Implement CRUD Operations in CakePHP
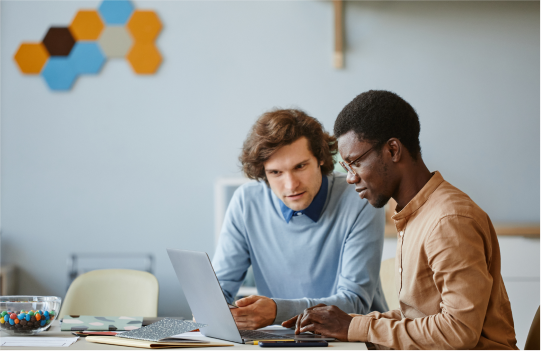
Time to read: 4 minutes
CRUD is an acronym for four basic operations that can be performed on a database: Create, Read, Update, and Delete. They are a set of operations commonly used in database and database management systems for viewing and modifying data.
This tutorial will teach you the essentials of implementing CRUD operations in CakePHP. It illustrates how users can create, read, update, and delete records, thus providing a guide to managing data in your application in CakePHP.
Prerequisites:
Before we dive into the tutorial, make sure you have the following:
- Basic knowledge of PHP and web development concepts
- PHP 8.2 installed with the PDO MySQL extension
- Access to a MySQL server
- Composer installed globally
Bootstrap a new CakePHP application
To install CakePHP, navigate to the folder where you want to scaffold the project and run this command:
When asked “Set Folder Permissions ? (Default to Y) [Y,n]?”, answer with Y.
The new CakePHP project will be available in a directory named cakephp_crud, and you'll have changed to that directory.
Configure the database
Once we've created, the next step is to connect to the database before starting up our development server. To do that, open up the project folder in your preferred code editor or IDE and navigate to config\app_local.php.
Where it says Datasource
and default
section, change the default configuration by changing the host, username, password, and database properties to match the credentials of your database, like so:
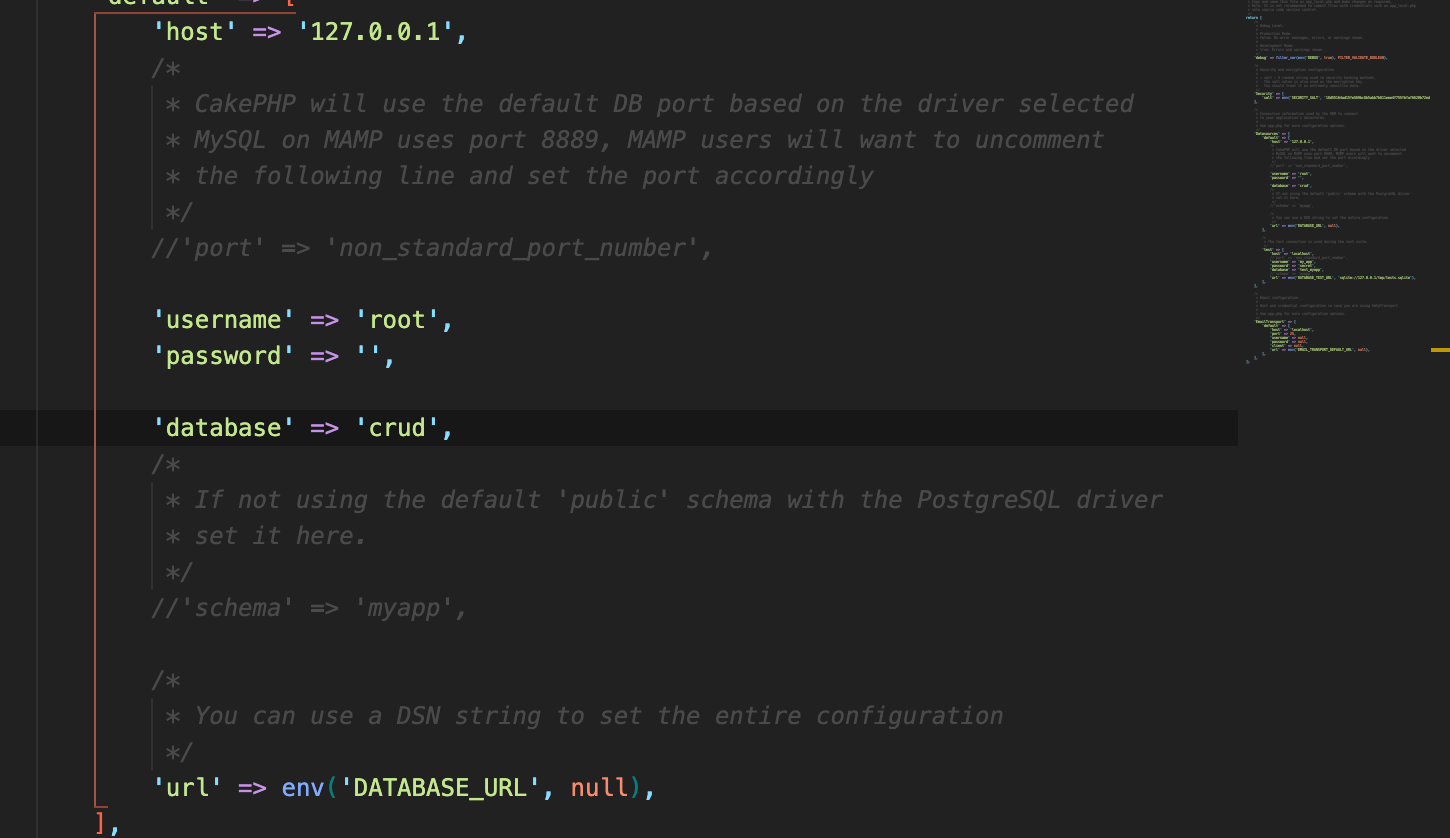
From the image above, the host was changed to 127.0.0.1
, the username to root
, the password was left blank, and the database was set to the one created earlier.
Set up the database for the project
To begin, we need a database with a table to store information about users. Create a database. You can name it anything, but I'm naming mine "crud". The next thing is to create a new table in your database called data
using the migrations feature in CakePHP. The table needs to contain the following fields:
- id: This field will serve as the unique identifier for each user. It should have a type of integer and be the table's primary index, with an auto-increment attribute attached to it.
- name: This field will store the name of the data input. It should have a data type of varchar with a size of 255.
- email: This field will have a datatype of varchar
- phone_no: This will also have a datatype of varchar
To do this, open up the terminal and run this command:
This will create a migrations file in config/Migrations/ ending with _CreateData.php. Open that file in your preferred text editor or IDE and replace the body of the change()
function with the following:
Next, run this command to run the migration:
This will create a table called data
in the database.
Now, start the development server in the terminal by running this command:
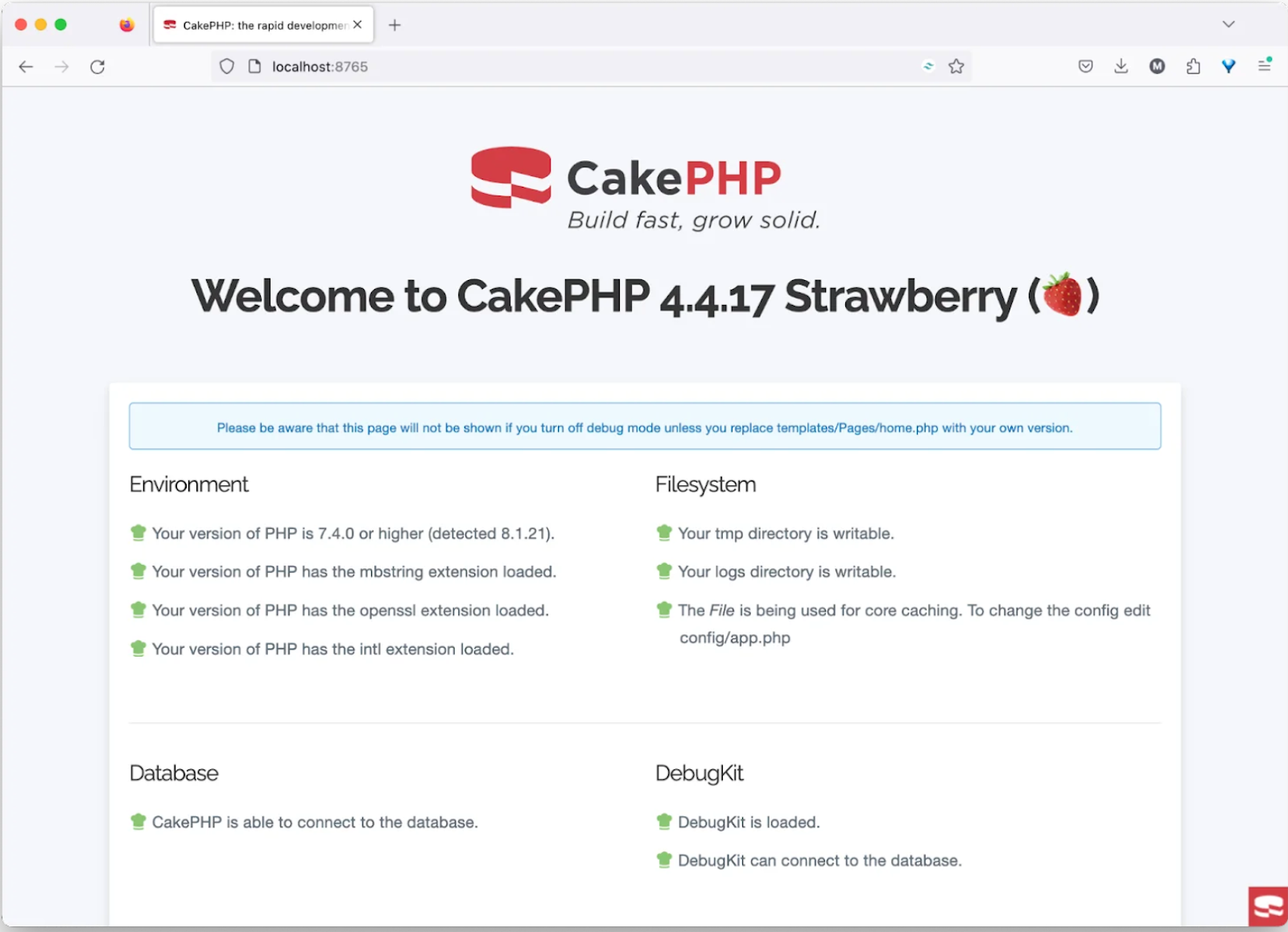
Create a model and an entity
Creating and configuring the model and entity will be the next step. A model contains the information of the table where we will perform CRUD operations. The entity defines the columns for value assignment.
To create a model, navigate to the src\Model\Table directory and create a file called DataTable.php. Then, paste the following code into the file:
The code above defines a model class named DataTable
. This class represents the application's database table, data
. It initializes the table's configuration, specifying its name, display field, and primary key. It also defines validation rules for the name
, email
, and phone_no
fields, ensuring data integrity and adherence to specified constraints.
Moving on to create an entity, this time inside the src\Model/Entity folder, create a file called Data.php and paste the following into the file:
The code above defines an entity class named Data
, that represents an individual record in a database table. The entity has properties for id,
name
, email
, and phone_no
, each with specific data types.
Create the controller
The controller governs the application flow. Inside this controller file is where the CRUD methods will be. These methods handle create
, read
, delete
, and update
methods. To create a controller, in src\Controller create a file called DataController.php, and paste the following code into the file:
So here's a breakdown of the CRUD methods in the controller:
- Create: The
add()
method is responsible for creating a new record. It first creates an empty entity usingnewEmptyEntity()
, then patches the entity with data from the request usingpatchEntity()
, and finally saves the entity to the database using save() - Read: The
index()
method retrieves all records from the database usingpaginate()
, and theview()
method retrieves a single record by its ID usingget()
- Update: The
edit()
method retrieves a record by its ID usingget(),
patches the entity with data from the request usingpatchEntity(),
and then saves the updated entity to the database usingsave().
- Delete: The
delete()
method retrieves a record by its ID usingget()
and then deletes the record from the database usingdelete().
Create the templates
The CRUD operations will need view files (templates) to achieve this. Navigate to the templates folder and create a folder called Data. Inside this data folder, create four files: add.php, edit.php, index.php, view.php.
The add.php creates a user interface for adding new data records to a database table. The edit.php creates a user interface for editing an existing data record. The index.php creates a template to display a list of data records in a tabular format. The view.php creates a template to display the details of a single data record.
Paste the following into add.php:
For edit.php, paste this:
For index.php, paste this:
For view.php, paste this:
Test the application
Refresh the browser so you can see the changes. After that, you can check out the CRUD project by navigating to this link http://localhost:8765/data. Create a new data by clicking on the New Data button, fill out the details. You can also view, edit, and delete data, like the animation below.
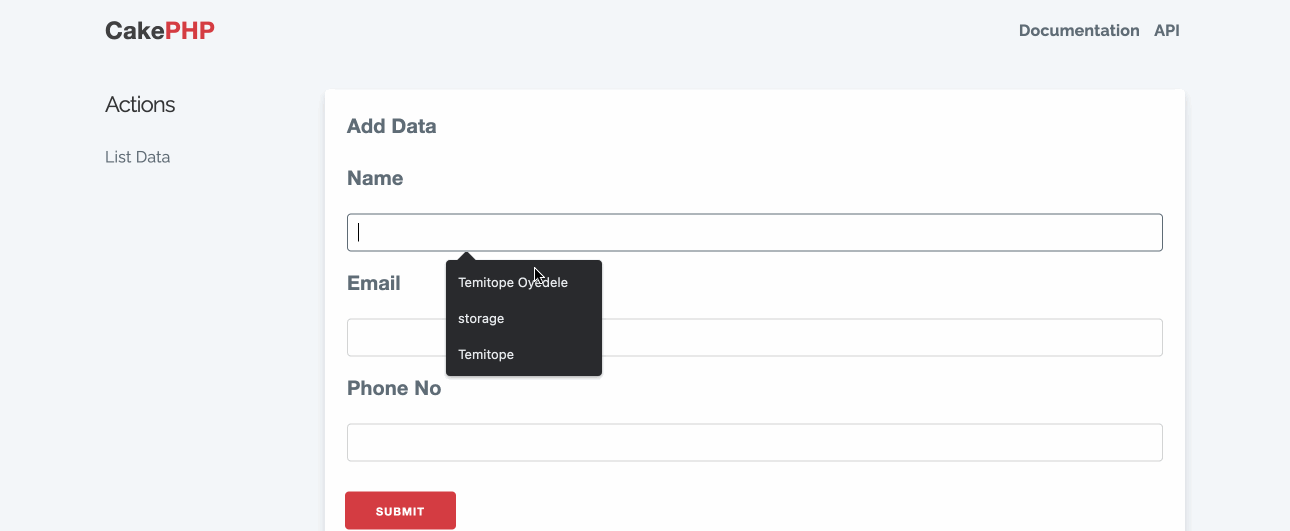
That's how to implement CRUD operations in CakePHP
So, in this article, we looked at how to perform crud operations in CakePHP. Understanding how to create, read, update, and delete data is fundamental to building robust, secure, and user-friendly web applications. CakePHP's built-in ORM (Object-Relational Mapping) system simplifies database interactions. Please share if you found this helpful!
Temitope Taiwo Oyedele is a software engineer and technical writer. He likes to write about things he’s learned and experienced.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.