if (ahem, when) anything goes wrong. Now let's look at some other great features you might like to add.
Where to Next?
PHP and Twilio - such a great combination. Here are just two other excellent tutorials for you to try out:
Increase the security of your login system by verifying a user's mobile phone in addition to their password.
Send your customers a text message when they have an upcoming appointment - this tutorial shows you how to do it from a background job.
Did this help?
Thanks for checking out this tutorial! Tweet @twilio to let us know what you think.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.