How To Initiate a Voice Call in CakePHP With Twilio Programmable Voice
Time to read: 3 minutes
How to Initiate a Voice Call in CakePHP With Twilio Programmable Voice
Developers and businesses often need to integrate voice communication into their applications. Whether it’s for customer support, appointment reminders, or notifications, Twilio’s Programmable Voice API provides a powerful solution.
In this tutorial, you’ll learn how to initiate voice calls within a CakePHP application using Twilio Programmable Voice. We'll cover everything from buying a phone number on your Twilio account and configuring your CakePHP environment to making the actual voice call.
Prerequisites
Before we begin though, ensure that you have the following:
- Basic knowledge of PHP and web development concepts
- PHP 8.2 installed
- Composer installed globally
- A Twilio account (either free or paid). If you are new to Twilio, click here to create a free account.
Buy a phone number
The first step in initiating a voice call is to have a voice-enabled phone number. Login to the Twilio Console, and on the left side click on manage, select buy a number, and choose from a list of numbers you prefer.
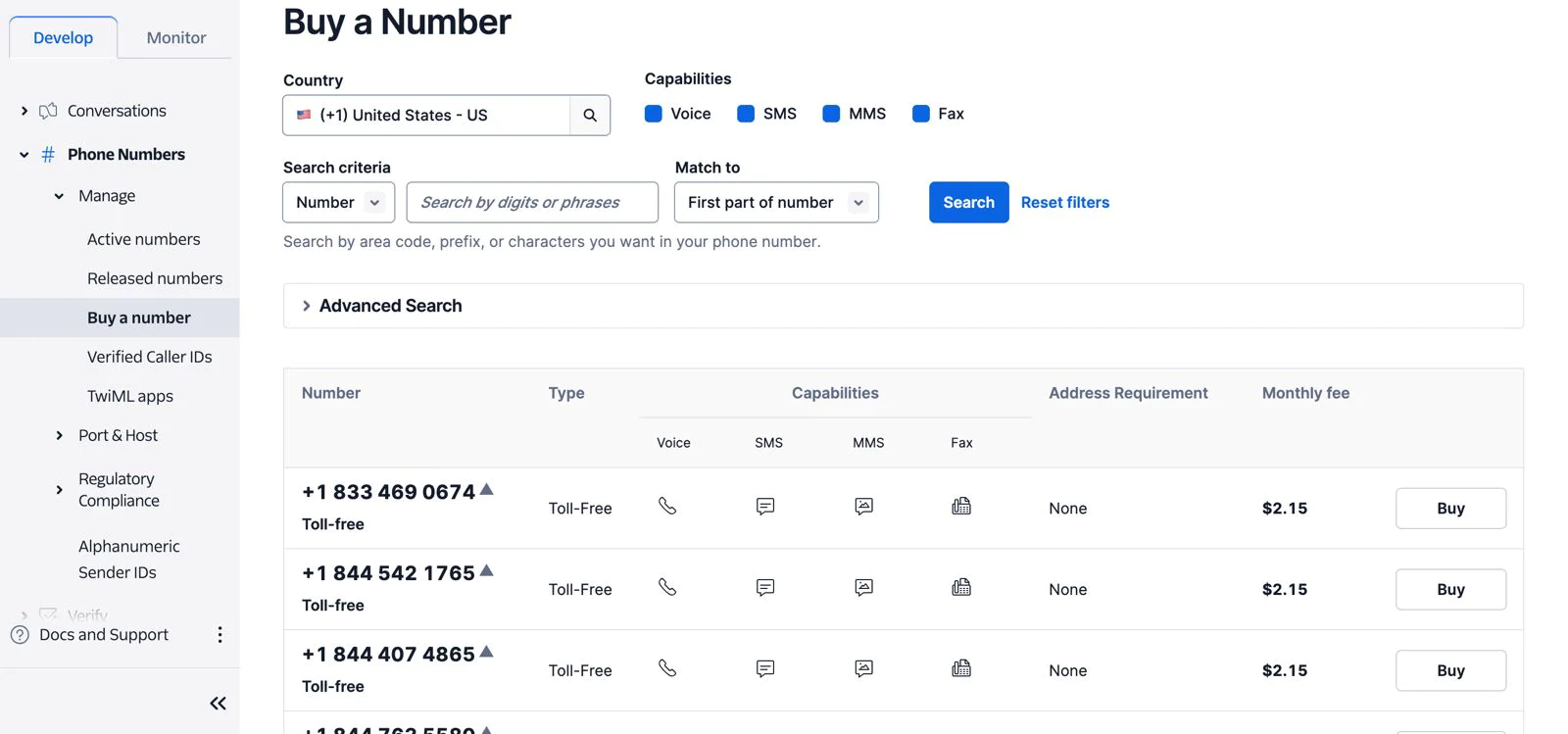
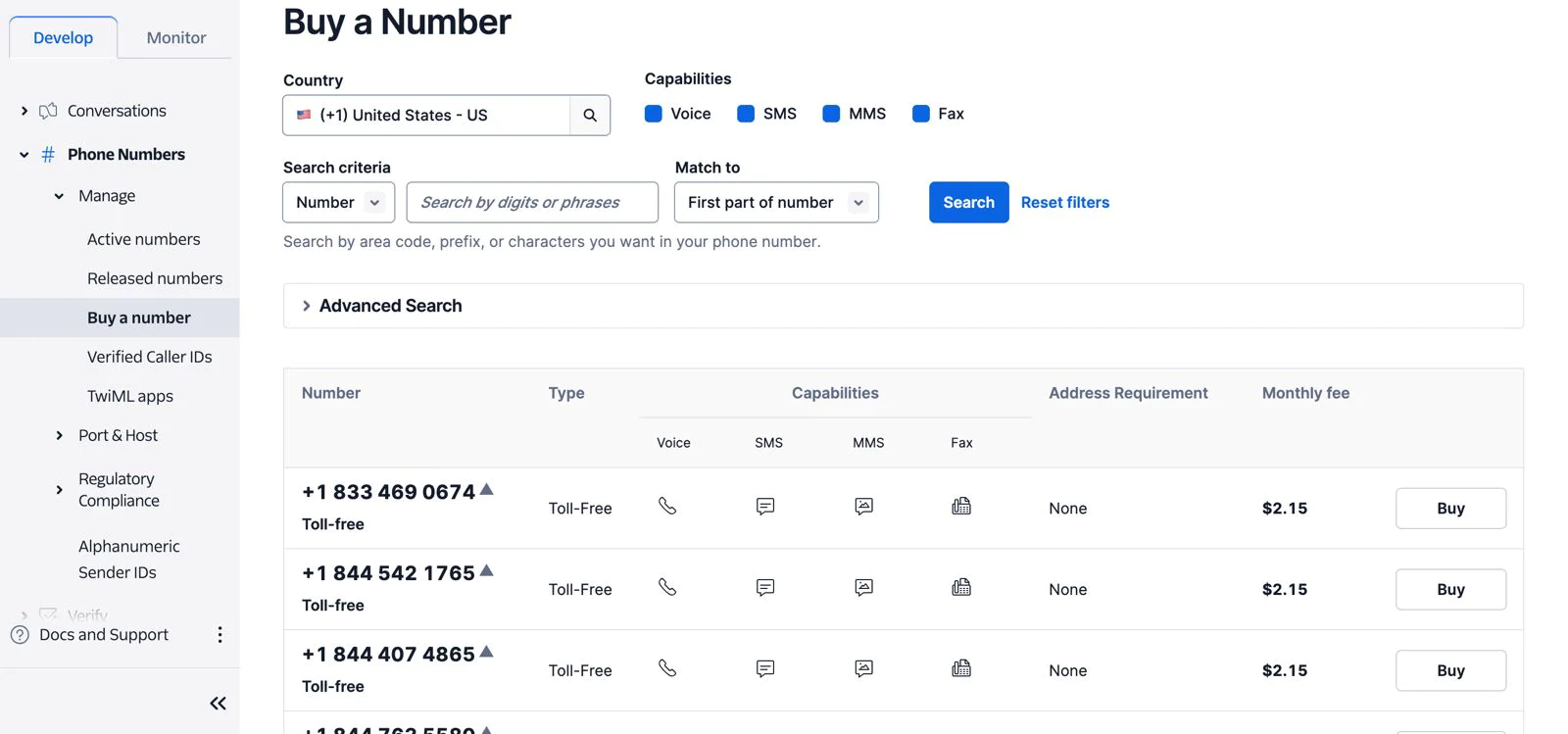
Bootstrap a new CakePHP application
To install CakePHP, navigate to the folder where you want to scaffold the project and run these command:
When asked "Set Folder Permissions ? (Default to Y) [Y,n]?", answer with Y.
The new CakePHP project will be available in a directory named cakephp_twilio_voice, and you'll have changed into that directory.
Install the Twilio PHP Helper Library
We next need to install the Twilio Helper Library for PHP. This package provides convenient methods for interacting with Twilio's APIs, including Programmable Voice. To install it, run the following command in your terminal:
Register environment variables
Copy .env.example, as .env inside the config folder by running the following command:
You then need to add your Twilio credentials by adding the following to the end of the file.
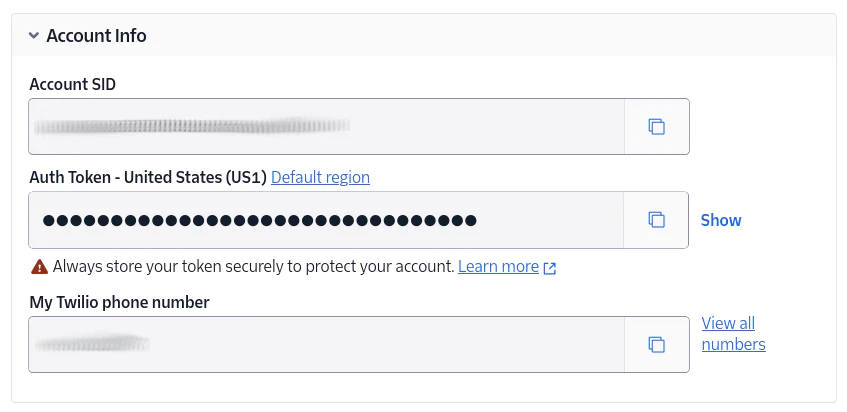
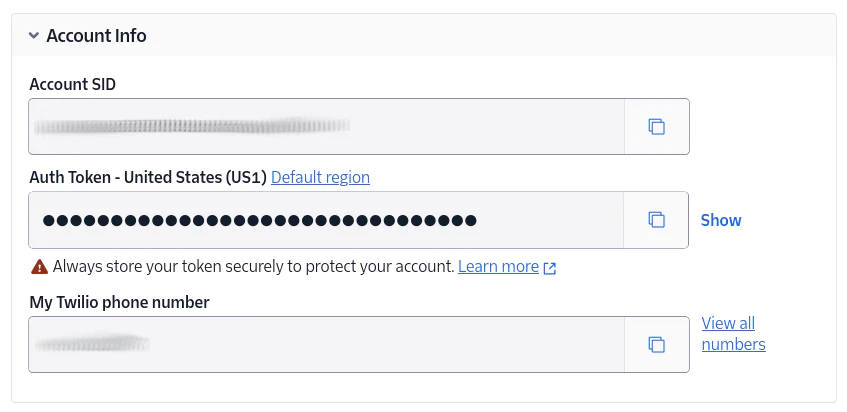
Then, go back to the Twilio Console that you opened in your browser, earlier. There, from the main dashboard copy your Account SID, Auth Token, and phone number. Then, replace the <<your_account_sid>>
, <<your_auth_token>>
, and <<your_twilio_phone_number>>
placeholders respectively, with the respective details which you just copied.
Then, navigate to config/bootstrap.php and uncomment the following:
Create the template
Next, you need to create the app's template to render a form for entering the recipient's phone number. To do so, navigate to the templates folder, and inside it create a folder called Voice. Inside the just created Voice folder, create a file called index.php and paste this into the file:
Create the controller
Next, you need to create a controller to handle the form submission. When the form is submitted, we should use Twilio's PHP Helper Library to initiate a voice call to the entered phone number.
Open up the terminal once again, navigate to the project directory, and run this command:
This command creates a file called VoiceController.php inside the Controller folder Open up the just created VoiceController.php, and add the Twilio PHP Helper Library:
Next, add this function to the class:
If you noticed in the code a response message was included so when the call is initiated we get a message stating an OTP. This is just for testing purposes.
Configure the route
Finally, we need to configure the route for the form action in our routes configuration. The route should direct requests to the initiateCall()
function of the VoiceController.
To do that, navigate to config/routes.php and inside the $routes->scope()
method, add the following:
Test that the application works
And that's it! With these steps, you can initiate a voice call from your CakePHP application using Twilio Programmable Voice. Start up your development using the command below in your terminal.
Using a web browser of your choice, visit http://localhost:8765/voice/.
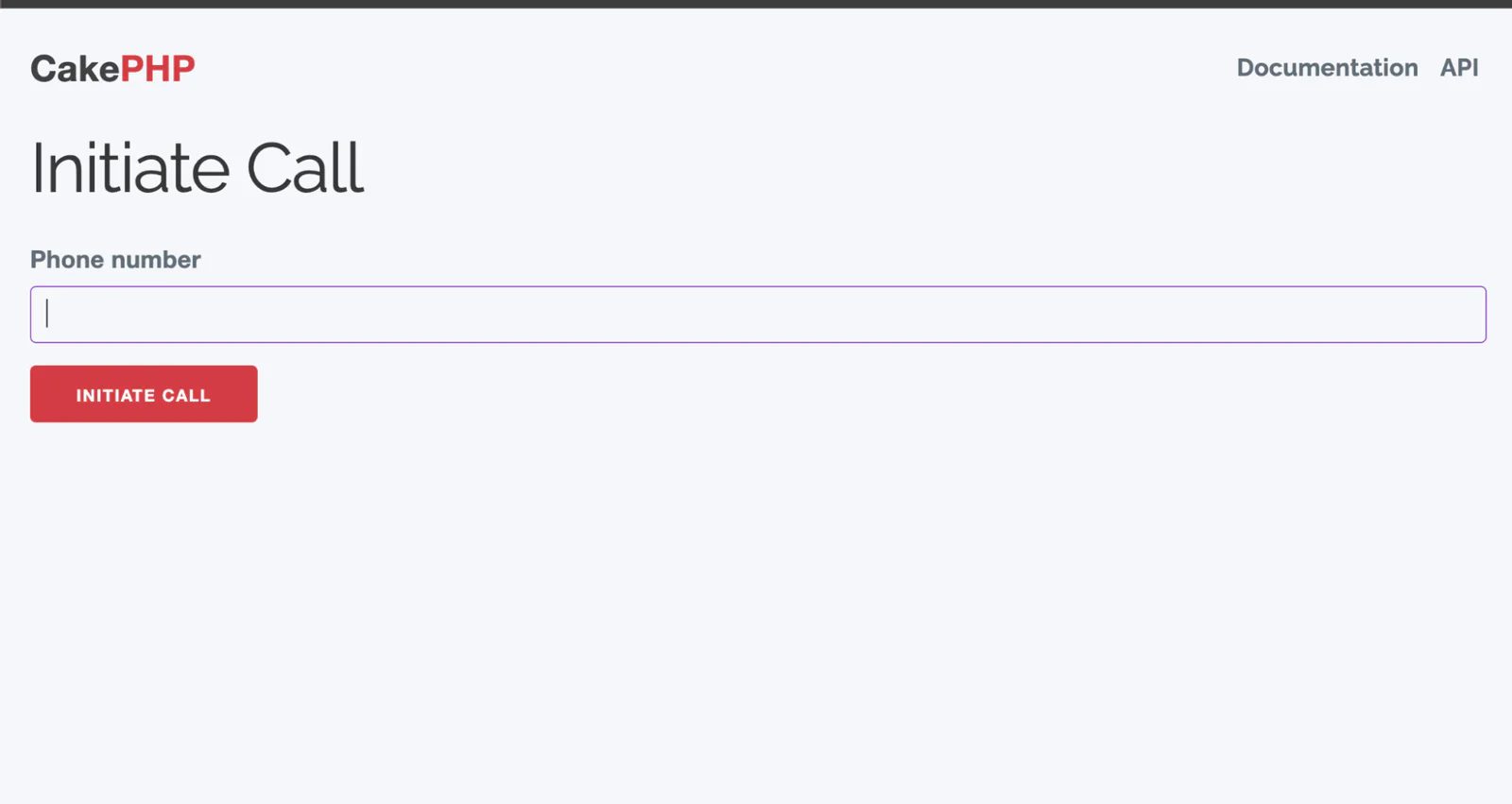
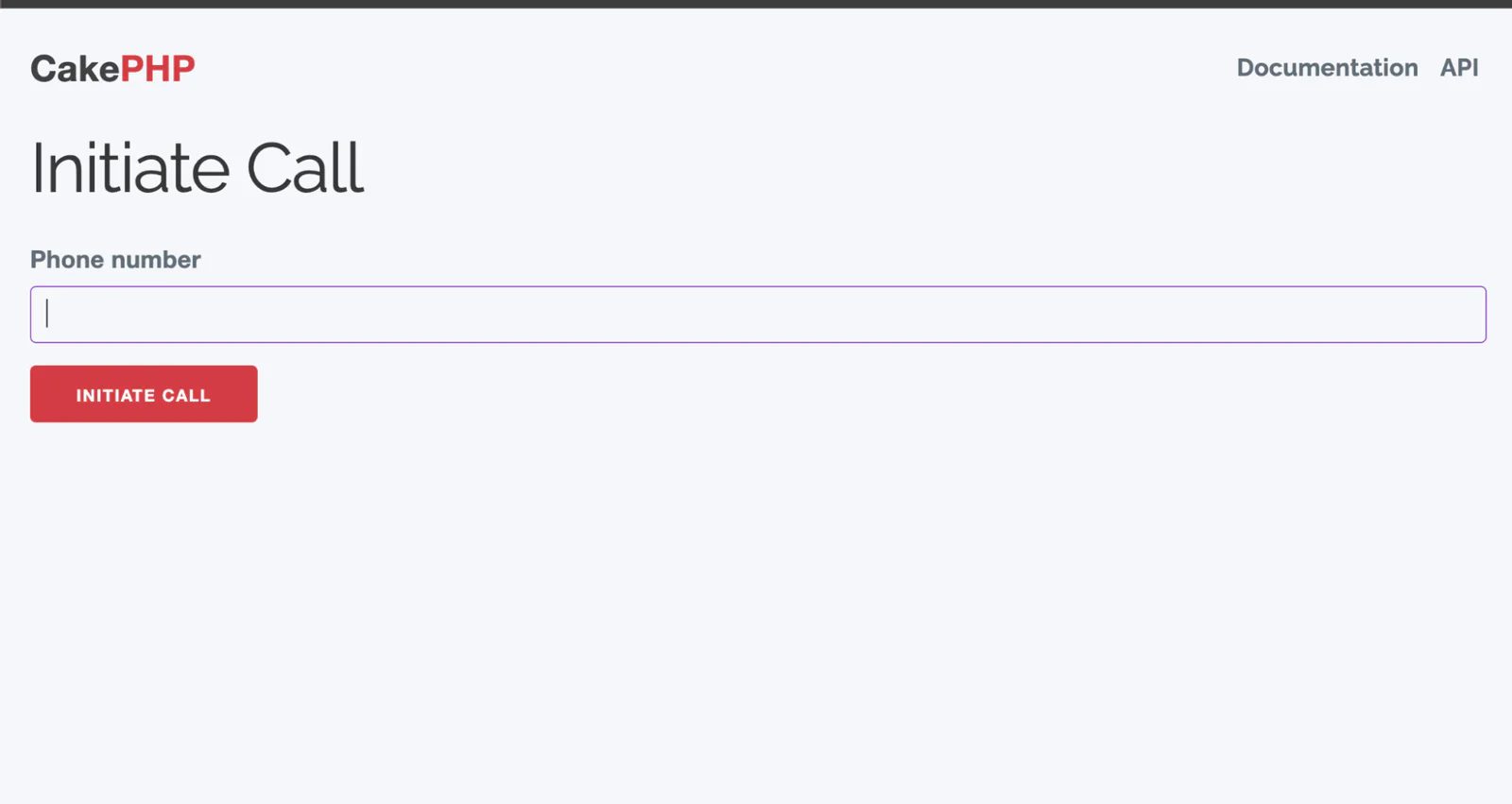
After submitting the form, the user will see a green confirmation popup saying, “Call initiated successfully”.
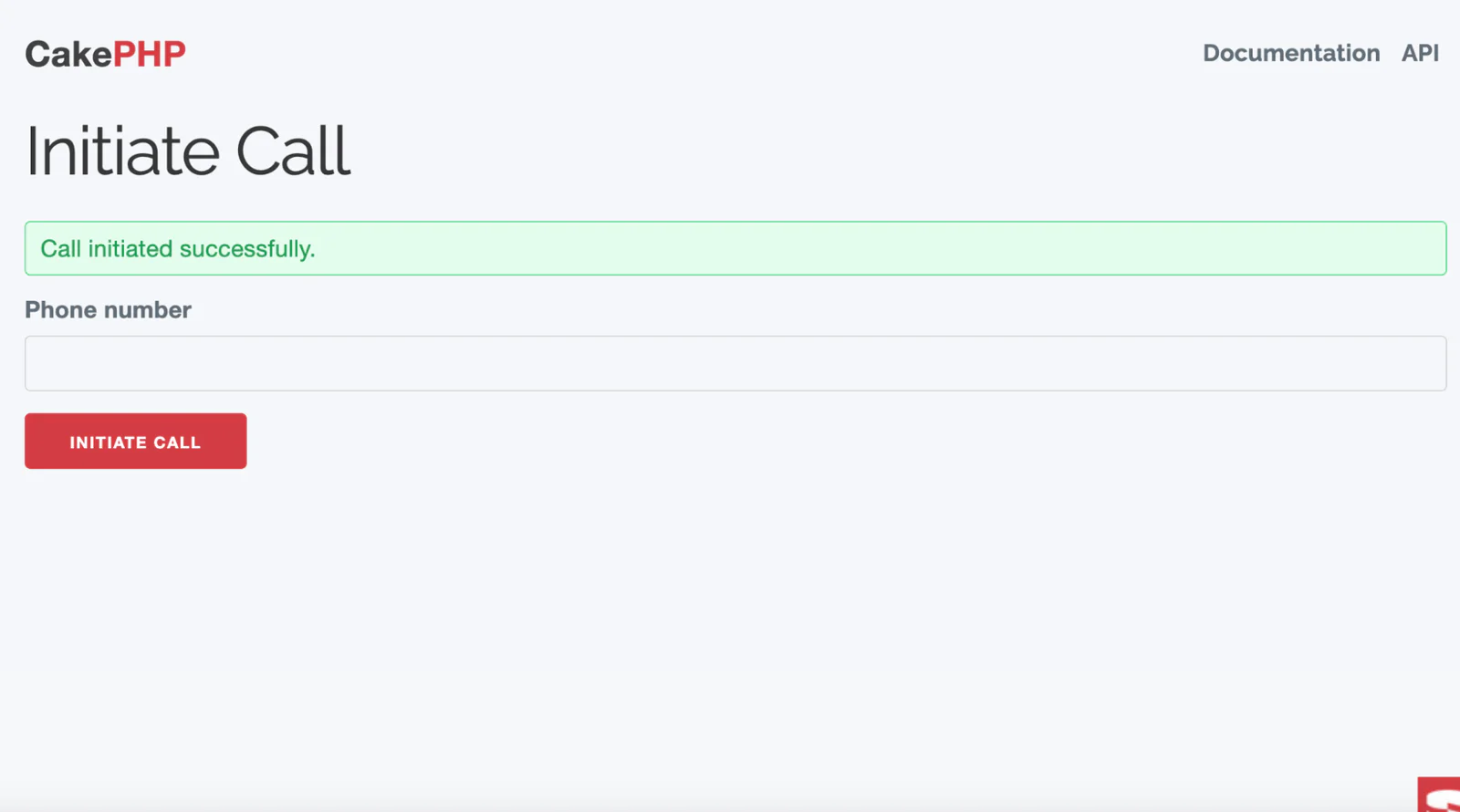
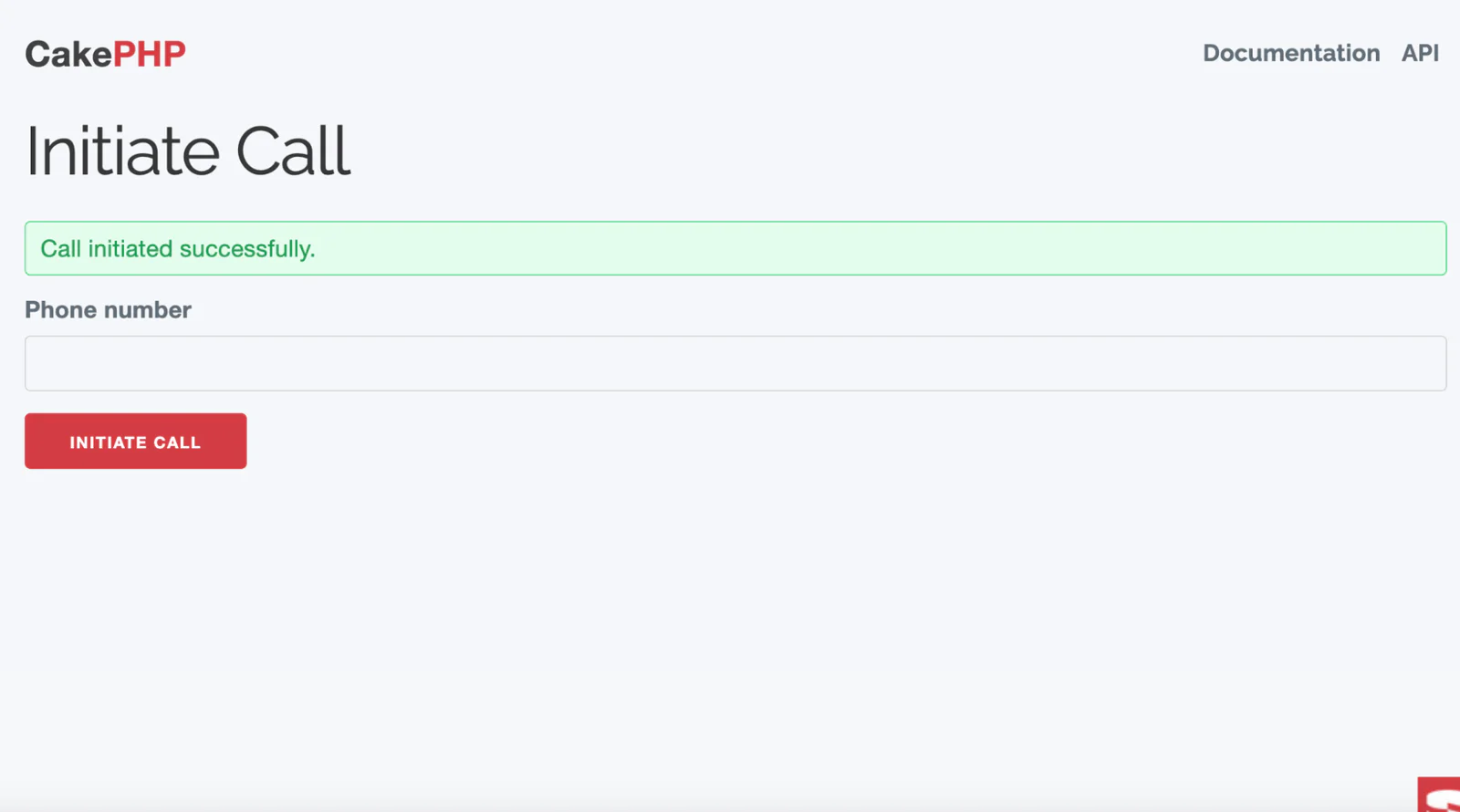
Afterwards, you'll receive a phone call containing your one-time passcode (OTP) on the provided number.
Congratulations! You just initiated a voice call in CakePHP. Easy right? That's because the Twilio Programmable Voice makes it easy to make outbound calls.
Conclusion
In this tutorial, you learned how to initiate a voice call in CakePHP with Twilio's Programmable Voice API. Each of these steps plays a crucial role in enabling your application to successfully initiate a voice call.
It's important to understand that while this guide provides a basic overview, real-world applications may require additional steps and configurations based on specific use cases and requirements. Happy coding!
Temitope Taiwo Oyedele is a software engineer and technical writer. He likes to write about things he’s learned and experienced.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.